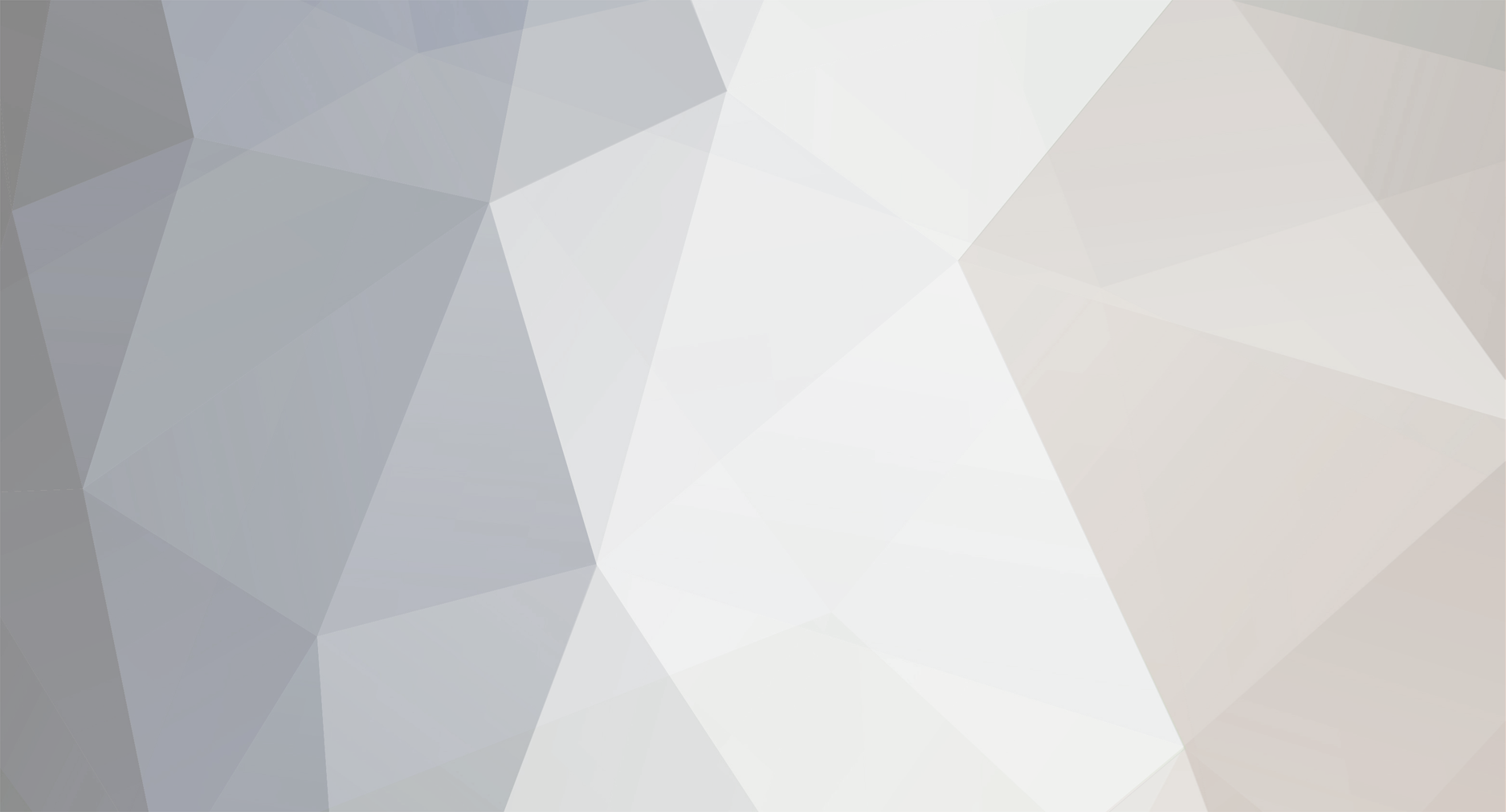
wickning1
Members-
Posts
405 -
Joined
-
Last visited
Never
Everything posted by wickning1
-
Let's try this and see what happens. Put "error_reporting(E_ALL);" at the top of your script, and remove the "or die()" from the mysql_connect() call.
-
Some people upgrade from 4.0 to 5.0, but you're right, it should be spitting out an error. Something must be crashing the thread because it should be printing the "after connection" part no matter what.
-
The only real way to find out if a script is working is to try it out and see if you get what you expect. If you have a syntax error, it should come up and tell you so when you try to access the page. You can always set up a test server, even on your home machine. You can try a XAMPP install: [a href=\"http://www.apachefriends.org/en/xampp.html\" target=\"_blank\"]http://www.apachefriends.org/en/xampp.html[/a]
-
Try resetting your password using: [code]SET PASSWORD FOR youruser@localhost = OLD_PASSWORD('yourpw')[/code] See this for more information: [a href=\"http://dev.mysql.com/doc/refman/5.0/en/php.html\" target=\"_blank\"]http://dev.mysql.com/doc/refman/5.0/en/php.html[/a]
-
Sounds like your MySQL version doesn't support subqueries. What version are you running? Consider upgrading to 4.1 or 5.0. Until then you will probably have to do this on the PHP end. I can't think of a pre-4.1 solution.
-
How to get last results out of my DB in order
wickning1 replied to Toni_montana's topic in PHP Coding Help
If your MySQL is 4.1 or greater you should be able to add another ORDER BY, basically a subquery: [code](SELECT * FROM table ORDER BY rating DESC LIMIT 10) ORDER BY rating[/code] I think that works but I can't remember trying it. If it doesn't, you can just make it a real subquery and put it in the FROM: [code]SELECT f.* FROM (SELECT * FROM table ORDER BY rating DESC LIMIT 10) f ORDER BY f.rating[/code] -
[code]SELECT t.person_id, t.date_started FROM table_name t INNER JOIN ( SELECT person_id, MAX(date_started) as max_date FROM table_name GROUP BY person_id ) f WHERE t.person_id=f.person_id AND t.date_started != f.max_date[/code] Note: If there are two entries with the same person and same date (down to the second), and the date is the most recent, then this will skip both entries.
-
Try using DISTINCT, e.g. [code]SELECT DISTINCT sport FROM articles[/code]
-
HELP!! how to retrieve data from database with encryption..
wickning1 replied to AeonE's topic in PHP Coding Help
The code for creating a user: [code]<?php $host = ''; $uname = "root"; $pass = ""; $database = "db"; $tablename = "login"; $username = $_POST[customerId]; $password = $_POST[password]; $enc_pwd = md5($password); $connection = mysql_connect ($host,$uname,$pass) or die("Database connection failed, please re-check!!"); $result = mysql_select_db($database) or die ("Database could not selected, please check again!!"); $query = "INSERT INTO $tablename VALUES('$username','$enc_pwd')"; //change $password to $enc_pwd for encrypted password!!! $result = mysql_query($query); if (!$result) { die ("query failed, please check again <br>"); } ?>[/code] -
$text = preg_replace('/<o:.*?\/>/', '', $text);
-
HELP!! how to retrieve data from database with encryption..
wickning1 replied to AeonE's topic in PHP Coding Help
Your insert statement is inserting $password when it should be inserting $enc_pwd. You have a comment there that says to switch it out, but the code you posted doesn't have them switched out. -
Looks right to me, as much as you posted. Note that you can do joins in a delete statement so you don't actually have to make a lot of queries. But yeah, it can get complex quickly. I always make sure to have a current reference diagram on hand until I really know the system (and it stops changing rapidly).
-
You should fetch all rows WHERE id=$id and then go through them in php and determine what to do with each one depending on what fieldname is set to.
-
The BLOB type is limited to 65000 characters (about 64kB). Use MEDIUMBLOB for up to 16MB or LONGBLOB for up to 2GB.
-
I use Visio on Windows, it was free for me because of college. :) It doesn't exactly do traditional ER modeling, but it does have a nice setup that serves the same purpose. There's a drawing program for linux called Dia that I use to draw real ER diagrams. It doesn't have a lot of nifty features, but it makes it possible to draw a competent diagram with some effort. Also it's open source and free as in beer. [a href=\"http://www.gnome.org/projects/dia/\" target=\"_blank\"]http://www.gnome.org/projects/dia/[/a]
-
It's possible the cookie is not taking. They're notoriously unreliable. The other possibility that comes to mind is that the credits column could be set to an integer type, which would prevent adding 0.5. Maybe double check that.
-
It should be sitting in the $argv global array. See here for more info: [a href=\"http://us2.php.net/manual/en/features.commandline.php\" target=\"_blank\"]http://us2.php.net/manual/en/features.commandline.php[/a]
-
A PHP script can be set up to feed the user a file download INSTEAD OF a web page. Set the content type with the header() function. Then use PHP to open the file on the local machine, read it in and output it to the user.
-
Look through the tutorials and find one on Pagination.
-
It really all depends on the specifics of your problem. There are a lot of strategies for increasing performance but we need to know what the data looks like. Spend a couple minutes describing your system, the data you store, the tables you've created, and what you'd like to do with them. Sending a query to the database is one of the most expensive operations in a PHP script, especially if the database is on another server, so yes, it is important to try to limit the number of queries. However, you should be able to fire off at least a couple hundred per page without killing your site, as long as they are simple queries.
-
Just make sure the host provides the technologies you plan to use, and has good pricing for a variety of service levels. It's hard to predict how much power/bandwidth you're going to need for a site, especially if you don't know if it will take off and become popular. Community sites in particular; they usually either experience exponential growth (a large user base attracts more users) or stagnate and die off. You should start off small and upgrade your service as needed. Make sure you write your web application in such a way that upgrading or moving servers will be easy. You'll probably want to make it PHP4 compatible as most shared-server hosts are still using it. If you rent a whole machine then you usually have the freedom to install whatever you want, but that can be expensive. Personally I would recommend a host that can offer you a linux/unix/bsd server running at least Apache, MySQL 5, Perl, and PHP 5. SSH access is often helpful as well. Be sure to consider price, storage, server specs, number of other users on your server(s), overall transfer limits, what happens when your users bust your limit, uptime, and turnaround on your support requests.
-
Shuffle really only makes sense if you're going to display more than one element from the array you're shuffling. If you're just returning one element, as it appears you are, then you are just picking a random element, not shuffling. Shuffling is like shuffling a deck of cards. You will never get two aces of hearts after shuffling a single deck. If you want it to behave like a shuffle, i.e. you don't want to repeat anything, you'll have to come up with a solution for keeping persistent data (probably the data would be a full shuffled list and an index of where you currently are). You can store it with the user's session, put it in a cookie, or keep sending it through as form input. To answer your aside, using ORDER BY RAND() on your query is exactly the same as using shuffle() on a PHP array. Doing it in MySQL is likely to perform better.
-
Your bracketing is all wrong. The correct way to access form input is $_POST['val1'] or $_POST['calc'], etc. Yours are all horribly disfigured.
-
You can achieve something close to overloading since parameters are optional. For instance, in your example, __construct() is unnecessary, because __construct($id) will accept both "new test()" and "new test(12)". $id will simply be unset in the first case. It's definitely not as flexible as true overloading, but like daiwa says, you can create a factory and pass it off based on the input you got. You can do type checking too with functions like is_numeric, is_array, is_object, and the language construct "instanceof" that checks if an object inherits a certain class (i.e. if ($inputobject instanceof myclass) {})
-
Not really, no. phpMyAdmin can do it for you, or if you have ssh access to the database machine you can use mysqldump. Otherwise you just need to write it for yourself.