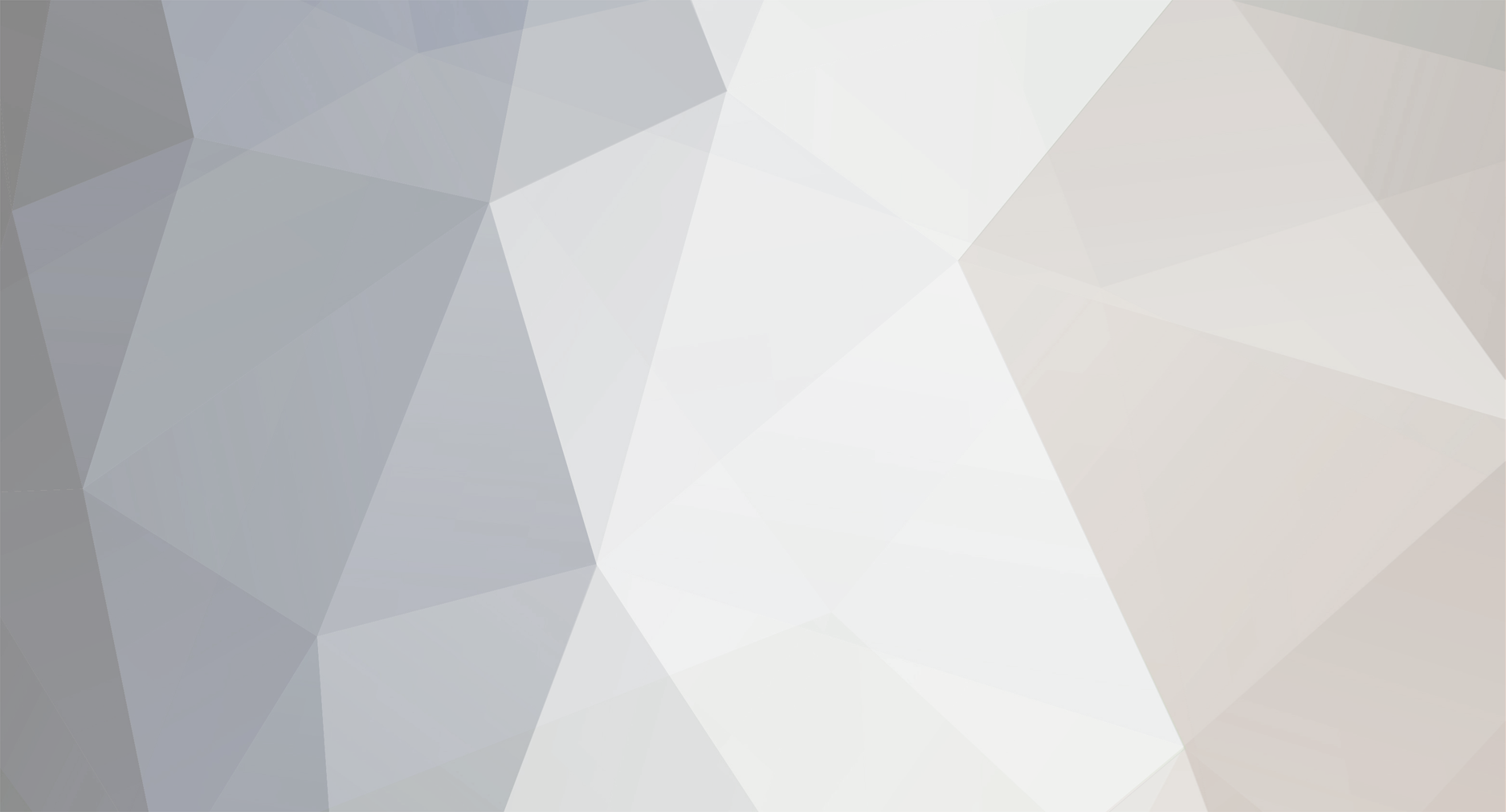
wickning1
Members-
Posts
405 -
Joined
-
Last visited
Never
Everything posted by wickning1
-
Make sure the array you want to sort on is listed first, and be careful to choose numeric sort or string sort. String sort is the default. [code]$plancost = array(2,3,1); $supplier = array('sa','sb','sc'); $plan_name = array('pa','pb','pc'); $add_on = array('aa','ab','ac'); $hosp_cover = array('ha','hb','hc'); $excess = array('xa','xb','xc'); $plans = array('ca','cb','cc'); array_multisort ($plancost, SORT_NUMERIC, SORT_ASC, $supplier, $plan_name, $add_on, $hosp_cover, $excess, $plans); echo '<pre>', print_r ($plans, true), '</pre>'; echo '<pre>', print_r ($supplier, true), '</pre>'; echo '<pre>', print_r ($plan_name, true), '</pre>'; echo '<pre>', print_r ($add_on, true), '</pre>'; echo '<pre>', print_r ($hosp_cover, true), '</pre>'; echo '<pre>', print_r ($excess, true), '</pre>'; echo '<pre>', print_r ($plancost, true), '</pre>';[/code]
-
Reducing storage cost is not just about hard drive space! First, on indexed columns saving space is very important because you want to keep as many indexes in main memory as possible. As your database grows, more and more indexes will be forced to reside on the disk during run time, which can severely hamper performance. Second, disk reads are the most time consuming operation in a database system. If you optimize for storage and reduce your static row length by 50%, operations on that table will happen twice as fast. Sure, lots of little systems will never reach any kind of performance limitation, but that's no reason to ignore performance completely. Now, for the topic - if your application is checking $var == "yes" in 5 million places then it's not reasonable to change the column to char(1) or bit or tinyint. What is reasonable is enum. You get the performance of tinyint without having to change code. The column acts exactly as if it were varchar, but you can only put in "yes" or "no". If you ever need to add another value, you alter the column so that it supports it. Personally when I store booleans I use tinyint 0 or 1.
-
Yeah, I like Perl quite a bit too. That's why when I started with PHP I rolled out my own database class that works like DBI. I parse out the placeholders myself.
-
It is a scoping thing. You need to figure out a way to have access to it all through your program, a way that seems elegant to you. You can use global $mysqli at the top of every function, that's pretty easy. What I did was build a class around mysqli that handles everything, and it stashes the link in a global variable, so that when you instantiate again, it can just check for the global variable and skip connecting.
-
Just change two lines: $exp_date=$_db->f('exp_date'); $sec_left=$exp_date - $now;
-
You can't use new when you're just declaring class variables. Put it in the constructor instead (PHP5 code): [code]class Test { private $user; function __construct() { $this->user = new User("geneanthony", "hea9671"); } }[/code]
-
Temporary table and persistant connection
wickning1 replied to realjumper's topic in PHP Coding Help
If I needed a table to persist across page loads, I would just create the table and drop it with a real DROP TABLE command. You can always add some cleanup somewhere to make sure you delete old temp tables that are accidentally still around. A persistent connection could indeed extend the life of the table, but I'm not sure if any other thread has permission to access it, and you can't be sure which connection the new page load will get from the persistent pool. You also can't be sure when or if the connection will be regenerated, dropping the table. Not solid enough to depend on. -
He'll probably be fine, most web hosts have magic quotes turned on (to help cover their butts from a security standpoint). Magic quotes are so evil. If you don't know already, do yourself a favor, google a bit, learn what magic quotes are, and learn how to deal with/without them. Maybe I'll write a tutorial I can link to.
-
Yes, both queries I wrote for you will do the exact same thing, have the exact same performance. They are just two ways to write the same thing. FULL-TEXT searches will automatically be ordered by relevance, so the more times each word matches (plus some other stuff), the higher it is in the return set. I'm not sure how well it will behave when you're searching two tables, but it probably handles it fine. It does take quite a bit of work to try and implement your own relevance rules. You basically need to create your own index and forget FULL-TEXT searching.
-
Help!! Trouble getting answers using sub-queries!!
wickning1 replied to noisyassassin's topic in MySQL Help
Looks like homework, so instead of one solution, I'll give you plenty to think about. I'm only going to answer Question 1 for now though. This is probably the first one somebody would come up with. It has a subquery in the WHERE: [code]SELECT CONCAT(o.forename, ' ', o.surname) AS name FROM owner o INNER JOIN pet p ON p.ownerID=o.ownerID INNER JOIN pettype t ON t.pettypeID=p.pettypeID WHERE t.pettypename = 'Cat' AND o.ownerID NOT IN ( SELECT DISTINCT p1.ownerID FROM pet p1 INNER JOIN pettype t1 ON t1.pettypeID=p1.pettypeID WHERE t1.pettypename = 'Dog' )[/code] This is another version, with the subquery in the FROM. It more closely resembles set subtraction: [code]SELECT CONCAT(o.forename, ' ', o.surname) AS name FROM owner o INNER JOIN ( SELECT DISTINCT p.ownerID FROM pet p INNER JOIN pettype t ON t.pettypeID=p.pettypeID WHERE t.pettypename = 'Cat' ) c ON c.ownerID=o.ownerID LEFT JOIN ( SELECT DISTINCT p.ownerID FROM pet p INNER JOIN pettype t ON t.pettypeID=p.pettypeID WHERE t.pettypename = 'Dog' ) d ON d.ownerID=o.ownerID WHERE d.ownerID IS NULL[/code] The two solutions above are MySQL solutions, here's an Oracle solution (since it looks like the homework calls for Oracle). The syntax may still be incorrect, my Oracle is rusty: [code]SELECT o.forename||' '||o.surname AS name FROM owner o INNER JOIN ( SELECT p.ownerID FROM pet p INNER JOIN pettype t ON t.pettypeID=p.pettypeID WHERE t.pettypename = 'Cat' MINUS SELECT p.ownerID FROM pet p INNER JOIN pettype t ON t.pettypeID=p.pettypeID WHERE t.pettypename = 'Dog' ) c ON c.ownerID=o.ownerID[/code] -
if($error==""){ No closing bracket on that. File you gave us has 50 lines, so it's tough to track down a bug on line 61.
-
That's simple, but does a little extra work. As soon as you find one, most of your work is done. Shouldn't be a big deal either way though. [code]$today = mktime(0,0,0); $arr = array(); $adv = 1; for ($d=1; $d<=30; $d += $adv) { $dt = strtotime("+$d days", $today); //get day of week $dow = date('w', $dt); // store dateval in array if (($dow==2) || ($dow==4)) $arr[] = $dt; if ($dow == 2) $adv = 2; if ($dow == 4) $adv = 5; } // print it like Barand showed you[/code]
-
This is purely PHP Help, but try this: [code]$result = mysql_query("SELECT * FROM maps"); while ($row = mysql_fetch_assoc($result)) { echo "Map Name: " . $row['map_name'] . "<br>\n"; $modes = array(); if ($row['mapmode_chaos']) $modes[] = "Chaos"; if ($row['mapmode_sts']) $modes[] = "STS"; if ($row['mapmode_predator']) $modes[] = "Predator"; echo "Modes: " . implode(', ', $modes) . "<br><br>\n\n"; }[/code]
-
Displaying multiple instances of a field under separate distinct field
wickning1 replied to neo926's topic in MySQL Help
Best you can do is use ORDER BY sport, author That way you get: MLB Joe MLB Steve NBA John NFL Mark NFL Matt You'll have to do some post processing in PHP to make sure you print, for instance, MLB only once. Something like: [code]while ($row = mysql_fetch_assoc()) { if ($save_sport != $row['sport']) { echo $row['sport'] . "<br>\n"; $save_sport = $row['sport']; } echo $row['author'] . "<br>\n"; }[/code] -
AND comes before OR in the order of operations. You just need to add parentheses. The way you had it, it was unable to use the join conditions. That's why it timed out your browser. [code]SELECT p.fp_id, t.ft_title, t.ft_id, t.ft_creationdate, t.ft_firstpostername , t.ft_firstposterid, t.ft_postcount , s.fs_id, s.fs_title FROM sed_forum_posts AS p, sed_forum_topics AS t, sed_forum_sections AS s WHERE p.fp_topicid=t.ft_id AND p.fp_sectionid= s.fs_id AND (MATCH (p.fp_text) AGAINST ('admin pro') OR MATCH (t.ft_title) AGAINST ('admin pro'))[/code] This is why I like to use the "INNER JOIN table ON joincondition=1" syntax. It separates your join conditions from the rest of the query, makes it easier to understand what's going on, and harder to mess up. Here is how I would do it (functions identically to what is above): [code]SELECT p.fp_id, t.ft_title, t.ft_id, t.ft_creationdate, t.ft_firstpostername , t.ft_firstposterid, t.ft_postcount , s.fs_id, s.fs_title FROM sed_forum_posts p INNER JOIN sed_forum_topics t ON p.fp_topicid=t.ft_id INNER JOIN sed_forum_sections s ON p.fp_section_id=s.fs_id WHERE MATCH (p.fp_text) AGAINST ('admin pro') OR MATCH (t.ft_title) AGAINST ('admin pro')[/code]
-
To retrieve the strings starting with numeric digits.
wickning1 replied to ganesh_g1982's topic in MySQL Help
Sorry, typo. It's REGEXP, not REGEX. Stupid SQL monkeys. -
AndyB is correct, but I'm not sure that would produce an error. Use this to find out what the error is: [code]mysql_query($query) or die(mysql_error());[/code]
-
[!--quoteo(post=354502:date=Mar 13 2006, 08:35 AM:name=Masna)--][div class=\'quotetop\']QUOTE(Masna @ Mar 13 2006, 08:35 AM) [snapback]354502[/snapback][/div][div class=\'quotemain\'][!--quotec--] That's not really necessary. Just process the results with PHP. [/quote] Bad advice. You should never return more data from MySQL than you are going to use.
-
You can do it in the MySQL query: [code]SELECT IF(mapmode_chaos, 'Chaos', '') as mapmode_chaos, anothercolumn, yetanothercolumn FROM maps WHERE something[/code]
-
Is it producing an error or just not returning data? Please print the query after all the variables have been evaluated.
-
$matched = preg_match_all("{m=more&id=(.*?)>([^<]+?)</a>}", $txtonly, $match); Try that.
-
To retrieve the strings starting with numeric digits.
wickning1 replied to ganesh_g1982's topic in MySQL Help
I thought you wanted things that started with any number. If you want to search for specific numbers, that's exactly the same as with letters. For 1, "SELECT * FROM company WHERE name LIKE '1%'". The MATCH AGAINST syntax only searches for whole words, if you just wanted a substring search, just keep using LIKE: "SELECT * FROM company WHERE name LIKE '%userinput%'" -
The MySQL server should only be accepting connections from your web server. This is part of MySQL's user management. If you do not own the server, ask the owner to secure it for you. That way even if someone knows the user name and password, he still can't get in. Also it should be fairly difficult to get access to your PHP source. An attacker would have to get into the web server first.
-
To retrieve the strings starting with numeric digits.
wickning1 replied to ganesh_g1982's topic in MySQL Help
First question: You can use the REGEX comparison: [code]SELECT * FROM company WHERE name REGEX '[[:digit:]].*'[/code] Second question: Use the MATCH AGAINST syntax, e.g. [code]SELECT * FROM company WHERE MATCH(name) AGAINST ('userinput')[/code] You'll probably want to create a fulltext index to make that fast. -
If you have any access to your database, run this: [code]SET PASSWORD FOR root@localhost = OLD_PASSWORD('yourpw')[/code] If you're not running phpMyAdmin as root, you can change it to whatever you're using.