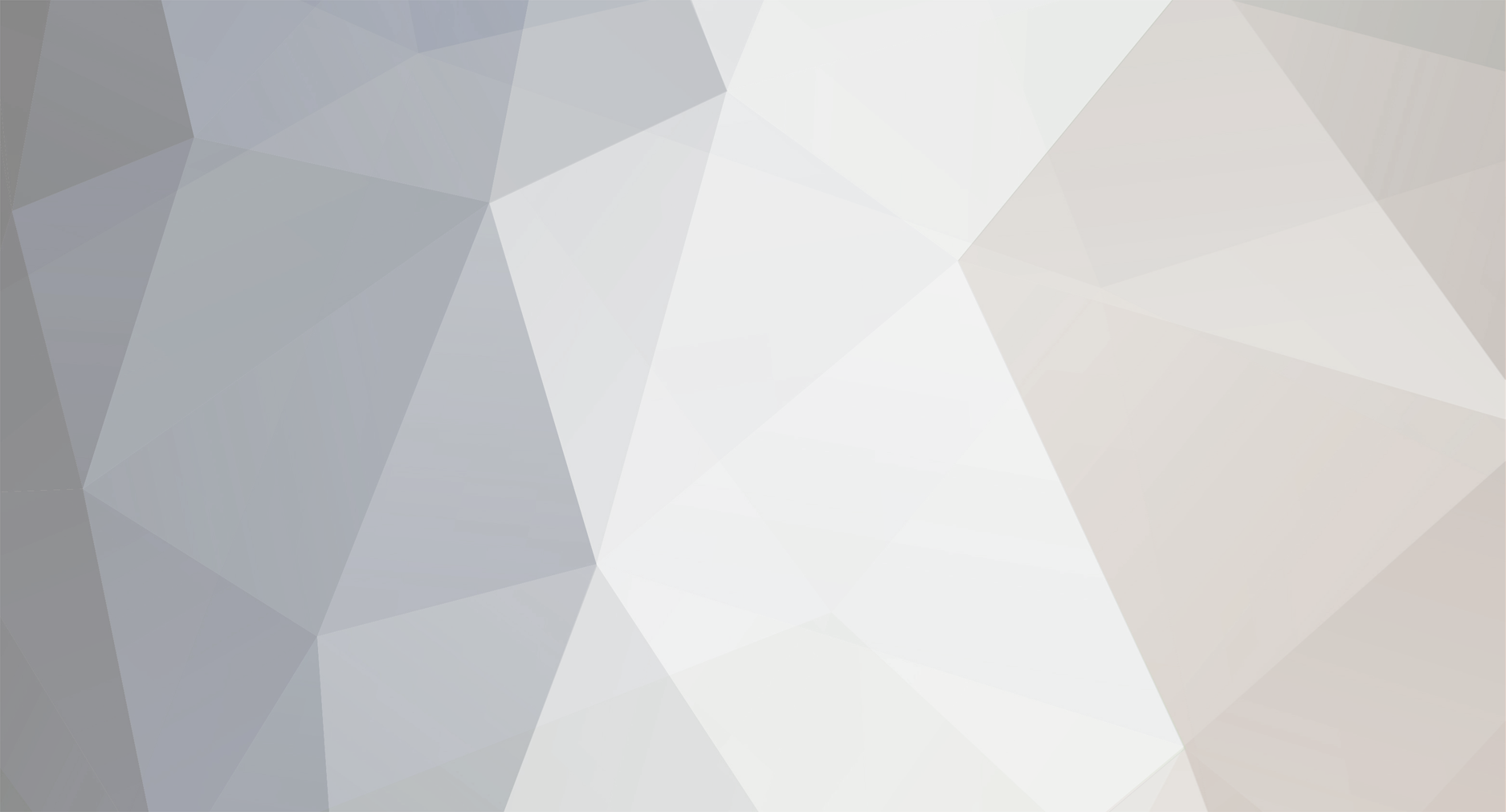
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
It might be easier to get all followups older than 7 days and then filter them in php. Doing it in mysql is not all that easy. You can get mysql to order them by date, which will make the php filtering quite simple.
-
The variable names you are using here [code=php:0]$first=$_POST['name']; $last=$_POST['type']; $phone=$_POST['phone']; $mobile=$_POST['city']; $fax=$_POST['county']; $email=$_POST['email']; $web=$_POST['web']; $description=$_POST['description'];[/code] don't match the variable names you are using here: [code=php:0]$query = "INSERT INTO customers VALUES ('$name','$type','$phone','$city','$county','$email','$web','description')";[/code] If you say [code=php:0]$first = $_POST['name'][/code] then you must use [code=php:0]$first[/code] in your query, not [code=php:0]$name[/code]
-
To add to what Hypnos said, if you want a simple way to store trusted data for the short term, use sessions. With sessions, you can be sure that the data you put in is what you get out. BUT, keep in mind that the name of the session is not 100% secure. Someone can monitor another user's session, take their session name and take over the session. It's not easy but it's possible. But the data inside the session can only be modified by your scripts.
-
If $p is "latest", what does it declare $p as? You can simplify it to [code=php:0]if (empty($_GET['p])) { $p = 'latest'; } else { $p = $_GET['p']; }[/code] Logically that code will produce the same output, but it's a little neater :) It'll also set $p from $_GET['p'] in all cases.
-
fairly hefty query with joins need to limit some data
btherl replied to c_shelswell's topic in MySQL Help
If you have duplicate rows in a table that you don't want repeated, you can use either DISTINCT or GROUP BY to eliminate them. For example. [code=php:0]SELECT DISTINCT purchases.item_price, purchases.qty, ....[/code] [code=php:0]SELECT purchases.item_price, ... GROUP BY purchases.item_price, purchases.qty, ...[/code] For the group by approach, you will need to list all your columns again. I would try distinct first. -
Multiple queries never count as one query in mysql, when using MyISAM tables. So the main risk of the approach you're suggesting is that if one query fails for whatever reason, recovery can be difficult. This approach would be fine for a situation where you can recover by simply dropping a table used for importing, for example. As for achieving it, I'm sketchy on the details, but you should be able to use the mysql command line client something like this: [code=php:0]mysql database < commands.sql[/code]
-
Add [code=php:0]or die(mysql_error())[/code] after your mysql_query() call, like this: [code=php:0]mysql_query("blah blah blah") or die(mysql_error());[/code] And then post what it says.
-
I don't think setting location.href will submit the form values. Try this: [code=php:0]document.order_form.submit()[/code] assuming the form is named "order_form". And be careful, if you have a form element named "submit" then this will fail! The only solution there that I know of is to rename the form element.
-
if (strlen($username) < 5) not working and more problems like this
btherl replied to Mythic Fr0st's topic in PHP Coding Help
This code you have in your last post is correct: [code=php:0]if (isset($_POST['username'])) { $username=$_POST['username']; }[/code] Then strlen($username) will only be less than 5 if the username really is less than 5 characters long. -
Try putting the query in a variable, then printing out out, then running the query. Then you can take a look at the query. [code=php:0]$sql = "UPDATE ... "; echo "About to run $sql\n"; $UpdateDatabase = mysql_query($sql) or die(mysql_error());[/code] If it looks ok and you still get 0 rows affected, try doing a SELECT instead of an UPDATE, to see which rows match the where condition.
-
Hmm.. what if you urlencode the "+" before sending it? + encodes to %2B. If you can send %2B from javascript and receive + in php, then all you'll need to do to fix it is to encode it before sending. A good test would be to use your existing code but hardcode "content=%2B".
-
More detailed errors will definitely help. You might even want to log at important points through the script to a file, and then check that logfile later. Such huge slowdowns are usually caused by network issues.. dns lookup or requests which time out. If none of those are present, then it's possible that there's a database locking issue. MySQL with MyISAM tables can have locking problems if it's under heavy load. Then the query may fail if it can't obtain a lock. More detailed error messages should pick that up if that's the case.
-
"+" happens to be the url encoded value for space. Could there be some url decoding going on (perhaps automatically)? To debug it, I would check first that the + are being sent to update.php, then that the + are being received by update.php (using what jesirose suggested). Once you've found where they are disappearing you can focus in on that.
-
You can increase your memory limit with: [code=php:0]ini_set('memory_limit', '16M');[/code] To increase it to 16 megabytes, for example. I suspect the memory consumption is due to the array of results. I don't see anything else that would consume memory. An option to reduce memory consumption is to write your data to temporary files instead of storing it all in an array. Or, you can write it out immediately rather than building an array, if that makes sense for how you are displaying the data. For example, you could have a status_200 file, a status_304 file, status_404 file, status_other file and "all" file. Then to do the final display stage you just read in each of those files and display the data immediately, consuming virtually no memory.
-
Sykoi, in what situations does checking the IP address not work? When the IP address changes due to proxy servers? If someone can access your session id, they could probably have accessed your username and password the same way. Presumably using a network sniffer or by intercepting your traffic at a proxy server. Like thorpe mentioned, https will largely solve those problems. Then you only need to worry about attacks on the client and server machines.
-
It's not possible.. but you can do this: [code=php:0]switch($var) { case 1: if ($something != $something_else) { break; } case 2: ..... break; }[/code] That will fall through to case 2 if $something == $something_else.
-
Try [code=php:0]<? $imm = "$_GET[url]"; $fstats = fstat($imm); if (!$fstats) { die("Couldn't fstat() $imm"); } $file_uid = $fstats['uid']; $my_uid = getmyuid(); print "File is owned by $file_uid, my uid is $my_uid\n"; ?>[/code] If the fstat() fails, then you may be looking in the wrong directory, or you may not have permission to read the directory the file is in. If the uids don't match, then that is why you cannot change permissions on that file.
-
Yes you can. But it may be easier to install an existing wiki package. Look on Google to find some.
-
table1: Id | Name 1 | Balkan 2 | Btherl table2: Id | Posts 1 | 5 SELECT * FROM table1, table2 WHERE table1.id = table2.id Id | Name | Posts 1 | Balkan | 5 Notice that "Btherl" is NOT in the results, because it is in only 1 table, not 2 tables. This may be why you are not seeing the results you want.
-
In Mysql, using the default MyISAM tables, it is very fast to count the number of rows in a table like this: [code=php:0]$query = "SELECT count(*) FROM p_poll"; $result = mysql_query($query) or die(mysql_error()); $row = mysql_fetch_array($result); if ($row) { $num = $row[0]; }[/code] I am rusty with mysql, so I may have made a mistake in there somewhere.
-
How about a table which stores a non-unique date for each username? Then, you create a row whenever a user logs in on that day. If no row exists for a given day, then the user did not login on that day.
-
What happens when you try to run it?
-
Post the code generating the warning.
-
When post data isn't appearing when I expect it, I would add this at the very start of the script: [code=php:0]var_dump($_POST);exit(0);[/code] That will display the entire contents of $_POST. If it's empty, then the problem is with how you call the script. I would guess that you need to add "method=post".
-
fert, can you try a few other common functions? Like puts() or fprintf()? Also, can you give the full output, from the command executed through to the error messages? The error messages in your first post don't match the command line in the later post. This makes me less confident that I am getting all the necessary information. Even seemingly unimportant things can make the difference.