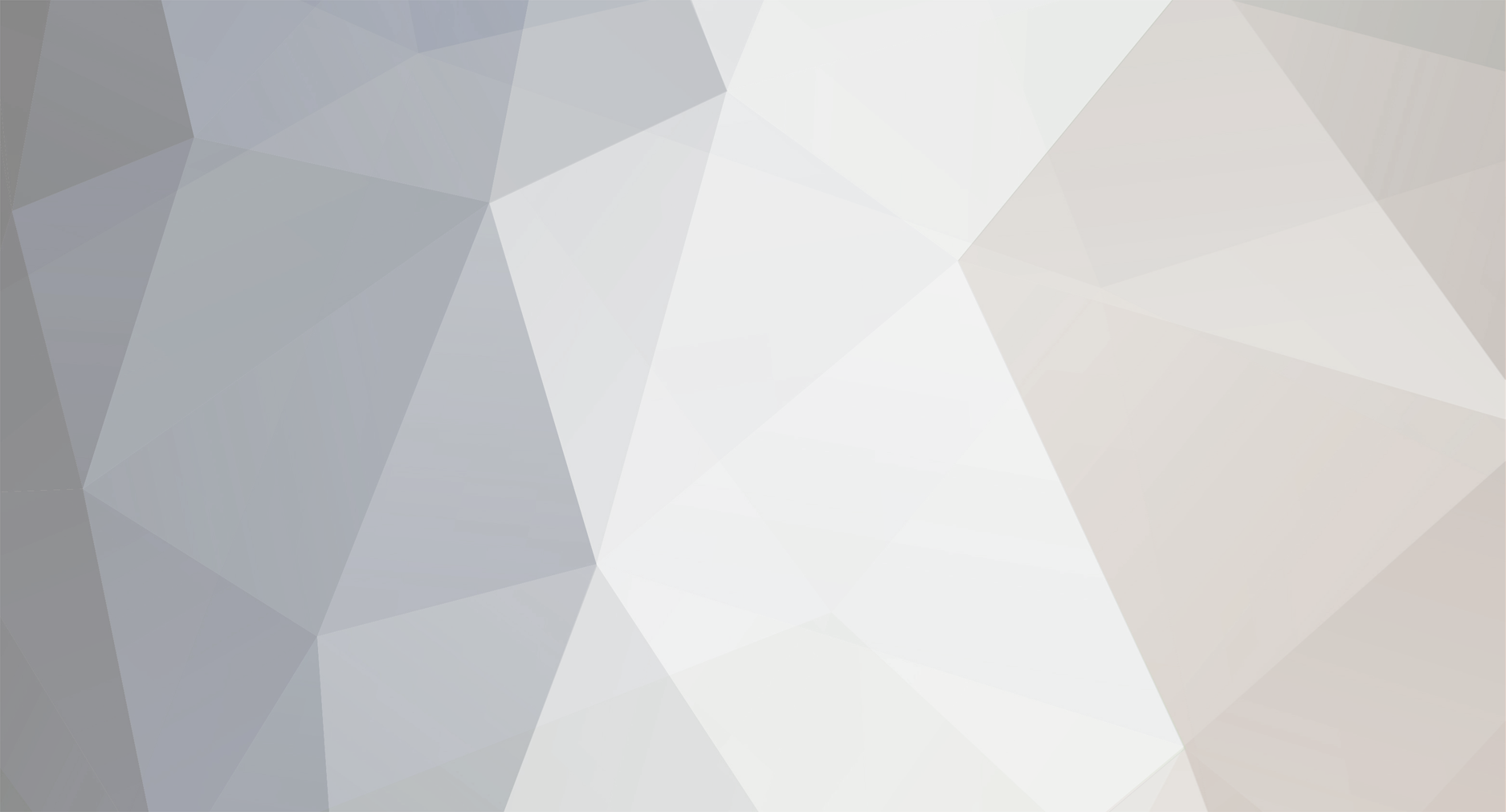
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Regarding this error: [code=php:0]Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in C:\Program Files\xampp\htdocs\srh\yellowedit.php on line 14[/code] Make sure ALL your mysql_query() calls check for failure of the query. The simplest way to do that is like this: [code=php:0]mysql_query($sql) or die("Error in $sql: " . mysql_error());[/code] If all your queries do that and you still get that error, it means you are trying to use something which is not a mysql result as a mysql result.
-
Your braces don't match up. As a result, login_box() is being defined outside the class definition. It's caused by your unusual indentation inside add_user().
-
PHP & Mysql caching problem - RESOLVED! Many thanks to forum helpers!
btherl replied to micky1955's topic in PHP Coding Help
Another way to avoid caching is to add a dummy argument to your scripts. If your script is always called with "×tamp=113768465", which changes every second, then even the most zealous cache can't use output from a previous call to the script that had a different timestamp. -
That means that the mysql extension for php is either not installed, or not configured for use by php. I can't help you with setting it up, sorry.. but at least you know where to look now :)
-
Problem in php4 Vs Mysql4(Select query unsupport"%")
btherl replied to cty's topic in PHP Coding Help
[code=php:0]$query="SELECT * FROM book where " . $searchtype . " like '%" . mysql_real_escape_string($searchterm) . "%'";[/code] This is very insecure, as $searchtype could be anything. It could be quite slow too, if there are many books. -
mysql query not updating (because of null fields)
btherl replied to leeming's topic in PHP Coding Help
Try printing out the query to see what is happening. And the mysql error too, like this: [code=php:0] ... or die("Error in $sql: " . mysql_error());[/code] Also, replace htmlentities() with mysql_real_escape_string(). That will do all the right escaping. If you haven't done so already, you may need to urldecode() the variables before escaping them for mysql. -
Try including your class definitions before session_start(). This is a shot in the dark but it may work. Also see http://sg.php.net/manual/en/language.oop.serialization.php
-
Here's another option.. [code=php:0]extract($_GET);[/code] See http://sg.php.net/manual/en/function.extract.php for details. It will import all your GET variables. Be aware that evil users may set extra variables you didn't expect, so be careful. Also, note that if some of your forms use post instead of get, this won't get those too. You might want to do [code=php:0]extract($_REQUEST);[/code] That will get you both get and post variables (and cookies too I think).
-
Can you post your database structure? How do you list the ingredients in the database? You can't access [code=php:0]$result['Submitted_by'][/code]. Instead you need to fetch the [code=php:0]$row[/code] as you do later on, and use [code=php:0]$row['Submitted_by'][/code]
-
What command did you use?
-
deleting rows depending on the contents of another table
btherl replied to miss_d_bus's topic in MySQL Help
Checking for matching rows in another table is done using a left join. For example: [code=php:0]SELECT * FROM categories c LEFT JOIN products p ON (c.id = p.category) WHERE p.category IS NULL[/code] The reason this works is that a left join puts NULL in the columns for the joined table if there is no matching row. Combining that with your query, you can do [code=php:0]DELETE FROM categories WHERE id IN ( SELECT id FROM categories c LEFT JOIN products p ON (c.id = p.category) WHERE p.category IS NULL )[/code] Make sure you have a backup of the table first, in case I made a mistake :) There is no need to use "distinct", because a non-matching row will only be listed once in the results set (though matching rows may be listed several times). -
As a first step, replace [code=php:0]$result = @mysql_query ($query);[/code] with [code=php:0]$result = mysql_query ($query) or die("Query failed: $query with error " . mysql_error());[/code] Then you can see if the query failed. "@" means "suppress errors", which also means you don't know what's going wrong with your script. It's usually a bad idea, and should only be used with a good reason.
-
Through the terminal, pgsql
-
Immortal, I think your problems will go away when you fix your indentation. Make sure every "while", every "if" and every "for" has a closing brace underneath it in the same column. In your latest code, the closing brace for your while loop is in the wrong column. This makes it unclear that the while loop finishes too early.
-
Runnerjp, i would guess that pagenum is equal to 0, and pagerows is 2. ($pagenum -1) * $pagerows is -2, which is not what you want. If your page numbers start at 0, you should just use $pagenum * $pagerows. If your page numbers start at 1, then there is a bug where you set your page number, and that needs to be fixed.
-
I have a very simple question.. how do I get pgsql (the CLI for postgres) to page results even when there's a small number of rows? Often the rows are long, meaning they scroll off the screen even though there are not many rows. pgsql doesn't enable paging because it thinks they will fit. This is driving me crazy :) Please help!
-
I would restructure the table so it matches how you want to display the data. [code=php:0]$data = array( 'eastern' => array( 'atlantic' => array( [0] => array( [id] => 18, [city] => new jersey, ), ) 'western' => array( 'pacific' => array( [0] => array( [id] => 4, [city] => dallas, ), ), );[/code] Hmm.. I hope that is clear :) Then you can just use foreach() loops to iterate through the conferences, then through the divisions, and then through the list of teams.
-
Calculating age from date of birth. Also time zone issues.
btherl replied to pthurmond's topic in PHP Coding Help
All your answers are here: http://sg.php.net/manual/en/ref.datetime.php I would recommend checking out date() and strtotime(), and have a read of the comments below. Many people have posted useful code in the comments. Edit: As for time zones, the forum software I've seen asks the user for their time zone on registration :) A lot try to guess the time zone with geolocation stuff, which I know little about.. but it seems quite effective. -
Str_replace and fwrite to replace a specific line?
btherl replied to Buschmaster's topic in PHP Coding Help
That's what you'll have to do, unless your lines are of identical length. Files are stored on disk sequentially, so replacing a line means moving ALL the following data forward to fill up any newly created space, or moving ALL following data ahead to allow space for a larger line. Better ways of doing it depend on what your application needs to achieve. -
I think the problem was your while() .. If I include the implicit braces, your code is this: [code=php:0] while (connect_to_db()) { if (!empty($_POST['username']) && !empty($_POST['password'])) { // Query the database $sql = "SELECT username FROM accounts WHERE username = '{$_POST['username']}'"; } /* end of if */ } /* end of while */[/code] What that will do is it will keep connecting to the db and setting $sql UNTIL THE DB CONNECTION FAILS, at which point it will continue. It should be this: [code=php:0] while (!connect_to_db());[/code] That would keep running connect_to_db() until it returns true, then it'll end the loop. Regarding global variables, they must be [b]specified[/b] in each function they are used in, but they are shared among all functions which specify them. That's why they are global. About your second question, I think you are missing braces. You should do like this: [code=php:0]if (condition) { blah; blah; } else { blah; blah; }[/code]
-
A global variable needs to be specified in every function it is used in. Other than that, it can be defined anywhere, inside or outside a function. I'm a bit suspicious about your "while (connect_to_db())" .. what is that intended to do? Why not just "if (connect_to_db()) { } else { echo "Couldn't connect"; }" ?
-
[quote author=thorpe link=topic=85628.msg389486#msg389486 date=1151645984] [quote]you could probaly learn it within 21 days with a good book or tutorial.[/quote] Im afraid no one is learning C in 21 days, whatever the title of the book says. [/quote] I disagree with this. If you put in an hour a day for 21 days, you can achieve incredible results. Most people who fail a "learn in X days" fail because they either didn't follow the instructions, or they didn't put in the time required. Following instructions and doing regular practice takes inhuman patience and ego-suppression. And if 99 out of 100 people who read the book don't learn in 21 days, that's because 99 out of 100 people are too lazy or to egotistic to put in the time and follow the instructions :)
-
I would make a variable (string or array), so you can collect ALL missing fields in one go. Just use a seperate "if" for each. Then after the checks, check if there are any messages, and if so, redirect and pass along the message to be displayed. You can do it with eg [code=php:0]header("Location: http://site.com/script.php?msg=" . urlencode($msg));[/code]
-
Try specifying the link identifier with each mysql_query() call. It sounds like you are triggering a new database connection when you don't specify it. [code=php:0]mysql_query($sql, $link);[/code] You'll also need to make $link global. Add [code=php:0]global $link;[/code] in connect_to_db(), and inside each function that you use $link in.