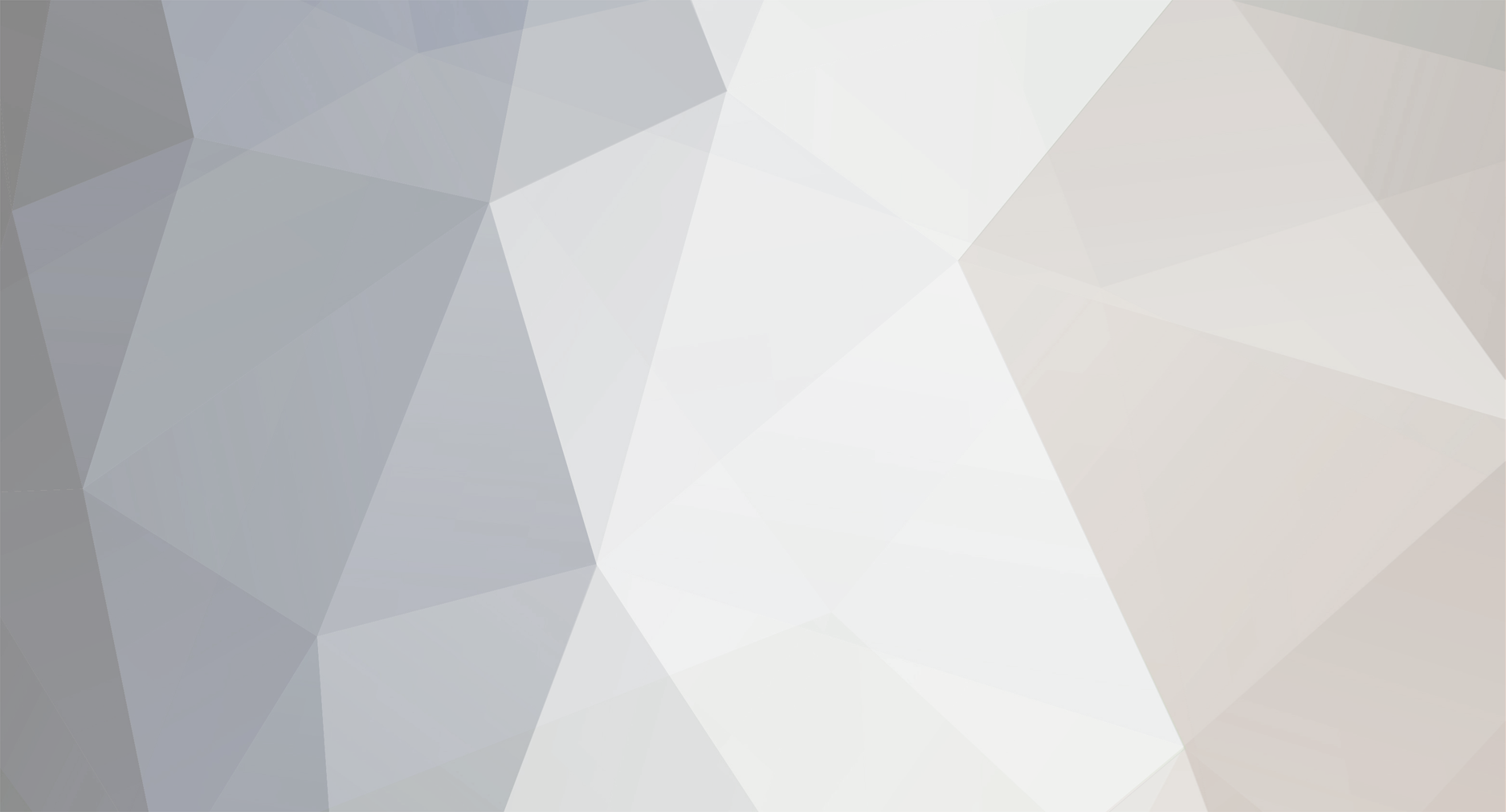
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Can you paste the actual output from each of the queries? Do you want to keep results from query 1 even when there is no matching ship from query 2? If so, use: [code=php:0]"SELECT sectors.*,ships.sector,ships.owner,ships.mothership,ships.name FROM sectors LEFT JOIN ships ON (ships.sector = sectors.id) WHERE (sectors.x BETWEEN '%s' AND '%s') AND (sectors.y BETWEEN '%s' AND '%s') ORDER BY sectors.x,sectors.y"[/code] That will put nulls for the ships table where there is no matching row.
-
MD5() giving valid results for complex strings?
btherl replied to soadlink's topic in PHP Coding Help
Oh.. about the undefined constant, did you try [code=php:0]md5(string);[/code] or did you try [code=php:0]md5('string');[/code]? Usually that warning is given if you don't quote a string literal. -
Can you give your actual output and expected output? I'm having trouble understanding from your description. The query looks logical. It should return a row with ship data from the ships table for every ship in the sectors with x between %s and %s and y between %s and %s.
-
mp3 tags are stored in the file itself. Same with version information and the like that you see on executables.. that is part of the file format. So to edit that information, you need a library which understands the file format (such as PE executable, or mp3 id tag) in order to modify it. As far as I know, there's no additional data stored along with files in windows. Just what is in the file itself.
-
MD5() giving valid results for complex strings?
btherl replied to soadlink's topic in PHP Coding Help
This is probably to do with character encodings. If you submit data on a website, it goes through several different encodings as it passes through your browser through to the receiving system, and then as it is decoded and passed to md5(). If you want to test different versions of md5(), you either need to take character encoding into account or use characters which are common to most codings, like alphanumeric characters. -
Are you sure this makes sense: [code=php:0]include("language.php?language=config/language.cfg");[/code] If you want to pass a variable to an included file, just set it like this: [code=php:0]$language = 'config/language.cfg'; include('language.php');[/code] $language will be available inside the included file.
-
Help Needed Porting this PERL routine to PHP
btherl replied to calane's topic in Other Programming Languages
My perl's a bit rusty.. is length() length of a string? I replaced it with strlen(). As for the ${$var} construct, you can do that as $$var in php. That means "The value of the variable named by the contents of $var". The regexp was simple so I used str_replace(). But in general you can use preg_replace() to do a perl style regexp replacement, and preg_match() to do a perl style regexp match. [code=php:0] for($y=1;$y<=100;$y++){ $thename="NAME_$y"; if(strlen($$thename)>3){ $num++; } } for($x=1; $x<=$num; $x++){ $qnt="QUANTITY_$x"; $prce="PRICE_$x"; $xtnd="XTEND_$x"; $ide="ID_$x"; $naam="NAME_$x"; $adlinfo="ADDTLINFO_$x"; $$naam = str_replace("\n", '', $$naam); $strMessageBody .= "Qty: {$$qnt} \nItem No: {$$ide} \nDescpition: {$$naam} \nAddtl. Info: {$$adlinfo} \nPrice: \${$$prce} Extended Price: \${$$xtnd} \n\n"; }[/code] -
If you're using large multi-dimensional arrays in php 4, and particularly if you are using serialize()/unserialize(), there are some memory leaks related to that. However it's more likely that the leak is due to something not being freed. Are you using a database interface? If so, which one and how do you ensure that it frees all space used after each query? Note that even if you let a postgres resource identifier go out of scope, you will lose memory! You must call pg_free_result() on them. If you're dealing with php data (except the case mentioned above), then as long as your variables go out of scope at the end of each function you should have nothing to worry about. PHP will automatically clean up variables that can't be referenced anymore. But it will not always automatically clean up after external libraries implmented in C. To see if you are losing data outside of php's tracking, use memory_get_usage(). If it reports a number vastly lower than the process's real memory usage, you have a leak. Keep in mind that if you have lots of modules compiled in, then your php processes will start with high virtual size. This is ok, as most of that is never used. Watch the difference between the process starting size and how much it increases if it starts out large.
-
[SOLVED] Error in SQL syntax... but i don't see it
btherl replied to jdubwelch's topic in MySQL Help
Try [code=php:0]`G8` = 'Middle Tennessee'[/code] instead of [code=php:0]'G8' = 'Middle Tennessee'[/code] -
[SOLVED] "Loading.. Please Wait" Can't figure it out..
btherl replied to scottrohe's topic in MySQL Help
[code=php:0]order by rand()[/code] will be quite slow, unless mysql has special code to optimize it. First, do you have an index on StateName? If not, adding that will be a big win for performance. If that's not good enough, then you could try pre-generating the data grouped by CountyName for each state. Again that will save you some time. Then to avoid the time spent ordering by rand(), you can assign consecutive id numbers to each state's data. Eg, state AZ will be numbered from 1 to n, state TX will be numbered from 1 to n, and so on. Then you can generate the random numbers yourself, and look them up using an index on State and id. That'll be ultra-fast. -
And [code=php:0]date('Hi');[/code] will give you 24 hour time, in case you want to do tests that cover both am and pm. If you want your input to use am and pm, then do a conversion to 24 hour time before doing the test. That's easier than trying to compare am/pm time directly.
-
[SOLVED] include files, from $data <? include $data.php ?> ??
btherl replied to ztealmax's topic in PHP Coding Help
Does it give any errors or does it just not include the file? -
[SOLVED] include files, from $data <? include $data.php ?> ??
btherl replied to ztealmax's topic in PHP Coding Help
What happens when you run this script? -
It could be that. Try using stripslashes() on the input to remove any extra backslashes. And print out the values before and after so you can see if it worked properly.
-
Trying to insert data when it's already there (and violating a private key or unique constraint) is an error.. so your insert statement will return an error status. How you test for that depends on which interface you are using (mysql_query() ? ). If you're using that, you can check the return value of mysql_query(). You might also try mysql_affected_rows(). I'm pretty sure that won't function if the query failed though, which will be the case if the insert failed.
-
Add some more "else" statements, to see what is failing. Currently you're not checking for failure of opendir(). If all your system calls are succeeding but nothing is happening, then it's time to start worrying :)
-
First thing to do is check for errors. It would also help if you used $sql instead of $query in mysql_query() :) [code=php:0]$mysql_result = mysql_query($sql) or die("Error in $sql:" . mysql_error());[/code]
-
I don't think this is a coincidence: [code=php:0]<?php $a = array(); $b = new stdClass; $a_string = (string)$a; $b_string = (string)$b; print "Array as string: $a_string\n"; print "Object as string: $b_string\n"; print "String 'Array' indexed by string: {$a_string[$a_string]}\n"; print "String 'Object' indexed by string: {$b_string[$b_string]}\n"; ?> [/code] See how the A and O come into existence? I'm pretty sure there's something like that in your code, though I haven't been able to find it.
-
If no output is appearing there are two possibilities 1. Your query is invalid. 2. Your query is valid, but produces no results.
-
Which columns match the sing and song tables? Said another way, how do you know which singer sings which song?
-
It looks to me like you want two seperate queries.. you can always UNION the results together afterwards. In your expected output, you never have both Specialty and Position set in the same row. But the purpose of join is to merge data into the same row. If you want seperate rows, you can do two queries followed by a union, leaving null values as placeholders for the rows which aren't involved in that particular query. [code=php:0]SELECT `Firms`.`name` AS `Firm`, `Employees`.`name` AS `Employee`, NULL AS `Specialty`, `Positions`.`name` AS `Position` ... SELECT `Firms`.`name` AS `Firm`, `Employees`.`name` AS `Employee`, `Specialties`.`name` AS `Specialty`, NULL AS `Position`[code=php:0]
-
The answer depends on what you're using the crawler for. For a simple crawler, you can use recursion. First, create a function which crawls a URL. Then when you encounter a link, just call that function again with the link as its argument, instead of the original url. For something more heavy duty, a good approach would be to store all links you've seen in a database. Then when you've finished with the current page, you pick another from the database and crawl that. This gives you much more flexibility in the order of crawling, which you'll need for something large-scale.
-
If you have a syntax error, try printing out [code=php:0]$sql[/code]. My guess is that one of your variables contains the ' character. You should always use mysql_real_escape_string() on any data you are putting into an SQL query.
-
[SOLVED] In what circumstances does (unset var) == 'string' ?
btherl replied to btherl's topic in PHP Coding Help
Here's what I'm using: Server: Apache/1.3.33 (Debian GNU/Linux) mod_gzip/1.3.26.1a PHP/4.3.10-18 mod_auth_pam/1.1.1 mod_ssl/2.8.22 OpenSSL/0.9.7e mod_perl/1.29 mod_choke/0.06 I suspect I've found an obscure bug in php 4.3.10 .. I guess it's time to upgrade :) The test script returns the expected results for me too. It's only the application when running on certain data sets that results in the strange behaviour. I've sat and stared at the output for quite some time, but no luck.. the if condition succeeds, but when I var_dump($_REQUEST) INSIDE the if, the tested array entry is not there. I tried a few others, like [code=php:0]if ($_REQUEST['bubbles'] == 'bubbly')[/code] and they succeeded too. All tests with === failed as expected, such as [code=php:0]if ($_REQUEST['bubbles'] === 'bubbly')[/code]. Thanks for your help guys :) I'm going to file this under 'unreproducible bugs' (I found some others related to large arrays causing seg faults too, all fixed in php 5)