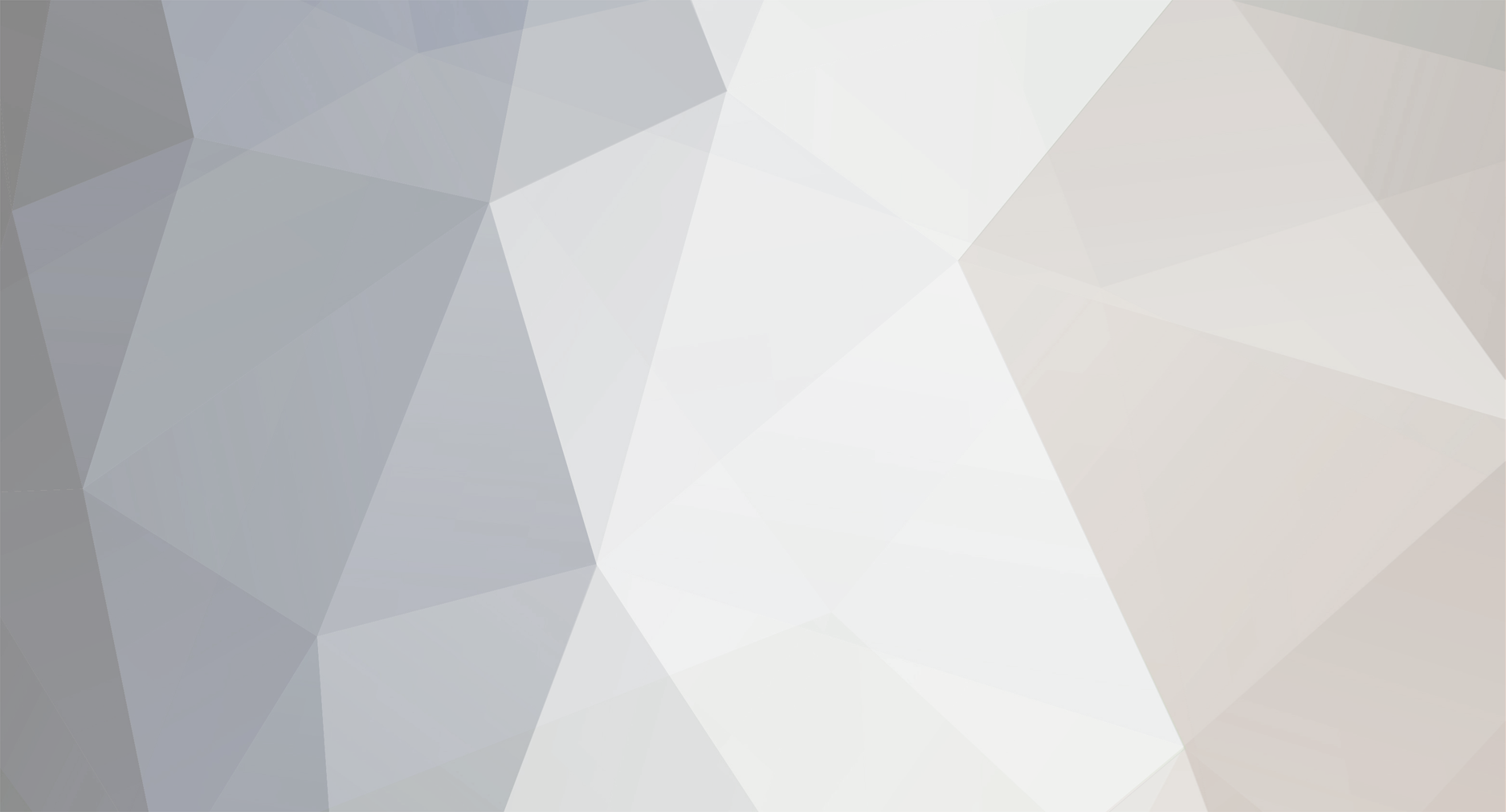
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
And your parse error is due to using " in a string delimited by ". Try [code=php:0]echo "<table width=\"100%\" border=\"0\">";[/code] Notice that I've added a backslash before every double quote except the first and last.
-
I'm not sure that I fully understand your question, but these variables may help to tell if you are in an HTTPS request: [code=php:0]$_SERVER['SCRIPT_URI'] $_SERVER['SERVER_PROTOCOL'] $_SERVER['SERVER_PORT'] # Usually 80 for HTTP, 443, for HTTPS[/code] These may not always be set..
-
The result from your outer query is called $item_result, but you are iterating using $sys_database->sql_fetchrow($result) .. could this be the problem?
-
Help really appreciated: Form caching problem
btherl replied to supergrover1981's topic in PHP Coding Help
That's the browser doing that.. one way to fix it is to make sure your users fetch the page again, rather than going back in the browser. You can force that by getting the browser not to cache the pages (I'm not sure of the mechanism to do that). If you want to solve it in the browser itself, look at javascript rather than php. And don't trust IE for refreshing pages. -
For dates in y-m-d format, you can just use [code=php:0]if ($start_date > $end_date) { ... }[/code] For your case, it's a bit messier. I think the easiest is to extract the year, date and month and compare each one. [code=php:0]if (preg_match('|[[:digit:]]*/[[:digit:]]*/[[:digit:]]*|', $start_date, &$matches)) { $start_m = $matches[1]; $start_d = $matches[2]; $start_y = $matches[3]; } else { echo "Invalid start date\n"; } if (preg_match('|[[:digit:]]*/[[:digit:]]*/[[:digit:]]*|', $end_date, &$matches)) { $end_m = $matches[1]; $end_d = $matches[2]; $end_y = $matches[3]; } else { echo "Invalid end date\n"; } $start_cmp = "$start_y-$start_m-$start_d"; $end_cmp = "$end_y-$end_m-$end_d"; if ($start_cmp > $end_cmp) { echo "Start date is after end date. Cannot lar!\n"; }[/code]
-
There's a fundamental flaw in the design of your data structure. You will have to redesign it to get it to do what you want. What you're doing now is: 1. Set $episodearray[104] to an integer 104 2. Treat $episodearray[104] as an array You simply can't do that. You will need to store that number 104 somewhere else, or you will need to store the additional data in a seperate array. For example, you could do: [code=php:0]$episodearray[104][0] = 104; $episodearray[104][1] = "Bleach-104.avi"; $episodearray[104][2] = "58mb"; $episodearray[104][3] = "www.akirablaid.com/media/downloads/";[/code] Or, you could do [code=php:0]$episodearray[104] = 104; $episodearrayextra[104][1] = "Bleach-104.avi"; $episodearrayextra[104][2] = "58mb"; $episodearrayextra[104][3] = "www.akirablaid.com/media/downloads/";[/code] Either will work. But you can't set something to an integer, and then treat it as an array. Whichever script is adding code like that needs to be fixed.
-
Yep, exactly :) I think you get what I mean by granularity. You're using half-hour granularity, and I used 5 minutes in my example. I use this kind of technique a lot.. instead of looping over an array multiple times, I make an associative array where the array index is exactly what I'm looking for. If I want to check if a string is in an array (and will be doing it several times), then I do this: [code=php:0]$arr = array( 'string1' => true, 'string2' => true, .... 'string100' => true, ); if ($arr[$test_string] === true) { print "Matched $test_string\n"; }[/code] That's much faster than checking against all of 100 array entries for every string you want to check. It only works for exact matches though.
-
Depending on the granularity of your times, you can use an associative array to record which events are at which times, like [code=php:0]$arr['20:05'] = 'event1'; $arr['20:10'] = 'event1';[/code] And so on.. then, if any event needs to go in an array slot that's used, you know there's a clash. This approach requires only one pass through the events. But if you're allowing fine-grained times, it may be cumbersome to make all the array entries.
-
From Google: http://support.microsoft.com/kb/175168 Searching for the string "Operation must use an updateable query" will give you many other helpful results too.
-
Try this for your queries: [code=php:0]$result = mysql_query($query) or die("Error in $query: " . mysql_error());[/code] Then we can see the actual mysql statement which had the syntax error. After that, solving it should be easy :)
-
Using CURL to submit form via post method - Tutorial?
btherl replied to doni49's topic in PHP Coding Help
There are some firefox extensions, including UrlParams, Tamper Data and Live HTTP Headers, which will let you log all the headers and post data from an actual request. You can compare that with your script, and add anything you think might make a difference. Usually it's the referrer, the user-agent or some hidden post field which is missing. Some sites need a "jsessionid" in the url. Unfortunately it's just trial and error.. They're not about to give you an API for the site :) -
It looks to me like you are creating a new Smarty object in members_main(). That object will be destroyed when the function ends. If that's the case, you can fix it by passing $smarty into the function, or by making it a class variable.
-
Yes, it's a requirement for curl to be installed. But I expect most sites would have it. Since your script doesn't crash, curl must be installed :) If it wasn't, it would fail when trying to call curl_init(). I'm not sure what you mean by "the script does return the url called via curl, it just does not post the results". What do you see when you echo $contents ? And about POSTFIELDS as an array or as a string, it may work on the site that it works on, but we are looking at the site where it doesn't work :) It's worth trying the string format instead. Maybe the site where it doesn't work doesn't support the array syntax. For the example you gave there, you would use: [code=php:0]$data = "zip=$value&distance=500";[/code] If you're dealing with strings rather than integers, then you should urlencode() each variable value in the postfields string.
-
I'll ask the obvious questions first.. is Curl installed on the site where it doesn't work? Does the script fail altogether, or do you just get an empty result? Also, according to the documentation, POSTFIELDS should be a string rather than an array. Eg [code=php:0]$data = "ZipCode=90277";[/code]
-
You can order and limit your results. [code=php:0]SELECT * FROM table ORDER BY id ASC LIMIT 0, 5[/code] I think that's the correct Mysql limit syntax. It means "start at row 0, and take 5 rows". Note that you have to order your results by something unique to every row, otherwise you may get different orders from each request. And that would mean your paging wouldn't work properly.
-
After query, would like selected items to be flagged and not reused
btherl replied to kenwx23's topic in MySQL Help
You can flag them by adding another column to the table for the flag.. Or you can make another table storing the ids you've already used. Then when you want to fetch more entries, you can join with the "used" table and only select rows that don't match that table. Basically, there's no way to "flag" something in a database without adding data to be the flag. There's no flagging mechanism. Or, another thing you could do is use a cursor and fetch 50 elements at once. As long as it's all being done in the same process, that ought to work with no problems. But I assume you will be doing these fetches over a period of time, and in seperate processes. -
Oh.. you want to redirect the user to the output of the paypal request? That's not really possible using curl, since you need the user's browser to make the request. You could do it with javascript.. Display a post form leading to paypal, with all the correct variables set (the form doesn't have to be visible to the user, since it will be submitted automatically). Name the form "paypal_form", eg [code=php:0]<form name="paypal_form" method="post" action="http://www.paypal.com/...">[/code] Then have some javascript that does [code=php:0]document.paypal_form.submit();[/code] I think it'll work if you put that javascript code down the bottom of the page.. the order of javascript code execution really confuses me! On the page which does that submit, you can display a big "Please wait ...". You could even make it in a pop-up, if you've ensured your users have turned off their pop-up blocker.
-
Ok.. I think that there is no way to DIRECTLY do what you want to do. You simply can't print out a variable before it's set. But you can do something equivalent. You can collect all the results from your database query and store them rather than printing them out. Then you can print $totalcommission, and then after that you can print your database results. Like this: [code=php:0]$output = ''; while($row = mysql_fetch_array($res)){ $link = "<a class=links href='vieworder.php?orderid=".$row["order_id"]."' target='_blank'>"; $check = "<input class=checkbox type='checkbox' name='chkorders[]' value='" . $row["order_id"]."`".$row["artist_id"] . "' > "; $amount = $row["total"]; $totalamount += $amount; $commission = (($amount*$artistcommission)/100); $totalcommission += $commission; $amountaftercommission = $amount - $commission; $totalaftercommission += $amountaftercommission; //THIS IS THE BOTTOM $totalcommission. echo $totalcommission; // Here is the important line $output .= $link . $check . "whatever else you want to print out ... "; $i++; }[/code] Now, after all that: [code=php:0]print $totalcommission; print $output[/code] Does that makes sense?
-
No, I don't see what you're getting at. Can you post your actual code and tell me what you're trying to do with it?
-
Or is it sessions you are looking for? That lets you keep values of variables between script calls.
-
It doesn't make sense becuase you said "After the script has been output". Once a script has run, no variables have any values. That's how I interpret it anyway :) This might be what you want: [code=php:0]$val2 = &$val; $val = 7; echo $val2; // Shows 7[/code]
-
He can't use $_GET because it's the referrer, not the script URL. [code=php:0]$query = preg_replace('|[^?]*\?|', '', $_SERVER['HTTP_REFERER']); $query_bits = explode('&', $query); foreach ($query_bits as $varval) { list($var, val) = explode('=', $varval); if ($var == 'q') { # Do something with val } }[/code] That ought to work, assuming the search engine does "q=search+term". The preg_replace is to cut off everything before the question mark.
-
Could you tell us the actual situation you want to apply this too? The example on its own doesn't make much sense :)
-
http://sg.php.net/manual/en/function.array-merge.php This will work, providing your array is numerically indexed (that is, as long as those are ints and not strings). BUT, it will re-index starting with 0, not with 1. Do you need it to start at 1 rather than at 0? array_combine() may also be useful.
-
Are the folders in the same location as your script? Also, file_exists() tells you if the folder exists, not whether there are files inside it.