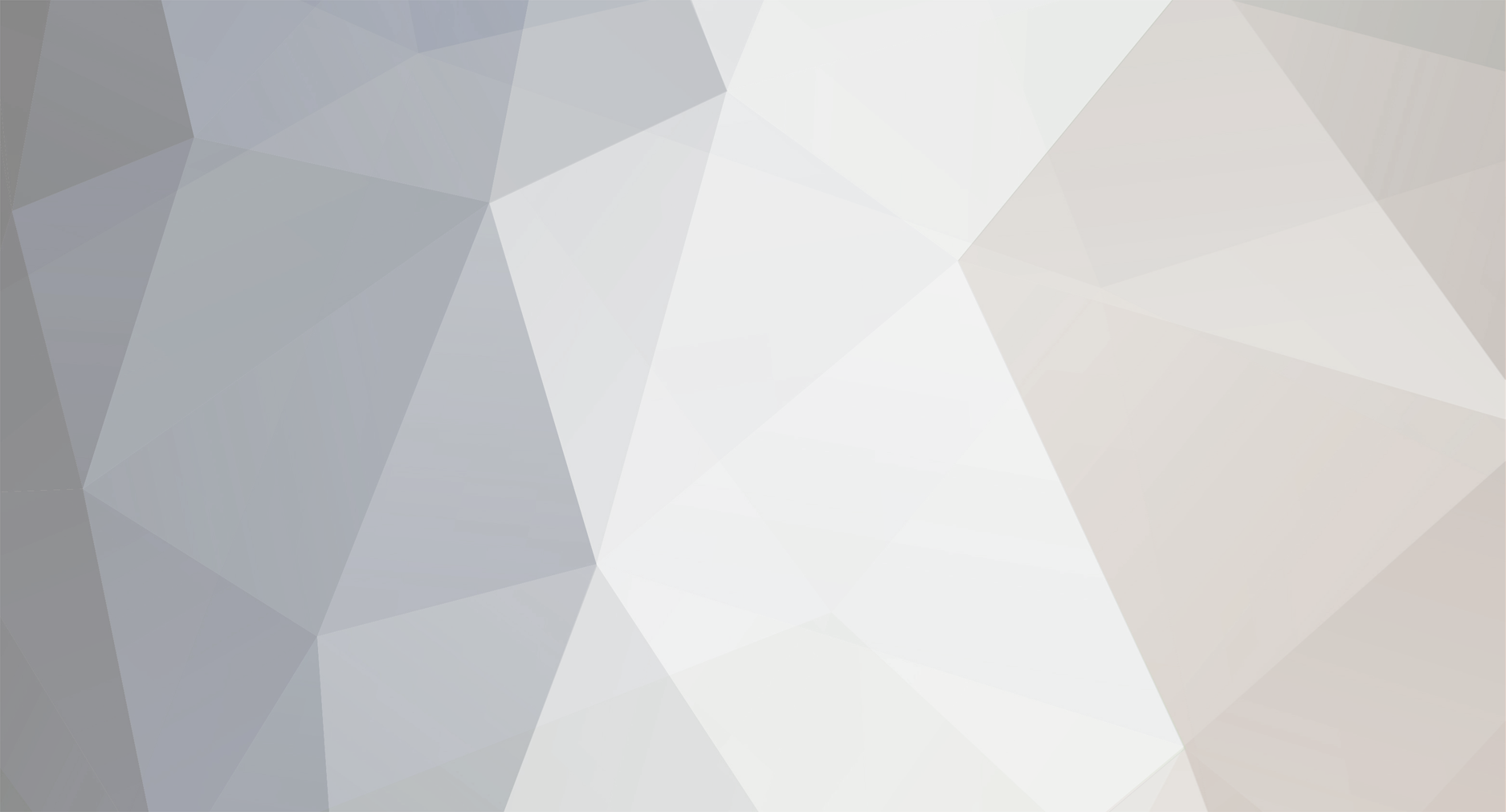
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Very confusing conditional statement problem
btherl replied to unitedintruth's topic in PHP Coding Help
Is your problem that the conditional statement is not generating the correct "align=", or that the "align=" instruction is not being followed by the browser? -
The only way to reliably order results in SQL is to use "ORDER BY". That means you need a column to order by. You can add an extra column which defines the sorting order, and order by that. The problem with relying on the order of data entry is that it may change, due to backups/restores, table optimization or other things. But the appropriate solution for your situation really depends on why you want to order your results that way.
-
To number each row, use [code=php:0]$row = 0; while ($row = mysql_fetch_array($mysql_result)) { print "Row number $row\n"; $row++ }[/code] About that big "if", you can do like this: [code=php:0]$table = array( '0' => 'NA', '1' => 'S', ... # and so on for each possibility 'Y' => 'F', ); echo $table[$b];[/code] That is more flexible and allows for easier editing.
-
Is it possible that headers have already been sent? Try calling setcookie() (with any arguments) at the very top of your script, right after the first [code=php:0]<?php[/code], and see if that call succeeds or fails.
-
If your form is short but the results are big, then it doesn't matter. The only thing that matters is what you are submitting.
-
Hmm.. it's messy. I think a recursive approach will work well. I can't spare the time right now to do more then pseudocode. [code=php:0]function rescue_array(&$lines, &$arr) { $line = array_shift($lines); # Fetches next line from the print_r output if (start of an array) { # Skip the '(' line rescue_array(&$lines, &$arr[$subarray]); } elseif (array entry) { $arr[$entry_name] = $entry_val; } elseif (line is ')' at end of array) { return; } # Process next element of array rescue_array(&$lines, &$arr); }[/code] Stack depth might be a problem if your array is REALLY huge..
-
number format.. identify commas and remove.. how?
btherl replied to jwk811's topic in PHP Coding Help
You could do [code=php:0]$num = str_replace(',', '', $num);[/code]. Then it's definitely got no commas :) -
What does this do please, in an email form? http_referrer = getenv
btherl replied to 11Tami's topic in PHP Coding Help
When you follow a link, your browser usually sends the address of the place you came from. That code grabs that address. If it's an email form, it's probably being grabbed to verify that the email form was called from your site, rather than any other site. It can be spoofed though, so be careful that your script is not abused by spammers. -
How do you know it is properly set? Try printing it out just before your "if", inside check_city().
-
Is [code=php:0]$ext[/code] the value you expect it to be? If yes, then the problem is with the preg_match(). If no, then the problem is with how $ext was set. You can find out where it goes wrong by printing out the values of each variable that led to the value of $ext.
-
You can say [code=php:0]check_email($addr)[/code] or [code=php:0]check_email($_REQUEST['bubbles'])[/code] or any other name. Inside the function, that variable will be called [code=php:0]$email[/code]. Was that what you were asking? And what [code=php:0]return[/code] does is it says what should happen if you do something like [code=php:0]$var = check_email($addr);[/code] The value you gave for [code=php:0]return[/code] is what [code=php:0]$var[/code] will be set to.
-
I suspect $filedir is not set correctly, probably because $HTTP_SERVER_VARS have different values in the different webserver. Try looking at the output from this: [code=php:0]echo "<pre>"; var_dump($HTTP_SERVER_VARS);[/code] That will show you what is available in $HTTP_SERVER_VARS You can also try [code=php:0]echo "<pre>"; var_dump($_SERVER);[/code] $_SERVER is the new name for $HTTP_SERVER_VARS.
-
Can you give more detail? Where do you see that url? And what is in your php script?
-
You probably need backquotes around the order column each time you mention it.. `order`. I'm a postgres guy, so that might not be the right escaping..
-
It may be your browser (or an extension, or some other add-on) making multiple requests. Another common problem is accidentally include()ing a file twice.
-
Parse error: parse error, unexpected T_BOOLEAN_OR
btherl replied to mforan's topic in PHP Coding Help
The problem here is your coding style.. it's terrible :) You can make things clearer with this technique: [code=php:0]$change6_bad = (($buildchange[6] != "0") && ($buildchange[6] != "")) && ($info[8] == "Private")); $change7_bad = (($buildchange[7] != "0") && ($buildchange[7] != "")) && ($info[8] == "Private") || ($info[8] == "Captain") || ($info[8] == "Major") || ($info[8] == "Lt. Colonel") || ($info[8] == "Colonel")); # and so on for each, then if ($change6_bad || $change7_bad | ... ) { }[/code] You may want to split it up even more. One thing you could use is a "rank_greater_than()" function, instead of doing all those individual tests. That will make the code much clearer. And with clearer code, you will notice syntax errors much more easily. [code=php:0]if ((($buildchange[6] != "0") && ($buildchange[6] != "")) && !rank_greater_than($info[8], 'Private')) || ...[/code] -
Hmph. Well, you can order by aggregates in postgresql. If mysql doesn't let you do that, then make it a subquery: [code=php:0]SELECT * FROM (select COUNT(id) AS count_id, id, ip, dateline FROM tablename GROUP BY ip) AS foo ORDER BY count_id DESC[/code] Semantically that's identical, but it may be less efficient due to use of a subquery.
-
Change your query to this: [code=php:0]$sql = "select COUNT(id), id, ip, dateline FROM tablename GROUP BY ip"; $result = mysql_query($sql) or die("Error in $sql: " . mysql_error());[/code] That will display what the error was.. then we can fix it based on that :)
-
Allow people to run sql on the web free form? Is this a BAD Idea?
btherl replied to jsladek's topic in MySQL Help
Each user has various priveliges.. in addition, they can only take actions affecting their own databases and tables, unless explicitly given permission to do otherwise. As long as you set sensible priveliges for your users, they won't be able to do anything evil. More info here.. http://dev.mysql.com/doc/refman/5.0/en/security.html And http://dev.mysql.com/doc/refman/5.0/en/security-against-attack.html The second link contains a list of privileges which may sound reasonable, but will expose you to serious security risks if you grant them. -
Rows in SQL do not have "numbers", like row 3. The only way to address them is by their columns, such as id. If you want to deal with the row with id 3, use [code=php:0]WHERE id = 3[/code] Regarding the error, try [code=php:0]$name = '<a href="a2.php">a2[/url]'; $result = mysql_query("UPDATE example1 SET name='" . mysql_real_escape_string($name) . "' WHERE id='1'")[/code] mysql_real_escape_string() will do all the proper escaping for a mysql query. The problem you were having was because you have double quotes within your query, but you are also using double quotes to indicate the start and end of your query.
-
"limit -9,9" means "Start from offset -9, and return 9 objects". That doesn't make any sense. The offset must be 0 or greater. Whichever code adds that "limit -9,9" is causing the problem.
-
You might be able to use [code=php:0]SELECT * FROM users WHERE username ILIKE 'jamie'[/code] ILIKE is case-insensitive. Another option is to compare [code=php:0]WHERE LOWER(username) = 'jamie'[/code]
-
Add [code=php:0]ORDER BY count(id) DESC[/code] at the very end, assuming that is what you want to order by. Alternitavely you can fetch the data and sort it in php, but this is the mysql forum :)
-
No (well, not unless you reconfigure your web server to allow it), but you can generate javascript using a php script. Then you will have a .php file which outputs javascript instead of html. Or you can output javascript inline into the html using PHP.