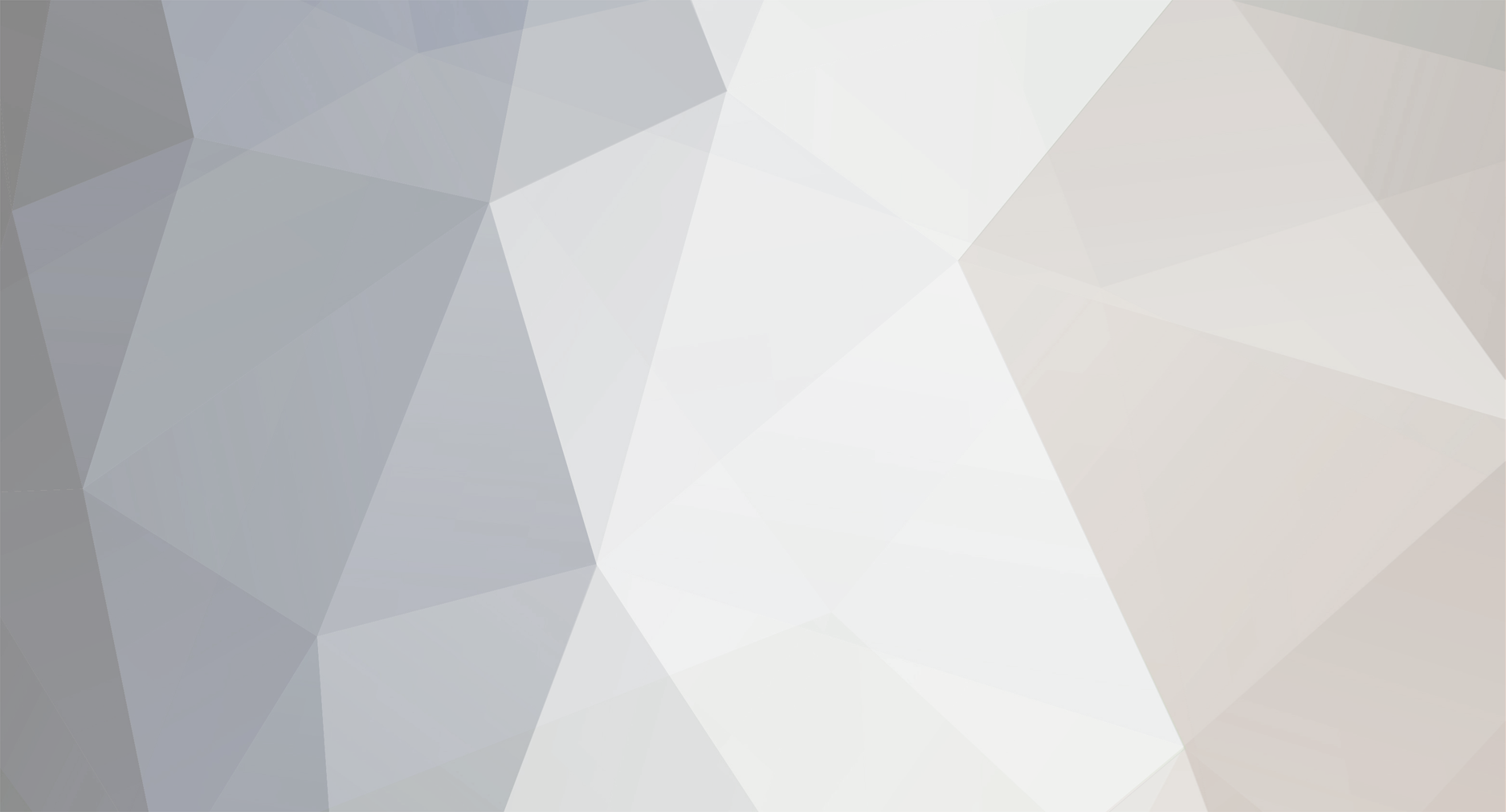
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
This may be your answer.. "Static variables in functions that are class members do not work quite as you would expect - they are not initialised only once throughout the life cycle of the program, but once per every object created."
-
Sorry.. I forgot which version of PHP I was using :) Well, here with php 5.1.4 it gives me a segmentation fault. That's pretty bad. I altered the code to this: [code=php:0]<?php class A { static public function instance() { static $instance; if(!isset($instance)) { print "Created instance\n"; $instance = new A(); } else { print "Didn't create instance\n"; } return $instance; } public function __construct() { new B; } } class B { public function __construct() { A::instance(); } } print "About to call A::instance()\n"; A::instance(); ?>[/code] The output is: About to call A::instance() Created instance Created instance .... Segmentation fault Edit: Oops.. I should have put the print earlier. Yes, it is looping.
-
Parse error: parse error, unexpected T_STATIC, expecting T_OLD_FUNCTION or T_FUNCTION or T_VAR or '}' in /var/www/www.prioritysubmit.com/t.php on line 5
-
You can only have one WHERE clause in a query. Break it up into seperate queries and you'll be fine :)
-
You're missing a comma.. [code=php:0]"SELECT id, team, against, kickoff, against, side, p1, p2, p3, p4, p5, p6, p7, p8, p9, p10, p11, p12, p13, p14, p15, p16, p17, p18, p19, p20, date, DATE_FORMAT(date,'%d-%m-%Y') AS dstamp FROM teamplayers WHERE team=".$team." "[/code] Also, you should check for errors after each mysql_query(). [code=php:0]if ($result === false) die(mysql_error());[/code] And yes, you can * everything else, while also adding extra columns.
-
Is your memory limit set to the default of 8MB? [code=php:0]print "Memory limit is " . ini_get('memory_limit') . " bytes<br>";[/code]
-
All of us started as newbs :) Try this code: [code=php:0]$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'http://www.paypal.com/script'); curl_setopt($ch, CURLOPT_HEADER, 0); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, 'submit=Submit&variable=value'); $content = curl_exec($ch);[/code] A lot of those are standard options.. it's just the last two that are specific to post forms. A good way to get the data you need to put in POSTFIELDS is to use a firefox extension like LiveHTTPHeaders, UrlParams or Tamper Data. That'll show you exactly what is being posted when you submit a form. Then you can grab that and put it into your own script. Keep in mind that all the variable values in postfields should be urlencoded. Eg [code=php:0]$variable_enc = urlencode($variable); $postfields .= "&variable=$variable_enc";[/code] BUT, if you never decoded your variables in the first place then you can pass them straight through.. just use trial and error :)
-
You can use http://sg.php.net/manual/en/ref.curl.php , which is ideal for this situation. Alternatively you can use AJAX, but I think CURL is simpler. CURL is also php-only, whereas AJAX would involve some javascript programming.
-
Send from where to where? From the form to php, or from the php back into the form?
-
I think you should be joining on the group_id between the groups table and the "ug" subquery, rather than using "NOT IN". That "NOT IN" will only check one single ug.group_id, rather than the whole set. Try:[code=php:0]SELECT DISTINCT g.group_id, g.name, g.maxmem maxmem FROM groups g LEFT JOIN (SELECT COUNT(* ) num, group_id FROM usergroup GROUP BY group_id) ug ON g.group_id = ug.group_id WHERE ug.group_id IS NULL[/code] The reason for "IS NULL" is that it will give you all rows not matching ug, which I think is what you want. IS NOT NULL will give you what you wanted when you did "g.group_id IN (ug.group_id)". Alternatively you can do: [code=php:0]SELECT DISTINCT g.group_id, g.name, g.maxmem maxmem FROM groups g WHERE g.group_id NOT IN (SELECT DISTINCT group_id FROM usergroup);[/code] No need to get the count since you aren't using it.. and no need to select from ug when it doesn't appear in the results.
-
I looked it up.. http://dev.mysql.com/doc/refman/5.0/en/delete.html It seems that DELETE t1, t2 syntax which operates on tables rather than columns. It's a mysql extension. "DELETE *" is not mentioned in the documentation, so I would recommend not using it. "DELETE FROM table WHERE condition" is the usual syntax.
-
You can do [code=php:0]function validate_reg() { ... return $error } $error = validate_reg();[/code]
-
"DELETE *"? What does that do? That's like no sql I've ever seen :)
-
You should ask your hosting provider about that. It's likely that you can't connect to the database from outside, and can only use localhost.
-
Usually sessions are implemented with cookies :) So the same behaviour will occur. You can change sessions so they work by passing variables.. that's significantly less secure, but will allow you to have different sessions in each window. The details are here: http://sg.php.net/manual/en/ref.session.php
-
Sorting two different database queries alphabeticly
btherl replied to Fallen_angel's topic in MySQL Help
If the tables are identical, then you can do this [code=php:0]SELECT * FROM reports UNION reports2 where name_id = '$id'[/code] If they're not identical but you only want fields which are common to both, you can also do [code=php:0]SELECT * FROM (select id, field1, field2 FROM reports) UNION (select id, field1, field2 FROM reports2) where name_id = '$id'[/code] Then you can order that query. If you want to merge matching rows between the two tables and sort those merged rows, then that's totally different, that's a join. If you want that, post again :) Table definitions would help. -
mansuang, I think he's looking at protecting files which should only be included, rather than files which should only be linked to from the CMS.
-
That's normal behaviour for a web browser..
-
Normally you would do that with .htaccess files, assuming you are using Apache as the webserver. Alternatively, you could put code at the top of each sensitive script which checks if it was included from the CMS or being called directly. The CMS can set a variable which the sensitive code can check before executing. If the variable is set, it runs as usual. If it's not set, then it displays an error message. Or to be truly paranoid, you can combine both methods :)
-
Your variable naming looks pretty good to me :) Variable naming is an art rather than a science.. there's no correct way or wrong way. There are certainly bad ways, like naming all your variables $foo1, $foo2, $foo3 :) The real test is when you forget what your code does and try to reverse-engineer it.. then you see how good your naming was. And good variable naming will reduce debugging time considerably, since you won't have mistakes due to forgetting what variables really are.
-
Sorting two different database queries alphabeticly
btherl replied to Fallen_angel's topic in MySQL Help
Can you post the table descriptions and tell us how you want to merge them? It depends very much on how you are combining the data. -
That's tough to express in SQL. I think it's easier just to fetch all the rows without the GROUP BY, and merge them in PHP. GROUP BY is great when you want to find sums and counts, but when you're merging text data.. the rules for merging duplicate entries get messy. In this case you want to append all the packages together (the duplicate merging rule), while grouping by name, username and address. Just use php :)
-
addslashes() isn't designed for HTML escaping. Try htmlspecialchars() instead http://sg.php.net/manual/en/function.htmlspecialchars.php
-
mysql_real_escape_string() already does the necessary escaping. As long as you use that string inside single quotes, you will be fine. There's no need for the other lines. You might need to urldecode() your input first as well, depending on what type of input it is. [code=php:0]$escaped_input = mysql_real_escape_string(urldecode($_POST['input'])); $sql = "INSERT INTO table VALUES ('$escaped_input')";[/code]
-
Can you give us an idea of your level of php experience? And more detail about what you're doing? For example, are you using a forum package?