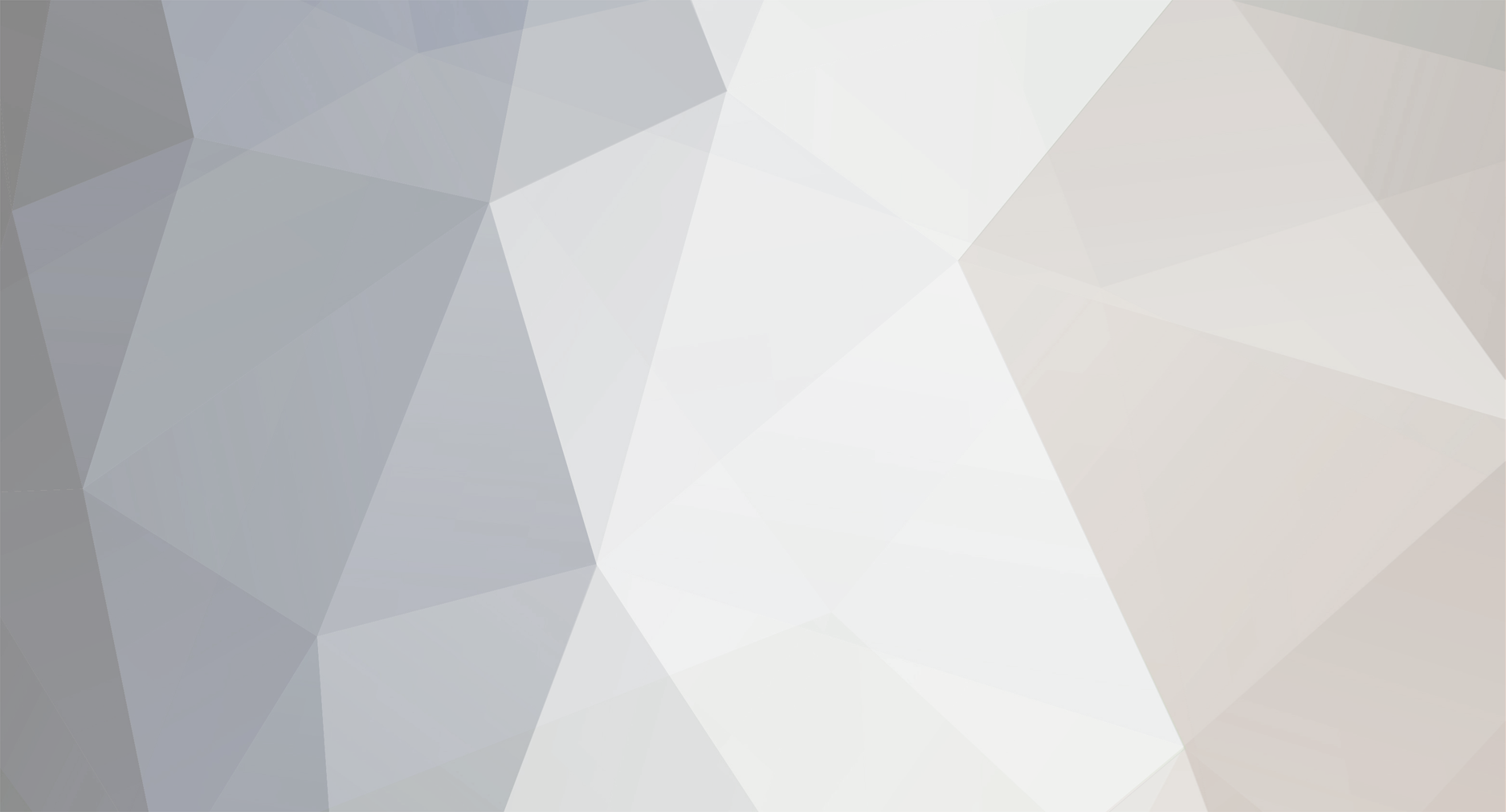
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Ok.. I assume that you see the message "You have logged out successfully", which tells you that the "if" statement worked properly? Then the problem is in your query. When you added the error check for the query, it worked correctly? If so, the next step I would take is to check mysql_affected_rows($result). [code=php:0]print "The query affected " . mysql_affected_rows($result) . " rows<br>";[/code] It should have affected 1 row. If it affected 0 rows, then $_email was probably not set correctly.
-
Do you have prior experience with forms, php and mysql? That will help us know what kind of answer you need.
-
Seraskier, can you give more details about what "didn't work". We need to know what behaviour you are expecting and what behaviour you are seeing in order to help you.
-
This script modifies $HTTP_SESSION_VARS['Id'] .. so I would assume that the modification causes it to fail the second time around. Checking your query for errors may help: [code=php:0]$rs = mysql_query($g, $link_id) or die("Error in $g: " . mysql_error());[/code]
-
Do you want to show all files in a folder (Mappe), sorted by name? These may help: http://sg.php.net/manual/en/ref.dir.php http://sg.php.net/manual/en/function.glob.php glob() sorts by name already. And I suggest you change the topic from "Sort mapfiles" to "Sort directory files" or "Sort folder files" :) Mach Spass :)
-
The code you already have looks fine. You might want to check for errors on your queries though: [code=php:0]$sql = "UPDATE users SET online = '1' WHERE email = '$_email'"; $result = mysql_query($sql) or die("Error in $sql: " . mysql_error());[/code]
-
How do you know that the images are stored correctly? There is something odd in your code.. you "SELECT image,type" then you list($image,$size) = mysql_fetch_array($result). Those don't match.
-
Try this to replace your mysql_query(): [code=php:0]$bagr = mysql_query ($storebag) or die("Error in $storebag: " . mysql_error());[/code] Can you tell us where you are finding only one item instead of all three items?
-
Making something change before your eyes not on page load.
btherl replied to 11Tami's topic in PHP Coding Help
You can change things without loading the page using javascript. If you need to fetch data from php without reloading the page, you can look into AJAX (it's a big topic) -
Is the "name" of the array "$column_fields"?
-
Get and post arguments are tab-specific, so you can use those to differentiate tabs.. it's a hassle to add them everywhere though. You could make a wrapper which generates links with a tab identifier added to each link. But really, why would you want your users to have multiple accounts open in seperate tabs? :)
-
And see http://www.php.net/manual/en/ref.dom.php
-
That's a pretty complex task.. and very uncommon too. I think you will have to do it manually. A good first step would be to extract all the sql statements. If the statements use things like "select *", then you'll need to look at the following code also.
-
how to sort multi-dimensional array with specified dimension ?
btherl replied to vesper8's topic in PHP Coding Help
usort() is good for this [code=php:0]usort(&$moyLig_array, "third_field_cmp"); function third_field_cmp($a, $b) { if ($a[2] < $b[2]) return -1; if ($a[2] > $b[2]) return 1; return 0; }[/code] Those comparison functions are confusing at first, but are very powerful once you get used to them. The comparison function defines the ordering by saying how to compare two items from your array. $a and $b are two array items. -
I am a little unclear on what you are asking, but yes, you can set values in $_REQUEST. That will affect any files included by the your script. [code=php:0]$_REQUEST['id'] = '12345'; include "page.php";[/code] page.php will see $_REQUEST['id'] as '12345', instead of the previous value.
-
You can use underscores, which look visually similar to spaces. [code=php:0]$fatm += ($row->fat_mono * $row->mgrams / 100);[/code] And you can rename the columns from the query appropriately by selecting "`fat mono` AS fat_mono", if this is database results you are dealing with. Or you can fetch the data as an array instead of an object.
-
You can fetch all the data sorted by timestamp, and then have a loop which extracts the day and time, and then displays according to those values. By keeping the last displayed day in a variable, you will know when to start displaying a new day (it will be whenever the current day is not equal to the last displayed day). [code=php:0]$last_day = null; foreach ($data as $d) { list($day, time) = extract_day_time($d['timestamp']); if ($day !== $last_day) { # New day } else { # Continue current day } }[/code]
-
"WHERE username LIKE '%$ptSearchFirstName%'" will give you all users whose username has $ptSearchFirstName as a substring. The '%' means "match any string". So it translates to "Match anything followed by $ptSearchFirstName followed by anything". It's actually Mysql code, not php code, but mysql is used often with php. If $ptSearchFirstName is empty, then it will return all users, since it'll just be "LIKE '%%'", and % matches anything.
-
if [current time] is between 7AM and 5PM, do this, else do this
btherl replied to DaveLinger's topic in PHP Coding Help
date('G') will give you the current hour in 24 hour format. so.. [code=php:0]$hour = date('G'); if ($hour >= 7 && $hour < 17) { # daytime } else { # nighttime }[/code] -
Mozilla - Running Query Twice, IE Running Query Once
btherl replied to lilman's topic in PHP Coding Help
Do you have any extensions installed in Mozilla? Something may be sending a second request to your script. Check your server logs to see if there is a second request being made. Alternatively you can log requests to a file at the start of the script. -
And yes it is common. Whenever php gives you an "unexpected something" error, that means that the error occurs at OR BEFORE the line number indicated. It may even be on the first line. "Unexpected $" means "Unexpected end of file". The easiest way to fix it is to use a php editor with syntax highlighting. It will find the problem for you instantly.
-
There's only two possibilities if an update of one column always triggers an update of another column. 1. The columns ARE linked. Maybe the table was setup so an update of one column triggers another. This seems very unusual for those columns though. 2. There's another sql statement being executed. My gut feeling is that it's #2, because it doesn't make sense to trigger an update of datejoined when lastvisit is changed. An initially time-consuming but worthwhile way to check this is to replace mysql_query() with a wrapper function. Then have the wrapper function print out every query that comes through it. Eg [code=php:0]function __mysql_query($sql) { print "$sql<br>"; return mysql_query($sql); }[/code] Then use __mysql_query() instead of mysql_query() everywhere. When you want to switch off the debugging output, just comment out that single print statement. Later you can use this wrapper to do timing of sql statements and other useful stuff. You can also put error checking inside the wrapper, to save yourself having to check for errors after every query.
-
From memory, you should insert null into the auto-incrementing column. Or you can just not specify it in the list of columns.
-
Are you sure that that sql statement is the only one being executed? Limit 1 won't do anything if users_handle is unique (which it should be). If users_handle doesn't have a unique index on it already, try adding one. It'll make your lookups faster and will help to detect bugs.
-
It's referenced from the current file. Which makes sense, since you may include a file from many other files in many other locations, particularly shared libraries.