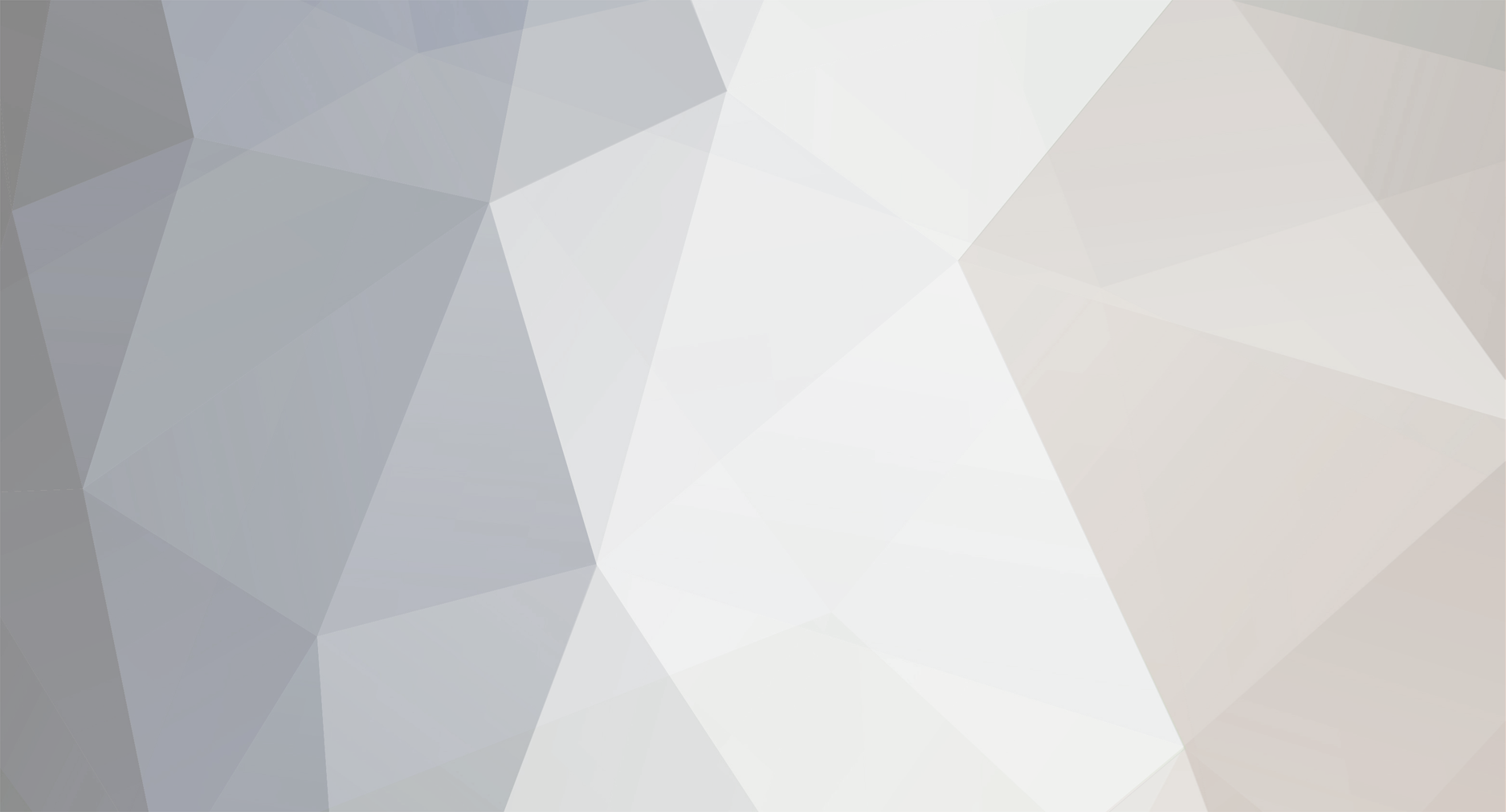
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
[code]echo '<td>' . $empid . '</td>';[/code] For example.. in your case, replace the <?php echo $empid; ?> with this: [code]' . $empid . '[/code] The dot means "stick two strings together". So you are sticking the first half of the string, $empid and the last part of the string together into one big string. That big string is then echoed.
-
The error occurs when you try to use a string as if it was an array. If the error occurs within tep_get_source_list() itself, then it must occur in this line: [code]$sources_array[] = array('id' => $sources[$i]['sources_id'], 'text' => $sources[$i]['sources_name']);[/code] And the cause would be that $sources, as returned from tep_get_sources(), is not the type of array that was expected. Try var_dump($sources) before the for loop, and see if it looks unusual. If $sources is fine, then the error may be occurring in a function called by tep_get_source_list().
-
A problem might be that you are grouping by aal.products_id, then counting distinct aal.id Also, you will need to aggregate all columns that do not appear in the group by, even ones from other tables. When you join tables, they all become one big table. If you want to aggregate and THEN join, you need to use a subquery. Regarding the error, try using mysql_error() to get a more helpful error message. If possible, use an interactive terminal interface to mysql (or phpmyadmin) to try out your query until you can get it right. It's much easier than doing it via php.
-
That doesn't make sense.. if the entry isn't in course_users, then there is no user_id. Or do you mean entries which either aren't in course_users, or are in course_users but don't match the user_id? With the intention being of finding all courses that a user is not currently associated with?
-
That means you ran out of memory.. and yes it's because the image is too large :) You can either increase the available memory: ini_set('memory_limit', '128M'); Or you can use the image size to estimate memory needs, and reject any images which are too large to process. Or maybe you can process the image another way, with command line tools for example.
-
I suggest you do 5 seperate queries rather than just 1, with error checking for each. It would also be a good idea to validate all of the emails, not just the first one. If a query fails, display the SQL so you can see what went wrong.
-
Try [code]case 'cart':[/code] The rest looks ok.
-
You're calling it $con['sock'] in one function, and $con['socket'] in the other :P I must confess that I have done that hundreds of times.. it's the simplest errors that are the hardest to find.
-
php mysql where statment partial match numbers only
btherl replied to vanderlay's topic in PHP Coding Help
A string starting with numbers will be converted into a real (float) matching those numbers. A string starting with letters will be converted into the real 0. Then the comparison proceeds as usual. See http://dev.mysql.com/doc/refman/4.1/en/type-conversion.html -
Remember that 10 items is still only 1 page.. subtract 1 from the number of items, and then your formula will work (or it may be off by 1, depending on if your pages start at page 1 or page 0). This ought to work: [code]$n = count($array) - 1; $last_page = round($n / 10) + 1;[/code] There's also some tutorials on pagination on this website somewhere..
-
http://sg.php.net/manual/en/function.fsockopen.php references the stream_set_timeout() function, which looks like what you want.
-
function => recursion problem *SOLVED ~ 10% sane-ness remaining lol*
btherl replied to leeming's topic in PHP Coding Help
Perhaps you should do [code]$treestack = initTree($req1, $tree_stack, $depth + 1);[/code] Or alternatively, you can pass $treestack by reference. -
If I understand your problem correctly, you can dynamically generate the SQL statement, something like this: [code]$sql = "SELECT * FROM `project_general` "; $added_where = false; if ($group) { $sql .= " WHERE `group`=\"$group\" "; $added_where = true; } if ($priority) { if ($added_where) { $sql .= " OR "; } else { $sql .= " WHERE "; $added_where = true; } $sql .= "`priority`=\"$priority\" "; } if ($type) { if ($added_where) { $sql .= " OR "; } else { $sql .= " WHERE "; $added_where = true; } $sql .= "`type`=\"$type\" "; } $sql .= " ORDER BY `rec_num` ASC";[/code] Yes, it's not elegant. SQL is messy to generate. If I was writing that I would put the SQL generation code into a separate function to clarify things.
-
What's going on there is that the array is further up.. for the expression $barrow[$jobinfobase2['uid']]['uid'] to work, $barrow must be an array, $jobinfobase2['uid'] must be an array, AND $barrow[$jobinfobase2['uid']] must be an array. If any one of them is not an array, then the expression doesn't make sense. How can you find the 'uid' element of a string? It's likely there's a bug in your code causing something to be set to a string when you want it to be an array instead. It may be at ANY level of that multi-level array you are working with. Try doing a var_dump($barrow) just before the error and see what output you get.
-
This table details the differences between the various tests: http://sg.php.net/manual/en/types.comparisons.php Like alpine said, you can simply check the submit button. Try var_dump($_POST) to see exactly what is set during a form submission. However, for a user-friendly interface it's nice to check each form variable separately and generate appropriate error messages.
-
[quote]because $results[$datastream['type']][$datastream['number']]['status'] doesnt exist, ...[/quote] It's not because it doesn't exist.. it's because it's a string rather than an array. You can do an is_array() beforehand to see if it's an array. Or you can stop putting strings where your code later expects to find an array :)
-
I don't think I was clear. What I mean is this: [code]SELECT itemName,itemId,itemPrice,itemSmall FROM `products` WHERE itemDesc like "%$descvalue%" OR itemName like "%$descvalue%" OR itemId like "%$descvalue%"[/code] Do you want to check if $descvalue is in any of those 3 columns?
-
Searching Text field full of '|' seperated numbers, how?
btherl replied to CalDude's topic in MySQL Help
If you don't mind redesigning your table, you can change from thing varchar, features varchar, Unique index on thing to thing varchar, feature integer, Non-unique index on thing (for use when updating a thing's features) Non-unique index on feature (for searching by feature) Then you can simply SELECT distinct thing FROM table WHERE feature IN (13,15,140,144,156); -
generate random number with variable probability
btherl replied to grilldor's topic in PHP Coding Help
I would do it like this: [code]$possi = array("0.1", "0.2", "0.2", "0.3", "0.3", "0.4", "0.4", "0.5", "0.5", "0.5", "0.6", "0.6", "0.6", "0.6", "0.6", "0.7", "0.7", "0.7", "0.8", "0.8", "0.9", "1.0"); $decide = rand(0,21); $extra = rand(0,99) / 1000.0; $damage = 500 * ($possi[$decide] + $extra); echo $damage;[/code] By doing it mathematically, zero-padding isn't an issue. This assumes you're ok with an even probability distribution for the extra 2 digits. -
There's no best way.. I suggest you adjust your style according to the circumstances. If it looks more readable to put more information into each variable, then do it. If it doesn't, then don't. The most important thing to consider is maintainability. When you want to change that code later, what style will make those changes easier? And that style may be different for different parts of your script. At my workplace we use Smarty templates for everything now. For large projects, it's the best solution. For small projects, the methods you described work just fine.
-
Perhaps the $_SESSION test is failing? I wouldn't count on cookies being sent for the files specified in the wmx file.
-
That query syntax looks suspicious.. I would parse it as [code]SELECT itemName,itemId,itemPrice,itemSmall FROM `products` WHERE itemDesc IS TRUE OR itemName IS TRUE OR itemId like "%$descvalue%"[/code] Not sure about the double quotes, since i'm a postgres user. But I'm pretty sure that you need to specify the condition for every column to be checked. If it's still not making sense, try printing the query to the browser, then run it in phpmyadmin (or whichever interface you have for mysql)
-
That's very odd.. because php is executed on the server, not in the browser. The browser cannot crash in php code. But the output displayed by a php script can cause a browser crash. It's likely that the javascript caused the crash, as javascript is run in the browser.
-
What does a browser crash look like? What message do you get?
-
There are some example here: http://sg.php.net/manual/en/ref.ftp.php