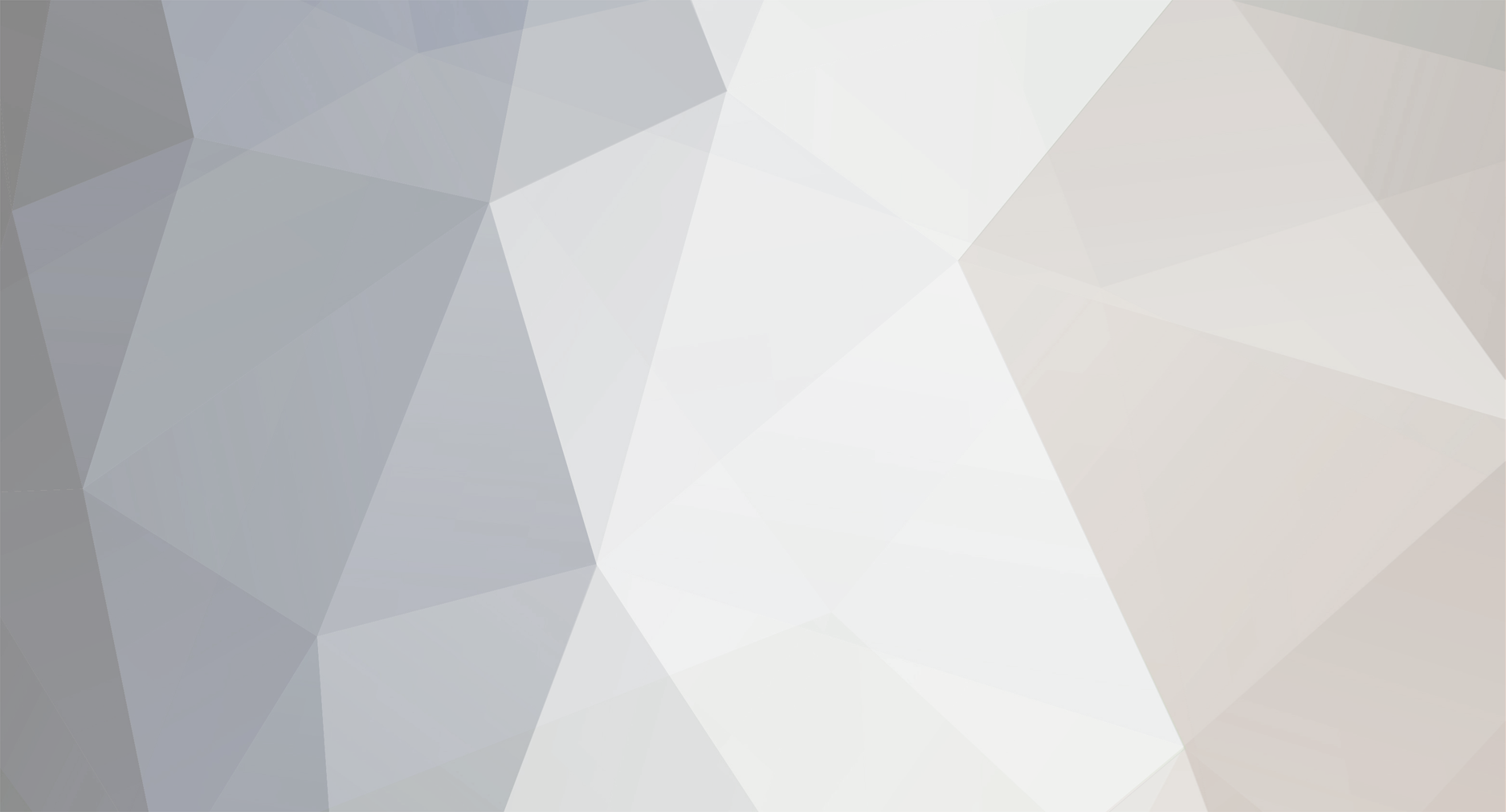
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
parse error, unexpected T_DOUBLE_ARROW
btherl replied to bassplaya4string's topic in PHP Coding Help
PHP uses >= instead of =>, because => has another meaning. [code] if ($a >= 5) { $arr = array('fred' => 5); }[/code] -
A critical difference between php's null and sql's null is that sql's null is not equal to itself. $a = null; $b = null; if ($a === $b) print "null === null\n"; This will show that php null is equal to php null. But SELECT null = null; in SQL will give you the result "false". SQL's null is never equal to itself, because it represents "unknown value". That's why you have to use "IS null" or "IS NOT null" to test for nullness in SQL, instead of using "= null". However, people very often use null in sql to mean "no value" as well as "unknown value". So whichever meaning you are using it for, you need to use the appropriate conditions. Typically you'll use "IS null" when null means "no value", but you'll check for equality when it means "unknown value".
-
MySQL Question - Pulling From Two Tables And Merging
btherl replied to JustinK101's topic in PHP Coding Help
That syntax will do a join.. I think you want a union. Try: [code](SELECT id, first_name, last_name, job_title_occupation, current_clock_status, last_succ_login FROM employees) UNION ALL (SELECT id, first_name, last_name, job_title_occupation, current_clock_status, last_succ_login FROM inactive_employees) ORDER BY last_name ASC[/code] That will merge the two tables. "UNION ALL" will not remove any duplicates, which is usually faster. "UNION" will remove any duplicates which are in both table. If you want to do some kind of merging between duplicates in the two tables, then you need to do a join instead.. post again and we can help you with that :) -
You can put things inside a div and then mark the div as hidden, using javascript. I can't give details as my memory is hazy, but that approach will certainly work. Or in PHP, you can put <? if (!isset($_POST['submit'])) { ?> <form> ... </form> <? } ?> That will only display the form if $_POST['submit'] is not set. I hope one of those approaches helps!
-
You can put all the complexity into sql like this: [code]SELECT searches, count(searches) as count FROM search_table GROUP BY searches ORDER BY count DESC LIMIT 100[/code] The "group by searches" takes the place of your "select distinct" .. a "group by" will make the columns you group by distinct. But when you group results, you need to use an aggregate like count(), sum(), max() on any other values which were not grouped (in this case you just want to count how many times each "searches" appears). After that, the ordering and limiting is straightforward. That query will give you the top 100 searches.
-
When comparing strings, "lexical comparison" is used. 1st character of string is compared, then 2nd character, then 3rd, ... If you compare "123" and "abc", php will check "1" and "a", using an ascii table like this: http://www.lookuptables.com/ If you compare "123" and "12c", php will check "1" and "1", then "2" and "2", then "3" and "c". It looks for the first difference. BUT, if you compare "123" and "321", php will compare them as numbers. It only compares strings if it cannot convert them to numbers. If you always want string comparison, you can use strcmp()
-
It's possible that your update query succeeds but affects 0 rows (that is, nothing was updated). You can try keeping the result of the query, then calling mysql_affected_rows($result) to see how many rows were updated.
-
Which parts don't you understand? There's probably something fundamental that, once you get that, it will all make sense :)
-
Removing the double quotes around the php command should help. The other double quotes can stay.
-
What output are you expecting and what are you seeing? ARe you expecting [code]Fred 1/1/01 Blah! Fred 2 2 Fred 1/1/01 Glerp Fred 1 0 John 2/2/02 Blah Fred 5 1 John 2/2/02 Fnord John 7 1[/code] But instead you are getting the same user on threads that belong to another user? Judging by your code structure, you would be expecting results like the above.
-
You might try [code]system('limit stacksize unlimited; ./objectfile');[/code] The reason being that the limit is probably reset between system() calls, since system() is opening a new shell each time.
-
You can use output buffering.. like this: [code]ob_start(); include('header.html'); $header = ob_get_clean();[/code] I'm pretty sure that'll do what you want. Then you have your HTML in a string.
-
Looks like a course exercise to me :) I think that any way to do it will be messy. I would do it like this: 1. Rearrange the data into an array using initials as the index, like: array( 'sk' => array(13, 15, 14), 'ge' => 13); 2. Print out the header ("SK GE HY ME"); 3. Print out the values, using an index variable to step through. Ie, for ($i = 0; $i < $num_values; $i++) { print values in index $i for each initial } That algorithm will work. It's not elegant, but I can't think of any elegant way.
-
Submitting a form without clicking submit button.
btherl replied to jeboy's topic in PHP Coding Help
You can do document.myform.submit() in javascript, for a form named "myform" That can even be done when there is no submit button (keep in mind that there'll be no "Submit=submit" type variables sent if you submit this way) -
In interesting issue when passing amounts.. Anyone seen this before?
btherl replied to programguru's topic in PHP Coding Help
I would try printing out the values you send to the gateway right before you send them. You can display them in the browser or you can write them to a file. If the gateway is receiving the wrong values, then printing out the values right before they are sent should show incorrect values too. Then, if they are incorrect, you can work backwards until you find where they become incorrect. -
It looks fine to me.. try printing out the values to double check: [code]print "hotelType: {$row_rsHotel['hotelType']}, Id: {$_GET['Id']}<br>";[/code] Maybe the hoteltype matches but the Id doesn't?
-
You might want to try this too: [code]print "I am in directory " . getcwd() . "\n"; print "My uid is " . getmyuid() . "\n"; print getcwd() . " is " . (is_writable(getcwd()) ? "writable" : "not writable") . "\n";[/code] It's possible that newfile.php exists and the permission problem is there..
-
A php extension would be ideal for this, but I think php extensions are C only, not C++ .. can anyone confirm this? The documentation for them is here: http://www.zend.com/php/internals/index.php
-
[quote author=fenway link=topic=107138.msg429493#msg429493 date=1157573385] Fourth, I couldn't live without hashrefs for everything, and I don't see that as native in PHP, though I might have missed it. The list goes on and on... I couldn't ever abandon Perl. Feel free to correct me if need be. [/quote] Since you made the invitation.. PHP references use C syntax, eg "$hashref = &$assoc_array". However, variables are copy on write, including function arguments, so in most cases you don't need references. For multilevel hashes, you can just write $assoc_array['level1']['level2'] = 'bleh'; It's not much different to perl really. Is there a use for hashrefs which doesn't come under one of the above? (that is, sharing data and multilevel structures) I haven't hit a wall with PHP.. I think it is equal in power to Perl. Just that perl is geared toward text processing, and php is geared toward web applications. At my workplace we use perl for backend scripts, and php for the frontend.
-
Look at how the domDocument object is created here: http://www.php.net/manual/en/ref.domxml.php#61088 Instead of using "new", it's returned from a function call. eg $domDocument = domxml_open_mem($string) will create a domDocument from a string.
-
Is character a reserved word? I forget how to quote reserved words in mysql, but that's my best guess. The syntax looks fine.
-
It's not a stupid question.. people spend hours debating it :) My experience is that perl and php are very similar in power. Perl is better suited for text processing, and php is better suited for web applications. Javascript is executed on the user's computer (in the browser), and it is usually used for simple tasks. Most of the brains will be in the perl and php running on the server. Pretty much anything you think of can be done by perl & mysql, php & mysql, perl & postgres, php & postgres, or other combinations. Some things are easier with a particular combination. PHP and perl are scripting languages, whereas mysql and postgres are databases. They store data and let you fetch data.
-
It's also possible with Ajax. I can't help you with details, but the idea is that you can make a javascript call to fetch the new data, and use javascript to update only the portion of the page you want updated. The flash object can keep doing its thing while that other update happens.
-
Ok, php4 will be using the DOM XML extension, but that code you are trying is for DOM, which is with php5. There's some examples for using DOM XML here: http://www.php.net/manual/en/ref.domxml.php Take a look in the user comments at the bottom of that page for some examples. Good luck :)
-
The purpose of this forum is to pester us with questions :) Then we get a warm fuzzy feeling when we can help! I'm guessing the problem here is that you are putting a literal string 'Line1\nLine2\nLine3' into the database.. notice the single quotes there. "\n" is only converted to a linefeed if it's within double quotes. So, this should work: [code]$content = "Line1\nLine2\nLine3"; # Double quotes means \n is converted to linefeed $escaped_content = mysql_real_escape_string($content); $query = "INSERT INTO table (content) VALUES ('$escaped_content')";[/code] Try that query and fetch the data back and see if it works. The key point is that by setting $content using double quotes around the \n characters, the \n are all converted to linefeeds. After that things should be fine. Actually you could use str_replace .. str_replace('\n', "\n", $string). It converts literal '\' and 'n' into the linefeed character "\n".