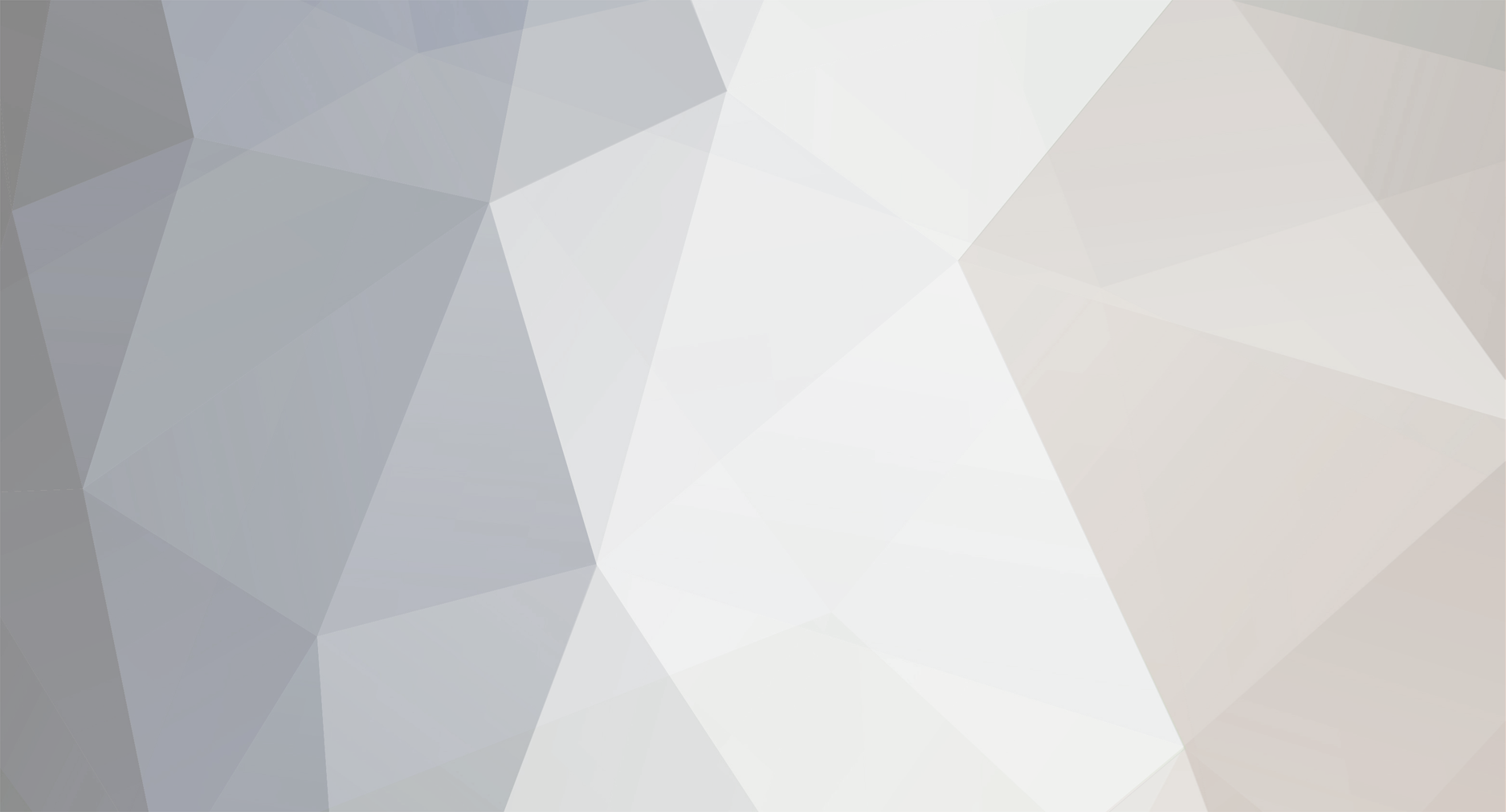
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
You can make a function, eg "validate_username()". Then put all the messiness inside there. Creating a function (or object, structure, etc etc) to hide the messiness is one of the most effective ways to simplify a program and make it easy to understand and get working properly.
-
Calc not working out correctly - Bad code - just needs to work :D
btherl replied to gaogier's topic in PHP Coding Help
I can't spot the error.. but the debugging method I would use here is this: 1. Find where the input data comes into the program. Print out the values. Are they correct? If no, the bug is in input handling. If yes, go to step 2. 2. Go down the code to where the first calculation is done. Print out the values both before AND after the calculation. Is the result correct? If no, the bug is in that calculation. If yes, go back to step 2 and repeat (with the next calculation that may be faulty) until bug is found. Once you've repeated that enough times, every calculation in your code will be correct and the script will be fixed. -
Oh I see .. you need to put the same session initializing code into EVERY php file. You can either copy and paste it, or put it all into one file such as "session.php" and include that into every other file. Otherwise captcha.php doesn't have access to your session.
-
You can connect to the mail server with SMTP and ask to send an email to that address, then cancel. It's not guaranteed to work but it's better than nothing. Keep in mind that you need to look up the MX DNS record for a domain to find where to connect to - if you just connect to the domain itself you might not find the SMTP server there and you'll get a false negative result for the test. There's an overview of the SMTP protocol in this article: http://en.wikipedia.org/wiki/Simple_Mail_Transfer_Protocol MX records: http://en.wikipedia.org/wiki/MX_record Yes it's complicated - that's why most sites send an email instead
-
It would take me between an hour and a day, depending on the exact spec. As for suggestions, start with something simpler and build up from there. Eg start with a script taking one input and displaying it. Then change it so it stores that one input into the database instead. Then have it check for duplicates before storing. Then add a second input item. Then add the rest of the input items.
-
Can you post line 144 of register.php?
-
Using a generated row number in another query
btherl replied to MargateSteve's topic in PHP Coding Help
This is what causes $i to increment by 1: $i++; If you want rank from the sql, you should use $row_table['rank']. There are actually two independent things there which happen to have the same values - $i and $row_table['rank']. If I was doing the task you want to do, I would read all the rankings into a php array and then deal with it there. Doing it in SQL will probably end up too complicated. -
Hmm.. very strange. Try adding this code directly after the delete: $sql = "SELECT * FROM ipdatabase WHERE userid='$userid' AND ipaddress='$ip'"; print "About to execute query: $sql<br>"; $select = mysql_query($sql) or die(mysql_error()); while ($row = mysql_fetch_array($select)) { print $row['userid'] . ":" . $row['ipaddress'] . "<br>"; } The reason is to check if the row is gone immediately after you delete it, because it may be that it gets deleted and then added back later. Once we're 100% sure the delete is working properly then we can look elsewhere for the bug.
-
What I mean is this: $sql = "DELETE FROM ipdatabase WHERE userid='$userid' AND ipaddress='$ip' limit 1"); print "About to execute query: $sql<br>"; $delete = mysql_query($sql) or die (mysql_error()); print "Query succeeded"; exit(0); Once your debugging is done you can remove the prints and the exit. I would also check to make sure there are not duplicate entries in your ipdatabase table with the same userid and ip address. Yes, the script will finish before the redirect. That is my experience anyway. If you produce a lot of output and your web server sends that output before your script has finished, you might get redirected before your script finishes. Safest would be to use ob_start() as Zurev suggested. I also agree with Zurev that setting the session variables and then redirecting will work.
-
Have you tried simpler recursive functions? I think you need more practice with recursion before tackling this, because the basics are not there. You can try factorial, reverse (make an array with the elements in the opposite order), "sum of array values" and "count of array values".
-
The header redirect must be put first, but it will not take effect until your script finishes. I would try printing out your SQL to make sure it's correct (make sure you disable the header redirect when doing this or you won't see it).
-
Try calling divide_array(array()) and see what happens. And try divide_array(array(1)). In both cases it loops forever until it crashes. One of the most important things with recursion is how to handle the base case, which in your situation would be an array of length 1 or length 0. You should probably have a special case for each of these. The other important thing is to ensure that each recursive call ALWAYS has a shorter array than what you were originally called with.
-
Edit: I wrote this at the same time as johnny's reply. PHP's bitwise operators work on integers, so you can use them for no more than 32 bits at a time (or 64 bits if you have 64 bit php). You might need to make your own bit manipulation functions. If I was doing it I would probably make a function which takes binary data encoded as a string, and does bitwise operations on 1 character at a time. Something like this: function string_or($a, $b) { $a_len = strlen($a); $b_len = strlen($b); $r = ''; for ($i = 0; $i < min($a_len, $b_len); $i++) { $r .= chr(ord($a{$i}) | ord($b{$i})); } if ($a_len > $i) $r .= substr($a, $i); if ($b_len > $i) $r .= substr($b, $i); return $r; } Note how chr() and ord() let you convert a character into an integer and back. $str{$i} gives you the i'th character from a string.
-
Remote login, source code scraper bot - Curl help needed.
btherl replied to Nuv's topic in PHP Coding Help
Yes i have.It doesn't work. What happens? And how do you determine the correct request to make after logging in? Did you find it from the HTML source, from a snooping add-on like LiveHTTPHeaders, or some other method? Ill look into it.Never worked with Perl before. The awesome thing about WWW::Mechanize is it will not only keep track of your cookies, it will also parse the html and let you select links by name or link text, and let you choose and submit a form without requiring you to parse it. People have tried to make an equivalent for PHP but there's still no real alternative. At my workplace we call perl scripts to do this sort of work, then pass the result back to PHP. -
kclark, can I get one more data item from you please, the output of this: print "Number of results: $num_results<br>"; This can go just after $num_results is set.
-
Remote login, source code scraper bot - Curl help needed.
btherl replied to Nuv's topic in PHP Coding Help
Have you tried doing another curl request using curl_exec() immediately afterwards, using the same $ch ? If Perl is an option, WWW::Mechanize is more suited for this kind of task. -
kclark, have you checked $num_results? You cannot extract a row unless $num_results > 0. When you join tables together using "INNER JOIN", results will only exist if there is data in ALL joined tables. If any table has the data missing, you will get nothing.
-
Can you use LiveHTTPHeaders to see the full request being made in firefox and post it here? A request has a few parts - there will be a line like GET /index.html HTTP/1.0 Then there will be the headers Host: lirr42.mta.info User-Agent: Mozilla/4.0 and so on. Then finally is the content, which looks like: RequestDate=2010-10-01&Foo=bar Apparently there is a curl_getinfo() function which can tell you what headers curl sent, though I have not used this myself. http://www.php.net/manual/en/function.curl-getinfo.php . It needs you to set an option before making the request. If this works, you can use it to check for differences in the headers. Some difference won't be important, but some might be.
-
What method did you use to verify that your requests are identical? Are the headers also identical?
-
webguync, did you want to insert data to the database or select it from the database?
-
help, with postcode splitting function / arrays...
btherl replied to ja_blackburn's topic in PHP Coding Help
What does check_form_postcode() return? Try calling it directly with known values, and then use var_dump on the result: $return = check_form_postcode("ABCDE"); var_dump($return); exit(); What should your code do if the postcode check fails? Also check the manual page for explode(): http://php.net/manual/en/function.explode.php . The arguments should be the other way around. -
That sounds like something for javascript.
-
Here you were using $files in the loop, instead of $files_per_page. The best way to debug a problem like "rsort() is not sorting" is to add this code: var_dump($files); # Show pre-sort data rsort($files); var_dump($files); # Show post-sort data exit(); And then stare at the output.. you would then see that it actually was sorting, and realize the bug is elsewhere. By repeating this process further down the code you can find the point at which the data changes from being what you expect to being not what you expect, and that's where the bug is. I would make this modification to sasa's code: $files_chunks = array_chunk($files, $max); $files_per_page = $files_chunks[$page - 1]; ... foreach ($files_per_page as $file) The behaviour is the same, but this makes it clear that array_chunk() produces chunks, and $files_per_page is one of those chunks. It's my habit to create a new variable for something which is a processed version of something else, so I don't get confused later on and think it's still the original. In any case he has fixed the bug in your latest version.
-
You would start with the page number and the number of galleries per page. $page = 1; $galleries_per_page = 6; Since you want to show the galleries in reverse order: $files = rsort($files); # rsort() instead of sort() Then to find the galleries for a page: $files_for_page = array_slice($files, ($page - 1) * $galleries_per_page, $galleries_per_page); For page 1, the array offset is (1 - 1) * 6 = 0, which is the $min for page 1 in your existing code For page 2, the array offset is (2 - 1) * 6 = 6, which is the $min for page 2 in your code. $max in your code is what I am calling $galleries_per_page.