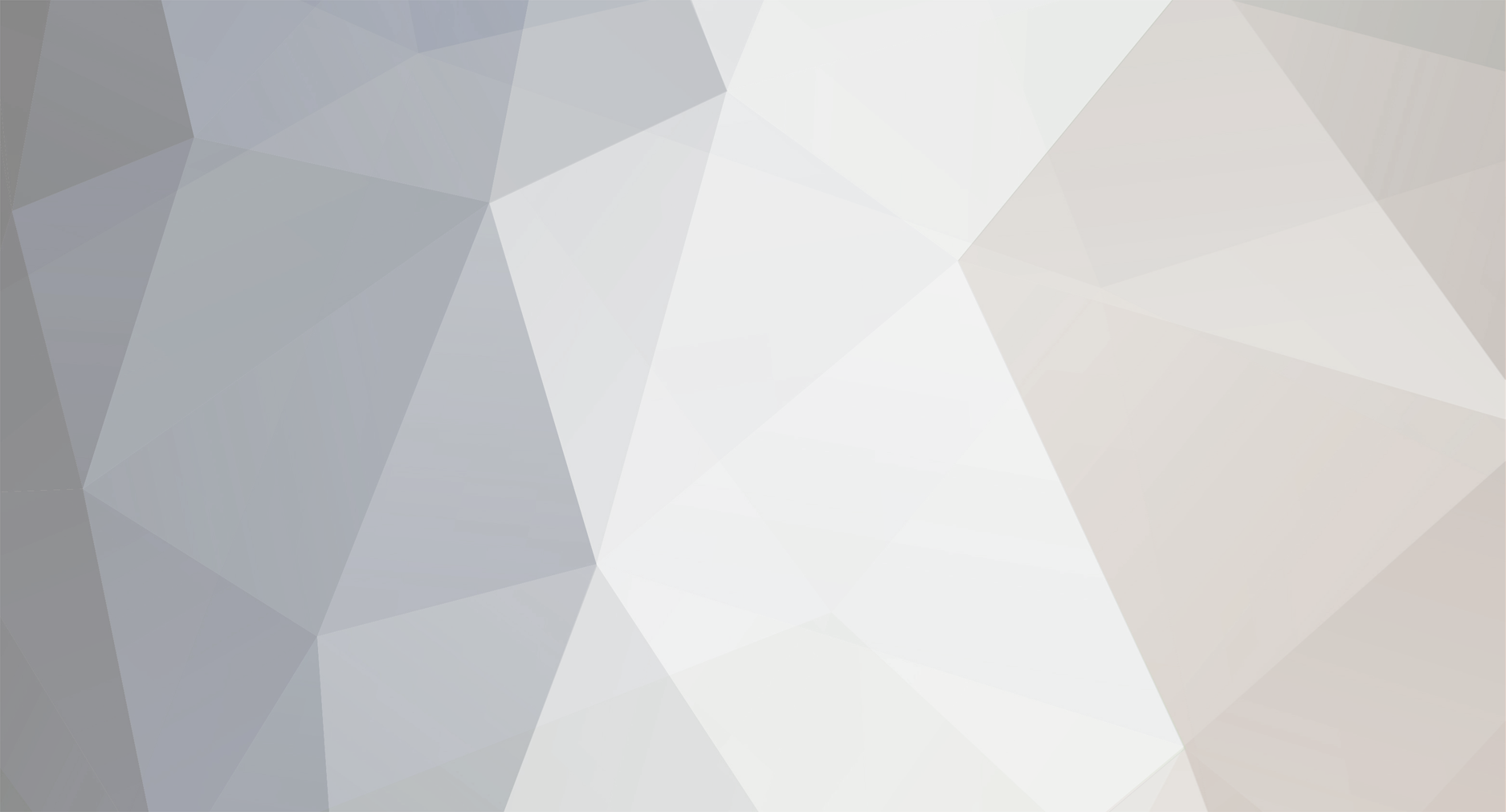
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
[SOLVED] Is this a good structure for a database?
roopurt18 replied to anon's topic in Application Design
When you have content getting slammed by millions of people every day expecting millisecond response times, then you can start thinking about spreading DBs across multiple servers. -
Then maybe: DELETE t1.*, t2.* FROM tableName1 t1, tableName2 t2 WHERE t1.msg_id IN (1, 2, 3, ..., n) AND t1.msg_id=t2.msg_id
-
What are the joining columns on these tables? You want something like: DELETE t1.*, t2.* FROM tableName1 t1, tableName2 t2 WHERE t1.id IN (1, 2, ..., n) AND t1.col=t2.col From the SQL documentation: For the multiple-table syntax, DELETE deletes from each tbl_name the rows that satisfy the conditions. In this case, ORDER BY and LIMIT cannot be used. So remove the LIMIT.
-
Echo the SQL query and paste it here.
-
As has been suggested, the best place for real-time help is an IRC channel. I used to hang out in #c #c++ #math on the efnet network. In any such channel, read the rules and always check the FAQ first; this information will be posted in the channel topic. Never ask to ask a question, that's just silly. If you've looked all over the place and can't find what you're looking for, rather than asking for help with the direct problem in chat, describe what you'd like to do and ask what topic that falls under. Chances are someone will tell you to google "a particular phrase" and they'll respect you more if you seem like the kind of person that doesn't just want to be spoon fed. (EDIT) Oh yah, NEVER post code into chat. Fastest way to get banned.
-
Let's say you have an array of integer IDs where each ID corresponds to a record in a table. You can easily delete all of the records corresponding to those IDs in the array: <?php $IDArr = Array( 1, 3, 5, 7, 9 ); $sql = "DELETE * FROM tableName WHERE tableName.id IN (" . implode(', ', $IDArr) . ") LIMIT " . count($IDArr); $q = mysql_query($sql); ?> Hope you can apply that to your situation.
-
You should be able to run multiple online games at a time through the same cable / DSL connection without causing much lag. So unless you are on dial up, I doubt it's his online game that's hogging the bandwidth.
-
Whether the bus is short or long, you still can't take half a bus.
-
Practice word problems. In grade school I hated word problems because I didn't understand their purpose. Let's say you're learning to add and subtract, I always considered a worksheet with 30 problems on it more effective than one with 5 word problems. I thought if I understood the core concept I could do any of the word problems. Well, here is the example that taught me the difference when I was about 12: 220 students are going on a field trip and each bus can hold 40 students. How many buses are needed? Simple! 220 / 40 = 5.5 I felt like a moron when the teacher asked me how I was going to take 1/2 a bus. The real world is nothing but word problems.
-
Thank you for your insightful input, it is much appreciated. Yes, of course. I should have labeled my code examples as "loose" PHP or psuedo-code; I was expressing what I wanted to do in the simplest terms to get the point across and wasn't concerned with correctness. This is a really good question. In the first site I wrote I don't recall ever having to use more than one at a time; however I'm not sure if that's because it was well-organized or because the site itself was rather simple. In my work project I find myself occasionally having to use more than one DAO at a time but I think that's because the project itself is unorganized in some areas. I think I'd like to plan for the ability to use more than one at a time if the need arises. I actually did this in the original site I wrote, but my work designs software for home builders in the U.S.; it is unlikely we will need to support this product outside the U.S. for at least a few years. The expanded code would just be a wrapper function that calls the corresponding function in the instantiated DAO object. Let us assume the following public methods in each of our objects: UserDAO Create() Delete() isValidUser() ProjectDAO Create() Delete() isValidProject() Then the instantiated DAOInterface object would have the following public methods: Create() Delete() isValidUser() isValidProject() Each of these methods would have essentially the same body, here is the psuedo-code: function lambaFunction(){ $funcName = get the name of this method if( this method does NOT exist in our active DAO object ){ return FALSE; } $args = get the args passed to this function return $this->_active->$funcName($args); } The one disadvantage that comes to mind with this approach is the extra overhead when calling functions; while I have no testing to back this claim up, my gut instinct tells me this extra overhead would not cause a major performance hit. I can think of a couple advantages off the top of my head. From the caller's point of view, it simplifies things greatly. The calling application only has to manage a single variable for all of its DB interactions. Also if I wanted to add code that needs to be called before and after every DB access function, then this approach makes that very simple. Just change the code of the dynamic function in one place and I'd be done. Traditional methodology would dictate the use of singletons or a DAOInterface object that returned instantiated objects like you suggested. My goal here is to simplify the DB interactions as much as possible in the calling application at the expense of extra complexity under the hood. I've never come across an implementation like the one I've proposed and I don't know if that's because it's been tried and failed or if it's because I'm thinking way outside the box.
-
I've an idea about creating the DB abstraction layer on a new project and wanted to get some feedback before I went ahead with it. I've only ever worked on two web sites; one I built from the ground up for my guild in WoW and the other I inherited from another programmer at my place of employment. The website I built from the ground up was PHP4 and I built my DB layer before I learned about the class keyword; as such the DB code was very procedural. I had a base file, mydb.php that had functions like: mydb_query, mydb_select, mydb_insert, etc. Then I created a new file for each interface I needed to expose; for example the file db_users.php with functions: users_get_all, users_create_user, etc. The website I've inherited at work is also PHP4 and takes a different approach. There is an instantiated $db object which exposes methods like insert, select, etc but it is declared globally. Each interface is a class declaration that is used statically; for example class UsersDAO with methods UsersDAO::getUsers, UsersDAO::createUser, etc. Since $db is declared globally, each method has to start with global $db. The second approach is obviously better than the first, but they both suffer from the same drawback. If the interface requires its own internal constants, they have to be created with define statements; I would much rather use static properties to avoid naming collisions. They both also use global variables; the first uses individual globals for the mysql connection, user, pass, etc. while the second uses a single global definition. The second approach also has the drawback that there are many files that might need UserDAO.php, so require_once('UserDAO.php') might appear many times in code. I do not like this because it is redundant work and I have read somewhere there are performance implications with many calls to require_once. Also, some of the DAO class and method names become quite long, leading to extra typing. The logical step in eliminating the extra typing is to create a variable for each DAO interface, but then I'm left with a bunch of variables floating around everywhere. So here is my solution to all of the above and what I'd like to hear your opinions on. This is intended to be run on PHP5. Create a DB class: <?php class DB { function constructor(){ } function query(){ } function insert(){ } function select(){ } // etc. } ?> Create a DAO class: <?php class UserDAO { function constructor(){ } function createUser(){ } function deleteUser(){ } function isValidUser(){ } // etc. } ?> Create another DAO class: <?php class ProjectDAO { function constructor(){ } function createProject(){ } function deleteProject(){ } function editProject(){ } // etc. } ?> That is fairly straightforward, here is the tricky part. In my program I want to have a single instantiated object that exposes all of the DAO classes. I will call this class DAOInterface and I'll start with how I want my main program to use this class. example.php <?php // Example code that shows how DAOInterface exposes the DAO objects within the program. require_once('DAOInterface.php'); $dao = new DAOInterface(); // Instantiate our object // Assume example.php needs to work with both the UserDAO and ProjectDAO interfaces... $dao->loadDAO('UserDAO'); $dao->loadDAO('ProjectDAO'); // At this point, the $dao object should expose all of the public methods contained within UserDAO // and ProjectDAO // Tell it which interface to use $dao->useDAO('UserDAO'); if( !$dao->isValidUser( 'Bob' ) ){ // isValidUser maps to UserDAO::isValiduser! echo "Bad user: Bob"; exit(); } // User is editing a project $dao->useDAO('ProjectDAO'); $OK = $dao->editProject( 15 /* ID */, "Smoogles" /* New name */ ); if( !$OK ){ echo "Couldn't edit project"; exit(); } echo "Project edited"; ?> And now the magic DAOInterface class: <?php class DAOInterface { // Define some member vars (Using PHP4 syntax to just give a rough idea) var $_db = null, // Our DB object $_daos = null, // Array of loaded DAOs $_active = null; // Active interface function constructor(){ $this->_db = &new DB(); // Create our DB object $this->_daos = Array(); // Init our array } /** * loadDAO * Ensures that the DAO interface is loaded and ready to use * @param string file path & name of DAO */ function loadDAO($dao){ if(!file_exists($dao)){ return; } // Doesn't exist if($this->_isLoaded($dao)){ return; } // Already loaded require_once($dao); $tdao = new $dao(); $tarr = Array(); $tarr["file"] = basename($dao); $tarr["dir"] = dirname($dao); $tarr["dao"] = $tdao; $this->_loaded[] = $tarr; // %% EXPANDED BELOW } /** * useDAO * Set a DAO to be the active DAO */ function useDAO($dao){ $idx = $this->_findDAO($dao); if($idx === FALSE){ $this->_active = null; }else{ $this->_active = $idx; } } } ?> In my loadDAO method I have a comment that says explained below. After a DAO object is instantiated and saved in the internal _loaded array, all of it's public methods need to be added to the DAOInterface object. For each public method of the DAO being loaded, DAOInterface needs to create a public method of it's own with the same name. Each of these public methods will essentially be the same function. The body of each of them will check if the called function exists in the "active" DAO object and if it is, call the function, otherwise return NULL or FALSE. What do you guys think?
-
I've been playing video games for 25 years. I'm 27. roopurt18 Azu
-
The short answer is, "No, it couldn't." I don't know if you've ever had to develop a grammar, but I think you'll agree that the grammar for PHP, C, and C++ combined is more simple than the grammar that represents the English language. Bearing in mind that humans are contextual and computers are literal, if it were possible for a computer to determine what a human intended to do based solely from text input and the context of how something was used, then the compiler / interpreter wouldn't bitch about errors; instead it would fix 99% of them for you. The problem is they can't reliably do that yet, not even with something as "simple" as a programming language. Understanding something through context is a recursive process; whenever we encounter a word or concept that we're unfamiliar with, we look it up and it's explained to us through words and concepts that we are familiar with. To do so with a computer would require a massive database, probably so large that the simplest of queries would take several seconds to run. Think about the complexity of this statement: "The blade is green." Am I referring to a metal blade? A blade of grass? The blade of a weapon? Or my tongue after sucking on a hard candy? You would need to ask me further questions yourself, refer to my previous statements, or take note of this statement in regard to my future statements. And once you finally had a system that could hold a text-based conversation, you would have to program in enough error to represent the errors an average human makes. My fiance is Filipino and speaks tagolog, a language that has no concept of masculine or feminine. Whenever she tells a story, she'll refer to the same person multiple times as any of the following: he, she, him, her, his, hers Or, since we're trying to create a machine that can fool us into thinking it's a human through text, take into account all the shortcuts that people take: gr8 u etc. Like I said, tough nut to crack!
-
I hope I'm using the right jargon, but it sounds like you're trying to create a program that can pass the Turing Test. I've thought about that from time to time, tough nut to crack (which is probably why it's still got the shell on).
-
I just wish there was an MMO, any MMO, that had a PvP server like Mordred in DAoC. Oh that was bliss!
-
I bet it gets hot in that thing.
-
The way I see it, we're trying to cure the symptoms and not the disease. One bullet for each person in marketing IMO.
-
Script can't finish: Memory limit or time limit?
roopurt18 replied to roopurt18's topic in PHP Coding Help
No. The PDFs are generated with PDFLib and the script performs the following operations: 1) Gather data from MySQL 2) Open disk files (images to place in the PDF and other PDFs to import into final PDF) 3) Loop over data from step #1 and create catalog 4) Set headers and dump PDF buffer as output In order to gather data from my tests I do some file logging as well. I suppose the time spent interacting with MySQL and the disk could be the difference. I should also note that get_memory_usage is not defined on my implementation so I'm using a custom one taken from the PHP documentation's user comments. -
Script can't finish: Memory limit or time limit?
roopurt18 replied to roopurt18's topic in PHP Coding Help
Yes, it does. What doesn't make sense is if the PHP timeout is 10s and the Apache timeout is 30s that the script records an execution time of 30s. And if the Apache timeout is 120s and the PHP timeout is 30s, how come the script times out at almost 40s? There aren't any system or shell commands that I'm aware of in this script so all execution time should be spent by the PHP interpretor. -
I have a script which takes user parameters and creates PDF catalogs that can't always finish. I think the bottleneck is a PHP or Apache configuration setting and ran some tests, but I don't know what to make of the results. I started with the following settings and ran the same parameters each time: Apache Timeout: 30 PHP max_execution_time: 30 PHP memory_limit: 8M In each of 5 runs the script was cut short between 30 and 32s of execution time and used between .5MB and 6.5MB of memory. I then changed PHP's max_execution_time to 10. In each of 3 runs the script was cut short between 30 and 32s of execution time and used between .5MB and 1.5MB of memory. I then changed PHP's memory_limit to 1M. In each of 3 runs the script was cut short between 30 and 32s of execution time and I had one run that reported 9.5MB of used memory. I then changed Apache's Timeout to 10. In each of 2 runs the script was cut short between 10 and 12s of execution time and had memory usages of 6.14MB and 9.15MB. So at this point I thought, "Aha! It's Apache that's causing the problem and it doesn't even appear that my settings in php.ini have any effect." I then changed Apache's Timeout from 10 to 120. Two runs of the script were cut short between 37 and 40s of execution time, each using about 9.5MB of memory. The third was able to finish in 37s of execution time using 1.08MB of memory. I then changed PHP's max_execution_time from 10 to 120. The script then ran successfully 3 times, each time taking between 36 and 40s of execution time and 2 of them using 9.28MB and 1 of them using 1.06MB. So now I'm really confused. On the way down, modifying php.ini didn't seem to have any effect. But on the way up, it seemed to be the setting in php.ini that made the difference, but only after I had made the change in Apache. Here is a log of my tests. The ones that finished have the text end _EndDocument() at the end of the line. [pre] Time: 30.93 Mem: 0.51MB Group: APPL Time: 30.87 Mem: 2.68MB Group: STRU Time: 31.43 Mem: 6.46MB Group: ALLW Time: 30.00 Mem: 0.58MB Group: PLUM Time: 31.66 Mem: 2.69MB Group: APPL CHANGE PHP MAX_EXECUTION_TIME FROM 30 -> 10 Time: 30.85 Mem: 1.38MB Group: SOLID Time: 31.50 Mem: 1.36MB Group: ALLW Time: 31.66 Mem: 0.75MB Group: WIND CHANGE PHP MEMORY_LIMIT FROM 8M -> 1M Time: 31.01 Mem: 9.49MB Group: GRA3CM Time: 30.98 Mem: 1.25MB Group: APPL Time: 30.68 Mem: 1.29MB Group: PLUMFX CHANGE APACHE TIMEOUT FROM 30 -> 10 Time: 11.20 Mem: 6.14MB Group: LAMI Time: 10.76 Mem: 9.15MB Group: GAR CHANGE APACHE TIMEOUT FROM 10 -> 120 Time: 37.92 Mem: 9.59MB Group: PLUMFX Time: 39.83 Mem: 9.56MB Group: LOWV Time: 37.43 Mem: 1.08MB end _EndDocument() CHANGE PHP MAX_EXECUTION_TIME FROM 10 -> 120 Time: 37.95 Mem: 9.28MB end _EndDocument() Time: 36.13 Mem: 9.28MB end _EndDocument() Time: 39.18 Mem: 1.06MB end _EndDocument() ### GOING BACK TO CTI'S WITH CURRENT SETTINGS ### PHP MAX_EXECUTION_TIME 120 ### PHP MEMORY_LIMIT 1MB ### APACHE TIMEOUT 120 Time: 79.07 Mem: 22.97MB end _EndDocument() [/pre]
-
A singleton is an object of which only one instance can exist at any given time. I take it you chaps didn't read the tutorial or have a great sense of humor.
-
I've written a short tutorial on creating singletons within Javascript. I'm posting it here for a couple reasons. The first is that I'm tired and likely made a mistake; if anyone notices any errors I'd like to hear about them. The second is it might help the readers of this forum. http://rbredlau.com/drupal/node/8 Cheers!
-
Does linux have a command similar to preg_replace()? I have a grep command dumping output into a file but I'd like to reformat the output by replacing certain bits of text (found with regexps) with other bits of text to make viewing in a web browser more convenient. Thanks!
-
That was pretty funny.
-
Then why are you having this discussion with people that already know the difference? Write letters to the biggest media companies instead of piddling around here increasing your post count.