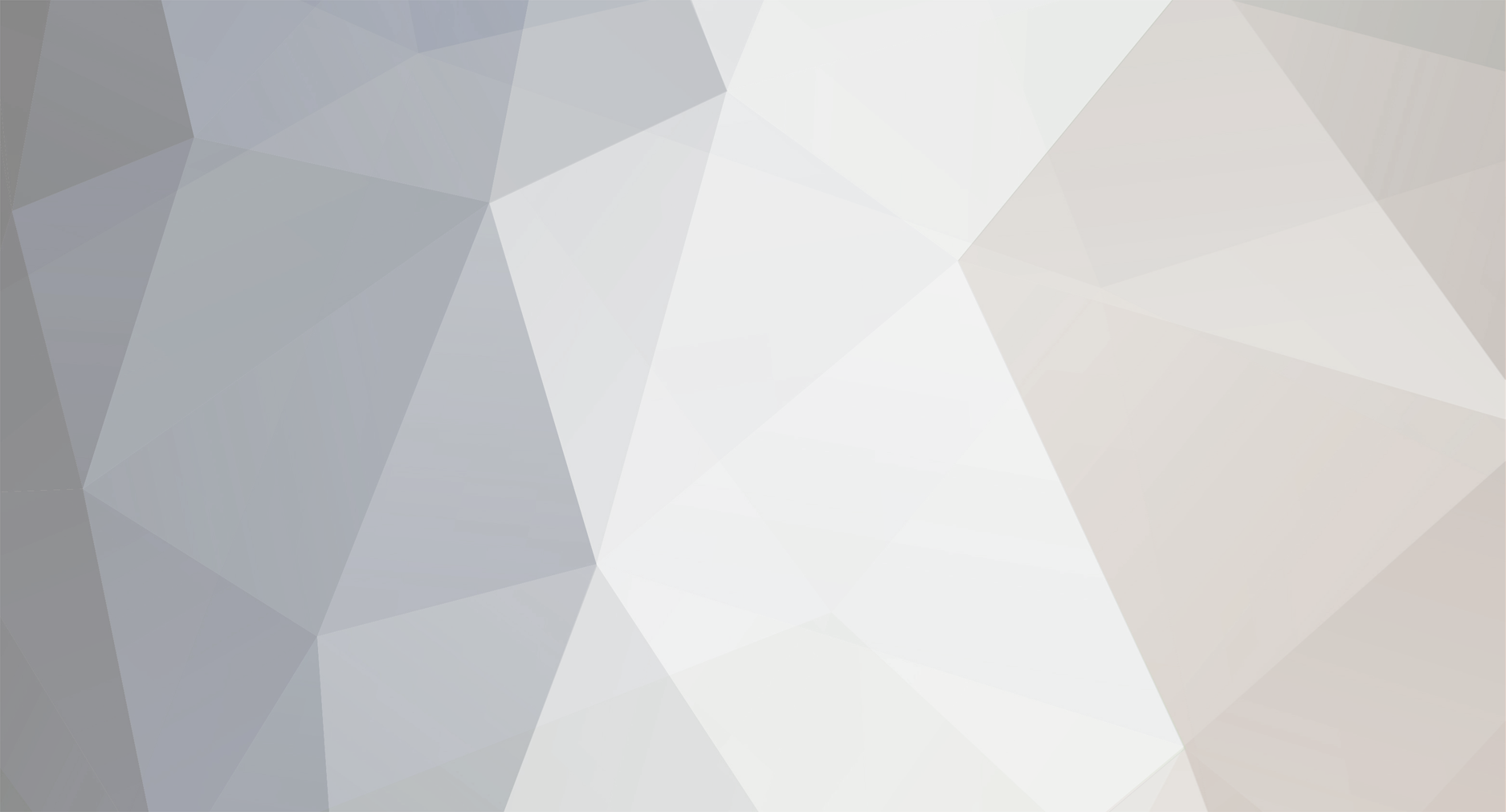
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
The underlying problem is you're trying to battle a bad database design through programming. You should break this apart into at least two tables, potentially three (if you plan to have repeat customers). order_desc id date requestor student_body district grant_or_other vendor_name addr1 addr2 phone fax order_items id order_id quantity catalog desc price ext_price You place the general order information (your top fields) into the order_desc table. Then you insert each item in the order into it's own row in the order_items table. For each item row inserted, you also insert the order_id the item was attached to. Note that I've intentionally left out the shipping estimate because this is something you should calculate on the fly and display to the customer but you may not really need to store it in the DB. I've also left off the total column because you can calculate an order total based off the items table, although you may want to add a column to the order_desc table indicating how much the customer was charged. You may find this Wiki article helpful: http://en.wikipedia.org/wiki/Database_normalization
-
So why do you need to add extra columns to your DB table? Your table should have at least 5 columns: quantity catalog desc price ext_price And probably an internal column: id Why does your table already have 30 columns? There's a good chance you're not normalizing your database. Post your table layout like someone suggested earlier and you might get some real help.
-
Convert the value you created earlier into a timestamp and use: WHERE date_field >= $begin_date AND date_field <= $end_date
-
How about a link to an example page of what you're trying to accomplish? From your description so far it sounds like you're going about this all wrong.
-
A couple of items: You're not sanitizing your values before putting them in your query. Why are you using 'SELECT * FROM' when the only values you're using in the loop are team_id, team_name, and team_rank? If there are extra fields in the table, or if you add fields later, you are just pulling out extra data you'll never use. 'SELECT team_id, team_name, team_rank FROM' would be better IMO. You could probably do this in one query with some form of UPDATE ... SELECT, although I'm not sure how exactly. Lastly, just because the script is simple doesn't mean it's not suitable as a cron job.
-
Why don't you just implement the script as a cron job? Then you don't have to log in as the admin and waste your time waiting for the script to finish. Even if you implement a progress bar, eventually you will reach a point where there are so many teams that the script takes longer than your webserver's timeout period.
-
[SOLVED] What does it take to make you turn down a contract?
roopurt18 replied to obsidian's topic in Miscellaneous
I would say it depends on your current or potential workload. If you have a choice between client A, who represents the scenario you're describing, and client B, who represents the scenario that you get to create a truly wonderful site worth adding to your portfolio, I think it's a no-brainer. You might even be able to turn their bad design to your advantage if the client is happy. Let's say you had stayed on board with that project. You could still add it to your portfolio with the phrase "implementing their design." That clearly states that you had no part in the design, only the implementation, and you always want to be associated with a happy client. Also, as a CYA policy, if you are really against the design, I'd have "implementing their design" as part of your contract. That way, if they later realize how much their design sucks but you've already done a ton of work, you won't be stuck with a loss having to redo the design on your own dime. -
When I built my last system I opted for 2 of the smaller WD 10K RPM raptor drives and set them up in a RAID stripe. So my system only has 140 GB of storage, only ~60 of which is ever occupied, but HDD access is fast. Long story short, I recommend 10K RPM drives.
-
running php processes for extended periods of time
roopurt18 replied to tobycatlin's topic in Application Design
Is your word processor set up so that it only saves files that have been modified? If I were to open an article in your word processor, not change anything, and close it, does it rewrite the XML file to disk? If the answer is yes, you need to change that behavior. Because then your script can keep track of the last time it ran and only modify files with a timestamp greater than or equal to the last time the script ran. I also suggest trying to reduce the input size so that there are less files to process and each file takes less time. It's still unclear as to what magnitude of complexity your algorithm is using while processing these files, so perhaps that can be optimized as well. -
I bought this book: How to Wow: Photoshop CS2 for the Web http://www.amazon.com/How-Wow-Photoshop-CS2-Web/dp/0321393945/ref=sr_1_6/103-2697599-1496625?ie=UTF8&s=books&qid=1177367134&sr=1-6 From the user reviews it appears you'll either love it or hate it. I didn't use it for much more than the first set of tutorials, but after doing those and reading a couple of Photoshop magazines I know enough to get stuff done.
-
running php processes for extended periods of time
roopurt18 replied to tobycatlin's topic in Application Design
You didn't really answer my questions about the origins of the XML file you are parsing. So here we go again: Is this a single XML file? In your original post you refer to it as a big xml file but in your second post you refer to each xml file describes. So now I'm unclear as to whether you're working with a single file or multiple files. What are the origins of the XML file(s)? Are they created by your software or some one else's? Do you have any control over the format / content of the XML file(s)? I asked previously if the XML file contains only new information or if it contains everything you may have processed previously as well. From your latest post, it sounds like the file contains old data that may or may not have changed. If a large portion of your XML file is mostly static data that changes infrequently, you're wasting an awful lot of time processing a file of data you've already processed before. If possible, you should set the XML file up so that it only contains data that needs to be inserted or updated and eliminate all data that hasn't changed. Even better would be to separate the contents of the XML file into two files: insert.xml and update.xml. Everything in insert.xml should be guaranteed to be a new article that requires insertion into the DB; everything in update.xml should be guaranteed to be data that is changed and requires updating. This reduces the amount of database checking your script has to make. How are you going about reading the file's contents into memory? Are you loading the entire file into a single variable and then parsing that? If so, I'd be curious to know what's happening with your page file usage. You could be killing your system by trying to load the entire contents at once. What kind of benchmarking do you have in place? Do you even know which part of the script is eating up the most time? Without proper benchmarking, you have no idea if its spending 80% of its time running DB queries and 20% doing file I/O or what's going on. Without knowing where it's spending it's time it's hard to optimize. The last thing I can think of is what does your table set up look like? More specifically, what types of indexes are declared on your tables? Hope some of that helps. -
Now that is an interesting idea.
-
My first site was done in 4 and my work site is set up in 4 as well. My latest personal project, of which I've not written any code yet, will be done in 5.
-
running php processes for extended periods of time
roopurt18 replied to tobycatlin's topic in Application Design
My first step in addressing this issue would be to optimize the process in any way possible. I'm curious as to the XML file itself. How big is the file? Does it only contain new information or is it a file that continuously grows in size as data is appended to it? I would also take a look at the operations your script is executing and in which order. You don't need rewrite the whole thing, but perhaps re-ordering some of the code will lead to faster execution times. As for invoking the script, you really have two choices. Either you do it from the command line or from the browser. As Patrick pointed out, directing the output to /dev/null will mimic a fork on *nix systems and is one option when invoking a script that takes several hours or even days to execute from a browser. The other choice would be to set up a cron job that searches a DB for a flag to start executing the script and setting that DB flag through the browser. However, due to the execution time required for your script I think your first step should be to optimize where possible. If you provide more details about the stuff I asked in the beginning of the post, I may be able to give you some ideas. -
Another factor to consider is the site's purpose and audience. If the site is for a hobby or your audience is friends and / or family, then people will be more patient. Which is to say it is acceptable if some of your pages take a little longer to reload. Now if the site is for paying customers and you expect traffic to be heavy, then it's really worth spending more time optimizing. But you never, ever, go about optimizing willy-nilly without a purpose. When you optimize code you need to target specific areas. If the average time for a page to generate is 2.3 seconds (which is a long time mind you) and you have a couple that are taking as long as 10 seconds, then it's worth looking at those couple pages that are taking a long time. There is usually a trade-off between code optimization and readability and maintainability; often, the more optimized a portion of code is the harder it becomes to easily read and maintain later. The common practice when optimizing is to target a specific area that is performing poorly, isolate the segment that is causing the poor performance, and then to optimize the Hell out of just that one segment of code. Hope that helps.
-
I don't worry about the size of my individual files. Computers are fast. Very fast. You would have to have an extremely large source file, probably over the 1MB mark, for it to make a notable impact IMO. What you want to do is try and organize your code so that only the required files are included in each script. There's no sense in including 3 or 4 .php files for DB stuff if the current script doesn't interact with the DB. The things I'd look for optimizing before I started worrying about the size of my files would be my algorithms and database set up. Adding the proper index to a sluggish query can be the difference in your query requiring 10 seconds to execute or .03 seconds to execute. The same thing holds for algorithms, which are the steps you take to accomplish your programs task. I.e. instead of looping over a set of 250 items three times, you'd be better off re-organizing your code so that you loop over the set at most once or instead cut the set down to only the items you need.
-
Mine stems from a guy I knew in high school named Matt. At the beginning of the year the teacher asked him if he had a nick name he liked to use. "Most people call me Rupurt." Which was total bullshit, but the teacher bought it and everyone called him Rupurt for that year in just that class. When I later went to sign up for a hotmail account, I couldn't think of anything else and just used roopurt18; the 18 is because I was 18 at the time. I actually have a pool of several user names that I use for various reasons. rbredlau First letter of first name followed by last name. Usually I don't need any digits because its rather unique. I use this mostly for things I need to keep semi-professional or personal. superfiend I use this as my login account for just about every video game. I've never named a video game character named superfiend, I just use this as the global name to access my account. sk.1, tdg|w00t I used these names back when I was big into Quake 2. sk.1 stood for a fake clan named Silent Killers where in my config file I had it set up so that I couldn't talk to anybody else on the server. I used that handle for well over a year and, true to my motto, never spoke to anyone. I used to get kicked and / or banned from a lot of servers because I was good at the game and many people thought I was a bot or at least cheating in some way. Also, I like to get under peoples' skin and consistently beating someone in a game and [i]never[/i] talking to them is a great way to do it. tdg|w00t was my identity when I wanted to talk to people; tdg stands for Too Damned Good and w00t is just an expression of happiness. The fun thing about that little experiment was watching the effect it had on people; the same people that liked me as w00t would be the first to try and votekick me as sk.1. Good times! Continuing my interest in themes, I tend to use odd names in CS:S whenever I play that: The Best I use this whenever I know I'll do good. LaP|Friend I use this on occasion, it's my guild and character back when I played WoW. [Arnold]Pump*YOU*Up I use only the pump shotgun when I use this handle. And the list goes on. So there you have it, some insight into the madness that is my brain.
-
Code optimization often entails undoing maintainability for a small portion of code. Maintainability and security are two different topics. There are plenty of books you can purchase about PHP or MySQL security. Maintainability is a broader software design topic, so you'd want a book that deals with software design in general to learn more about that.
-
Ninja points and young maidens. Hot, young maidens.
-
Jebus, if only there were destructors or an ondestroy event.
-
An algorithm that uses a rotation would yield more predictable results. An algorithm that assigns priority based on age would also be useful. Who wants to modify SMF to make it happen! Not it! Not it!
-
Look up classes in the documentation. The this keyword is pretty standard for all OOP languages.
-
I've heard that argument myself and quite frankly, it annoys the crap out of me. There's a couple of factors that go into a pricing a product: 1) It has to at least break even. If your product isn't breaking even your business won't last long. 2) You have to pay your employees. If you can't pay your employees competitive wages, they'll go work for your competition. After 1 and 2, the final factor in determining pricing is: 3) How much can we get away with charging? Once you're breaking even (or earning profit), you can afford to ask yourself how much people are really willing to pay. A price increase from this factor usually results in a split of money. Some of the extra money coming in goes into R&D for the company, some of it goes into the company execs' pockets. I'm perfectly fine with all this. When I create software, I don't do it because I'm bored with nothing better to do. I have bills to pay and I want to retire early. So I have no problem with others that do the same thing. I don't mind paying money for a software product that I utilize and makes my life easier. I don't mind paying $.99 per song if I enjoy and listen to it often either. What I really hate is the price of movie tickets and the movie industry is another that uses similar logic quoted at the top of this post. Yah sure, the reason movie prices went up is because teenagers download movies on the internet; never mind the fact that any cinaplex has a billion adolescents milling about outside on any given Friday / Saturday. I don't think movie prices have anything to do with the millions of dollars <random movie star> gets paid or the insane markup on condiments at the snack bar. I still don't steal / download movies though. I just wait, at most 6 months, for them to hit DVD and rent them with Blockbuster Online. The other thing we all have to remember is every business plans for a specific amount of revenue to be lost to stolen / pirated goods. If the cost of goods / services lost was greater than the cost of enforcing legitimacy, it would be harder to steal them.
-
The news is board dependent for me. Right now it says: "News: We are constantly trying to improve phpfreaks and these forums, so feel free to go to the PHPFreaks Comments/Suggestions board and point out anything you'd like to see different!" EDIT: Well, it doesn't appear to be page or board dependent. But it's definitely inconsistent. EDITx2: Looks like it picks a topic at random on each page.