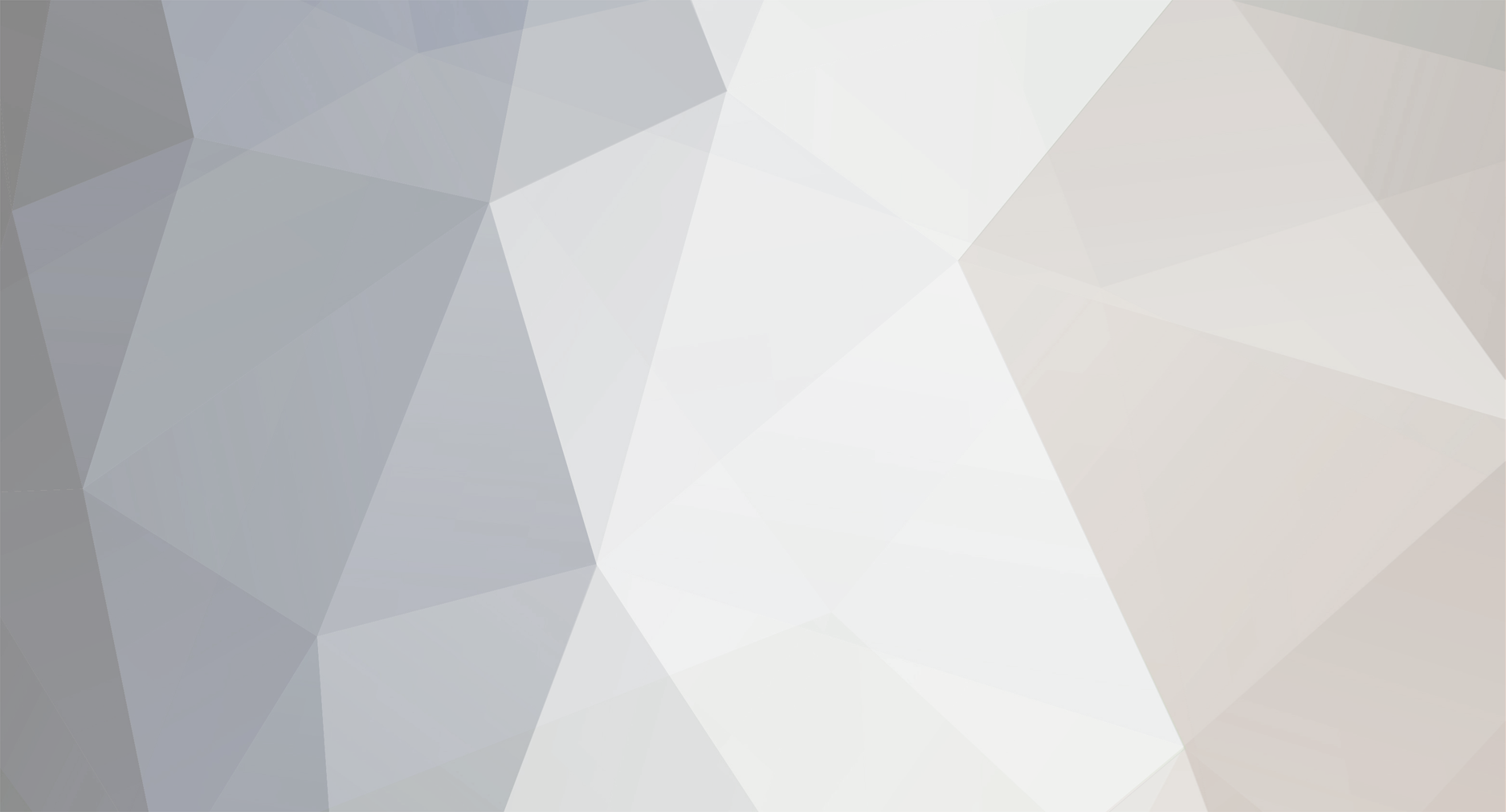
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Thinking about this more, I can get around the potential timer problem by enabling the client to disable / enable the timer. // This code is going to detach and re-attach a node, so we must disable Events // from cleaning it's event stack during this time. var node = document.getElementById("someNode"); Events.stopCleaning(); node = node.parentNode.removeChild(node); document.getElementById("someOtherNode").appendChild(node); Events.startCleaning(); But if I'm going to place this extra level of burden (2 function calls) on the client, I might as well just not have the self-cleaning feature with the timer. Instead, I could just add a single function: Events.removeNodeEvents(node); that removes all events registered for this node through my interface. The client would then need to be responsible enough to remember to call this function before permanently removing a node from the document. Basically the burden on the client is lessened from two function calls to one. The interface also eliminates the timer using this method. I'd still prefer a method that doesn't require any intervention by the client though, so I'll keep thinking about it.
-
Like I'm going to notice news thats 8 pixels tall and only visible on the home page. 8P
-
I agree. However, it takes money to make money, as they say. And purchasing tools that continue to make me money is a good investment, as well as tax-deductible.
-
Back when I was a poor student and had no money, if I couldn't get it at student discount I rarely paid for it. But now that I have my feet on the ground, yes, I do pay for software. After all, what kind of human would I be if I expected people to buy my software but give me theirs for free? Also, I'm planning on releasing some of my own online applications so it's important to ensure everything about them is legit. No need to get entangled in some nasty lawsuit.
-
I purchased photoshop a while back and while it was expensive, only CS2, and I use only a fraction of photoshop's features, I don't regret it. I bought two books, one based on using photoshop for web design and another outlining type effects, and a few magazines. All-in-all I spent around $825, which is a big hit for me, but now I'm confident I can do my own web design or at least come up with a basic design to pass off to a professional, if I needed to. @ober, when I installed linux on my windows box using VMWare, I showed my penny-pinching fiance Gimp and said, "Look baby, free photoshop!" She just about had a heart attack.
-
I'd never seen a lightbox until someone made that post asking what it was called on this board, but I have to say I do like them. You could always create a "Customize this site" link and give people the option of which method they'd like to use. Also, Melissa Burnos is quite the hottie.
-
Noticed you have a Zend certification avatar now, if that's a recent development then "Congrats to you, Sir!" Otherwise, just ignore me.
-
This question is related to Javascript but I felt it was more of a design question so I'm placing it here; feel free to move it if necessary. I've been working on a Javascript event wrapper to unify the interface between those browsers that support the DOM Level 2 specification and the world's favorite browser. Here is a brief example of some Javascript using my wrapper: if( Events.supported() ){ // Event wrapper is supported // We'll add two handlers to an element with id="testID" Events.addHandler("testID", "mousedown", myMousedown); Events.addHandler(document.getElementById("testID"), "mouseup", myMouseup); } function myMouseup(oEvent, oNode){ oEvent = new Events.Event(oEvent, oNode); alert(oNode.id + " mouseup!"); } function myMousedown(oEvent, oNode){ oEvent = new Events.Event(oEvent, oNode); alert(oNode.id + " mousedown!"); } Now you will notice a couple things about this wrapper: 1) The first parameter to Events.addHandler can be either a string or a DOM object (that supports event handling) 2) My event handlers take two parameters: oEvent and oNode. oEvent is what you would expect, the event object in DOM L2 browsers and null in IE. oNode is the node the event was originally attached to; this is primarily to help resolve the difference between the following possible properties of the event object: srcElement, target, and currentTarget. It's also handy because 99% of the time the event handler needs to work with the node associated with the event anyways. I also need to mention that my Events interface internally assigns all events to an anonymous function: function Events.addHandler(n, e, h){ /** * n DOM Node * e Event name (string) * h Event handler (function) */ // Do some stuff // Create the event handler var handler = function(oEvent){ var oNode = n; h(oEvent, oNode); } // Attach event if( /* IE Event Model */ ){ n.attachEvent( "on" + e, handler ); }else if( /* DOM L2 Event Model */ ){ n.addEventListener( e, handler, false ); }else{ // Unsupported return false; } return true; } At this point you're probably slapping your forehead, "Roopurt, you idiot! You can't detach events assigned as anonymous functions!" Well, actually I can. The last step in attaching an event handler is to create an object that records the event that was added: var e = new Object(); e.node = n; // Record the DOM Node e.handler = h; // Record the function the client passed in e.actual = handler; // Record the anonymous function we assigned e.event = e; // Record the event name This object is then pushed onto the back of an Event Stack array, which is a private variable of my Events interface. Later, when the Events client wishes to remove the handler, it can call Events.removeHandler with the same arguments passed to Events.addHandler. In the removeHandler function, the interface will search for a matching Event Stack object and detach / removeEventListener to remove the event. Long story short, a client to Events can do: Events.addHandler("testID", "mousedown", myMousedown); Events.removeHandler("testID", "mousedown", myMouseDown); I'll flatter myself and say the whole method is rather genius except for one thing: I'm stuck over the best way to handle the case where a client to Events adds a handler to a node and, before the handler is removed, the node is removed from the document via removeChild or replaceChild. When this occurs, my Events interface will be stuck with an Event Stack object in its array that will never be removed and no longer has any business being there. My initial way to overcome this obstacle is to set up a function on a timer that will search this array for objects who contain a node whose parentNode / parentElement is null, signifying the node has been removed from the document. There are two problems with this approach: 1) I don't like timers. 2) More serious, just because a node is removed from the document doesn't mean it won't be re-inserted. If the node is removed from the document and then re-inserted after the Event Stack object is removed, the client will have no way of removing the event! My other idea, which I've not yet tested and don't know if it's possible, would be to override the default removeChild and replaceChild methods so that when they're called my Events interface will automatically remove Event Stack objects for that node. However, the one potential problem I can see with this method would be if the client set the innerHTML property of a node such that an internal node with an assigned event was erased I don't know that either of these methods would trigger. This problem is less worrisome though because I don't use the innerHTML property for more than testing. Can anyone else offer up any other alternatives to overcome this problem? P.S. While writing this I had a light-bulb moment. I'm going to change my anonymous event handler to: var handler = function(oEvent){ var oNode = n; oEvent = new Events.Event(oEvent, oNode); h(oEvent); } I'll make oNode become a property of my Events.Event object. This will allow all of my event handler functions to become: function some_event_handler(oEvent){ // oEvent is now an instance of my event object from the Events interface. // I no longer have to worry about if oEvent is set or if i should use the window.event object // YAY! }
-
An oldie but goodie. I'd seen this years ago and almost forgotten about it until it popped up again. I laugh harder every time.
-
I Love the MS iPod one. I even left my first Youtube comment; I knew the name of the song! Buahahaha
-
I see your dilemma; unfortunately I don't think you'll find what you're looking for in most books or JC courses. Most books or classes for learning a language touch on barely more than that language and a few simple scenarios. I think what you really want is a course or book that touches on a high-level concept such as algorithms, theorems, graphs, abstract data types, etc. I've never really seen any such courses offered at the JC near my house so it may be hard to find an appropriate course that you can easily enroll in. There are plenty of books out there but most of them read like mud. As an example: Here's the Wiki on Big-O: http://en.wikipedia.org/wiki/Big_O_notation And if you found that enthralling, here's the standard text for computer algorithms: http://en.wikipedia.org/wiki/Introduction_to_Algorithms Most of that material you would never have a need to use in PHP, but suffering through it may allow you to create more efficient programs than the other guy. You could probably go your whole programming life without knowing what Big O is or most of what they teach you in college, but having that knowledge will occasionally give you a big edge over other programmers IMO. The other option would be to look into titles that deal with advanced problem solving or advanced techniques with a particular language. I've not purchased such a title but I'm sure you could pull some practical information from one.
-
I took a few programming courses at JC almost a decade ago and I was very pleased with their quality. However I wouldn't consider taking one now because they're no longer time efficient for me. A course will draw the learning experience out over the course of a semester and you'll be forced to spend a lot of busy time coding the examples. The way I learn now is I just pick up and plow through a book and skip writing any code or reading the code examples. My goal is to go through it quickly and make a mental checklist of basic ideas: the language supports looping, the language supports anonymous functions, the language supports objects, etc. Then when I want to accomplish a specific task within that language I'll open the book back up, look for a specific example illustrating what I want to do, and just apply it to my current problem. I find this works well with more than just learning a language; I've used the same technique for books on security techniques and database design. Currently, I pick up a book on whatever topic I'm interested in (AJAX atm) and when I get about 60-70% of the way through the book I buy another. Most of my collection are from O'Reilly, but my AJAX book is from wrox and I'm quite happy with it as well. I also read a few web-related magazines here and there to stay in the loop on what other technologies exist. Ultimately you have to find a learning technique that is cost and time-effective for you.
-
I do that all the time in Javascript; drives me crazy.
-
To do this in PHP requires the user's page to reload. If you want to do it in a more up-to-date method, use Javascript and CSS.
-
[SOLVED] returning mulitple variables from a function
roopurt18 replied to s0c0's topic in PHP Coding Help
As a simple example, let's say your class represents a rectangle. It would have the properties [i]length[/i] and [i]width[/i]. It would also likely have the methods [i]getArea()[/i], [i]setWidth()[/i], and [i]setHeight()[/i]. In order to understand why a constructor is useful you have to understand languages other than PHP. In PHP, you don't have to declare or initialize variables. It's considered [i]bad programming practice[/i] to do so, but you can almost always count on PHP to initialize your variables to empty or zero. As an example, if $var is undeclared and you do: [code] $var += 5; $var will then hold the value 5. This is because PHP will declare and initialize your variable for you. Now let's look at a typed language such as C++. In C++ you must declare your variables and you should initialize them as well. In order to understand why this is the case, you have to understand how variables work. When you create a variable in a program, your interpreter or compiler grabs a chunk of memory out of the available memory in the computer. That memory that is grabbed is available now for your program, but it may not have been available 2 minutes ago; 2 minutes ago it may have been in use by another program and that program may have left a value in that memory. So in a language such as C++, when you declare a variable: int my_num; There is no way for you to predict what value that variable holds. It could be 5, it could be 10, it could be -32,322. You can not safely use this variable in any calculations. The following code will most likely crash your program in C++: int compute( int param ){ int my_num; // UNINTIALIZED!!! return param * my_num; // UNPREDICTABLE RESULT } Going back to our rectangle class: class Rectangle{ var $length, width; // Not defining a constructor! function setWidth($val){ $this->width = $val; } function setLength($val){ $this->length = $val; } function getArea(){ return $this->length * $this->width; } } When you create an instance of this class in PHP, the rectangle's width and height will most likely be set to zero for you. Calling getArea() on this instance would return 0 as well, which is fairly safe (but still bad programming practice because you are relying on the interpreter to perform steps for you). If someone in C++ creates an instance of that class and then calls getArea(), there is no telling what value they will get back. The length and width properties have not been set, so the return value of getArea is unpredictable. Enter the constructor: function __constructor(){ $this->length = 0; $this->width = 0; } Now it is guaranteed that if someone in C++ creates an instance of Rectangle without specifying a width and length that when they call getArea they will get a predictable result. This is a necessary requirement for them to write a program that uses this class. Hope that helps. P.S. Yes, I'm aware of the syntax differences between a class in PHP and C++; I kept it simple on purpose. P.P.S. Always provide a constructor and always initialize your variables before using them, in any language, and regardless of whether the compiler will initialize them for you. You never know when your code will be moved to an installation or platform that doesn't initialize them or uses a different initialization value, so provide one yourself.[/code] -
[SOLVED] Fathers tries talking son out of career in development
roopurt18 replied to s0c0's topic in Miscellaneous
That's a great point as well. Basically, developers that are hot shit get a lot of kudos. I have a game programmer friend who lives in So. California but works for a company in San Francisco. He's so far above and beyond most of the other developers that they begged him to come back when he left and when you're in that position you have a lot of power. So now he works from home and goes to San Fran only when they're about to release a title. He has to be near the phone while he's at home, but he only really works about 2 hours per day as far as I can tell. And he can get away with it because in that two hours he produces more and better working code than other programmers at the company. -
[SOLVED] Fathers tries talking son out of career in development
roopurt18 replied to s0c0's topic in Miscellaneous
I'm one course away from a B.S. in computer science and a minor in physics and only working 35 hours / week at my current job. The job was advertised as entry level but I'm the only developer working on the project so in addition to PHP, MySQL, Javascript + AJAX, I also do application / DB design and maintenance, UI design and implementation, and everything else associated with the project. I'm currently at 59k / year and this is my first job. I actually got very lucky with this job; I expected it to be entry level where I just document or debug code that already exists. But since I'm the only programmer I have a lot more responsibility and freedom which equates to more useful experience IMO. I figure if I pull another year here and I could get another job, regardless of degree, at 70k / year at least as one of the main developers of a project. -
I'm taking this history course at JC to finish my degree; on Valentine's the teacher brought a huge bag of chocolate in. When I wouldn't take any and everyone asked why, my response: "Chocolate's period food."
-
What you look for when buying something
roopurt18 replied to The Little Guy's topic in Miscellaneous
I usually do my own side-research when making a big purchase, such as product reviews in magazines, forum posts, etc. However, for most other things, I love neweggs system, especially if I'm juggling between a couple of similar items. -
Agree 100% with what ober said. My father tried to run his own (non-programming) business for about 15 years and it was brutal on the whole family. When he had lots of jobs lined up, he pulled 10 to 14 hour days 6 (or even 7) days per week. When he had none instead of enjoying himself he had to do paperwork and office-type crap. You never knew how much he'd make in a given month which makes it hard to enjoy the finer things in life and most of the time we didn't have medical or dental; I went 7 years without a trip to the dentist. Moral of the story for me: Work for a faceless corporation. Now, having said that, I do have plans to launch a website of my own in the next 6 - 12 months that I'm working on in my spare time. The goal of the site is to provide some supplemental income for a bare minimum of time invested, but it has potential to take off. If the site reaches a point where its worth more money to me to devote more time to it than my current job, I'll negotiate with my employer to work contract on the side and run my site full-time. There's nothing wrong with running your own company or doing freelance work, but you shouldn't rely on it as your only source of income until you've learned the science of it.
-
Googling 'xmlHttpRequest', top hit => http://en.wikipedia.org/wiki/XMLHttpRequest Googling 'xmlHttpRequest w3schools', top hit => http://www.w3schools.com/dom/dom_http.asp
-
You need Java applets (or possibly ruby?) to gain access to the user's machine; Javascript has no permissions to browse files, at least not in the way you're thinking of.
-
The rule of half and all: They own half of everything and have all of the p!@#$. Except my fiance calls it the rule of all and all.
-
n/m, I got it sorted.
-
I have two web servers that I'd like to give the ability to ssh into each other; I can already access each of them from my development PC but I need them to talk to each other. So far I've tried (using cPanel): Host 1: View Private Key <key_name> Host 2: Import Private Key I then copy the contents of the private key into the input box and import the key with <new_key_name> Now from the CLI I run: ssh -i ~/.ssh/<new_key_name> <host_address> and receive the "The authenticity of host ... can't be established." I'll keep banging my head against it, but if anyone could point me in the right direction I'd appreciate it.