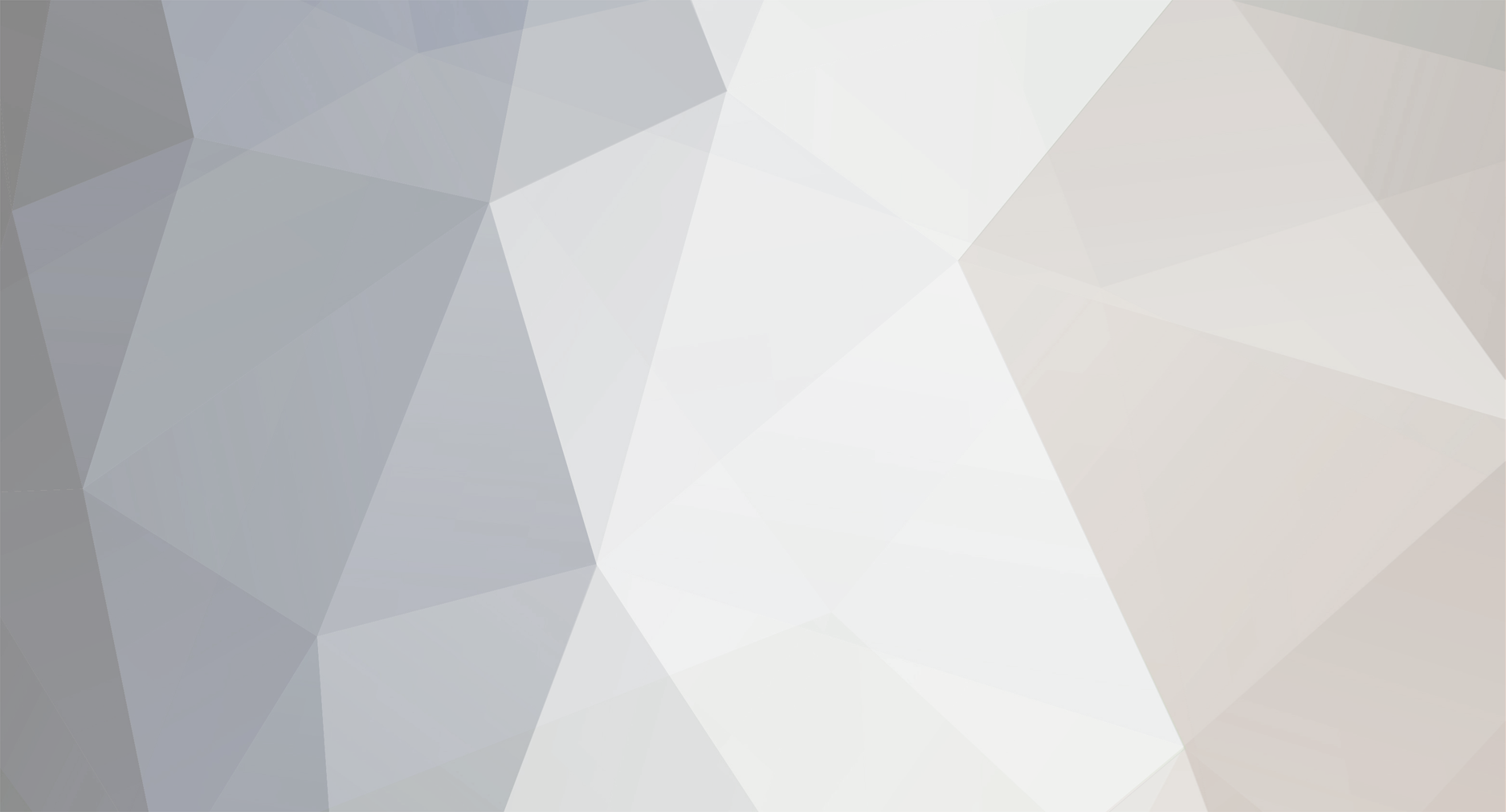
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
A session will take care of it.
-
How do you build a query from a number of search fields
roopurt18 replied to 86Stang's topic in PHP Coding Help
I realize you may be past this point in your search for help, but I usually build queries like so: <?php /** * Demonstrates query building with all fields required * * @param string $last_login * @param string $user_group */ function find_user_by_login_and_group( $last_login, $user_group ) { // validation $last_login = strtotime( $last_login ); if( in_array( $last_login, array( -1, false ), true ) ) throw new Exception( '$last_login is invalid' ); if( ! preg_match( '/[a-z][a-z0-9_]+/i', $user_group ) ) throw new Exception( '$user_group is invalid' ); $last_login = date( 'Y-m-d H:i:s', $last_login ); // escaping $last_login = mysql_real_escape_string( $last_login ); $user_group = mysql_real_escape_string( $user_group ); $select_stmt = " SELECT * FROM `users` WHERE `group`={$user_group} AND `last_login`>={$last_login} "; // Now run the query... } /** * Demonstrates query building with optional fields * * @param string $fname * @param string $lname * @param int $age */ function find_user( $fname = null, $lname = null, $age = null ) { $wheres = array(); if( is_string( $fname ) ) { if( strpos( $fname, '%' ) !== false ) $join = ' LIKE '; else $join = ' = '; $wheres[] = "`fname` {$join} " . mysql_real_escape_string( $fname ); } if( is_string( $lname ) ) { if( strpos( $lname, '%' ) !== false ) $join = ' LIKE '; else $join = ' = '; $wheres[] = "`lname` {$join} " . mysql_real_escape_string( $lname ); } if( is_int( $age ) ) { $wheres[] = "`age`={$age}"; } $wheres = count( $wheres ) > 0 ? " WHERE " . implode( ' AND ', $wheres ) : " "; $select_stmt = " SELECT * FROM `users` {$wheres} "; // Now run the query... } ?> -
Doctype: Reality/Transitional
-
What's this print: <?php $haystack = explode( ' ', $desc); $haystack = array_unique( $haystack ); echo '$haystack<pre>' . print_r( $haystack, true ) . '</pre>'; echo '$desc_array<pre>'.print_r( $desc_array, true ).'</pre>'; if(count(array_intersect($haystack, $desc_array)) > 0 ){ echo $dbtime; } else{ echo 'nope<br>'; } ?>
-
if( count( array_intersect( $haystack, $terms ) ) > 0 ) echo $dbtime;
-
I'm not sure it'll be any faster. But you could try: // $haystack contains text to search // $terms is array of terms to search $haystack = explode( ' ', $haystack ); $haystack = array_unique( $haystack ); $found = array_intersect( $haystack, $terms ); print_r( $found );
-
You do not ever, ever, not in a million years, display_errors on a live production web site. So do not follow the suggestion given by $Three3. Instead look into set_error_handler(), which allows you to define your own error handling function. Then you can log the errors to a file that only you have access to. Or e-mail them to yourself. Or any number of alternative solutions that do not involve letting the general public and would-be hackers see the innards of your website. In all of my code, production or development, I have: ini_set( 'display_errors', 'off' ); error_reporting( 0 ); set_error_handler( 'my_own_error_handler' ); My users get to see nothing.
-
One of my favorite memories from 7th and 8th grade were when groups of ill-tempered students would physically injure the kids whose birthday it was on that given day. Good intentions gone awry by the school's administrators.
-
IPs are also shared. Hard-linking a user to an IP address is never a good idea.
-
Actually that last line is saying: .* -- Match any characters any number of times \. -- Match a single dot (jpg|jpeg|gif|png|bmp|pdf) -- Match any one of jpg, jpeg, gif, png, bmp, pdf $ -- Match the end of the line All of those essentially say match any request ending in .jpg, .jpeg, .gif, etc. csedinc.com -- Redirect to this location R,NC -- No redirect code specified, NC means no-case (or case insensitive) So the match would also match: .JPG, .PdF, etc So altogether the rule is saying any requests that look like images should go to http://csedinc.com (which loads an index page of some sort I'd guess, which is why the images are broken) There's common rules people place on image requests. I'm not sure what the intention of that rule is because it just blocks all images no matter who requests them. Essentially it's probably malformed.
-
Can I open a new page when the form processing completes?
roopurt18 replied to SteveMann's topic in PHP Coding Help
Good work on answering a solved topic by repeating what's already been stated. -
Is there any server-side way to make a background color print?
roopurt18 replied to DWilliams's topic in PHP Coding Help
Trying to make professional reports in HTML and CSS is a fool's errand. There are libraries that can convert HTML and CSS into a PDF; you might look into that as a way of displaying the page. I use BIRT to create reports. -
Can I open a new page when the form processing completes?
roopurt18 replied to SteveMann's topic in PHP Coding Help
http://www.phpfreaks.com/forums/index.php/topic,37442.0.html -
You don't need to program anything. You can do this all with wget which is a free utility. But if the mobsters game has capcha built in you're SOL.
-
Ah! I see now. Well, the easiest thing to do is e-mail the admin of the Mobsters game and ask him to give you the credentials to his database. Then you can easily write a PHP script to attack your enemies. However, since what you want to do is probably considered cheating by the game's designer(s), you're going to have to defeat whatever counter-measures they've put in place to stop would-be hackers. In that case the info you need can be found at: http://www.i-wanna-be-a-script-kiddie.com
-
You didn't answer my question. Are you creating A your own original Mobsters game? Or are you B trying to interact with the existing Mobsters game? A or B, which is it?
-
For interacting with MSSQL, take a look at ODBTP. It works better than ODBC and even talks to FoxPro!
-
Can I open a new page when the form processing completes?
roopurt18 replied to SteveMann's topic in PHP Coding Help
header( 'Location: newpage.php' ); exit(); -
WHERE CONDITION_A OR ( NOT CONDITION_A AND CONDITION_B )
-
Are you trying to write your own Mobsters game? Or are you trying to write a script that hacks the existing Mobsters game and forces all accounts in the entire game to attack someone who was mean to you?
-
$userIPAddress = $_SERVER['REMOTE_ADDR']; and then "SELECT id FROM users WHERE ip = '$userIPAddress'; You realize IPs change?
-
What's the code on your login page?
-
Oh. I see now why it's not performing the verification. <form action = "changepw.php" method = "POST"> Your form is a POST, yet in your code you are doing: $submit = strip_tags($_GET['submit']); $newpw = strip_tags($_GET['newpw']); $newpwrt = strip_tags($_GET['newpwrt']); Do you see the problem there?
-
Is this a good database structure for a forum?
roopurt18 replied to corrupshun's topic in PHP Coding Help
I don't understand the meaning of Date or IP in the users table. You don't need separate forums and topics tables. Just one table, called Topics, would suffice. This would be a hierarchical table where topics can contain any number of sub-topics. Any topic without a parent is effectively a board. There is a good article on the MySQL website about hierarchical data organization; a google search should turn it up. -
New Password: <input type = "text" name = "newpw"><br> Repeat Password: <input type = "text" name = "newpwrt"><br> Both should be: type="password" Unless you like friends and coworkers looking over your shoulder to know what your passwords are.