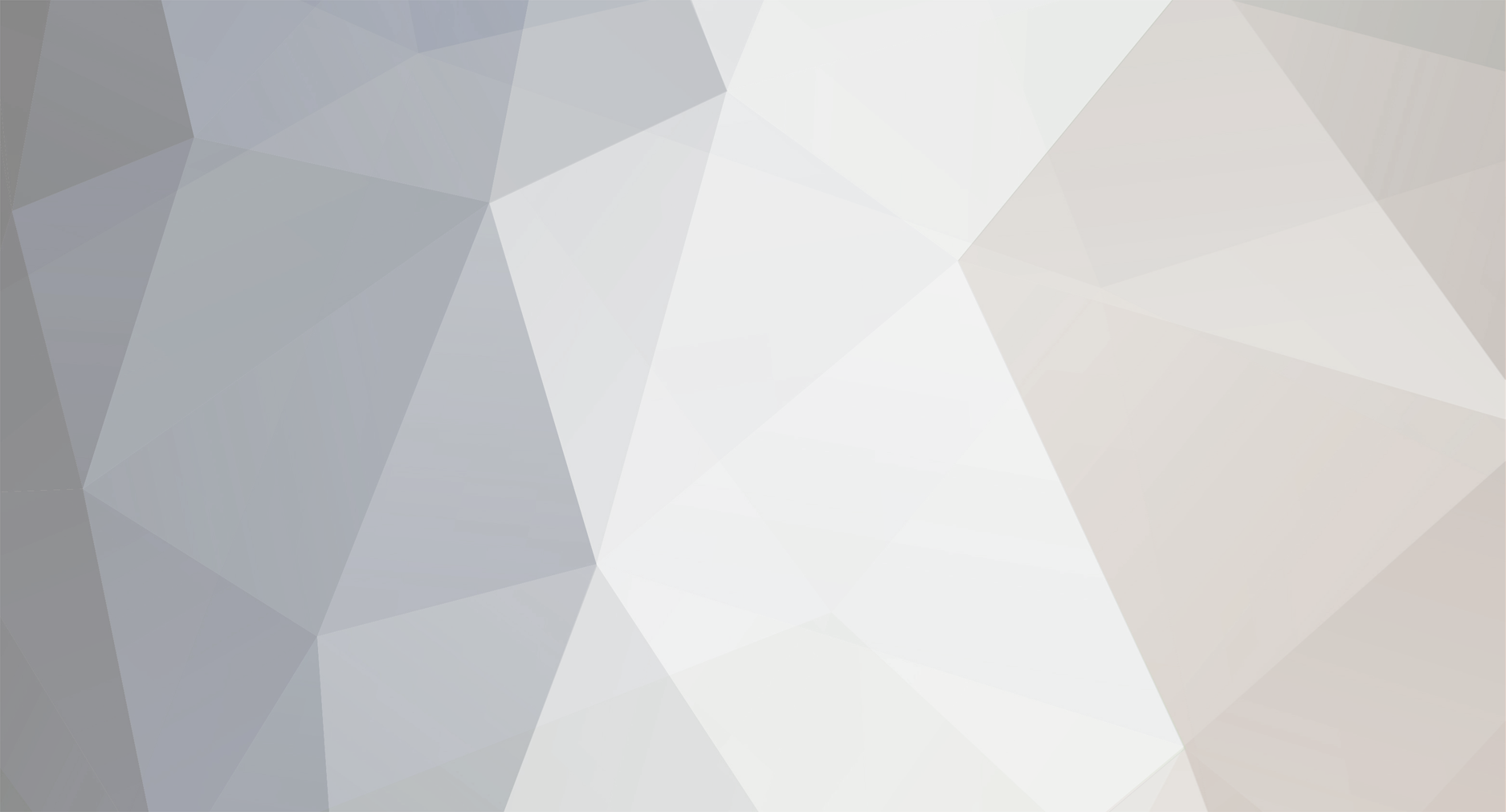
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
[SOLVED] passing null varchar value from php form to mysql
roopurt18 replied to nomis's topic in PHP Coding Help
<?php // Assume connect to mysql is made if( empty( $_POST ) ) { // // DISPLAY FORM // Here we retrieve value from database based on GET parameter named 'id' $sql = "select `column_name` from `thetable` where id='" . mysql_real_escape_string( $_GET['id'] ) . "'"; $value = ''; // Initialize value to empty string $q = mysql_query( $sql ); if( $q ) { $row = mysql_fetch_object( $q ); if( $row ) { $value = $row->column_name; // If we made it this far then the value was retrieved } } // if value has zero length it is set to zero-length string // this effectively turns raw NULL from the database into empty string $value = !strlen( $value ) ? "" : htmlentities( $value ); // dump the form echo <<<FORM <form action="{$_SERVER['REQUEST_URI']}" method="post"> <input type="text" name="txtTest" value="{$value}" /> <input type="submit" value="Submit" name="btnSubmit" /> </form> FORM; }else{ // // INSERT INTO DATABASE // Extract value from $_POST values if it's there and positive length; // otherwise we'll insert a NULL into the database $value = isset( $_POST['txtTest'] ) && strlen( trim( $_POST['txtTest'] ) ) ? "'" . mysql_real_escape_string( $_POST['txtTest'] ) . "'" : 'NULL'; $sql = "insert into `thetable` ( `column_name` ) values ( {$value} )"; mysql_query( $sql ); echo mysql_affected_rows() > 0 ? 'success' : 'failure'; } ?> -
[SOLVED] passing null varchar value from php form to mysql
roopurt18 replied to nomis's topic in PHP Coding Help
1) User submits the form. 2) NULLs go into the database. 3) The form reappears, some fields say NULL in them. 4) User submits the form again. 5) The database now has the string 'NULL' in it. Is this what is happening? -
[SOLVED] passing null varchar value from php form to mysql
roopurt18 replied to nomis's topic in PHP Coding Help
I'm not sure exactly what you mean, but trim(), rtrim(), or ltrim() might be what you're looking for. $var = "'hello there'"; // 'hello there' echo trim( $var, "'" ); // prints: hello there echo ltrim( $var, "'" ); // prints: hello there' echo rtrim( $var, "'" ); // prints: 'hello there -
[SOLVED] Okay, I can't seem to wrap my head around this...
roopurt18 replied to applejuicerules's topic in PHP Coding Help
The code I gave you, exactly as it is, displays: Array ( [2007-07-29] => 1 [2007-06-29] => 1 [2007-08-29] => 2 ) So if given an array strictly of dates it can count how often each date occurs in the array. I see no reason why you can't modify it to your needs. Or if necessary you can do a print_r() on your data so we can see how it's presented and help you on your way. -
[SOLVED] passing null varchar value from php form to mysql
roopurt18 replied to nomis's topic in PHP Coding Help
You're printing $middle_name if it is EQUAL TO null. Instead you want to print it when it is NOT EQUAL TO null. if( $middle_name != null ) <-- NOT EQUAL -
That depends on your form. You told us they were submitted like tag1, tag2, tag3 so I showed you how to split and insert them if there is a variable holding a string like that. Just replace my $tags = with something like $tags = $_POST['tags'].
-
While I agree with phpSensei in theory, in practice the less people know about the internals of your site the better. I see no reason to use code such as this: mysql_query( "select ..." ) or die( mysql_error() ); One of the first things I do on any new project is: error_reporting( 0xffffffff ); set_error_handler( 'my_custom_error_handler' ); function my_custom_error_handler( /* whatever the arguments are */ ) { // append the error to a file } It doesn't look exactly like that but since you're going to be setting up error logging anyways for production you might as well use it all the way through. Then my code would look more like: $q = mysql_query( $sql ); if( !$q ) { trigger_error( $sql . ' caused error ' . mysql_error() ); } Now I only have to refer to my error.log to see what went wrong and I don't have to worry about giving away more info than I intend to visitors.
-
And don't forget to call session_start()!
-
1) Your function needs to be available to the code that wants to use it. You do this by using require_once() on the file. <?php // this is accounts.php require_once( 'functions.php' ); $mem = theMem( 1 ); print_r( $mem ); 2) Your function needs to return a value. [code]function theMem($id){ $sql = "SELECT * FROM members WHERE memID = '$id'"; $rs = mysql_query($sql); $rw = mysql_fetch_assoc($rs); return $rw; } Also, if you use mysql_fetch_object() instead of mysql_fetch_assoc, you'll have less typing to do. $r = mysql_fetch_assoc(); echo $r['id']; $r = mysql_fetch_object(); echo $r->id; <-- easier to type IMO
-
[SOLVED] passing null varchar value from php form to mysql
roopurt18 replied to nomis's topic in PHP Coding Help
Just a small point. It's generally a good idea to include curly brackets even though they might be optional. The rationale is you may later add a statement (such as for debugging) and then forget to add the curly brackets. Then your code may do all sorts of weird things and you'll go on some pretty wild goose chases. if (!empty($_POST['middle_name'])) { $middle_name = "'$middle_name'"; }else{ $middle_name = 'NULL' ; } -
<?php $tags = 'tag1, tag2, tag3, ..., tagN'; $tags = explode( ',', $tags ); foreach( $tags as $k => $v ) { $v = "'" . mysql_real_escape_string( trim( $v ) ) . "'"; $tags[$k] = "( {$v} )"; } $insert = "insert into `tags_table` ( `tag` ) values " . implode( ', ', $tags ); echo $insert; // You should know what to do from here! ?>
-
The way you've come up with is probably the most simple to implement.
-
The latest version of Excel will warn users before opening the file though. Fine for a free site maybe, but not in a business environment. I've used each of the following to create Excel files and they work great: http://pear.php.net/package/Spreadsheet_Excel_Writer http://www.codeplex.com/PHPExcel
-
extension_dir doesn't change in php.ini
roopurt18 replied to WalterItinOrient's topic in PHP Installation and Configuration
I would just use Add / Remove programs to remove PHP altogether and use regedit to make sure it's gone. Then extract a .zip distribution and add the path to your environment variables. Then you'll have less problems most likely. -
Create a new SELECT tag. Give it a class="has_other" Add an option value="other" to it Add an input after the new select. Give the input class="hidden other_specifier" Refresh the page and it should just work. As long as the input comes in the DOM after the select and you assign the proper classes it'll just work.
-
<style type="text/css"> /*input.other_specifier {*/ .hidden { display:none; } </style> <!-- assume class hidden means css: display: none; --> <!-- I had the class attribute listed twice, it should be --> <input class="hidden other_specifier" type="text" id="txtRace" name="txtRace" value="" size="10" /> And you have to add Dojo to your project if you want to use it. http://www.dojotoolkit.org/
-
http://www.pandora.com is another similar service that used to be free. They changed recently to 40 free hours per month and then you have to pay $0.99 for the rest of the month and you'll still hear ads. If you want it completely without ads it's like $36 / year. They do a good job of picking songs for your stations though. It's definitely worth the 40 free hours you get each month. I also listen to: http://www.technobase.fm/ http://www.trancebase.fm/ I'm not really a big techno / trance person but for some reason this type of music really goes well with coding or painting.
-
Heh, I must have brain damage today. include() is a better solution. /facepalm
-
[SOLVED] Okay, I can't seem to wrap my head around this...
roopurt18 replied to applejuicerules's topic in PHP Coding Help
Did you bother to try or look at the code below? -
echo eval(file_get_contents($file); You're missing a closing-paren before the semicolon.
-
function to strip all non-alphanumeric characters?
roopurt18 replied to dadamssg's topic in Regex Help
Doh! -
And your markup will be invalid and can cause all sorts of problems with CSS and JavaScript. But be my guest.
-
Right, right. My mistake. IF( DAYOFWEEK( NOW() )=6, NOW(), CASE DAYOFWEEK( NOW() ) WHEN 1 THEN NOW() - INTERVAL 2 DAY WHEN 2 THEN NOW() - INTERVAL 3 DAY WHEN 3 THEN NOW() - INTERVAL 4 DAY WHEN 4 THEN NOW() - INTERVAL 5 DAY WHEN 5 THEN NOW() - INTERVAL 6 DAY WHEN 7 THEN NOW() - INTERVAL 1 DAY END ) AS `MyValue Maybe? I dunno! I stopped using MySQL years ago.
-
Read the whole file and pass it into eval(). <?php $file = "/some/other/file.php"; eval( file_get_contents( $file ) ); ?>
-
[SOLVED] Okay, I can't seem to wrap my head around this...
roopurt18 replied to applejuicerules's topic in PHP Coding Help
<?php $dates = array( '2007-07-29 00:00:12', '2007-06-29 00:00:12', '2007-08-29 00:00:12', '2007-08-29 00:00:12' ); $count = array(); foreach( $dates as $d ) { $d = date('Y-m-d', strtotime( $d ) ); if( !isset( $count[$d] ) ) { $count[$d] = 1; }else{ $count[$d]++; } } print_r( $count ); ?>