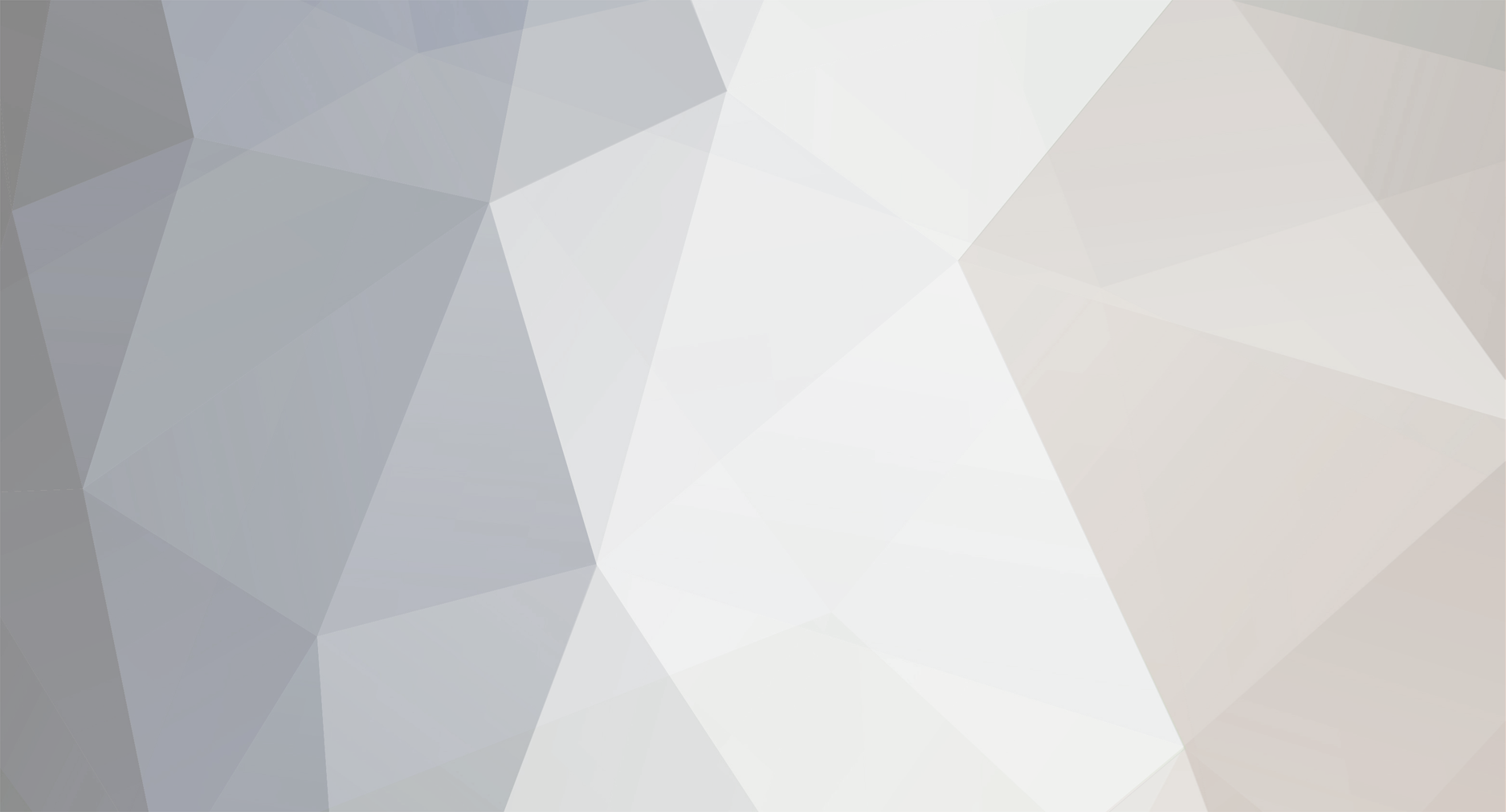
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
<?php // Going to load up a list of dates $dates = array(); $base = strtotime( '1999-12-31' ); for( $i = 0; $i < 200; $i++ ) { $offset = rand( 1, 365 * rand( 0, 10 ) ); // day of year $offset = $offset * 24 * 60 * 60; // seconds into the year $offset = $offset + rand( 0, 24 * 60 * 60 ); // seconds into the day $dates[] = date( 'Y-m-d H:i:s', $base + $offset ); } sort( $dates ); $curYear = null; // keep track of which year we're displaying echo <<<TABLE Year | Rest TABLE; foreach( $dates as $d ) { $stamp = strtotime( $d ); $y = sprintf( "%-10s", date( 'Y', $stamp ) ); // We only display $y as the year IF its not the current year we're tracking if( $curYear === $y ) { // It's the one we're tracking, so zero it out $y = ' '; }else{ $curYear = $y; // Track the new year } $disp = date( 'F jS, g:i A', $stamp ); echo <<<TABLE {$y}| {$disp} TABLE; } ?>
-
DISTINCT selects distinct records; it has no bearing on how the records are displayed. You need to handle that yourself in the code used to display the results. Give me a moment and I'll work up an example.
-
[SOLVED] Query with some type of IF statement???
roopurt18 replied to tekrscom's topic in MySQL Help
AND NOT (Users.Yahoo='' AND Users.MSN='') -
http://dev.mysql.com/doc/refman/5.0/en/select.html How many limits does it say you can set?
-
If there's no records don't output a table at all.
-
ob_start() and echo ob_get_clean() will fix the problem. Since you are dealing with multiple files, where you put them will make a difference. ob_start() should really be at the very beginning of execution and echo ob_get_clean() should be at the very end.
-
Now I know that the headers are susposed to come BEFORE any output Fixed. Is error reporting turned on? Perhaps an error is being reported in production and causing premature output. I see you calling mysql_query() before making a database connection and using or die() which echoes output.
-
If you do not need to perform searching or mass updates on the items themselves, then I'd just store a serialized PHP object in the users table in a single text column. I do this often with things like configuration since asking the question, "How many users prefer a blue background?" does not come up very often. If you do[/i] need to perform searching or mass updates on the items then you're better off with your first choice. Examples of questions that could lead to this situation are: Which users have the same item as I do (and maybe the same level)? Replace item #19283 with item #2919239 for all users Increment or decrement the item level without having to pull anything out of the database Does a certain item occur too often? When doing a select on items, could just pull out this string and use in a where ... in clause. WHERE ... IN( ... ) does not work like that.
-
First off you could add a unique key to the table so they don't get in there in the first place. As far as deleting them, you can do it without the temp table if your existing table has a column that is different for every row, such as a primary key. Does your table have such a column?
-
Instead of creating a bunch of tables, why not create just one table with more rows? Obligatory remark about not using tables for layout.
-
I mean how you can drag Chrome tabs out of the browser and create a "new" browser instance or drag a free-floating Chrome instance back into a group of tabs. I take advantage of this feature a lot since I have dual monitors at work and a 30" at home. It's a feature I've wanted in FF for a long time and Chrome came up with it first (AFAIK). Between that and the faster JS engine I really don't see how I could use FF for primary web browsing. Oh, and I also like being able to see which Chrome tab is sucking the life out of my computer. And if I happen to force close it for whatever reason the rest of the tabs remain intact. For me it's a more fluid and less disruptive user experience.
-
Performance and speed will be based on just how the PHP code is "compiled." Compiled code always executes faster than interpreted code, so it depends on if the PHP "compiler" actually compiles the code or not. That said PHP is extremely fast. I've written a PHP program that loops over 9 million MS SQL records, performs a little arithmetic, and it finished in probably less than two minutes. You can also have user input. On Windows with COM you can also accept strings of text such as passwords in a manner that the user's input will not appear on the screen.
-
I didn't like Chrome's interface at first, but I kept using it anyways because of: 1) Speed in JavaScript applications 2) The ability to drag tabs anywhere I'd like Eventually the interface grew on me and I rather like it now. I'm not sure I want Chrome to have all of the fancy plug-ins that FF has either. Eventually it'll be just as bloated.
-
Chrome works fine in youtube for me...as far as I've noticed.
-
You are limited to what extensions and / or libraries exist for the language. But in principle they are much the same. I write quite a few command line PHP programs for work.
-
How can I convert html file/string to image??
roopurt18 replied to c815902's topic in PHP Coding Help
You can probably do this with the GD (or graphics) extension. It will likely not be an easy task though. Font calculations can be a pain in the ass to deal with. -
Anyways, that is not your problem. mysql_query("INSERT INTO country (`Country` `CountryCode`) VALUES ($countryandcodesdata[0], $countryandcodesdata[1]);") or die(); // ^--- WHERE IS THE COMMA BETWEEN THE COLUMN NAMES?
-
If I had use single quotes would I not be able to refer to call the variable as a value in that PHP string? Fixed. It's a string variable. Regardless of the fact that it also happens to be a SQL statement. You can only put variables inside double quoted strings. It will not work with single quoted strings. $name = 'Larry'; echo "Hello, {$name}." . PHP_EOL; // just one string with variable inside echo 'Hello, ' . $name . '.' . PHP_EOL; // have to use multiple strings and concatenate
-
Selecting random records and holding for pagination
roopurt18 replied to erme's topic in PHP Coding Help
If there are many rows in the database, then benphelps approach will be inefficient and essentially defeat the purpose of pagination. Also, his syntax is wrong. I believe sasa is saying to use the same seed every time you run the query, which will produce the same random values. You just have to track it in the user's session then and that may work. I don't know if MySQL supports them, but you may be able to accomplish this with a cursor. -
My guess is it's the missing comma between `Country` `CountryCode` in your query. The quotes you surround the query with, single or double, have no effect. mysql_query() accepts a string as its first argument and in PHP strings are enclosed in either single or double quotes. In your case double quotes are appropriate since you have chosen to include variables inside the string.
-
It returns whatever you tell it to. If you tell it to return $this->pass, then it returns the value in the pass member. echo $someObj->SetPass( 'asdf' ); // Sets pass to 'asdf' and also echos 'asdf' since SetPass() returns its parameter If you tell it to return $this, then it returns a reference to the object. This allows you to daisy-chain function calls. $objA->SetPass( 'asdf' ); $objA->SetUser( 'joeboo' ); // or with daisy chaining $objB->SetPass( 'asdf' )->SetUser( 'joeboo' );
-
A destructor is the opposite of the constructor. When an object no longer has any references pointing at it, it is automatically destroyed and the destructor is called. So the destructor is a good place to handle any necessary clean up. A Registry is a commonly implemented object. The purpose of the registry is to store references to globally needed values or objects. It's not really, but you can almost think of it as a "safer" way to implement globals without using the global keyword. <?php // example of how I use the registry in my application $cfg = Registry::getInstance()->GetConfig(); // Returns Config object $db = Registry::getInstance()->GetPrimaryDatabase(); // Returns database interface object (PDO, mysqli, etc) ?> The Registry::getInstance() syntax is used because the registry is a singleton. A singleton is an object that is never instantiated more than once (Sometimes it's useful to guarantee that an object can ever have only one instance). You implement a singleton through a combination of static methods and a private constructor(). <?php // A sample singleton class // try and run this code class TheExample { // NOTICE THE PRIVATE CONSTRUCTOR private __construct() { } } // The constructor is private; that means it can not be called from outside the class. // Since the constructor can not be called, we can not instantiate the class. $foo = new TheExample(); // This line crashes ?> <?php class WorkingExample { static private $s_instance = null; // Store a handle to the instance // This function returns an instance of the object static public function getInstance() { if( self::$s_instance === null ) { // If the above is null, then the object has not yet been instantiated. // Since the constructor is private, only methods of the class can instantiate // objects. // So we create one and store the instance to it echo 'Instantiating...' . PHP_EOL; self::$s_instance = new WorkingExample(); } return self::$s_instance; } private $_name = null; private function __construct() { echo __METHOD__ . PHP_EOL; $this->_name = 'Sam'; } public function GetName() { return $this->_name; } } // We need to use the static method to return an instance $foo = WorkingExample::getInstance(); echo $foo->GetName() . PHP_EOL; $bar = WorkingExample::getInstance(); echo $bar->GetName() . PHP_EOL; // $foo and $bar are actually the same instance! Notice how many times the construct was called ?>
-
function CloseMysql(){ $this->mysql->close(); } You probably do not need that function. And the code contained within can be moved to the object's destructor.
-
function __construct($db_name, $db_user, $db_pass, $db_table){ $this->mysql = new mysqli($db_name, $db_user, $db_pass, $db_table); } What happens when you have more classes that access the database? Are you going to keep instantiating mysqli objects? Chances are you only need one mysqli object that can be referenced throughout your code. Consider adding a new class, DatabaseManager or Registry, to create and maintain a single instance of your database connection that other objects / code grabs as necessary. Something like: $db = Registry::getInstance()->GetDatabase(); // returns mysqli $db = DatabaseManager::getInstance()->GetDefaultConnection(); // returns mysqli Your class is making assumptions about the presentation layer by having <br /> tags in it. You'd be better off having an $errors private member that is an array. Each time you have an error, instead of appending to an error string, push onto the $errors array stack: if( /* something fails */ ) { $this->_errors[] = 'Username is invalid.'; } Then provide a method GetErrors() that returns this array. Now your functions can return primarily boolean and if the calling code needs more information it can call GetErrors() to display to the user. Moving on, you allow both conventions: $user->user = 'Joeboo'; $user->SetUser( 'Joeboo' ); You should pick one or the other and stick with it to keep your client code consistent.