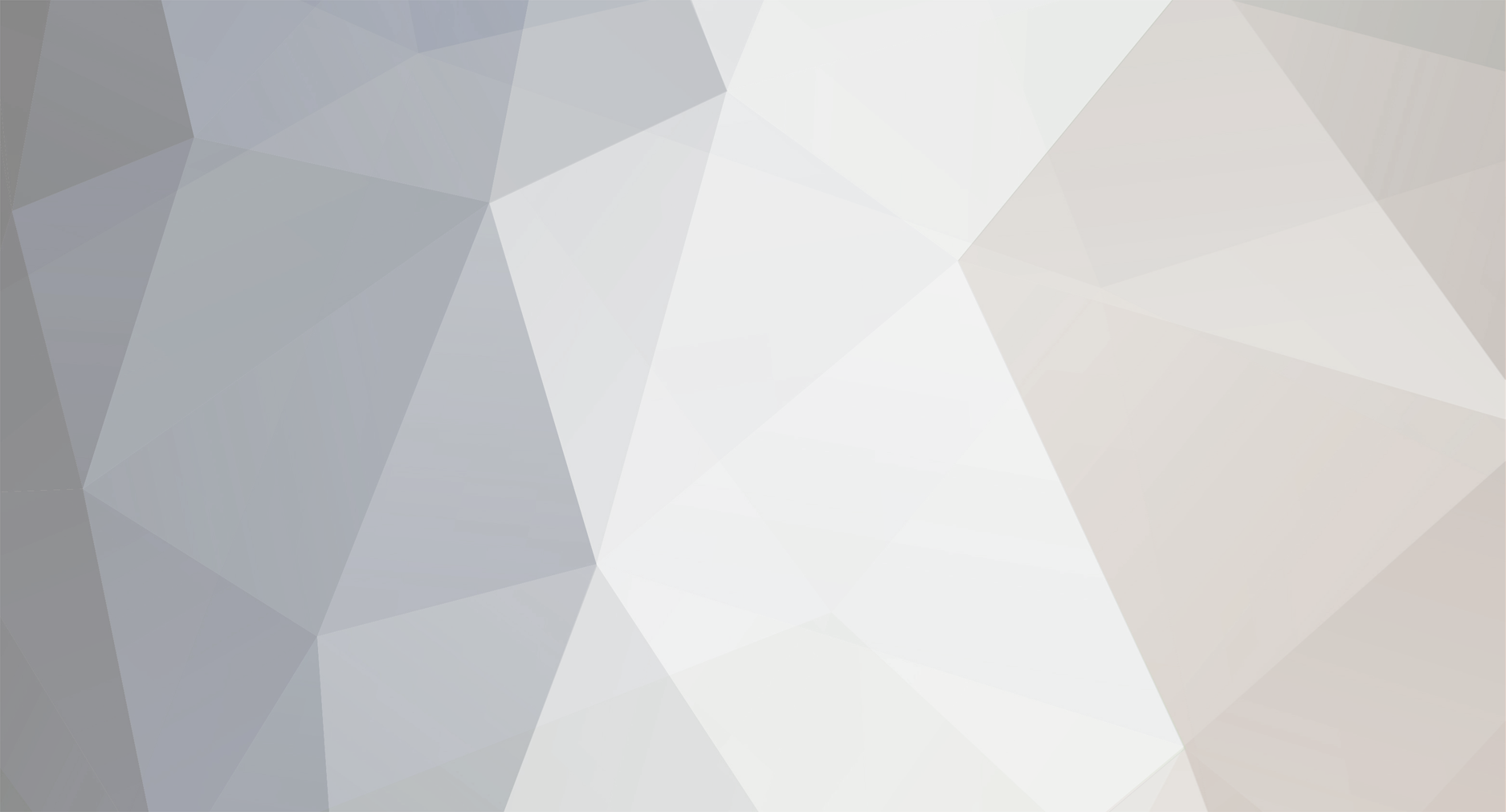
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Unless I'm mistaken you can only have one WHERE in your query. mysql_query("DELETE FROM messages WHERE sendto='$username2' AND sentfrom='$username' AND subject='$subject'") or die(mysql_error()); I hope you called mysql_real_escape_string() on $username2, $username, and $subject. Otherwise you're going to have a heck of a time when I tell your site to delete the message with subject: '; delete from users where 1=1 -- '
-
Dojo is the only one that I'm familiar with and I know that what he's asking for is a breeze with it, which is why I recommended it. And I only recommend a framework because I don't feel like wading through yet another "I-tried-to-make-my-own-XHR-calls-and-it-doesn't-work" thread. These things are a dime a dozen.
-
Here's one that's a tight pass through the string with no external function calls. Could possibly be optimized but I must get back to work! function dateConvert_mdy2ymd( $date ) { $date .= '-'; $ymd = ''; $part = ''; $char_count = 0; $hyphens = 0; for( $i = 0; true; $i++ ) { if( $date[$i] == '' ) { break; } if( $date[$i] == '-' ) { $len = $hyphens < 2 ? 2 : 4; // m, d should be len 2, y should be len 4 while( $char_count < $len ) { $part = '0' . $part; $char_count++; } if( $hyphens === 0 ) { $ymd = $part; }else if( $hyphens === 1 ) { $ymd .= '-' . $part; }else if( $hyphens === 2 ) { $ymd = $part . '-' . $ymd; } $part = ''; $char_count = 0; $hyphens++; continue; } $part .= $date[$i]; $char_count++; } return $ymd; }
-
It appears strtotime() is not able to handle that format, so returns false. That causes date() to return the earliest possible time it is able to handle, which is the 1969 date. There's a handful of ways you can do this: 1) regexp, simple to implement but may be computationally expensive to invoke the regexp engine for a small task 2) explode(), array_shift(), and string concatenation, may be overkill on memory but simple to implement 3) repeated calls to strpos() 4) for loop Here is a solution using strtok(): <?php header( 'Content-Type: text/plain' ); $dates = array(); $dates[] = '12-31-2009'; // dec. 31st $dates[] = '7-4-2008'; // july 4th $dates[] = '1-5-2008'; // jan 5th foreach( $dates as $date ) { echo "{$date} yields: " . dateConvert_mdy2ymd( $date ) . "\n"; } function dateConvert_mdy2ymd( $date ) { $m = sprintf( "%02d", (int)strtok( $date, '-' ) ); $d = sprintf( "%02d", (int)strtok( '-' ) ); $y = sprintf( "%04d", (int)strtok( '-' ) ); return "{$y}-{$m}-{$d}"; } ?>
-
If your problem is urgent, then you should post in the freelancing forum and pay money to have it resolved. Otherwise it's just as urgent as every other thread / problem.
-
IN ( ... ) works great when you have a PHP array of possible values because of the implode() function (in PHP). array_fill() (also a PHP function) comes in handy if you use prepared queries with the ? placeholders.
-
I recommend doing this with Dojo. http://www.dojotoolkit.org/ Documentation: http://api.dojotoolkit.org/ Specific to your problem: http://api.dojotoolkit.org/jsdoc/1.3/dojo.xhrPost http://api.dojotoolkit.org/jsdoc/1.3/dojo.query http://api.dojotoolkit.org/jsdoc/1.3/dojo.formToObject http://api.dojotoolkit.org/jsdoc/1.3/dojo.formToJson
-
Nobody here knows what medium traffic means, so you'll have to be more specific. Nobody here can really tell you which is faster either, since it depends on many factors. The size and types of hard drives and the MySQL configuration can all have big impacts on performance. None of us know what your hardware or software configurations are. Nobody here knows how many pictures you're expecting to deal with? 5 pictures? 10? 4 million? That too makes a big difference. Anyways, typically the answer to this question is the database is better. That's why we invented them.
-
If your version of MySQL has good support for sub-queries, the following may work: SELECT b.* FROM ( SELECT `Race_ID` FROM `Results_tbl` WHERE `Rider_Id`=1 AND `Position`=1 ) AS a INNER JOIN `Results_tbl` AS b ON a.Race_ID=b.Race_ID WHERE b.`Rider_ID` IN ( 1, 2 ) ORDER BY b.`Race_ID`, b.`Position`
-
[SOLVED] passing null varchar value from php form to mysql
roopurt18 replied to nomis's topic in PHP Coding Help
Good! -
[SOLVED] passing null varchar value from php form to mysql
roopurt18 replied to nomis's topic in PHP Coding Help
I'm sorry, the if should be as follows: if ( strlen( trim($middle_name) ) === 0 ) { $middle_name = 'NULL'; // Set middle_name to the string NULL, which MySQL will insert as the value NULL because it is not wrapped in single quotes }else{ $middle_name = "'" . mysqli_real_escape_string( $dbc,$middle_name ) . "'"; } -
[SOLVED] passing null varchar value from php form to mysql
roopurt18 replied to nomis's topic in PHP Coding Help
$query = "INSERT into person (last_name, first_name, middle_name)" . "VALUES ('$person_type','$last_name','$first_name',$middle_name)" ; The query above, without the single quotes around $middle_name, is the proper query to use. As soon as you add the single quotes MySQL will treat the value as a string and will insert whatever is in $middle_name as a string, be it zero length or not. So right above that query, you need to have something like: if( strlen( trim( $middle_name ) ) { $middle_name = 'NULL'; // Set middle_name to the string NULL, which MySQL will insert as the value NULL because it is not wrapped in single quotes }else{ $middle_name = "'" . mysqli_real_escape_string( $middle_name ) . "'"; } $query = "INSERT into person (last_name, first_name, middle_name)" . "VALUES ('$person_type','$last_name','$first_name',$middle_name)" ; Now, by the time you've gotten to the part of your code where you output the form, $middle_name is tainted. It is no longer what the user submitted because you have either: 1) Set it to the string NULL 2) Or wrapped it in single quotes and mysqli_real_escape_string()'ed it So right before outputting the form, reset $middle_name back to what the user submitted. <?php // database stuff above $middle_name = $_POST['middle_name']; // Now echo your form ?> <!-- some html --> <input type="text" name="middle_name" id="middle_name" value="<?php echo $middle_name; ?>" /> <!-- finish the html --> Since $middle_name has been reset to the value in $_POST, the form input will get what the user initially entered and you are probably done. Unless... Your server has Magic Quotes turned on. If that's the case there's one more thing we must do. -
It depends on your target audience and how you're using the JavaScript. If you're using JavaScript to open multiple browser windows and bounce them around all over the screen, then you're using JavaScript to be a nuisance. Historically this is one of the things that has given JavaScript a bad reputation. If you want your site to be as widely accessible as possible and it won't work without JavaScript, then you've used JavaScript improperly. JavaScript should only be used to enhance an already functional site when you want to target the widest possible audience. If you are designing a commercial product or a custom product, for example for a kiosk, then it's fine if you're product requires JavaScript. Often times with such products there exist time or money constraints that do not allow you to make it work and then make it better with JavaScript. In those situations I typically decide to make it cool and make JavaScript a requirement for the product's use. So, like almost everything else in life, the answer to your question is: Yes. No. It depends.
-
[SOLVED] passing null varchar value from php form to mysql
roopurt18 replied to nomis's topic in PHP Coding Help
If you want the input to have an empty value, then the input must look something like this: <input type="text" name="blahblah" id="blahblah" value="" /> It's the value="" that makes it empty. In the part of code right before you echo your form, add this: <?php // bunch of stuff // now right before you echo the form add: echo '$person_type: '; var_dump( $person_type ); echo '<br />'; echo '$first_name: '; var_dump( $first_name ); echo '<br />'; echo '$last_name: '; var_dump( $last_name ); echo '<br />'; echo '$middle_name: '; var_dump( $middle_name ); echo '<br />'; // now echo the form... ?> Now take very careful note of what the values actually are when you expect them to be empty? Are they what you expect them to be? If they aren't, either fix your existing code so they are what you expect or add code to make them empty. In this case, empty should be either the empty string '' or the value null. As an example: <?php if( /* do a test for $middle_name IS empty */ ) { $middle_name = ''; } ?> <input type="text" name="middle_name" id="middle_name" value="<?php echo $middle_name; ?>" /> Also keep in mind the null value is NOT the same as the string containing the characters N, U, L, L. -
It sounds to me like the proxy is at fault then. Perhaps it is adding characters somewhere in the page?
-
I use DOCTYPEs and session_start() all the time without problems. Try changing the first line to: <?php session_start(); >
-
What does view source from the browser show?
-
[SOLVED] passing null varchar value from php form to mysql
roopurt18 replied to nomis's topic in PHP Coding Help
More code would be helpful. You are displaying 1 form. The form will be pre-populated with values when either: 1) It is loaded from the database 2) It is submitted by the user but with errors, thus it must be re-displayed. How you check for empty values to populate the form depends on 1 or 2. It is NOT typically the same for both. Post more code and add comments on where you think it may be going wrong if you want more specific help. -
I didn't read all the back and forth, but: <?php $dates = array( '2009-01-01 23:43:01', '12/31/09 12 AM', '3:45 PM' ); // Assume `column` is either TIMESTAMP or DATETIME foreach( $dates as $d ) { $temp = strtotime( $d ); if( $temp === false ) { echo "can't insert {$d}"; continue; } $temp = "'" . date( 'Y-m-d H:i:s', $temp ) . "'" if( mysql_query( "insert into `yourtable` ( `column` ) values ( {$temp} )" ) ) { echo "inserted {$d}"; }else{ echo "failed on {$d}"; } ?> The trick is to use strtotime on the input if it is in a common enough format to get a timestamp out of it. Then pass it through date( 'Y-m-d H:i:s' ) to put it in YYYY-MM-DD HH:MM:SS format, which is what the database accepts.
-
I've used the professional / commercial version of PDFLib to create PDFs and it works very well. I've also used BIRT to the same effect. I find BIRT to be easier to use. (edit) I actually haven't spent any great length of time exploring alternatives to the above two for generating PDFs from PHP. Since almost all of my projects use BIRT for reporting anyways it's simple for me to just create a BIRT report and run it as PDF output and forward that along to the user.
-
You know there is a INSERT ... ON DUPLICATE KEY UPDATE ... syntax, right?
-
I don't see the lines you're talking about, but your page does render slightly differently in IE7, FF3, and Google Chrome.
-
Getting crazy while making DB structure for Network Marketing
roopurt18 replied to watsmyname's topic in MySQL Help
http://dev.mysql.com/tech-resources/articles/hierarchical-data.html http://www.google.com/search?q=storing+hierarchichal+data -
[SOLVED] passing null varchar value from php form to mysql
roopurt18 replied to nomis's topic in PHP Coding Help
The only object oriented thing in that script is mysql_fetch_object() and the only effect it has is how you access columns in the returned database row. As an example: <?php mysql_query( "insert into `some_table` ( 'first_name', 'last_name' ) values ( 'Larry', 'Smith' )" ); $q = mysql_query( "select `first_name`, `last_name` from `some_table`" ); $row = mysql_fetch( $q ); echo $row[0]; // Larry $q = mysql_query( "select `first_name`, `last_name` from `some_table`" ); $row = mysql_fetch_assoc( $q ); echo $row['first_name']; // Larry $q = mysql_query( "select `first_name`, `last_name` from `some_table`" ); $row = mysql_fetch_object( $q ); echo $row->first_name; // Larry ?> Everything else in my post was demonstrating how you properly convert empty POST values into NULL to insert into the database and how to take NULLs from the database and use them as value-attributes in INPUT-tags.