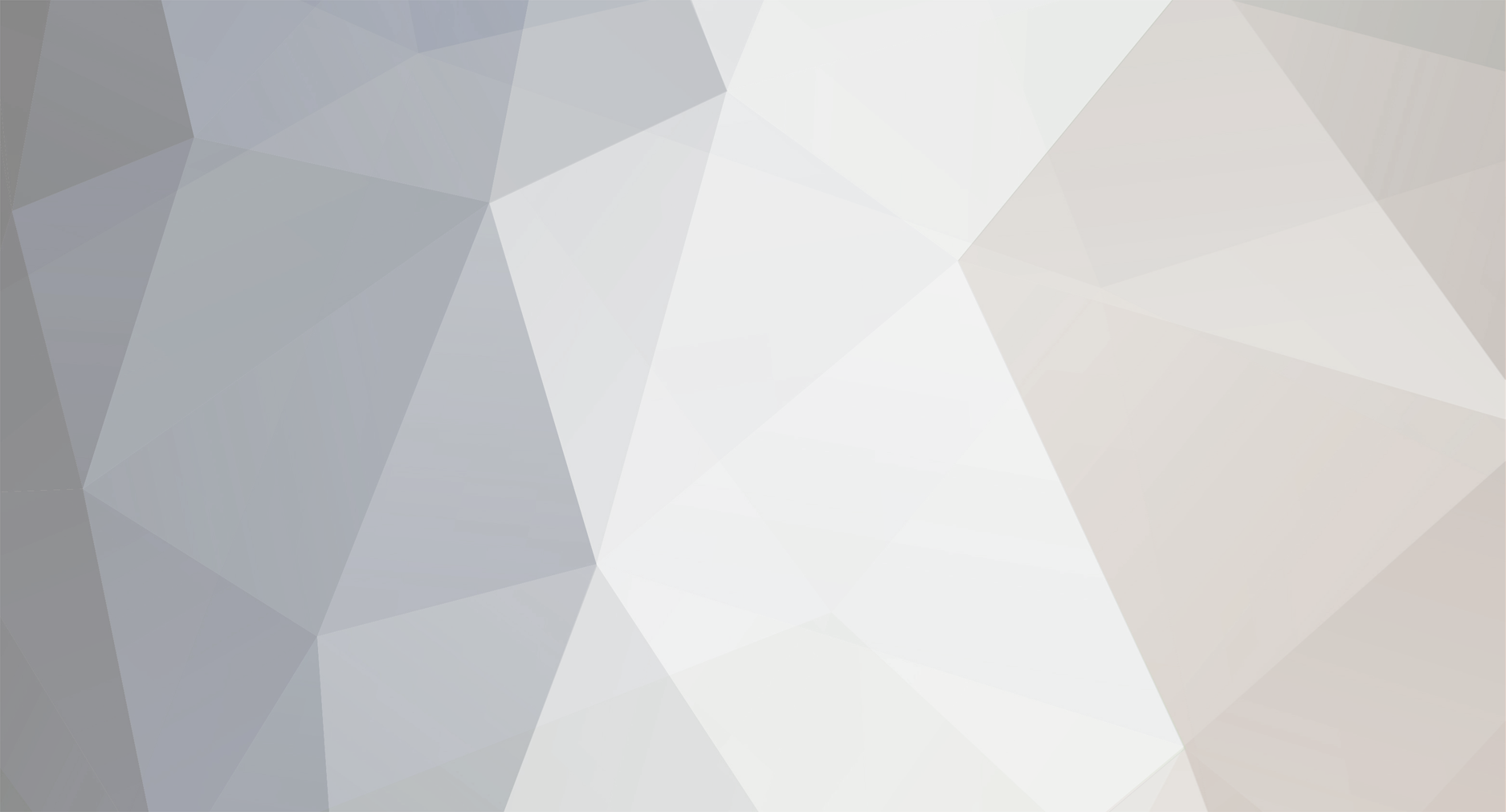
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
They're both readable and they both perform the same function. The difference is that yours has greater volume and also references a function. More code means more keystrokes which means more chances for mistakes. The fact that it's a function also means I will likely have to cross-reference points in the code to figure out just what this get_midnight does. Doing the work in MySQL is shorter and puts everything you need right there in front of you. Hence it's easier to maintain. I made the general statement that a smaller volume of code is easier to maintain given that it is still readable. You can condense a 100 line program into 20 lines of unreadable "super-code" that nobody can decipher. Doing something like that is counter-productive.
-
From the documentation I linked to:
-
[SOLVED] [PHP] I don't understand this logic.....
roopurt18 replied to Eowyne's topic in PHP Coding Help
Try changing OR to || and AND to &&, also bear in mind && has higher precedence than || -
function get_midnight($timestamp) { $day = date("j", $timestamp); $month = date("F", $timestamp); $year = date("Y", $timestamp); $midnight = strtotime("$month $day $year"); return $midnight; } vs. where `column` >= curdate() Good rule of thumb, the less code you have the easier it is to maintain as long as it's still readable, which it clearly is in this case. You also avoid any problems where the apache and mysql servers might not be the same physical machine and could potentially have different times.
-
Perhaps the better question is why do you have to prefix the table names? Maybe you can get around having to do that. However, if you really want to set accomplish what you're after, you will have to parse each query yourself. This is akin to writing your own compiler in that you need to need to create a grammar (which matches MySQL's grammar exactly), create a tokenizer, and create a parser. There is a lot more to take into account than just the table names between FROM and WHERE. You can have joins, sub-queries, and tables that haven't been aliased within the query. The only way to do this accurately is to create a proper parser. It's in your best interest to not have to do this.
-
It's best to leave logic like that within the database; however the OP's code doesn't do it as optimally as it could. It's always worth checking out the available functions in the documentation for this type of thing: http://dev.mysql.com/doc/refman/5.0/en/date-and-time-functions.html#function_curdate select ... where table.date_column >= CURDATE()
-
PHP Form Handling Multiple Dynamic Fields *HELP*
roopurt18 replied to monkeytooth's topic in PHP Coding Help
The best way to do this is to create the HTML for the checkboxes so that they are an array. You can then differentiate the selected IDs by one of two means: 1) Set the array index to the ID 2) Set the value attribute to the ID The goal is to have your HTML checkboxes look like this: <input type="checkbox" name="selected[1]" /> <input type="checkbox" name="selected[2]" /> <input type="checkbox" name="selected[3]" /> This will cause $_POST['selected'] to be an array where each index in the array is the ID of a database item that was checked. After you've modified your output to be like the above, add this at the top of your processing page and see if you can't figure out the rest: <?php echo '<pre style="text-align: left;">' . print_r( $_POST['selected'], true ) . '</pre>'; ?> (edit) rhodesa beat me to it, but since I'd already typed out my response I just let it through. -
@dannyb785 He's calling it checkbox[] to place them into a numerically indexed array named $checkbox. He's distinguishing the IDs in the value attribute of the checkbox. Basically, the method he has should work fine if he can sort things out on the back end.
-
I never noticed you could set the domain when setting a cookie so I usually fixed this with a redirect to a domain starting with www. Is either of these methods better than the other. Initially, I'd have to say the redirect is preferred because the user might browse to http://domain.com and then click on a link pointing to http://www.domain.com. Not a problem for the cookies, but if you have JavaScript in each page that needs to access objects in the other you will have problems there.
-
http://us3.php.net/manual/en/language.types.string.php#language.types.string.syntax.heredoc You have PHP opening and closing tags in the middle of your heredoc.
-
Procedural versus OOP - Talk Me Into It!
roopurt18 replied to cngodles's topic in Application Design
Pretend you design and sell models (aka figurines or something similar) for a living. Procedural is like creating each and every model from scratch, even if it's the same figure. OOP is like creating a single model (or bits of models) and casting them so you can re-create them any time you want to. OOP is a tool, just like any other. It has proper (and improper) uses. Use it correctly and it will save you oodles of time and frustration. Use it incorrectly and you wonder why you even bothered. The trick is to just write OOP code. Write a lot of it. After each project you code in OOP, go back and revisit the principles of objects, such as polymorphism and inheritance. In time it starts to make sense. -
I'm locking this thread as this is ridiculous. If you want help with something you've coded, then PM me and I will unlock it so that you may post your code and ask for help in fixing it. If you want someone to code this for you, we have a freelance section.
-
The code you have looks like it's most of the way there. What exactly isn't working? What have you done to try and troubleshoot it? Do you understand the concepts behind the code the other person helped you with? Because once you understand the concept the steps for troubleshooting are always the same: 1) Know what data to expect 2) Look at what you have Are 1 and 2 the same? Yes - Your code works (assuming you got #1 correct). No - What did you get that you didn't expect and why?
-
I don't think you can break up a heredoc like you've done.
-
As far as I can tell you're using $forum_look without it being defined and you're expecting it to be a $_GET parameter. Place this at the top of your script and see if it doesn't contain the information you want to use in your query: echo '<pre style="text-align: left;">' . print_r( $_GET, true ) . '</pre>'; Once you get it working, come back and ask us how to stop SQL injection.
-
Glad it's working. I would have given more detailed responses as of late but work has been keeping me very busy.
-
It's the other way around. The function returns a value that you assign to the variable. Read the documentation on functions and look for an example.
-
You can't access local variables globally. That's the whole point of making them local. Instead, change your function to return $errors and call it like so: $errors = connect_to_mysql();
-
The best permission systems use a groups and users model, where groups is sometimes replaced by roles. You can create a group or user and assign specific privileges to them. For you instance, you can create a Department Head group that has the ability to make purchases for the department, while regular members of the Department group can only view purchases. You can create individual users and assign the same permissions, but you can also assign users to groups so that you can manage everyone at the same time easily. You have to take a look at the kind of operations you want to control access on within your system. For each operation, give it a short but unique text association. First you have the basic CRUD operations: Create - c Read - r Update - u Delete - d But maybe you also want to support operations such as: Exporting - xp Executing - x Importing - i Then take a look at the areas within your application that you wish to expose and control these actions. Let's say for instance we had a news section. News can be created, read, updated, deleted, but let's say it can also be imported and exported. I like to save my permissions as if they were a file system all of their own. So each of those actions for the news can be expressed as: /news/c /news/r /news/u /news/d /news/xp /news/i Then I can save this in a table like this: permissions id, entity_id, path, access id is an auto incrementing PK. entity_id associates to a group or user in another table that supports hierarchies. path is the text strings I listed above (/news/c, /news/r, etc) access is 0 if user can not do this, 1 if the user can, and null if we inherit from the parent entity of the current entity When someone logs into your system, you pull out all of their permissions from the database and you can easily display and hide links based on what they can do. You also have to build each page so that they can't bypass your lack of links by just typing in URLs manually. You can easily extend this path-like approach to other areas of your system. Let's say you've designed a database view, call it recent_purchases, that gives a listing report of purchased items with columns: product, qty, price. Power users should be able to view all of the columns, but others might only need to see the product and qty and we don't want them to see the price. You can create a new path in your permissions: /system/db/views/recent_purchases/columns/product/r /system/db/views/recent_purchases/columns/qty/r /system/db/views/recent_purchases/columns/price/r Now when you either call the view or display the results, based on what columns the user has access to, you can display or not display them. Depending on how you organize your paths there is no limit to what operations you can control.
-
AFAIK yes you can. But there is absolutely no reason to do so for what you're asking. In your example of mysql_fetch_assoc(), that is a function that you are passing a result set to. That function extracts a record from the result set. You have to use a special function (mysql_fetch_assoc) in this case because the argument ($result) is a resource. A resource is a built-in PHP data type, but PHP itself has no idea what the resource represents. Perhaps the resource represents a MySQL result set, or perhaps the resource represents an image from the GD library. In either case, PHP doesn't know what the resource represents. In those cases, you have to use a special function to get at the results (in your example, mysql_fetch_assoc()). An array on the other hand is a built in data type and PHP knows everything about how it's stored. PHP may not know what's in the array, but it does know enough about arrays to cycle through them. In this case (when using arrays), PHP has several built in methods of cycling through the elements. The most convenient of which is the foreach. I hope that explanation helps some.
-
JavaScript has nothing to do with this. Also, JavaScript is not a substitute for server-side validation. The error results because you are echo'ing $error1 before assigning anything to it. By default variables in PHP hold null and if you use the value of an undeclared variable you get null, with the option to have PHP complain about the error or not. Try adding this at the top of your script: $error1 = '';
-
So you built an application and failed to: 1) Use standard practices that worked in all browsers 2) Test it in different environment Thus the conclusion that you draw is that IE is garbage? I use FF over IE myself but only because I perform web development, otherwise I'd be perfectly happy using IE for basic web browsing. You're free to distribute your application as you see fit, but intentionally limiting your market is rarely a successful strategy. Web applications require Javascript to be enabled.
-
my .htaccess file causing problems.. please help!
roopurt18 replied to kee2ka4's topic in Apache HTTP Server
AFAIK 'nocase' and 'last' are incorrect. I've only ever seen them entered as NC and L, respectively. I can't say more than that because I'm at work. -
Multiple tables, one-to-many relationship, multiple matches using AND
roopurt18 replied to zq29's topic in MySQL Help
Add in a good night of sleep and I see why my solution doesn't work! -
Multiple tables, one-to-many relationship, multiple matches using AND
roopurt18 replied to zq29's topic in MySQL Help
Hmmmm. Does this help? SELECT r.`name` FROM `registration_country` rc INNER JOIN `registration` r ON rc.`registration`=r.`id` INNER JOIN `country` c ON rc.`country`=c.`id` WHERE c.`name` LIKE '%united%' OR c.`name` LIKE '%british%' ORDER BY r.`name` GROUP BY r.`id` They're almost identical but with a GROUP BY thrown in to remove duplicate records from showing up. I guess a distinct could handle that as well. Also, I'm not keen on the: c.`name` LIKE '' AND / OR c.`name` LIKE '' AND / OR ... If there's a way to do LIKE matching but using an IN ( 'val1', 'val2', ... ) I think it'd be much cleaner; but I don't know if that functionality is possible.