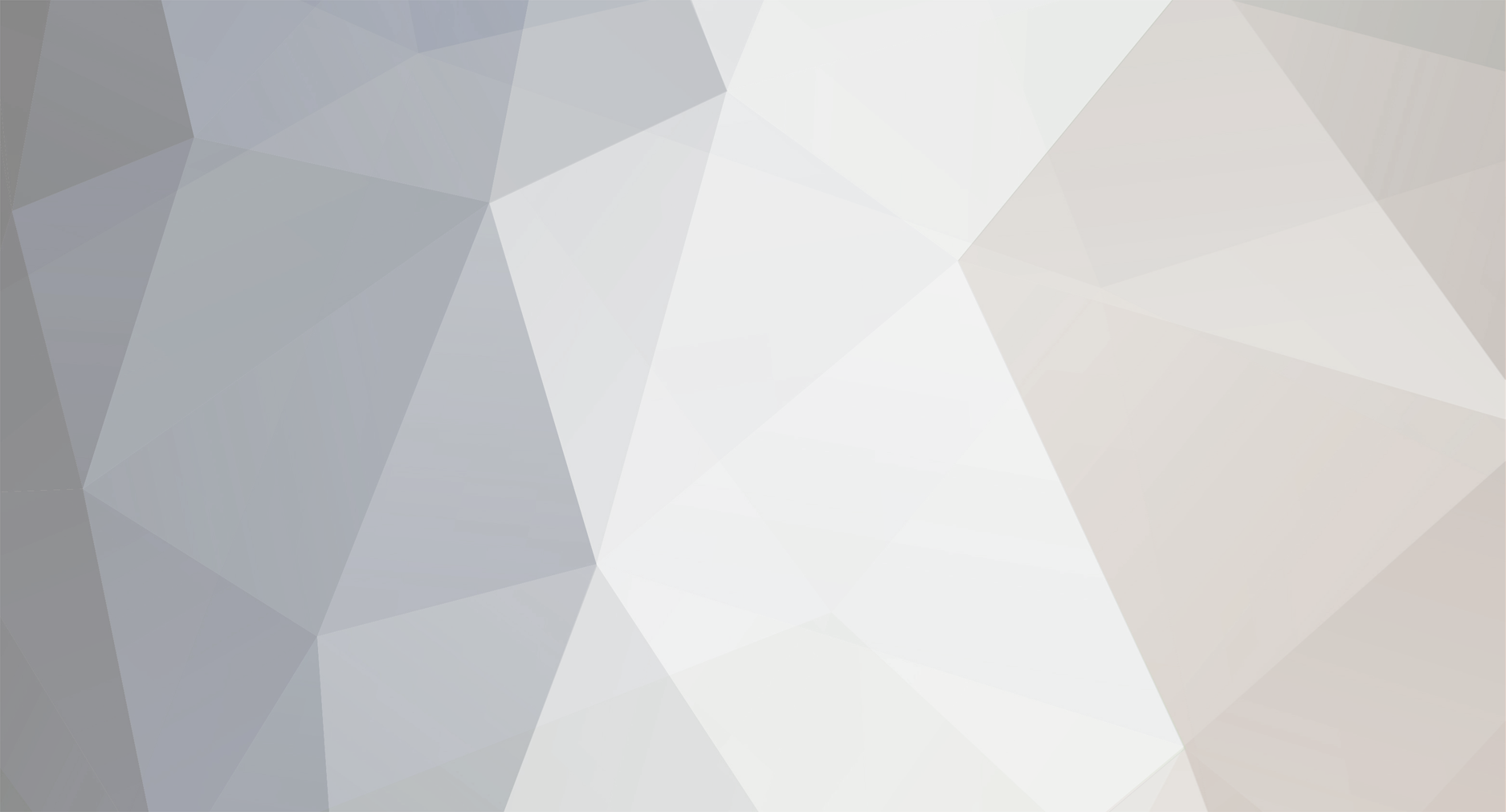
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
After reading all of the responses and then re-reading your original question, I think we've all missed the mark in answering the original question. IMO, the answer to the OP is there is no difference. Zip, nada, nothing. They're essentially the same. Regardless of doing it procedurally or with OOP, the code has to do the following things: Parse the URL into a controlling script (index, users, clients, etc.) and possibly a sub-function within that script (add, delete, edit, etc.) Parse any parameters in the URL (if using mod_rewrite, otherwise the params are in $_GET) include the controlling script (ensure that the file exists) and invoke the sub-function (ensure the sub-function exists) within it dump the output The algorithm doesn't change whichever you choose. I'll even go as far to say that neither method is easier or more difficult to maintain than the other, regardless of the size of the site. Now OOP does excel over procedural in many ways. Cosmetically OOP usually turns out to be less typing in the client code. Since all function names need to be unique, in procedural programming you end up with really long functions: <?php $board = forum_create_board(); forum_set_board_title( $board, 'The New Board' ); forum_set_board_description( $board, 'This is our newest board!' ); forum_save_board( $board ); ?> vs. the OOP client code: <?php $board = new Forum_Board(); $board['title'] = 'The New Board'; $board['description'] = 'This is our newest board!'; $board->save(); ?> That's so much more pleasant to type. OOP also gives the creator of the server code more control over how it will be used. Most compiled procedural languages group data into structs (short for structures), which are most similar to associative arrays in PHP. Most languages that use structs do not provide any sort of visibility into the members of a struct; therefore all members are what we'd call public. For example, if we had an employee struct with members: id, name, age, then client code could do this: /* This is mock C code */ struct employee = employee_find_by_id( 1 ); /* find employee in DB */ employee.id = 2; /* the id is managed by the DB and should therefore be read-only, but we can't enforce this in C */ employee_save( employee ); However, with OOP the programmer of the employee class can control how the id property is accessed and thus control if it is read-only or writable. This type of control helps make client code less prone to errors and hacks (quick fixes to get something to work). Where OOP really takes off is in concepts like inheritance, polymorphism, interfaces, etc. You can accomplish all of these things in procedural programming, but only through writing the code that makes these behaviors possible, which is tedious for the programmer. These concepts are built directly into an OOP language, so you don't have to write any extra code to make inheritance or polymorphism work, they just do.
-
[SOLVED] How can I identify duplicated images?
roopurt18 replied to marcosr's topic in PHP Coding Help
@CV, I think the reason most people don't bother with it is because of how cheap storage is. Use a lot of programming time to come up with sophisticated methods of detecting duplicate media in addition to the added CPU strain VS. spending a couple hundred on a TB of storage. -
I disabled Javascript in my browser and it works fine. In FF and using the Web Developer toolbar, I chose: View Source -> View Generated Source and your form looks like this: <form name="sel" method="post"></form> Which means that none of your inputs are actually in the form. I'm guessing that the browser is sending up all of the inputs that actually use an input tag in this case and ignoring the select tags. Basically, either your markup is screwed up or your JavaScript is screwing it up.
-
[SOLVED] How can I identify duplicated images?
roopurt18 replied to marcosr's topic in PHP Coding Help
But that's not usually the case IMO. The more common case would be a piece of media originating at a site, traveling from person to person, and then being re-uploaded by someone else further down the chain. And this would really be the first line of sense for detecting duplicate media. -
[SOLVED] How can I identify duplicated images?
roopurt18 replied to marcosr's topic in PHP Coding Help
I've often wondered why more of the social media sites don't do this. It's so easy to detect duplicate files (at least IMO). The only method to determine if two files A and B are duplicates is to determine if they have the same exact sequence of bits. However, you don't want to go about trying to compare a newly uploaded file with every other existing file on your site for obvious reasons. What you can do is create a key from each file that is uploaded, and an MD5 hash seems appropriate enough, and store that key in a DB along with the rest of the file's information. Keep in mind that MD5 can have collisions, so it is possible for two files to generate the same hash, but that's not really a problem as the likelihood is low. So every time a new file is uploaded, hash it and look for entries in the DB that have the same hash. For each entry in the DB, compare the newly uploaded file with the existing files on disk to determine if they are the same file or not. Keep in mind the one place where this can fall apart is if what would be the same file is uploaded in different formats. For example, the same image uploaded as a gif and a jpg. Or a jpg is uploaded in different resolutions. Or a movie file in different formats. In these cases it is difficult to automatically detect duplicate submissions and as such, you should build a user-oriented feature into the site for users to report duplicates (along with the original item). -
[SOLVED] How do I call multiple, specific rows from from my database?
roopurt18 replied to b's topic in PHP Coding Help
What's this output? <?php //connection $con = mysql_connect("localhost","root","..."); if( !$con ) { die('Could not connect: ' . mysql_error()); } mysql_select_db("...", $con); //query $query = "SELECT * FROM retailers WHERE id = 7000"; $result = mysql_query($query); while ($list = mysql_fetch_assoc($result)) { echo '<pre style="text-align: left;">' . print_r($list, true) . '</pre>'; } // end while ?> If it outputs personal data, replace it with fake data. If there's a lot of it, just give the first couple of rows. -
Well if you look at the CPU % column, MySQL is using 45% of the CPU in that snapshot, which indicates you probably have some poorly written queries bogging down your server.
-
As far as I can tell it should work, so when all else fails, comment out the broken code and echo a single value. <?php include("DBManager.php"); include("DBInfo.php"); class MysqlManager extends DBManager { function MysqlManager() { $startTime = $this->getMicroTime(); echo 'DBInfo::$host: ' . DBInfor::$host . '<br />'; /* if (!$this->connection = @mysql_connect(DBInfo::$host,DBInfo::$user,DBInfo::$pass,true)){ $this->errorCode = mysql_errno(); } */
-
Your form certainly looks correct. I suspect that: $row['state'] $row['city'] are empty and your echo's at the top are working but printing empty strings. Try using this to see what data is set in $_POST: echo '<pre style="text-align: left;">' . print_r( $_POST, true ) . '</pre>'; If $_POST has indexes named stateinput and cityinput then it's the $row[] data that is empty. If $_POST doesn't have those indexes set then something else is wrong.
-
Whenever you refer to associative array indexes, enclose the index in quotes. Use $array['index'], do not use $array[index]. Whenever you include a variable within a double quoted string, enclose it in curly brackets. Use echo "Hello, {$name}!", do not use echo "Hello, $name!" Putting it together: echo "Congratulations, {$players['mvp']}!"
-
[SOLVED] Another way to break an if statement?
roopurt18 replied to Kane250's topic in PHP Coding Help
1) As stated, break has no bearing on an if-statement. 2) Even if it did, your break comes right before a closing curly bracket, which ends that block of an if-statement anyways. 3) Then something else is wrong and you have a serious misunderstanding of how logic works. <?php // Your code, simplified if ( A ) { if ( B ) { if ( C ) { } elseif ( D ) { print "That e-mail address has already been added!<br />"; break; } else { } } else { // Call this NOT-B, or !B print "Please enter an e-mail address<br />"; } } ?> You're saying that if you remove the break the Please enter an e-mail address line prints? How is that possible. break can only execute if B is true. The Please enter an e-mail address can only print if B is NOT true. So how can the break have any bearing on that? B can't be true and false at the same time. 4) In your original code, it appears you are missing a closing curly bracket, which Prismatic added when he cleaned it up. -
This might be more than you can chew at this point, but check out the sample chapter from O'Reilly's Head First Design Patterns. http://www.oreilly.com/catalog/hfdesignpat/chapter/ch03.pdf Try and do that with procedural code (it can be done, just not as easily).
-
This should give you an idea: <?php // Untested... // Array of players $players = array( 'Steve', 'John', 'Al', 'Mike', 'Jill' ); // We can use a single INSERT statement to insert multiple rows, with the // format: // INSERT INTO `table_name` ( `col1`, `col2`, `col3` ) VALUES // ( row1_val1, row1_val2, row1_val3 ), // ( row2_val1, row2_val2, row2_val3 ), // ... // The next code segment builds every combination of the VALUES-part for our // array of players $value_parts = array(); $num_players = count( $players ); for( $i = 0; $i < $num_players - 1; $i++ ) { for( $j = $i + 1; $j < $num_players; $j++ ) { // $i: player1 // $j: player2 $value_parts[] = sprintf( "( '%s', '%s', '%s', 'no', NOW() )", $cupname , $players[$i] , $players[$j] ); } } // Now we create our sql statement $sql = sprintf( " INSERT INTO `gamessch` ( `cup`, `player1`, `player2`, `played`, `date` ) VALUES %s", implode( ', ', $value_parts ) ); echo $sql; ?>
-
This code snippet should answer your number two. <?php $idx = 0; $i = 0; while( $row = get_row( query() ) ) { echo $row . '<br /><br />'; // Comment out this if block to see what would happen in the real world if( $i++ == 10 ) { break; } } function get_row( $data ) { global $idx; if( $idx > 2 ) { return false; } echo 'get_row()<br />'; return $data[$idx++]; } function query() { global $idx; echo 'query()<br />'; $idx = 0; return array( 'a', 'b', 'c' ); } ?>
-
Put double quotes around 5 so that it's a character and not a number.
-
Use regular expressions: http://www.w3schools.com/JS/js_obj_regexp.asp The regexp you would use would be something like: /5[a-zA-Z]{5}/ Your while construct would be something like: var pass = ""; do{ // not sure if i'm using prompt() correctly, look it up online (maybe confirm?) pass = prompt( "Enter a password" ); }while( /* pass fails regexp */ ); // pass is now valid, do whatever
-
You've already answered your own question then. That's about as good as you can do: 1) Create a key when they load the page 2) Check the key when they submit the score and erase the key so they only get a single submittal. This could break games depending on how the scores are submitted though. If the flash game submits the score as soon as the game is over, then the key will be eaten by the game and the user would have to reload the page to play again. This would break any 'Replay' buttons built into the game. If the score is submitted by the user, then this is less of a problem.
-
nloding; Let's say you are working with a language like C++ that has arrays, but they can not be resized easily. For example in C++ when you create an array you need to know how many items will be in it. If you create it with storage for 10 items but later need room for 20, you have to allocate more memory and do some data shuffling in memory. Now I won't go into the different ways of doing this, all I'll say is we want to design our dynamic array class so that I can use code like this: MyArray arr = new MyArray( 10 ); // grow the array in chunks of 10 for( int i = 0; i < 10; i++ ) { arr[i] = lookUpValue( i ); } // Later in the code we have cause to use indexes 10 through 19. // Our array class is smart enough to resize itself automatically, so our // client code (i.e. the code below) just uses the indexes as if // they were there. for( int i = 10; i < 20; i++ ) { arr[i] = lookUpValue( i ); } Now let's say we'd our class MyArray to be able to sort its data. We'd write a function the client can call like so: // Assuming this is a continuation of the code above... arr->sort(); Let's suppose that MyArray was written as a template, meaning it can hold any type of data: ints, floats, doubles, strings, Employees, Vehicles, LotteryNumbers, etc. How can MyArray have a sort() method? Given two pieces of data A and B, in order to sort them you have to be able to determine if: A < B, A == B, or A > B. So the sort() method has to know something about the data it holds, which is bad. (Side note, I haven't programmed C++ in a long time so maybe C# or another language would be better for what follows.) So how can we design a generic array class that knows just enough about the data it contains in order to sort it? By using an interface. We create an interface ISortable and every object that implements this interface must support the basic equality operators. We place a restriction on our array class that sort() can only be called on data elements that implement the ISortable interface.
-
Example 1 has it. http://us3.php.net/manual/en/language.oop5.decon.php
-
I hate to say this, but I can't think of a single way to make this secure without the source to the flash game.
-
seperate the float and integer value of a result.
roopurt18 replied to art15's topic in PHP Coding Help
lol Neal. Too bad this wasn't C; I could have written a cute little solution that just doesn't translate to PHP very well. -
Fix the contents of $footer?
-
seperate the float and integer value of a result.
roopurt18 replied to art15's topic in PHP Coding Help
Without caring about performance: list( $front, $back ) = explode( '.', $decimalValue ); $front = intval( $front ); $back = intval( $back ); -
It would be better to just store the images on file system, named after their unique auto-incrementing `id` column in the database. Then all you need to store in the DB is the file extension so that your PHP code append it when outputting images. images id, ext 1, jpg 2, jpg 3, jpeg 4, gif 5, png On your file system you'd have: 1.jpg, 2.jpg, 3.jpeg, 4.gif, 5.png in a predetermined directory. When you select the images: SELECT CONCAT(`id`, '.', `ext`) AS `name` FROM `images` WHERE ... Then it's as simple as construction an img tag: echo sprintf('<img src="/the/path/%s" alt="An Image" />', $row['name']); Granted there are some pieces missing here, like the fact that images are attached to specific questions and have a placement within that question. But you can fill those in. As for the why, consider this. If the images are stored in the DB you have to create a special script that takes the image id, grabs the image from the DB, sets up the header, and outputs the image contents. So each of your images refers to a PHP script, which requires extra processing on the server just to open the file and feed it into the PHP interpreter. In addition, this script has to create a connection to the database, thus eating up your database connections. So if your single script that displays a question has 5 images embedded in it, you get 5 more connections to your database. Then you have to query the database for the image. If the image exists, the DB will return the contents over the MySQL connection; even if the smallest image is only 50kb, that's still 50 thousand characters, which is a substantial amount of data. So you perform all of this when the original script could just pull the file locations out of the DB and have apache handle the delivery of the files for you.