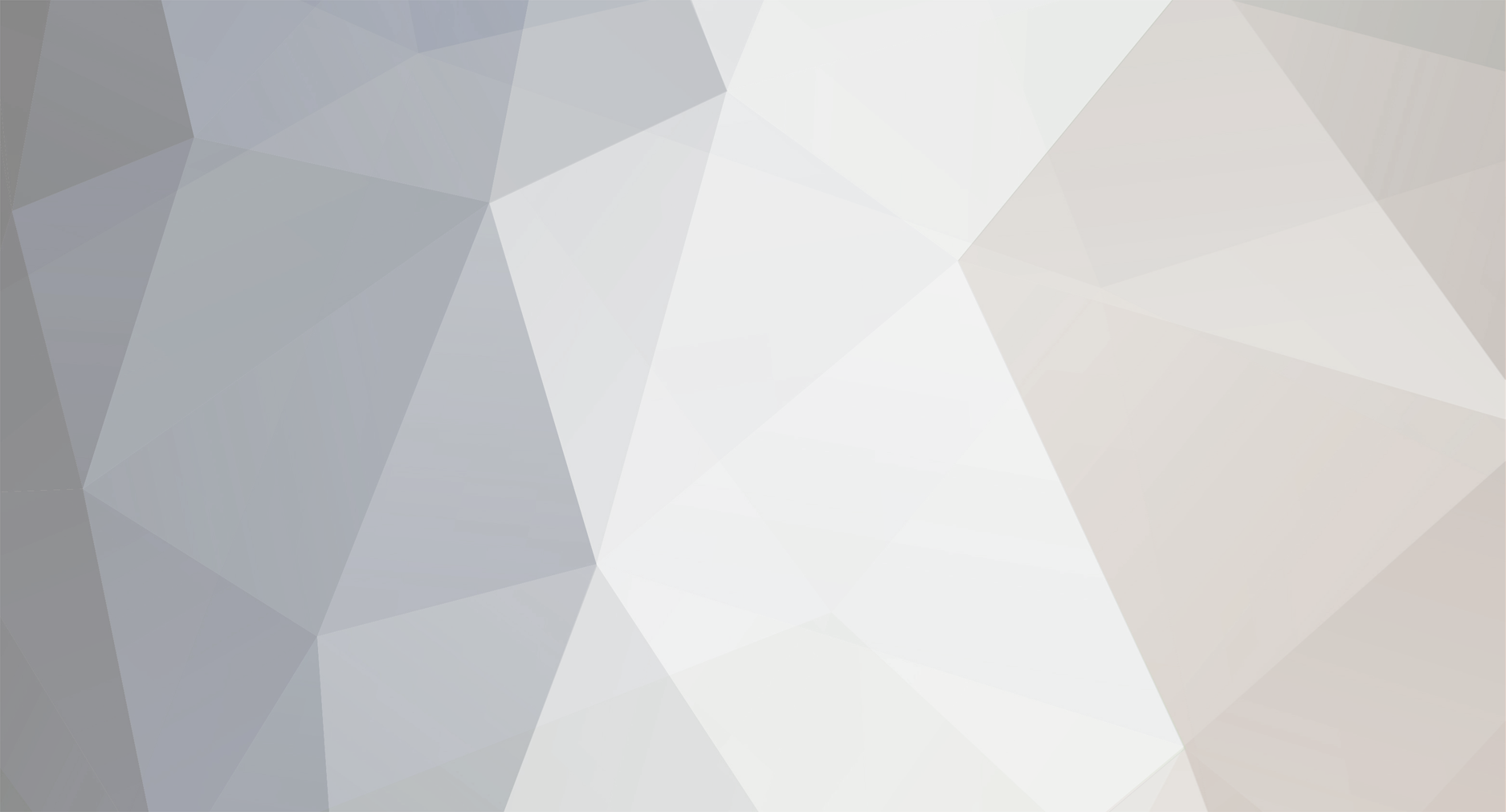
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
$count = count($array) $i =0; $match =0; while($i<$count || $match ===0){ if($item == $array[$i]); $match =1; $i++; } I highly doubt that's the code that in_array() invokes. I'd wager that internally arrays are stored as hashes or binary search trees, or a combination of both. In any case, using an array does add more overhead, I won't argue that. The array is created long enough to be passed to a function but not stored in any permanent variable, thus it is thrown away after passed to the function. This is no different than the PHP interpretor allocating space in a symbol table for each of the strings "ASC" and "DESC".
-
Eh, I'd say until you can trace it down as causing a huge impact on performance it's fine. It allows for any case-combination of 'desc' or 'asc', which if they're being inserted into a query is perfectly legal. I suppose you could get sort of ugly and do this: <?php if(isset($_GET['dir']) && ($tmp = strtolower($_GET['dir'])) == "desc" || $tmp == "asc"){ $dir = $_GET['dir']; } ?>
-
$dir = isset($_GET['dir']) && in_array( strtolower( $_GET['dir'] ), Array( 'desc', 'asc' ) ) ? $_GET['dir'] : "some_default_value"; They each accomplish the same thing, so they're each just as effective. One is slightly easier to read while the other uses less lines of code. It's up to you to determine which to use.
-
[SOLVED] How to extract from one table into another
roopurt18 replied to bri4n's topic in PHP Coding Help
I want to be sure you understand why the query was failing. vote CREATE TABLE `vote` ( `choice` int(11) NOT NULL default '1', `charity` varchar(50) NOT NULL default '', `votes` int(11) NOT NULL default '0', PRIMARY KEY (`choice`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1 The insert statement is only updating the `charity` field, which means each of the entries was getting a `choice` value of 1, since that's the default you set. However, `choice` is also set as a PRIMARY KEY; there can only be one PRIMARY KEY defined per table and it must be unique across all of the rows. In other words, once a value is assigned to the PRIMARY KEY of one row that same value can not be used in the PRIMARY KEY field of another row. That is the reason you got the duplicate index. Now, you are likely to encounter a problem with your script that you weren't expecting. From the structure of your CREATE TABLE for `vote`, it appears you want to update each charity with the number of users that have it in their profile. Basically, let's say there are 3 users that chose charity ABC and 2 users that chose charity XYZ. Which of the following sets of data should appear in `vote`: `vote` dataset 1 +---------+-------+ | charity | votes | +---------+-------+ | ABC | 1 | | ABC | 1 | | XYZ | 1 | | ABC | 1 | | XYZ | 1 | +---------+-------+ `vote` dataset 2 +---------+-------+ | charity | votes | +---------+-------+ | ABC | 3 | | XYZ | 2 | +---------+-------+ Likely you are wanting to query these later and count the number of users supporting a particular charity. You can do this with either table structure, although structure 2 is more compact. Also, with your current DB structure, it will be difficult for your users to support more than one charity. Are you sure they will only ever support a single charity? Or in the future do you think you will need to allow them to support multiple charities? -
Noob at PHP- making a "higher/lower" card game.
roopurt18 replied to frenz_hilpur's topic in PHP Coding Help
Homer -
[SOLVED] How to extract from one table into another
roopurt18 replied to bri4n's topic in PHP Coding Help
The error indicates that the destination table has a unique constraint on one or more columns. In phpMyAdmin, run the following queries and paste the output: SHOW CREATE TABLE vote; SHOW CREATE TABLE userinfo; -
[SOLVED] How to extract from one table into another
roopurt18 replied to bri4n's topic in PHP Coding Help
Bah, I have brain damage. I forgot to call mysql_query(). Change: $sql = "INSERT INTO vote (charity) SELECT u.charity FROM userinfo u WHERE 1" or die("Error: " . mysql_error()); To: $sql = "INSERT INTO vote (charity) SELECT u.charity FROM userinfo u WHERE 1"; mysql_query($sql) or die("Error: " . mysql_error()); -
I was going to say why not just change the input type from button to submit.
-
[SOLVED] How to extract from one table into another
roopurt18 replied to bri4n's topic in PHP Coding Help
Oh, oops. I made a mistake. There is an error in my MySQL query; I misspelled WHERE. I suspect that it's not inserting any rows and that the row you think its inserting is already present in the table. <?php include("include/assorted.inc.php"); /*Connect to database*/ $connection=mysql_connect($host,$user,$password) or die("Error: Could not connect to the server"); $db=mysql_select_db($database,$connection) or die("Error: Could not connect to the database"); $sql = "INSERT INTO vote (charity) SELECT u.charity FROM userinfo u WHERE 1" or die("Error: " . mysql_error()); ?> -
[SOLVED] How to extract from one table into another
roopurt18 replied to bri4n's topic in PHP Coding Help
There query that I gave you inserts into the table and uses the values to insert as those pulled from the SELECT part. In other words, the query performs the SELECT and insert and eliminates your need to SELECT and then loop in PHP code. Here is a script that should work: <?php include("include/assorted.inc.php"); /*Connect to database*/ $connection=mysql_connect($host,$user,$password) or die("Error: Could not connect to the server"); $db=mysql_select_db($database,$connection) or die("Error: Could not connect to the database"); $sql = "INSERT INTO vote (charity) SELECT u.charity FROM userinfo u WHER 1" or die("Error: " . mysql_error()); ?> I'll follow up in a moment with some reasons why your other one was wrong. ******************** ******************** Here is the follow up with what was wrong with your previous attempt. <?php include("include/assorted.inc.php"); /*Connect to database*/ $connection=mysql_connect($host,$user,$password) or die("Could not connect to the server"); $db=mysql_select_db($database,$connection) or die("Could not connect to the database"); // Everything before this line is OK //Retrieve information from database and store result in variable $query = ("SELECT charity FROM userinfo") or die(mysql_error()); $result = mysql_query($query); // The above two lines are PROBLEM #1. You have the right idea, but you need // to remove the parens from around the SELECT string, they're not necessary. // Next, move the or die() part down to the second line. Here is how they // should look: /* $query = "SELECT charity FROM userinfo"; $result = mysql_query($query) or die(mysql_error()) */ while ($row = mysql_fetch_array($result)){ $query = "INSERT INTO vote (charity) SELECT charity FROM userinfo WHERE 1" or die ("Could not execute query"); } // As I explained in my previous post, there is no need to loop anymore. // The INSERT ... SELECT ... statement performs both operations at once. It // select data from one table and immediately inserts it into the other, // eliminating the need for you to SELECT and then loop in your PHP code. // Also, you forgot to call mysql_query() on the query so it wouldn't have // ran anyways. echo "Vote section successfully updated!"; ?> -
Automatically searching an array inside an array?
roopurt18 replied to Gibbs's topic in PHP Coding Help
This is as good as I can come up with based on what you've posted so far. It looks to me like you have a list of associations like: ASSOC=>VALUE LLL => E TEX => A Using those you want to search each $arrayData['sect'][ASSOC][0][name] to see if it's equal to VALUE. In the following code I set up those associations in $arrSearch. Then I just use a couple of foreach loops to find what you're looking for. I store the results in $arrFound and just dump its contents as the last line. <?php /** * Set up an association of which array after ['sect'] we want to search and * what value in that array we want to search for. */ $arrSearch = Array( 'TEX' => 'A', 'LLL' => 'E' ); $arrFound = Array(); // values that we found, empty for now // Now loop over each section that we want to search foreach($arrSearch as $sect => $search){ $tmp = $arrayData['sect'][$sect][0]; // just a shorthand, makes it easier // to use foreach($tmp as $name => $value){ if($value == $search){ // we found it $arrFound[] = Array( 'name' => $name, 'value' => $value ); break; } } } echo '<pre style="text-align: left;">' . print_r($arrFound, true) . '</pre>'; ?> -
[SOLVED] How to extract from one table into another
roopurt18 replied to bri4n's topic in PHP Coding Help
Unless there is a difference in how MySQL handles each of them, they both seem about the same amount of typing and just as readable to me. -
[SOLVED] How to extract from one table into another
roopurt18 replied to bri4n's topic in PHP Coding Help
INSERT INTO vote (charity) SELECT charity FROM userinfo WHERE 1 Try that query instead. Also, in case you keep down the same path. Your while loop is only assigning to a variable and not actually executing a query. As for your error message, whenever you use a variable in a double-quoted string its a good idea to enclose it in curly brackets. Try changing the line to this: $query = mysql_query("INSERT INTO vote(charity) VALUES {$row['charity']}") or die ("Could not execute query"); -
Returning the next integer from an auto_increment field
roopurt18 replied to zq29's topic in MySQL Help
If you know the id of the image you're currently displaying is some value, and you want the next image with a larger id: [code] SELECT * FROM table WHERE id > {$id} AND cat='PHOTO' ORDER BY id ASC LIMIT 1 [/code] [quote]But do you have to be a jerk? You're a moderator, right? Isn't part of your job to help?[/quote] AFAIK, none of the moderators are paid to run this site, so it's none of their jobs to do anything. Also, when fenway said "look it up first," I think he may have meant look up the next record that is of the same category and create a link for it. Not everyone is out to get you, ya know? -
Think very carefully about what your original code was doing. You were querying the database. Then you looped over the results and assigned each to a variable. But then you didn't do anything with the variable! So it doesn't matter if the query pulled 1 row, 10 rows, or 5 million. You were constantly overwriting the variables without using them and this would just continue until the last one; which is why it was only printing the last record in your DB. teng84 correctly moved the table generation before the loop and then correctly creates each row inside the loop, with one problem. He has embedded the variables directly in the string, which is OK if the string begins and ends with double quotes; his uses single quotes however so it won't work. Also, I'm fairly your if / else if / else if / etc is meant to be a part of the loop. I'm guessing that one of your fields is an int that correlates to a string. You should place your if block in the loop just before echo'ing the row. Instead of returning you should assign to a variable and print that variable in the loop. You only use return when exiting a function, which it doesn't appear your code is doing. Some how I think you got the idea that you have to use return to execute the code in the if or something like that.
-
First off, try entering your query directly into phpMyAdmin to see if it behaves expectedly. SELECT SUM(TotalPrice) FROM Cart Once you're sure of that, you can check your PHP code for problems. The first obvious one I can see is in your first code post: $query1 = mysql_query ("SELECT SUM(TotalPrice) FROM Cart"); if(!qyery1) echo "Query lesh"; You have mistyped the variable name. In your second code post, you execute a query and save the result in $query, but echoing query isn't going to print the value from the DB. You still have to call mysql_fetch_array; normally I would use mysql_fetch_assoc but since you didn't alias the column, mysql_fetch_array is easier IMO. $query = mysql_query ("SELECT SUM(TotalPrice) FROM cart"); if(!$query) // <-- YOU FORGOT THE $ IN YOUR CODE { echo "error"; } else{ $row = mysql_fetch_array($query); echo $row[0]; } Basically you are being very sloppy. You should be able to catch things like missing dollar signs and misspelled variables based on interpreter errors, or just carefully reading your code. Also, even though they are optional when the body is a single line, you should always enclose your if and loop bodies in curly brackets. Don't be lazy.
-
Storing numeric values efficiently in a text file
roopurt18 replied to tcjohans's topic in PHP Coding Help
From the comments in the PHP Manual: As for your second part, you're better of just using a standard one size fits all. First, it keeps things simple. If you want to use variable byte width you will also have to place flags in the file to indicate how many bytes the next value is. That will complicate both your code that will read and write to the file. Second, you won't gain much space. Let's say an int is 4 bytes and a short is 2. Let's say that you write a single int and a single short, thats 6 bytes. You will also need to use at least 1 byte to flag each of them, bringing the total up to 8 bytes. So you've saved nothing. Of course, this depends on your data you'll be writing and the actual size of an int or short on the target platform. -
When you have error reporting set to report everything, the interpreter will raise errors whenever you use a variable or array index that has not yet been defined. <?php session_start(); if($_SESSION['auth']){ echo "logged in"; } ?> On the first hit to the page, session_start() will create an empty session, meaning that $_SESSION is empty and the index 'auth' in $_SESSION doesn't exist. In that case, you do one of two things: 1) Change the level of your error reporting. 2) Change the comparison <?php session_start(); if(isset($_SESSION['auth']) && $_SESSION['auth']){ echo "logged in"; } ?>
-
payney, I gave you an example of how to use the NOW() function, but here it is again: $query = "insert INTO home_page (title, content, `date`) "; . " VALUES ('$title','$content', NOW()) "; NOW() is a MySQL function, which means you can't call it in PHP. In other words, PHP doesn't execute NOW(). You place NOW() in your query just as if it were any other value, except you don't put it inside single quotes. Basically, your final query has to look like this: INSERT INTO table (datetime_column) VALUES (NOW()) MySQL sees NOW() and replaces the function call with the actual time and uses that value.
-
Storing numeric values efficiently in a text file
roopurt18 replied to tcjohans's topic in PHP Coding Help
In binary, all values of a particular type are the same size. For example, an integer may be 4 or 8 bytes. All integer values, regardless of the value, will use the same amount of storage space. So the value 1 uses the same amount of space as 32,000. If you know that all of your numbers are smaller than the maximum size for a short integer, you can typecast them all to (short); note that I don't actually know for a fact that PHP supports the short integer data type, just that other languages do. If you know that all values are positive, you can typecast them as unsigned, assuming that PHP supports that as well. -
Noob at PHP- making a "higher/lower" card game.
roopurt18 replied to frenz_hilpur's topic in PHP Coding Help
It depends on the site IMO. I wouldn't take the time to do it in the majority of sites I created. But if you were running an online MMO that had paid subscribers, I think you owe it to your users to try and eliminate cheating as much as possible. Also, setting a very clear standard that you attempt to detect cheating and punish those that do is a good way to prevent it in the first place. After all, you'd be scaring away cheaters, not honest customers. Just MO. -
Noob at PHP- making a "higher/lower" card game.
roopurt18 replied to frenz_hilpur's topic in PHP Coding Help
You totally missed the point. I was agreeing with you that a $_SESSION variable is the best way to temporarily retain the value, since it can't be changed by the user like a hidden input could. But I was then saying add the hidden input anyways. Anyone who is tempted to cheat is first going to inspect the HTML of the form. If you fool them into thinking the value that will be compared is stored as an input it should be easy to discover who are the users that attempt to cheat. You would know they attempted to cheat because you could compare the value from the hidden field to the correct value in the $_SESSION. -
Noob at PHP- making a "higher/lower" card game.
roopurt18 replied to frenz_hilpur's topic in PHP Coding Help
IMO you should still include the original value as a hidden parameter; that way you can check it against the one stored in the sessions, discover who the cheaters are, ban them from your site (temporarily), and maybe even redirect them to tub girl. -
Use them where they make sense. I use the class keyword in PHP4 to create a name space to wrap up common database access functions. For example, the blunt of functions that return user data are accessible from UserDAO. I don't actually instantiate a UserDAO object, I just call the methods statically, i.e. UserDAO::getUser($id). Some people like to create objects to encapsulate the data returned from the database. Personally I find this is rarely necessary. In most cases, you are just querying for data for simple output; for this, arrays work just fine. There is no reason to create 200 objects just to display a table with 200 rows of some type of data. I frequently use objects to represent entities that are part of a site's framework. For example, I might have a Page object that defines characteristics common to all pages. Each page in the site is derived from this object and overrides functionality where necessary, but otherwise using the base functionality. This is the type of stuff in web development that I use OOP the most frequently for.