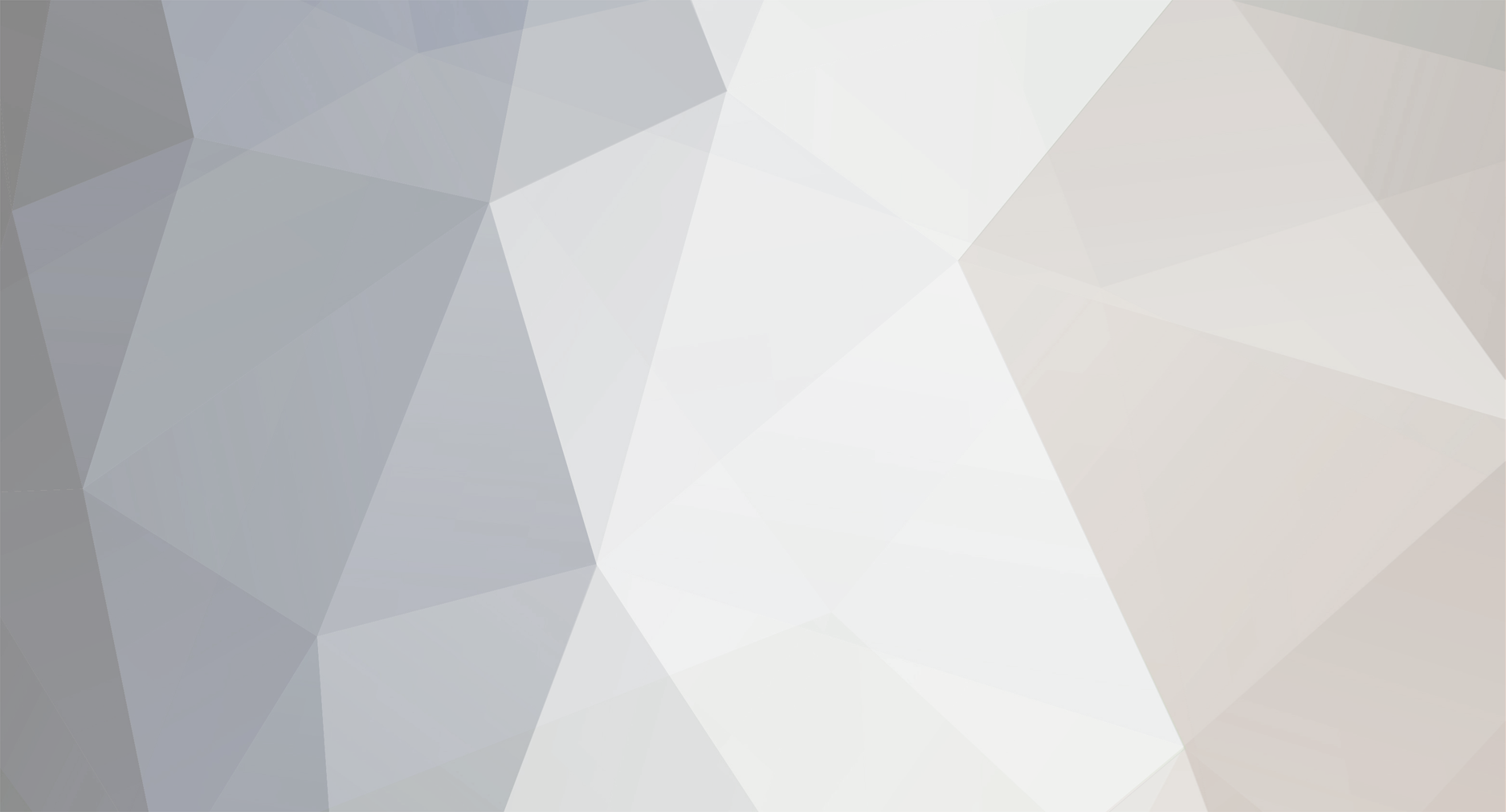
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Noob at PHP- making a "higher/lower" card game.
roopurt18 replied to frenz_hilpur's topic in PHP Coding Help
You're trying to generate an HTML form, so this really belongs in the HTML board. Anyways, here is a short example: <?php // First check if the form was submitted if(count($_POST)){ // There are items in the $_POST array, thus it was submitted echo "<pre style=\"text-align: left;\">" . print_r($_POST, true) . "</pre>"; exit(); } // If we made it here the form was not submitted, so we display the form instead // Generate a single random number $num = rand(1, 25); // Exit PHP mode and display a form ?> <form method="post" action=""> <p>The number is:</p> <p style="text-align: center;"><?php echo $num; ?></p> <p style="text-align: center;"> <input type="hidden" name="num" value="<?php echo $num; ?>" /> <input type="submit" name="lower" value="Lower" /> <input type="submit" name="higher" value="Higher" /> </p> </form> There is a glaring security hole in the design of this game, but we'll worry about that later. -
Learning how things work under the hood simply opens up your options. If you have two different functions that perform the same task but perform it differently and you understand the differences, you can better decide which one to call based on your current situation. Likewise, when you are faced with a "special case" for which there is no built in function, understanding how others solved similar, more simple, problems can help you solve your own. Lastly, writing your own functions to duplicate built in methods is a good way to learn about algorithm analysis and code efficiency. There should come a time when one is familiar enough with such concepts that they use the built in methods and tools where available though.
-
mjdamato didn't say you couldn't. He said the variable defined in the function isn't visible outside it. I do that frequently when I have to perform a sequence of conversions on the single value, so its perfectly valid to do so. However, in this case it is a mistake (most likely) because the function is returning the index of the smallest value but without the array to use as a reference, the index isn't worth much. @ the OP <?php function hittaminstatal($lista){ $min = FALSE; if(!is_array($lista) || !count($lista)){ return $min; } foreach($lista as $key => $val){ if($min === FALSE){ $min = $key; }else if($val < $lista[$min]){ $min = $key; } } return $min; } $lista = array(8, 23, 12, 4); $min = hittaminstatal($lista); // This $min is not the same as that in the function, google 'variable scope' echo $min . ", " . $lista[$min]; ?>
-
Well that seems to work. Do you know why it is that makes the difference? I only need enough information to research it further myself.
-
The only thing I can think of is a bug [fix] in the MySQL 5.0.x client.
-
There's a test server and live server. Test server is MySQL Server version: 5.0.27-standard. Live server is MySQL Server version: 4.1.22-standard-log. The PHP code on both servers is identical. A database was copied from the live server to the test server to troubleshoot a problem. The particular web page is supposed to pull information from the database and just create a table. On the live server a table is generated. On the test server a message is generated saying that no documents could be found. These documents are not stored on disk so that is not the problem. Test Server Query SELECT t.*, o.*, t.RevNum AS TrackRevNum FROM POOptions o, wsjob j, IBSSubName2WVSubName sn LEFT JOIN DocTrackingPOs t ON t.PONum=o.PONum WHERE o.SubName=sn.IBSSubName AND sn.WVSubName='BBP' AND o.JobCode=j.job_code AND j.pro_code='7795' Live Server Query SELECT t.*, o.*, t.RevNum AS TrackRevNum FROM POOptions o, wsjob j, IBSSubName2WVSubName sn LEFT JOIN DocTrackingPOs t ON t.PONum=o.PONum WHERE o.SubName=sn.IBSSubName AND sn.WVSubName='BBP' AND o.JobCode=j.job_code AND j.pro_code='7795' Both queries are identical. Copying either query into phpMyAdmin on the test server gives: #1054 - Unknown column 'o.PONum' in 'on clause' Copying either query into phpMyAdmin on the live server gives 74 rows. I've not checked that they're identical, but I'm pretty sure they are. All four of the tables involved in the query have the same number of records according to phpMyAdmin, although I've not checked that they're identical. Any thoughts?
-
DATE fields in MySQL are stored as 'YYYY-MM-DD'. Any time you insert into one of these fields MySQL will try and coerce the value you're inserting into that format. If it can do so, it will. If it can not, it will use NULL or '0000-00-00'. So the end result doesn't matter if you use PHP's date() function to create and format it or MySQL's NOW() function; in both cases the date appears in the same format, 'YYYY-MM-DD'. Many PHP programmer's, especially beginners, will extract the date from the DB just as it appears and then use PHP's functions to format it. Some people will use multiple calls to substr or explode to break the date string down into the individual parts and then create a timestamp with PHP's mktime function. A more efficient method in PHP is to just use strtotime(). The best method that thorpe hinted at but didn't elaborate on, is to use another MySQL function DATE_FORMAT(): http://dev.mysql.com/doc/refman/5.0/en/date-and-time-functions.html#function_date-format Let's say that you are extracting the date and want to display it as a more readable format. SELECT DATE_FORMAT(dateCol, '%b. %D, %Y') AS `dateCol_disp` FROM myTable WHERE ... What this will do is format the column dateCol to something like Jan. 1st, 2007. Now you don't have to do any formatting in your PHP! While looping over the result set you can just say echo $row['dateCol_disp']. Notice how the name referred to the column in PHP is the one in the query where it says AS alias. Whenever you return an aliased column, I recommend giving it a name that is not used by any of the columns in any of the tables in the query; it is not necessary to do so, but it helps avoid difficult to find bugs in the WHERE and ORDER BY parts of a query. MySQL also has other functions to extract just parts of a date, such as the year or month; they are listed on the same page as the DATE_FORMAT() function I linked you to.
-
@ the OP Let's start backwards and work our way into it. Your error is Call to undefined function showerror(), which is a PHP error. You are calling this function when your query fails, but it is not a built in function and you didn't write the actual function so PHP can not find it and creates an error. To get rid of the PHP error, write a function: function showerror(){ echo mysql_error(); } showerror() is only called when the query fails, so the next step is to determine why it is failing. There are two possible reasons that it could fail. The first depends on $content. I highly suspect that $content should be enclosed within single quotes inside your query. The second reason is your table contains a column named date. date is a reserved word in MySQL so you must enclose it in backticks (the unshifted key above the tab key on your keyboard). Try replacing your query generating lines with this: $query = "insert INTO home_page (title, content, `date`) "; . " VALUES ('$title','$content', NOW()) "; Two general comments about your code. If you're doing many concatenations on a string variable its slightly more efficient to do so the way I have written in the fixed query. The reason is because every line below the first contains as much of the string as possible and since there is no assignment except for the first line, it is slightly easier to read that all of it is happening to the variable on the first line. The second is that you have if statements with no curly braces; I know that they are optional if the body of the statement is a single line, but I highly recommend always using them, as well as with loops. @ ~n[EO]n~ The OP was placing the call to NOW() as part of his query, not as part of his PHP code. What he was doing was perfectly valid and also the recommended method. You should always strive to place as much of the work as possible on the database engine. Why bother with creating extra PHP to generate the time, assign it to a variable, and then insert that into the query when you can just write NOW() inside the query where the PHP-generated date would have appeared? @ both of you Neither of you cleaned your data with mysql_real_escape_string() so both sets of code are vulnerable to database attacks. Also, be consistent with your capitalization in your MySQL, it just makes it easier to read.
-
What corbin is hinting at is called automatic type conversion, which is to say that PHP will automatically convert from one data type to another where it is able to. An example <?php // Here we declare an integer, it is an integer because it is a numeric value, not // contained within quotes, and has no decimal $int = 5; // Here we declare a float (or double), it is a float (double) because it is numeric, not // contained within quotes, but it does have a decimal $float = 5.0; // Here we declare a string, it is a string because it is enclosed in double quotes $str = "5.0"; ?> I will use an analogy to gloss over this next part. Let's consider three types of engines: a lawn mower motor, a car engine, and a jet engine. All three of those are the same in that they're each an engine. But I shouldn't have to prove to you that underneath the hood they are all vastly different in organization and complexity. The same thing occurs with those three variables above. They are the same in that in some manner they all represent the value 5, but how the computer stores and recognizes them internally is as varied as the three engines. However, it is quite often the case in programming that we have a value stored in one format and want the equivalent value in a different format. Converting from one format to another is called type conversion. There are some conversions that can be handled automatically. For instance, if we continue with the variables I defined above: echo $int * $str; Here we are using the multiplication operator. Multiplication is well understood in math and is applied to numbers; thus the multiplication operator expects two numeric values. However we are giving it a numeric value and a string value. The PHP interpreter will automatically attempt to convert the string value to a number for us. Since the string is the value "5.0", the conversion is easy and PHP will calculate 5 * 5 and print out 25. There are many values that we can convert automatically: int => floating point int => bool There are some conversions that aren't straight forward: "Hello World" => integer ??? In these cases PHP picks a default value, such as FALSE, null, zero, an empty array, etc. The default picked depends on the originating data type and the desired converted data type. Anyways, to make a long story short, you don't really care what the actual data type of a variable in PHP is for 99.99% of the time. You just care that it is a valid value for how you intend to use it. For instance, values contained in $_POST and $_GET are actually strings. Yet you can take a value from $_POST and perform math on it without performing any manual conversion. Pretend you had written a function that expected a numeric input. a_func(5); a_func("5"); function a_func($val){ if(!is_int($val)){ return FALSE; } // else do something } Notice that the function is called twice, once with an int argument and another time with a string argument. Because the function is checking if the parameter is of type integer, the second call (with the string) will return false, even though the string could have been used numerically. The better version of the function would change the call from is_int() to is_numeric(). Hope that helped some.
-
Storing numeric values efficiently in a text file
roopurt18 replied to tcjohans's topic in PHP Coding Help
Next time you encounter this problem, just open the file for binary access and use fwrite(). -
If you note the documentation for the trig functions, such as sin(), the documentation states the expected argument is in radians. It is hardwired. If you're users are entering a value as degrees, then you must convert using deg2rad(). "degree" and "radian" are not data types in any language that I know of as they're already perfectly encapsulated as floats or ints. Degrees, radians, miles between two points, your age, they're all just numbers. It is the context in which you use them that gives them meaning.
-
Now that is one busy guy.
-
In order to create a programming language you must do (at least) the following (I'm a bit rusty so there might be more): * Develop a grammar; this can be done on pen & paper * Write a parser / tokenizer; this is what opens the source files and structures their contents for processing The next step depends on if you're creating an interpreted or compiled language. If an interpreted language, like PHP, you execute the instructions represented by the data structures created from parsing. If you are creating a compiled language you translate the instructions represented by the data structures from the parsing step into binary instructions for your platform and dump them into a file. This means you need to learn about that platform's expected executable file format. Most compilers, not sure about interpreters, won't go directly from parsing to executable. They'll create an intermediate source file that is basically a generic form of assembly (I think it's referred to as byte code). What this means is its cryptic like assembly (ADD, SUB, MUL, DIV, MOV, etc) and uses fake registers ($R1-$RI, $S1-$S8 as examples) but most assemblers won't be able to assemble (compile) it. The reason they do this is it helps with code optimization. As a quick example, let's say we had the following intermediary source: mov $r2, $r1 # Move $r1 into $r2 # 100 lines of source code mov $r2, $r6 # Move $r6 into $r2 Both of those mov statements assign to the same register, $r2. However, if we can determine that in the 100 lines of commented code that $r2 is never used, we can safely remove the first assignment and save ourselves an instruction. From this assembler-like intermediary code, I think most compilers will then convert into correct assembler for the target platform. Another stage of optimization will occur. During this stage instructions are re-ordered where possible to take advantage of the CPU pipeline. That's most of what I can remember when I studying this stuff in college. I can assure you, ronald, that this can all be accomplished in PHP.
-
[SOLVED] Get line of string from a text file?
roopurt18 replied to Pentti's topic in PHP Coding Help
You might want the function to return FALSE or -1 as the last line of the function for cases where the search string is not found. Also, instead of calling count() and using a while loop, you could use a foreach loop, which is intended for looping over arrays. This is a very, very minor optimization note, but you can apply the concept to other areas of your code. while($i <= $c_size - 1){ That is perfectly fine for your while loop, but you are forcing the interpreter to re-evaluate the expression $c_size - 1 each time through the loop. So let's say the file was 1000 lines long, the expression $c_size - 1 will be calculated 1000 times; however, it's always going to be the same result. This means we can move the calculation outside the loop so that it is only ever calculated once: $c_size--; while($i <= $c_size){ You can eliminate the -1 operation altogether by changing the comparison in the while loop as well: while($i < $c_size){ Like I started off by saying, that is a very minor optimization and barely worth performing; it's only going to shave maybe a millisecond off your execution time, if that. However, if the $c_size - 1 had been a different, more expensive (expensive in terms of computation time) operation, such as a function call, it's a good optimization to make. Lastly: if(strpos(Trim($c_array[$i]), $searchstr)) return $i; That code is perfectly legal, but I highly recommend always, always, always placing curly brackets around the bodies of your conditional statements and loops, even when they're a single line and optional. The reason for this is its very easy to add another line to the body of a conditional or loop to enhance functionality or for debugging purposes but then forget to add the curly brackets, which breaks the loop / conditional. It only takes an extra second to type the keystrokes and sure it adds one more line to your source file, but honestly it can save you a good half hour or more of frustration down the road. if(strpos(trim($c_array[$i]), $searchstr)){ return $i } You should also always indent the bodies of your conditionals and loops as well, to enhance readability. I only bring this stuff up now because you are learning so its easier to develop good habits now rather than break bad habits later. When I was learning to program, my sources (books / professors) said the same things and you just have to take it on faith. I decided to listen and I can't tell you how many times throughout college I saw people have trouble debugging or fixing their code because they didn't do little things like that. Best of luck to you. -
I have better than 20/20 vision and I still think your font is too small. Ditto to what others said.
-
From the documentation on mysql_query(). I'd say in most instances if you've run the query multiple times in testing and it appears to work, just check the return value of mysql_query and don't worry on the exact number of queries deleted, updated, or inserted. If you really start to think about it, trying to define success in queries across multiple tables becomes difficult. Even in an UPDATE statement that could affect many rows, mysql_affected_rows() only returns the ones actually affected. In your case, it looks like you're deleting forum posts or messages. It'll be pretty easy to know when it's not working because the post / message will still be there.
-
I could be mistaken, but I think I once read somewhere that mysql_affected_rows has trouble when used with statements that delete from multiple tables.
-
[SOLVED] Get line of string from a text file?
roopurt18 replied to Pentti's topic in PHP Coding Help
The code I gave you IS the function body. Just make it return $key and you're done. You don't need to loop or perform any comparisons yourself; there are already built in functions to handle this stuff. Why re-invent the wheel? -
I think your query needs to change to: DELETE `posts`.*, `content`.* FROM `posts`, `content` WHERE posts.id = content.id AND posts.id in (59) I could be wrong though, so try that on test data first.
-
They still make those?!
-
[SOLVED] Get line of string from a text file?
roopurt18 replied to Pentti's topic in PHP Coding Help
There is already a built in function to open a file and return everything as an array. There is already a built in array search function. $file = file("the/file"); $key = array_search($search, $file); if($key === FALSE){ echo "Not found"; } If you want all matching lines, use the array_keys() function. -
If you look carefully you will see an extra double quote in this line: if(!file_exists($dirname")) mkdir($dirname, 0755);
-
Convert the value to an integer. if($empty($miles) || ((int)$miles) < 0){ return false; }
-
This is all a matter of opinion, but for me C is simply the best language there is all around, followed closely by C++. There simply isn't any program that couldn't be written in one of those two languages. They both provide low-level hardware interaction which makes them ideal for embedded and real-time systems as well as games. They're both compiled so they execute fast. The advantage that C provides over C++ is that it's easier to write a working C compiler than it is a C++ compiler. So if you're working with state of the art hardware and have to write a compiler for it your job is easier. C++ has the advantage of OOP (and a few other things) and wrapping things up behind more simple interfaces. I've never programmed in Java but IMO the one thing holding it back is garbage collection. While garbage collection certainly makes things easier on the programmer, it hurts application performance IMO. From my experience using Java programs, they typically run great for a while but they longer they're left executing the more sluggish they become. Garbage collection makes using Java for certain systems impossible because you can't predict when it will kick in. For example: As far as learning one of the languages goes, you're best off buying a book as it will have examples and instructions geared to your experience. If that's not an option, you should Google search for specific programming examples as they relate to the language. For example: c++ loop tutorial c++ function tutorial c++ class tutorial etc. When reading the examples and you don't understand what something is, look it up in Google. char line[256]; cin.getline(line, 256); Google for 'c++ getline' to learn about it.
-
$_POST variables are available from form posts. $_GET variables are available from the URL. So AFAIK you can't directly do it. You could place it in the URL and at the top of the script do: $_POST['someVar'] = $_GET['someVar']; and set the value manually if something in your script relies on it.