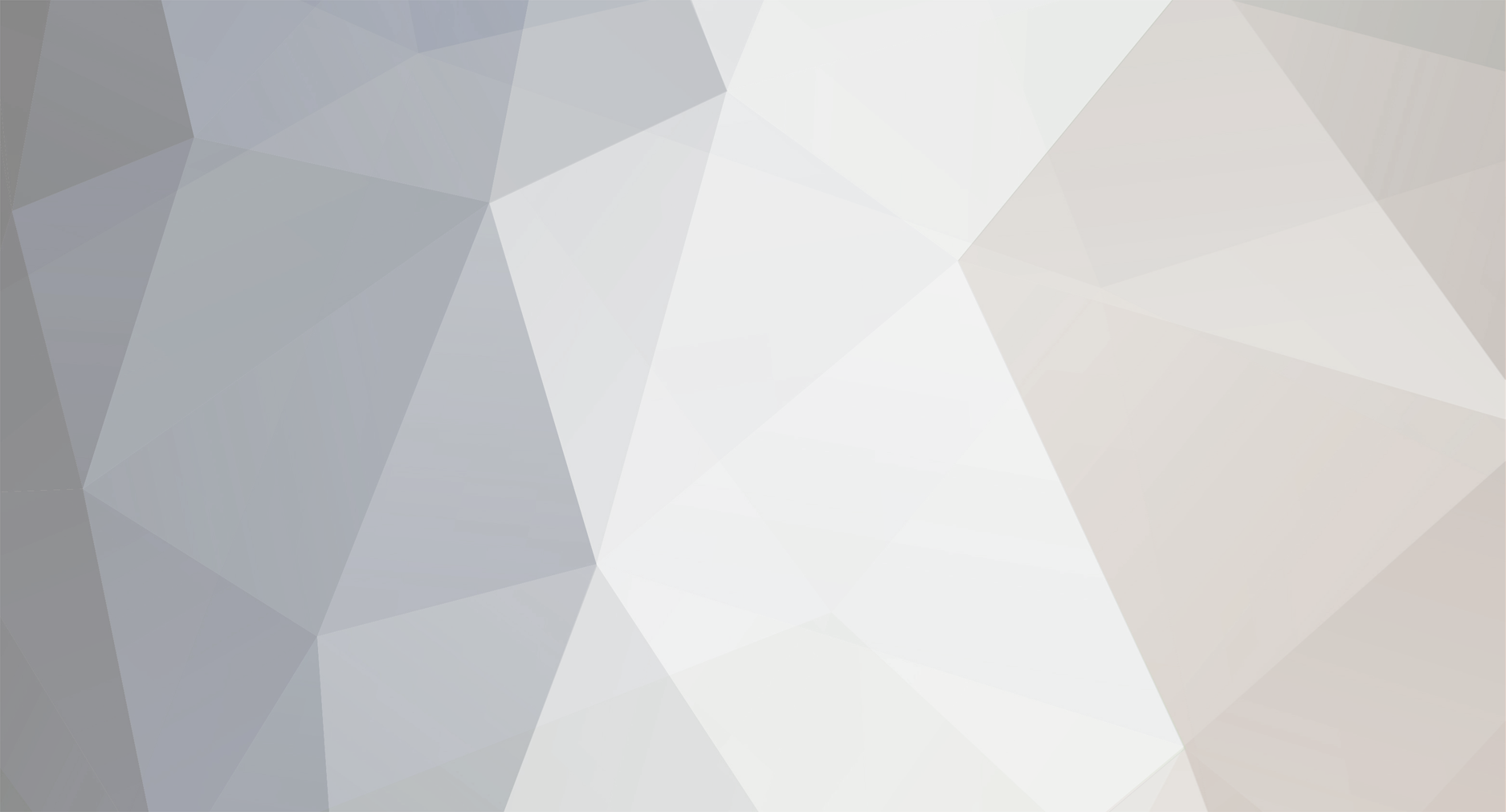
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
this is just HTML code, you need to make sure it's clean
for example, the <body onload=\"add();\"> shouldn't be in the middle of the page, you can just take it out (I added it just in the example)
the <div id=\"code\"> should have a close tag
[code]
<div id=\"code\"></div>
[/code]
You can put it anywhere you want (for example, before the <p>)
-
the onclick='add()' doesn't work because you are using document.write()
document.write will over write the whole document (including your javascript code), you will need to use innerHTML to add the HTML to a div or another element
Here is a sample HTML and JavaScript code
[code]
<html>
<head>
<title></title>
<script language="javascript">
function add() {
var i = 1;
var txt = "<input type='checkbox' name=prot[] value='prot'> Selection of Protonation of Residues:<br>SEGID : <select name='segid'><option value='PROA'>PROA<option value='PROB'>PROB<option value='PROC'>PROC<option value='PROD'>PROD</select>";
txt += "Residue : <select name=resi> <option value=ASP>ASP <option value=GLU>GLU <option value=LYS>LYS </select>";
txt += "Residue Id : <input type='text' name='resid' onClick='add();'><br>";
document.getElementById('code').innerHTML += txt;
}
</script>
</head>
<body onload="add();">
<div id="code">
</div>
</body>
</html>
[/code] -
you need to include the value in " in your HTML
[code]
<input type="text" name="first" value= "<?php echo $fname ; ?>" size="40%">
[/code] -
if your document.write there is a
value="prot">
change all the " to ' inside the document.write and it should work. -
you can do something like this
[code]
<a href="#" onclick="window.open('sc.php'); return false;">What is this?</a>
[/code] -
Ok, I am attaching a version without the ajax stuff, you'll notice I have names and ids for everything. This should work in any browser
[code]
<html>
<head>
<title></title>
<script language="javascript">
function which_sel(){
// you can do this
var sel = document.places.sel2;
alert(sel);
// or this
var sel2 = document.getElementById('sel2');
alert(sel2);
}
</script>
</head>
<body onload="which_sel();">
<form id="places" name="places" name="form1" method="post" action="">
<select name="sel1" id="sel1" onchange="javascript:sendRequest('foo')">
<option>unnamed1</option>
<option>unnamed2</option>
</select>
<select name="sel2" id="sel2" onchange="javascript:alert(this);">
<option>unnamed1</option>
<option>unnamed2</option>
</select>
</form>
</body>
</html>
[/code] -
-
you miss understood my comments
If you want to use document.getElementById("sel2") the select have to have an id
you cannot have document.places.getElementById
If you use your same code above (the same script you posted) all you need to do is add the name to the form
[code]
<form id="places" name="places"............
[/code]
and keep the sel as is
[code]
var sel = document.places.sel2;
[/code] -
I am not sure if there any sites that shows this, but it happens to me sometimes when I build CSS layout templates.
I don't know why it happens, but I know it's related to floating elements inside floating elements, and IE sometimes will show it if you select the whole thing or click on it. -
when you use document.getElementById, the object has to have an id property
[code]
<select name="sel2" id="sel2".........
[/code]
I tried your original code, and I found out you have an id for the form, but not a name
just add the name property to the form and it should work
[code]
<form id="places" name="places"............
[/code] -
I think you can do it without sending an email, but with communicating with the mail server, check this tutorial in zend.com
http://www.zend.com/zend/spotlight/ev12apr.php
The problem will be if someone entered a valid email, but someones else. So sending an email and require validation will be the best option. -
First make sure the page load before you try to access any objects, but your script in a function and call in when the page load.
Also, it's always better to use document.getElementById -
This is a CSS problem, not PHP.
Since you have floated elements, you'll need to clear them at some point.
Try to add style="clear:both;" in the content div and see it shows or not.
Also, try to remove the class all togather and test it. -
You'll need a payment gateway and online merchant account. verisign provide online payment service.
-
Hotmail spam filter is very strict, check your spam score (using spam assassin if you have access to it). If your message is higher the 1.5 points, hotmail will block it.
-
in your suspend.php
when you echo the $newsettingscontent, is it the correct string?
I notice you have the function file_put_contents_php4 () but you are not calling it anywhere in the script, so the file won't be edited.
Let me know if this solves the problem. -
If you have no links to your directory /scripts/ anywhere and no one else is linking to that folder, search engines shouldn't find it.
robots.txt was created to stop search engines from getting pages that have linked but access requires sign up or something else. Or the site owner doesn't want his page on search engines.
Seconds, search engines only read the output of the file (so, php, asp, html, or whatever) will be the same for search engines. So, if your script files don't output anything, nothing will be spidred. -
you need to add a \ infront of the $ in your strings, otherwise the script will look for the $maintenance value
[code]
$newsettingscontent = str_replace("\$maintenance = 0;", "\$maintenance = 2;", "$settingscontent");
[/code] -
sorry, I miss understood your question
You need to set the meta tag so the page is not saved in the browser cach
[code]
<META HTTP-EQUIV="CACHE-CONTROL" CONTENT="NO-CACHE">
<META HTTP-EQUIV="EXPIRES" CONTENT="Mon, 22 Jul 2002 11:12:01 GMT">
<META HTTP-EQUIV="PRAGMA" CONTENT="NO-CACHE">
[/code] -
I think you need a \ before the $ in your mode strings
[code]
23 $mode[0] = "\$maintenance = 0;";
24 $newmode[0] = "\$maintenance = 2;";
[/code]
If you want to change the actual text "$maintenance" not the variable value of $maintenance
Also, since you are not using any reg expressions, it's best to use str_replace() because it runs faster and uses less resources. -
if you slice it in photoshop, you gotta set the image as background. This is just an example
Change this
[code]
<td colspan="5">
<img src="images/Untitled-1_20.gif" width="366" height="59" alt=""></td>
[/code]
to
[code]
<td colspan="5" background="images/Untitled-1_20.gif" width="366" height="59">
Your text goes here
</td>
[/code]
-
try history.go(-2) or another number
-
can you post your javascript code,
Also in FF, you can always click on "Tools"->"JavaScript Console" to find out the error with your code. -
take a look at this
http://www.phpfreaks.com/forums/index.php/topic,95771.msg383392.html#msg383392
Javascript with PHP
in PHP Coding Help
Posted