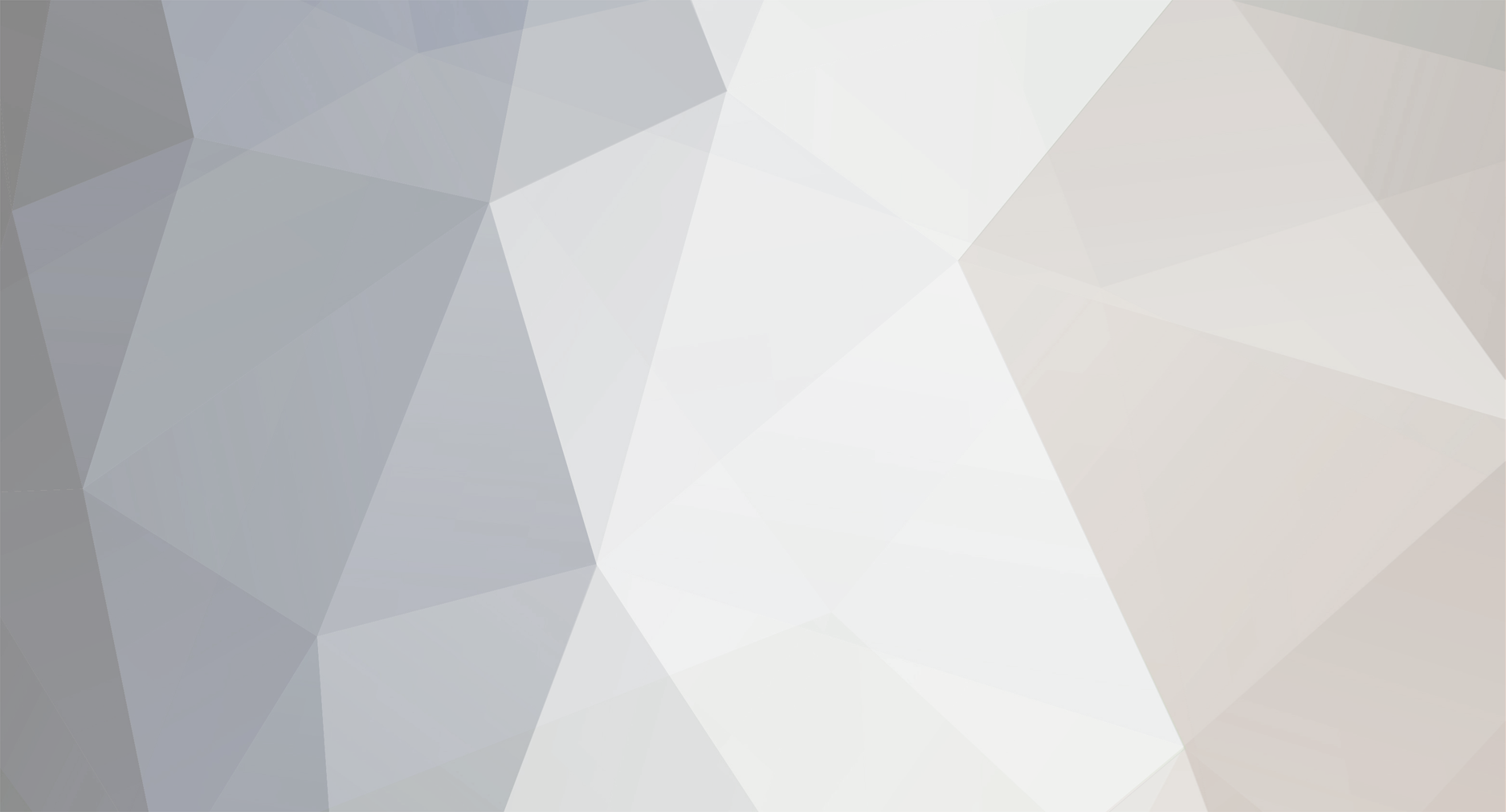
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
The variable names are the problem since they should be overwritten in those scenarios. Besides, the error given by PHP is . . . That means the query failed. You are calling a stored procedure twice with the same parameters. not knowing what that stored procedure is it is impossible to know if that is correct or not. but, you can help yourslef out by checking the actual error from mysql <?php require_once ('connection.php'); //First Query and Output $query = "CALL C01_Client_Summary_ByAccount(1, '2012-02-27', '2013-03-29');"; $result = mysql_query() or die("Query: $query<br>Error: " .mysql_error()); while($row=mysql_fetch_array($result)) { echo $row['CommisionPercentage']; } //END First Query and Output //Second Query and Output $query = "CALL C01_Client_Summary_ByAccount(1, '2012-02-27', '2013-03-29');"; $result = mysql_query() or die("Query: $query<br>Error: " .mysql_error()); while($row=mysql_fetch_array($result)) { echo $row['Turnover']; } //END Second Query and Output ?>
-
No "strangeness" to it. Take a look at this tutorial on file uploads and you should see what the problem is. http://www.tizag.com/phpT/fileupload.php
-
I would have guessed that PHP would have a built in function to do an array_intersect using the values of one array against the indexes of another, but I don't see one. So, I see two options that would not require a foreach loop. 1. Use the POST array values to create an array using those as keys using array_fill_keys() and use that with array_intersect_key() to get the resulting array. $selectedValuesAry = array_intersect_key($callstoaction, array_fill_keys($_POST['calltotor'])); 2. To make things VERY simple you can just modify your checkboxes so you have ALL the information in the POST array. Use the keys from the $callstoaction array as the keys of the checkbox array and the values as the values: <input type="checkbox" name="calltotor[defaulttor]" value="Default Tornado Warning"> Default Tornado Warning<br> <input type="checkbox" name="calltotor[mobilehome]" value="Mobile Home"> Mobile Home<br> The post value $_POST['calltotor'] will now have all the selected options and ther keys and values will be the same as the associated keys and values from the master array. You SHOULD be creating those checkboxes dynamically from the master array anyway, so this is by far the easiest and smartest option, in my opinion.
-
Toggle Visibility of Multiple Items Simultaneously
Psycho replied to sleepyw's topic in Javascript Help
I'm not sure how this being JavaScript vs. PHP is an issue. The problem is not language specific - it is a simple logic problem that is independent of the language. You could just code the function to iterate through all of the DIVs. Ones that are not the clicked one would be automatically hidden. And the one that was clicked would be toggled (as you have now). Without being an expert in JS you could just hard code the function for the four DIVs, But, a beter way is to name all the DIVs the same and reference them as a collection. <html> <head> <style> .unhidden { background-color: #ff0000; height: 190px; width: 300px;} .hidden { visibility: hidden;} </style> <script type="text/javascript"> function taggleDivs(selectedDivID, divsName) { var divList = document.getElementsByName(divsName); var styleName; for(i=0; i<divList.length; i++) { //Set default for all unclicked divs classNameVal = 'hidden'; if(divList[i].id == selectedDivID) { //Set value for the clicked div classNameVal = (divList[i].className=='hidden') ? 'unhidden' : 'hidden'; } divList[i].className = classNameVal; } return; } </script> </head> <body> <div><a href="javascript:taggleDivs('ONE', 'toggleDiv');" >ONE</a></div> <div id="ONE" class="hidden" name="toggleDiv">Text that only shows up when ONE is clicked.</div> <div><a href="javascript:taggleDivs('TWO', 'toggleDiv');" >TWO</a></div> <div id="TWO" class="hidden" name="toggleDiv">Text that only shows up when TWO is clicked.</div> <div><a href="javascript:taggleDivs('THREE', 'toggleDiv');" >THREE</a></div> <div id="THREE" class="hidden" name="toggleDiv">Text that only shows up when THREE is clicked.</div> <div><a href="javascript:taggleDivs('FOUR', 'toggleDiv');" >FOUR</a></div> <div id="FOUR" class="hidden" name="toggleDiv">Text that only shows up when FOUR is clicked.</div> </body> </html> EDIT: not sure why you set the style properties as you have them. Why not define the size as static and just toggle the display property instead of the visibility? -
Displaying Column in One Table Based on Another Table
Psycho replied to loren646's topic in MySQL Help
You should NOT be storing information into the database to save a selection that a user made on a page to determine what happens on another page. That is what session data is for! But, in this case you don't need to use session data at all. In fact, you only need 2 pages (although you can create separate pages for separating the code into logical chunks for the purpose of including in page 2) Here is a framework of how page 2 might work <?php if(!isset($_POST['landlordselect'])) { //No landlord selected, redirect to or includepage 1 header("Location page1.php"); exit(); } //Create vars from POST data. $landlordselect = trim($_POST['landlordselect']); //These vars will be used to repopulate the form if an error occurs to make the form "sticky" $building = isset($_POST['building']) ? trim($_POST['building']) : ''; $apartNo = isset($_POST['apartNo']) ? trim($_POST['apartNo']) : ''; $price = isset($_POST['price']) ? trim($_POST['price']) : ''; //Set default error message $errorMessage = ""; //Check a field to see if data to add apartment was submitted if(isset($_POST['price'])) { //User submitted form to add apatment //Perform validation of input data //If there are NO validation errors then insert the record and set //the form vars to empty strings so the form does not repopulate //If there are errors, then set the error message var accordingly //The vars for $building, $apartNo, etc. will be used to repopulate the form } ?> <html> <head></head> <body> <?php echo $errorMessage; ?><br> <form action="" method="post"> <input type="hidden" name="landlordselect" value="<?php echo htmlspecialchars($landlordselect); ?>" /> Building: <input type="text" name="building" value="<?php echo htmlspecialchars($building); ?>" /><br> Apartment #: <input type="text" name="apartNo" value="<?php echo htmlspecialchars($apartNo); ?>" /><br> Price: <input type="text" name="price" value="<?php echo htmlspecialchars($price); ?>" /><br> </form> </body> </html> -
Displaying Column in One Table Based on Another Table
Psycho replied to loren646's topic in MySQL Help
I'm just getting more confused. Based upon some earlier code you were using a value passed int he POST data ($_POST['landlordselect']) to determine which records to display. But, now you show a table which has a user and selected property values. So, is the dynamic value going to be the property or the user? Also, you should not be using the property name as the values that ties the two tables together. You should have "property" table that has each unique property name with a unique ID. Then use that ID in place of the property name in the related tables. Anyway, with the structure you have (which I don't advise) you can get those record either with a variable property name SELECT building FROM land WHERE landlord = '$landlordselect' Or with a variable value for the username SELECT building FROM land JOIN user ON user.addbuilding = land.landlord WHERE user.user = '$username' -
Not sure exactly what you are referring to when you say "globals" since that can mean a few different things. I'm guessing in this context you are referring to one of the superglobal arrays such as $_POST and $_GET. While you *could* use them to transfer information from page to page, it would not be a good solution. You definitely want to go with session data.
-
PHP Checkbox Echo - The Solution Must Be Simple - I Need Help Finding It
Psycho replied to surge42's topic in PHP Coding Help
OK, let's take one step at a time. OK, how do you know it is stored? Have you verified the value through PHPMyAdmin? Have you echo'ed $user_type_detail["projectcomplete"] to the page to verify it's contents? have you verified that the field name is correct (you state the last example works but it has a typo in the field name)? Are you sure the value is exactly "Yes" and not "yes" or "YES"? Don't assume what variables contain because you think you know what they should contain. Test Them! Echo them to the page or write some code to test them. That is the basics of debugging. Also, I would highly suggest NOT using "Yes" or "No" for a field value. instead make the field an INT and store 0/1 which can be logically interpreted as TRUE and FALSE. Then you don't nee to worry about putting a typo in your comparisons such as if($var == 'Yes') Instead you can test the variable directly if($var) which will be true is $var is a 1 and false if $var is 0 -
OK, so you want TWO COMPLETELY DIFFERENT solutions. I don't see where you clearly stated that. All I see was a request to have the records ordered by data AND grouped by category. In any event, this is still a MySQL problem (moving to the MySQL forum). Request #1: Show posts in order of submission and include the Category name in the output. You will need to JOIN the posts table onto the Category table (assuming there is a separate table - which there should be). I already showed how to do that, but I added an order by Category due to the previous confusing request. You should be using something like this. BUt, this is only an example because I do not know your table/field names. $query = "SELECT p.Title, p.Author, p.content, p.date c.Category_ID, c.Category_Name FROM posts AS p JOIN categories AS c ON p.Category_ID = c.Category_ID ORDER BY p.date DESC"; Request #2: Show all the posts for a specific category. For this you will want to build a page that takes an optional parameter on the query string: e.g. show_category.php?catID=3. Then on the script you will use that category ID to get all the posts associated with that category. Here is an example of the query to use $catID = intval($_GET['catID']); $query = "SELECT p.Title, p.Author, p.content, p.date c.Category_ID, c.Category_Name FROM posts AS p JOIN categories AS c ON p.Category_ID = c.Category_ID ORDER BY p.date DESC WHERE c.Category_ID = '$catID'";
-
Login Password Encryption Problem
Psycho replied to Hazukiy's topic in PHP Installation and Configuration
I missed that he was using that in his MySQL query. But, the problem is still the same - the password being stores and the password being compered were two different values. Need to use the SAME hash process for both. -
Um,yeah. Your explanation is very confusing. You state you want the most recent post first, but that you want them sorted by category as well. That doesn't make sense. So, let's create a scenario: Post 1, Time 8:00AM, Category A Post 2, Time 9:00AM, Category B Post 3, Time 10:00AM, Category B Post 4, Time 11:00AM, Category A How do you want those displayed?
-
And, again, where is your code?
-
Login Password Encryption Problem
Psycho replied to Hazukiy's topic in PHP Installation and Configuration
You stated previously that you were hashing the value before storing it in the database. According to that code you are not hashing the password before you store it in the database. You should create a function to do your hashing. Use that function to hash the password before you store it. Then when the user logs in, use the same function to hash thier input value and compare that against what is in the database. -
Here is an example script that has both the JavaScript and the PHP code to implement the client-side logic and the server-side verification. To test the server-side logic you would need to to one of three things: 1. Turn off javascript in your browser so you can select more than 12 options 2. Remove the onclick even in the checkboxes 3. In the limitCheckboxes() javascript function put a "return;" on the very first lime. This will bypass the Javascript function and allow you to select more than 12 options for the purpose of testing. I put such a line into the function (which is commented out) and you can uncomment it to test. <?php error_reporting(E_ALL); $maxVoteCount = 12; //If form was posted verify number of checked items $result = ""; if($_SERVER['REQUEST_METHOD'] == "POST") { if(isset($_POST['vote']) && count($_POST['vote']) > $maxVoteCount) { $result = "You checked too many items. Bad Job."; } else { $result = "You did not check too many options. Good Job."; } } //Dynamically create input fields $inputFields = ""; for($i = 1; $i <=20; $i++) { $inputFields .= "<input type='checkbox' name='vote[]' value='{$i}' id='{$i}' onclick='limitCheckboxes(this.name, {$maxVoteCount})'> Option {$i}<br>\n"; } ?> <html> <head> <script type="text/javascript"> function limitCheckboxes(groupName, maxCount) { //Uncomment next line to test PHP code for verifying max count //return; var checkboxGroup = document.getElementsByName(groupName); var checkedCount = 0; for(i=0; i<checkboxGroup.length; i++) { checkedCount += checkboxGroup[i].checked; } //Set disable state based upon number checked var disabledState = (checkedCount >= maxCount); for(i=0; i<checkboxGroup.length; i++) { //disable/enable the unchecked boxes if(!checkboxGroup[i].checked) { checkboxGroup[i].disabled = disabledState; } } //Enable/disable submit button document.getElementById('submit').disabled = disabledState; return false; } </script> </head> <body> <?php echo $result; ?> <br><br> Select up to 12 options: <br><br> <form action="" method="post"> <?php echo $inputFields; ?> <br> <button type="submit" id="submit">Submit</button> </form> </body> </html>
-
So, you have multiple checkbox options for the user to choose from (at least 20) but you want to limit the user to only selecting up to 12, correct? The best solution for this, in my opinion is to 1) Implement JavaScript to disable the checkboxes (or provide some type of user response) once 12 options are selected. But, you must also 2) implement code on the back-end page that receives the form submission to verify that there were no more than 12 options selected.
-
Hmm, you say you want the newest posts first, but that you also want them sorted by category. I'm not sure how you mean for that to happen. If the newest post has a category of A and the second newest post has a category of B, do you want all the category A posts shown before the category B post? This is really a MySQL question and not a PHP question. Also, you haven't stated what your DB structure is. I will assume categories are defined in a related table. The below only groups by category and doesn't do anything regarding the newest posts because I'm not sure what you really want. <?php include('includes/connect.php'); function getPosts() { $query = "SELECT p.Title, p.Author, p.content, c.Category_ID, c.Category_Name FROM posts AS p JOIN categories AS c ON p.Category_ID = c.Category_ID ORDER BY Category_Name"; $result = mysql_query($query) or die(mysql_error()); while($post = mysql_fetch_assoc($result)) { echo "<h2>{$post['Title']} af {$post['Author']} Katagori {$post['Category_ID']}</h2>{$post['Content']}\n"; } } ?>
-
Login Password Encryption Problem
Psycho replied to Hazukiy's topic in PHP Installation and Configuration
OK, your code had some inefficiencies. For example, you had two instances of the form. You should only have one form and design it for multiple uses (e.g. with and without errors). Additionally, you should have a common method of displaying the errors to make your code more flexible (i.e. not one type of error at top and a different one at bottom). Here is a rewrite that is in a more logical format <?php session_start(); // dBase file include "dbConfig.php"; $errMsg = ""; //Check if user was brought here due to error if ($_GET["op"] == "fail") { $errMsg = "You need to be logged in to access the members area!"; } //Check if Login form was submitted if ($_SERVER['REQUEST_METHOD'] == "POST") { //Pre-process input data $username = trim($_POST["username"]); $password = trim($_POST["password"]); //Verify fields were entered if (empty($username) || empty($password)) { $errMsg = "You need to provide a username and password."; } else { // Create query $usernameSQL = mysql_real_escape_string($username); $passwordSQL = MD5($_POST["password"]); ## Hash password same as it was for the DB value //ONE query for data needed - in this case ID $q = "SELECT id FROM `TABLENAMEHERE` WHERE `username`='{$usernameSQL}' AND `password`='{$passwordSQL}' LIMIT 1"; // Run query $r = mysql_query($q); if(!$r) { //Error running query $errMsg = "There was a problem verifying your credentials. If problem persists contact administrator!"; //Uncomment next line for debugging purposes //echo "Query: {$q}<br>Error: " . mysql_error(); } elseif(!mysql_num_rows($r)) { //User not found $errMsg = "Sorry, couldn't log you in. Wrong login information."; } else { // Login good, create session variables and redirect $_SESSION["valid_id"] = $obj->id; $_SESSION["valid_user"] = $username; $_SESSION["valid_time"] = time(); // Redirect to member page header("Location: members.php"); } } } ?> <h1 class='FailLoginState'><?php echo $errMsg; ?></h1> <form action="?op=login" method="POST"> Username:<br> <input class="InputForm" type="text" name="username" id="username" value="<?php echo htmlentities($usernameSQL); ?>"> <br><br> Password:<br> <input class="InputForm" type="password" name="password" id="password"> <br><br> <button type="submit" name="submit" class="InputButton" value="Login">Submit</button> </form> -
Login Password Encryption Problem
Psycho replied to Hazukiy's topic in PHP Installation and Configuration
You need to do the same hash to the password that the user enters and THEN compare that to the value in the database. Just using MD5() is a very poor implementation. But, get your script working then you can implement a better hashing method. -
As we've stated the error is NOT that line. The problem is in how $p['cast'] is defined - or probably not defined in this case. So, unless you know where that it is, it may take someone who understands PHP to analyze the code to figure out where it is supposed to be defined and trace back what that problem is.
-
Your question is a littlee confusing because the answer seems painfully obvious. If you have a value from the database, just set the width to what you want. I have no idea how you plan to set the width based upon the value from the database. Do you want the value to be set to the actual value from the database or calculated based on that DB value? Also, you can set the value in the actual HTML or within the Style sheet. <table style="width: <?php echo $valueFromDB; ?>px">
-
"Believing" something is true and "knowing" are two different things. It is apparent that it is NOT an array - else you would not be getting the error. You can very easily verify that it is or is not an array using the function is_array(). if(is_array($p['cast'])){ echo "it's an array"; } else { echo "it's NOT an array"; } But, we already know it is not an array. So, you might as well look to see where it is defined.
-
Well, per the documentation for implode() there are two parameters (note: it can also take a single parameter, but that's not commonly used): Your first parameter is obviously a string. So,the problem has to be the second parameter: $p['cast']. Have you verified the contents of that variable and ensured it is, in fact, an array?
-
Displaying Column in One Table Based on Another Table
Psycho replied to loren646's topic in MySQL Help
Ahh, so you are getting an error. That would have been helpful information with your initial post. That error means that your query is failing - and the result ($result1) is false. You need to check what the error is. Change the following line as shown $query_result1 = mysql_query($category1) or die (mysql_error()); However, I can see that the query is "off" in that you have the same condition for the JOIN as you do in the WHERE clause. That makes no sense. Start by removing the WHERE condition. Or change it to something like you had before: $catergory1 = "SELECT l.landlord, l.building, l.location FROM land as l INNER JOIN updater as u ON l.landlord = u.addbuilding WHERE l.landlord='$landlordselect'"; -
Displaying Column in One Table Based on Another Table
Psycho replied to loren646's topic in MySQL Help
And what is the problem? -
Why do you need multiple sessions?