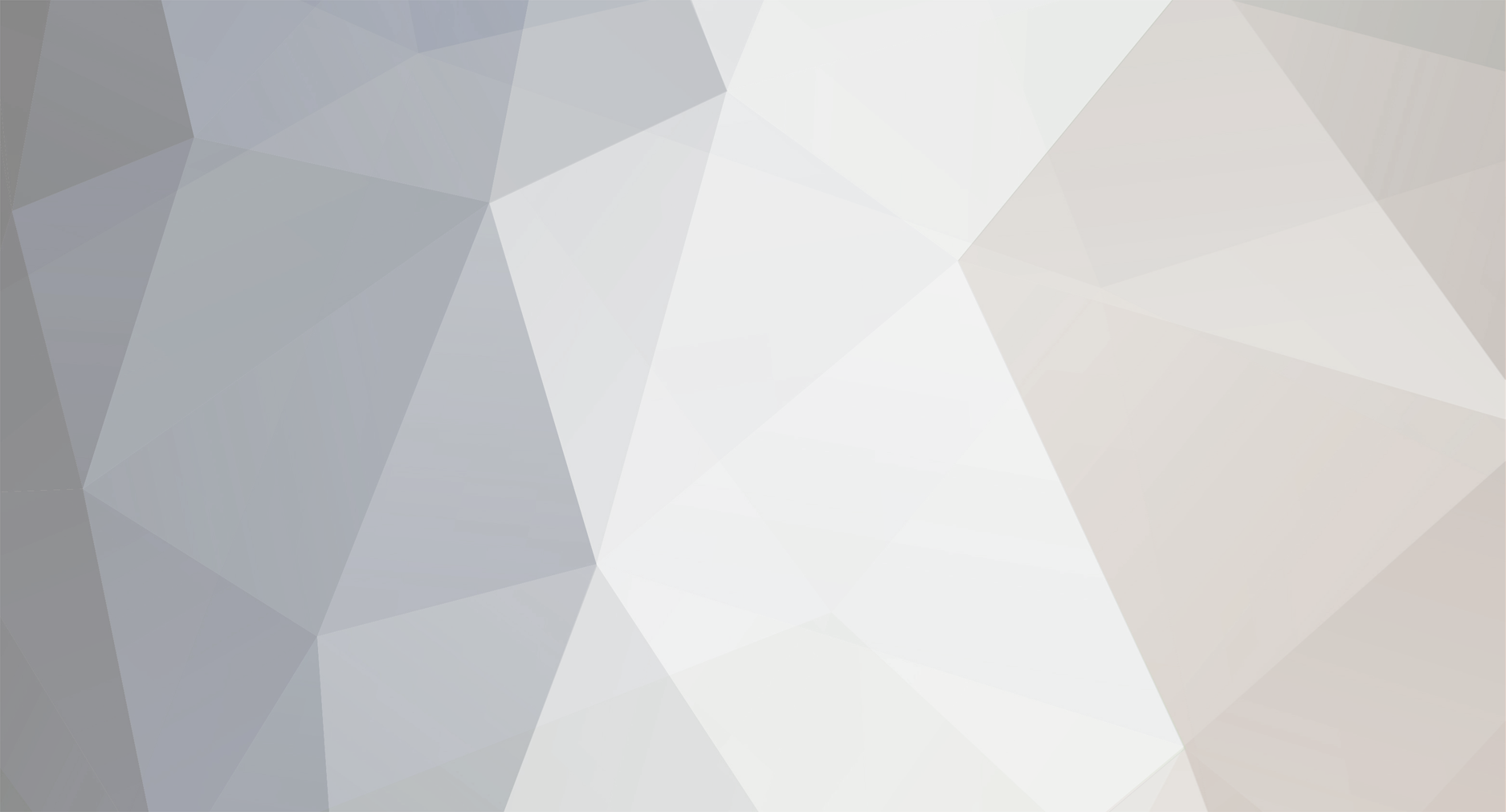
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Your question kind of changed direction midway. For the LOGIN process you would likely use one table. As part of the login process, once the user is successfully logged in, you would want to store something in the session information to identify that the user is logged in. Typically this just requires the user ID. Then, on every page that requires the user to be logged in you could add this at the top of the page: if(!isset($_SESSION['user_id'])) { include("login.php"); exit(); } As for the actual applications/features, yes you would want multiple tables based upon the data to be stored.
-
STOP 1. Your script has many more problems aside from this issue. You really need to go back and rewrite it to improve efficiency. And stripping characters out of the password?! Ridiculous, you are only reducing the security of their password and creating a security hole. 2. You have a simple problem to debug. You've already verified that $_POST contains the value that you expect. The problem you have is that the check of if(!$email) is returning false. So, do a check at each step where that variable is created and changed. Then you will see EXACTLY where the problem lies echo "Email Post: {$_POST['email']}<br>\n"; $email = stripslashes($_POST['email']); echo "Email after stripslashes: {$email}<br>\n"; $email = strip_tags($email); echo "Email after striptags: {$email}<br>\n"; $email = mysql_real_escape_string($email); echo "Email after mysql_real_escape_string: {$email}<br>\n";
-
Then it doesn't work. PHP code is executed server-side, Javascript is executed client-side. I suppose you could do it with an applet or some other client-side plug-in that would require the user to install - but that's worse than relying on Javascript. Using Javascript to add functionality such as this is the best approach. But, if you want your application to also work without Javascript then you need to build it in such a way that the page will work either way: e.g. unobtrusive Javascript. In this approach you build the page first to work without Javascript. Then you add Javascript on top of that code so that if JS is disabled it will gracefully fall back to the version that will work without JS. In this case you build your form such that the user has to make a selection using the dropdown and then click a submit button. Then you do two things. 1) And an onchange event to the drop-down to auto-submit the form. 2) Add an onload event to the page to remove/hide the submit button. The result is that if JS is enabled the submit button won't display and when the user selects a dropdown value the page automatically submits. If the user does not have JS enabled then the user can select a value and then click the submit button.
-
Yes, with Javascript.
-
You shouldn't put something such as (count($data)-2) as the comparison within a for loop. That makes the server do a calculation on each iteration of the loop. Better to set a variable using count() before the loop. In any case, I think this is more efficient. foreach(file('file.txt') as $idx => $line) { echo (($idx) ? "$idx " : '') . $line . "<br>\n"; }
-
show if date is before and total is less than
Psycho replied to petattlebaum's topic in PHP Coding Help
How is your data for the workshops stored? If this is in the database, then you should implement the functionality in your query. If not in a database, then (based upon your code above) it seems you would be repeating the logic over and over for each set of fields. You should create a loop for this. if data is in a database, then only pull records for the workshops matching your condition. Note this is just an example since I would have no idea what your table structures are SELECT list, of, fields, needed, SUM(reservations.attendees) AS total_reservations FROM workshops LEFT JOIN reservations ON workshops.id = reservations.workshop_id GROUP BY workshops.id WHERE workshops.date < NOW() AND total_reservations < 25 If you have the data stored another way please share that info to get a good solution. -
Possible? want one combobox to populate another combo box
Psycho replied to loren646's topic in PHP Coding Help
If the lists of total landlords and total properties is "reasonable" then you can accomplish it with just javascript by writing all the data as Javascript variables into the page. But, yeah, if you want this to happen without doing a page refresh then you have to do it with javascript and the only question is if you are going to make a trip back to the server (AJAX) or do it completely client side. -
That error is either a problem with the web server or their could be some significant error in the script that is causing the web server to choke. But,the script is a complete mess - so I'm not going to fix it. 1. Why do you define a bunch of variables as TRUE and then have if() condition checks later to output content. Create a separate script to output the content based upon those variables. Then, for your pages, define the variables and include that script. 2. You have a lot of code after the closing body tag (</body>) which outputs more content to the page. 3. At the very end you have this header("Location:asd.php"); You cannot send headers to the page after you have sent any content to the browser. This may be why you are getting the error above.
-
<?php $query = "SELECT * FROM log WHERE DateOpened BETWEEN $date_1 AND $date_2 ORDER DateOpened DESC"; $result = mysql_query($query); if(!$result) { //There was a database error echo "Query: $query<br>Error: " . mysql_error(); } elseif(!mysql_num_rows($result) { echo "There were no results"; } else { while($row = mysql_fetch_assoc($result)) { //Do something with the data } } ?>
-
What does " . . . will not run the search" mean? Are you getting errors? Are you even checking for errors (and the answer is no)? Why are you using > and < comparisons when you asked for help and were given examples to use the BETWEEN operator? Is the date field int he database an appropriate "type" for a date value: e.g. date, datetime, or timestamp? What are the values of $date_1 and $date_2? Also, you would always get NO results if $_GET['DateOpened'] is empty based on that logic because you have the where clause as WHERE 1 = 2 What is the purpose of that? If you don't want to run the query - then don't run it. Don't create a query that you know will never return results.
-
$query = "SELECT * FROM Log WHERE Date_Opened BETWEEN '{$Date_1}' AND '{$Date_2}' ORDER BY DateOpened DESC ";
-
Probably because there is no such thing as a wildcard character when using in_array(). Either build your own function to do a search using a wildcard character of your choosing - or put the values into a database.
-
A little explanation of what the relationship between tables is would be helpful. I don't know if there can be multiple associated records in the game table for each user. But, it seems you are wanting to find the users that have NO associated records in the game table. If so, then use this: SELECT users.id FROM users LEFT JOIN game ON users.id == game.id WHERE users.computer_player IS NULL
-
I never said remove ALL of them. I only stated you should use the correct process based upon the data type. For fields that are text you should use mysql_real_escape string(), For integer fields you should use intval() or something similar. For date fields you should do something to verify the string is an acceptable date, etc. I'm really not following you. You want to take the data from the XML field and insert/update your current records, correct? Then you want to add data for two other fields ("remarks" and "afk")? Do you want to do that as part of the INSERT/UPDATE process or after? There were no such fields in your script above, so that script would do nothing to overwrite any existing data in those fields unless you modified the query to do so.
-
Date / Time difference using only working hours
Psycho replied to Clarkey's topic in PHP Coding Help
Here's my attempt. I've tested it a little and it seems to work correctly. It looks like a lot of code, but I added a lot of validation in the code. For example, if the start date is after the end date then a false will be returned. A couple notes: - If the start and end go over a weekend, no time is allocated for Sat & Sun - Holidays are supported (i.e. time will not be allocated for holidays), but you will need to provide an array of holidays to pass to the function workDays(). There are two functions: workTime() and wordDays(). workTime() takes four parameters: the start time and end time in a string format. It optionally takes a begin and end valid times in a float format (so if the work day starts at 8:15 am the value should be 8.25). The function will calculate the total seconds between the start and end date/times. If the two are on the same date then that difference in seconds is returned. If the dates are different, then the function workDays() is called to count the number of work days that the period falls on - weekends and holidays are excluded in that calculation. 2 is subtracted from that result to get the number of days where the full time should be applied. Then the function workTime() will calculate the time on the first partial day, the full workdays, and the last partial day. I'm sure there are a few things I would improve on - especially from an efficiency perspective. Also, if a workday can go through the night this might have problems for days where the clocks adjust for daylight savings time. This is a fully working script with an form for testing. Enjoy: <?php error_reporting(E_ALL); //Set defaults for form fields $startStr = '2013-02-18 16:30:00'; $endStr = '2013-02-19 09:55:00'; if(isset($_POST['start']) && isset($_POST['end'])) { //Set vars to repopulate form fields $startStr = $_POST['start']; $endStr = $_POST['end']; $totalTime = workTime($_POST['start'], $_POST['end']); echo "Start: " . date('l, F j, Y H:i:s', strtotime($_POST['start'])) . "<br>\n"; echo "End: " . date('l, F j, Y H:i:s', strtotime($_POST['end'])) . "<br>\n"; $hours = floor($totalTime / 3600); $minutes = floor(($totalTime-($hours*3600)) / 60); echo "Total time: $hours:" . str_pad($minutes, 2, '0', STR_PAD_LEFT); } function workDays($startTS, $endTS, $holidays=array()) { $dayTS = strtotime('12:00:00', $startTS); //Set to noon to avoid daylight savings problems $endDateStr = date('Y-m-d', $endTS); $workDays = 1; while(date('Y-m-d', $dayTS) != $endDateStr) { //If day not a weekend or holiday add 1 if(date('N', $dayTS)<6 && !in_array(date('Y-m-d', $dayTS), $holidays)) { $workDays++; } $dayTS = strtotime('+1 day', $dayTS); } return $workDays; } function workTime($startStr, $endStr, $validStartFloat='9.0', $validEndFloat='17.0') { $startTS = strtotime($startStr); $endTS = strtotime($endStr); //Verify end time comes after start time if($startTS > $endTS) { return false; } //Verify start and end times are within valid work times $startFloat = floatval(date('G', $startTS) + (date('i', $startTS)/60)); $endFloat = floatval(date('G', $endTS) + (date('i', $endTS) / 60)); if($startFloat<$validStartFloat || $startFloat>$validEndFloat) { return false; } if($endFloat<$validStartFloat || $endFloat>$validEndFloat) { return false; } if(date('Y-m-d', $startTS) == date('Y-m-d', $endTS)) { //Start and end are on same day $work_seconds = $endTS - $startTS; } else { //Start and end are on different days $endOfFirstDaySec = ($validEndFloat - $startFloat) * 3600; $begOfLastDaySec = ($endFloat - $validStartFloat) * 3600; $fullWorkDays = workDays($startTS, $endTS) - 2; $fullWorkDaysSec = $fullWorkDays * ($validEndFloat-$validStartFloat) * 3600; $work_seconds = $endOfFirstDaySec + $fullWorkDaysSec + $begOfLastDaySec; } return $work_seconds; } ?> <html> <head> </head> <body> <br><br> <form action="" method="post"> Start: <input type="text" name="start" value="<?php echo $startStr; ?>"><br> End: <input type="text" name="end" value="<?php echo $endStr; ?>"><br> <button type="submit">Submit</button> </form> </body> </html> -
Date / Time difference using only working hours
Psycho replied to Clarkey's topic in PHP Coding Help
A few questions/comments: I think an easier approach would be to just calculate the total seconds between the start and end and then subtract any time needed if the start and and are on different days. Also, how do you need to account for weekends? If the start is on a Friday and the end is on a Monday do you count time for Saturday and Sunday? -
@kicken: I think the issue is in 'how' to implement that after 40 seconds. Well, there are a few options. This is the easiest in my opinion is this: You apparently only want it to run 4 times since each interval is set to 10 seconds. So, set a global counter that is used inside loadUpdateLevelPercentage(). Then at the end of each iteration of the function, increment the counter and do a check to see how many times it has executed. If >= 4 then use clearInterval()
-
Never, ever run queries in loops. Here is what you should be doing: 1. Add a new field to the table called "last_modified" 2. Iterate over the records in the XML file and create the INSERT values. Before the loop create a timestamp for the last modified date that will be included in the last_modified field 3. Run ONE query to INSERT all the records using the "ON DUPLICATE KEY UPDATE" 4. After you INSERT/UPDATE all the records, run ONE query to DELETE all the records that have a last_modified < (less than) the timestamp created before the loop. A few other comments. Why are you using mysql_real_escape_string() on fields such as 'startDateTime', 'baseID', etc.? You shoudl verify/format those values as appropriate for the data type. mysql_real_escape_string() is for STRING data. I didn't make any changes for this in the same code below because I don't know the data types for all of them. I see in a couple instances you appear to be inserting an ID and a value for a couple things (Base, ship, location). You should insert only the ID and then save the id/name to another table. This is just an example - I don't have your database or input data. <?php // INCLUDE DB CONNECTION FILE include("includes/connect.php"); // CHANGE THE VALUES HERE include("includes/config.php"); // URL FOR XML DATA $url = "test.xml"; // For Testing Purposes // RUN XML DATA READY FOR INSERT $xml = simplexml_load_file($url); // Loop Through Names $insertValues = array(); $modifiedTS = date('Y-m-d h:i:s'); foreach ($xml->result->rowset[0] as $value) { //Prepare the values $characterID = mysql_real_escape_string($value['characterID']); $name = mysql_real_escape_string($value['name']); $startDateTime = mysql_real_escape_string($value['startDateTime']); $baseID = mysql_real_escape_string($value['baseID']); $base = mysql_real_escape_string($value['base']); $title = mysql_real_escape_stfdsg($value['title']); $logonDateTime = mysql_real_escape_string($value['logonDateTime']); $logoffDateTime = mysql_real_escape_string($value['logoffDateTime']); $locationID = mysql_real_escape_string($value['locationID']); $location = mysql_real_escape_string($value['location']); $shipTypeID = mysql_real_escape_string($value['shipTypeID']); $shipType = mysql_real_escape_string($value['shipType']); $roles = mysql_real_escape_string($value['roles']); $grantableRoles = mysql_real_escape_string($value['grantableRoles']); //Create INSERT value statement $insertvalues[] = "('$characterID', '$name', '$startDateTime', '$baseID', '$base', '$title', '$logonDateTime', '$logoffDateTime', '$locationID', '$location', '$shipTypeID', '$shipType', '$roles', '$grantableRoles', '$modifiedTS')"; }; //Create and run ONE INSERT statement (with UPDATE clause) $query "INSERT INTO `ecmt_memberlist` (characterID name, startDateTime, baseID, base, title, logonDateTime, logoffDateTime, locationID, location, shipTypeID, shipType, roles, grantableRoles, last_modified) VALUES " . implode(', ', $insertvalues) . " ON DUPLICATE KEY UPDATE name = '$name', startDateTime = '$startDateTime', baseID = '$baseID', base = '$base', title = '$title', logonDateTime = '$logonDateTime', logoffDateTime = '$logoffDateTime', locationID = '$locationID', location = '$location', shipTypeID = '$shipTypeID', shipType = '$shipType', roles = '$roles', grantableRoles = '$grantableRoles' last_modified = '$modifiedTS'"; mysql_query($result) or die(mysql_error()); //Create and run ONE query to delete records that were not just inserted/updated $query = "DELETE FROM `ecmt_memberlist` WHERE last_modified < '$modifiedTS'"; mysql_query($result) or die(mysql_error()); ?>
-
No, that would not be the case. In the bits of code above you have not applied any styles to the input fields. So, I must assume you are trying to apply those styles through Javascript. You do have one class, "search", which is applied to the form element - and that makes no sense. The "form" is not a visual element that is displayed. If you mean for those properties to be applied to the INPUT elements of the form - then you need to specify that. #search input { width:50%; padding:10px; outline:none; height:36px; }
-
Hidden fields are "hidden" - i.e. they are not shown. Therefore you can't style them. They never get focus either. What you are asking makes no sense. If you want a field that is displayed, but not editable - then you don't want to use a hidden field.
-
Many (most) of the time when you get that type of error message, the actual error is on a previous line. It's just not till the line in question that the PHP parser can be sure that an error exists. For example, if you open a new string and for get to close it, the PHP parser will think that all the following lines of code are part of the string - until it hits another quote mark. In this case your error is here "do".__FILE__ => $configArr['do".__FILE__], Do you see the problem? EDIT: By the way, why are you creating an array key with a dynamic value? How would you know what key to reference in the resulting array?
-
Returning the Correct ID with column groups and...
Psycho replied to AnalyzeThis's topic in MySQL Help
You have logic such that IF(`tanning_courses`.`name` LIKE '%VEGITABLE%', DATE_ADD(max(`date`),INTERVAL 10 YEAR) Are there different courses with the name 'VEGITABLE' in them? You state That won't work since a user *could* take two courses of the same type. Your GROUP BY is only based upon the user and the course name. -
Returning the Correct ID with column groups and...
Psycho replied to AnalyzeThis's topic in MySQL Help
You need to provide details regarding your table structures and the relevant fields used as foreign keys -
Some more things to improve 1. Don't use SELECT * when you only need specific columns 2. You have at least one table where you are defining a date in PHP and then using that in the INSERT query. You should instead create that date field as a timestamp with a default value of NOW(). Then you don't need to include the date field in your INSERT query at all - it will be handled automatically.
-
how to terminate everything and only show the error message
Psycho replied to et4891's topic in PHP Coding Help
It doesn't absolutely NEED to be, but it is good programming standard. When developing code you should turn on error reporting to E_ALL (see the manual on how to do this). As you know when there are critical errors the PHP parser will display an applicable error. But, there are also less critical errors (warnings) which don't normally get displayed. But, by turning up the error reporting you will see them. In this case, I define $selectOptions before using it - otherwise it would generate a warning. That .= is used to concatenate a string to an existing string. If you just used = then you would be overwriting the previous value with the new value. It doesn't NEED it, but it is the standard I prefer. You can put variables within strings that are defined with double quotes and the string will be interpreted. In a single quote string the variable is treated as a literal string $color = 'blue'; echo 'The car is $color'; //Output: The car is $color echo "The car is $color"; //Output: The car is blue however, if certain characters are next to the variable in the string the PHP parser can get confused. But, putting the {} brackets around the variable name it tells the PHP where the beginning and end of the variable is. plus, in some development IDE's it allows the variable to be color coded in the string. $amount = '15,990'; echo 'The car is $$amount'; //Output: The car is echo "The car is ${$amount}"; //Output: The car is $15,990 [/code] The first one shows not value for amount because the double dollar signs makes PHP look for a variable variable - i.e. a variable named as $15,990 - which is invalid