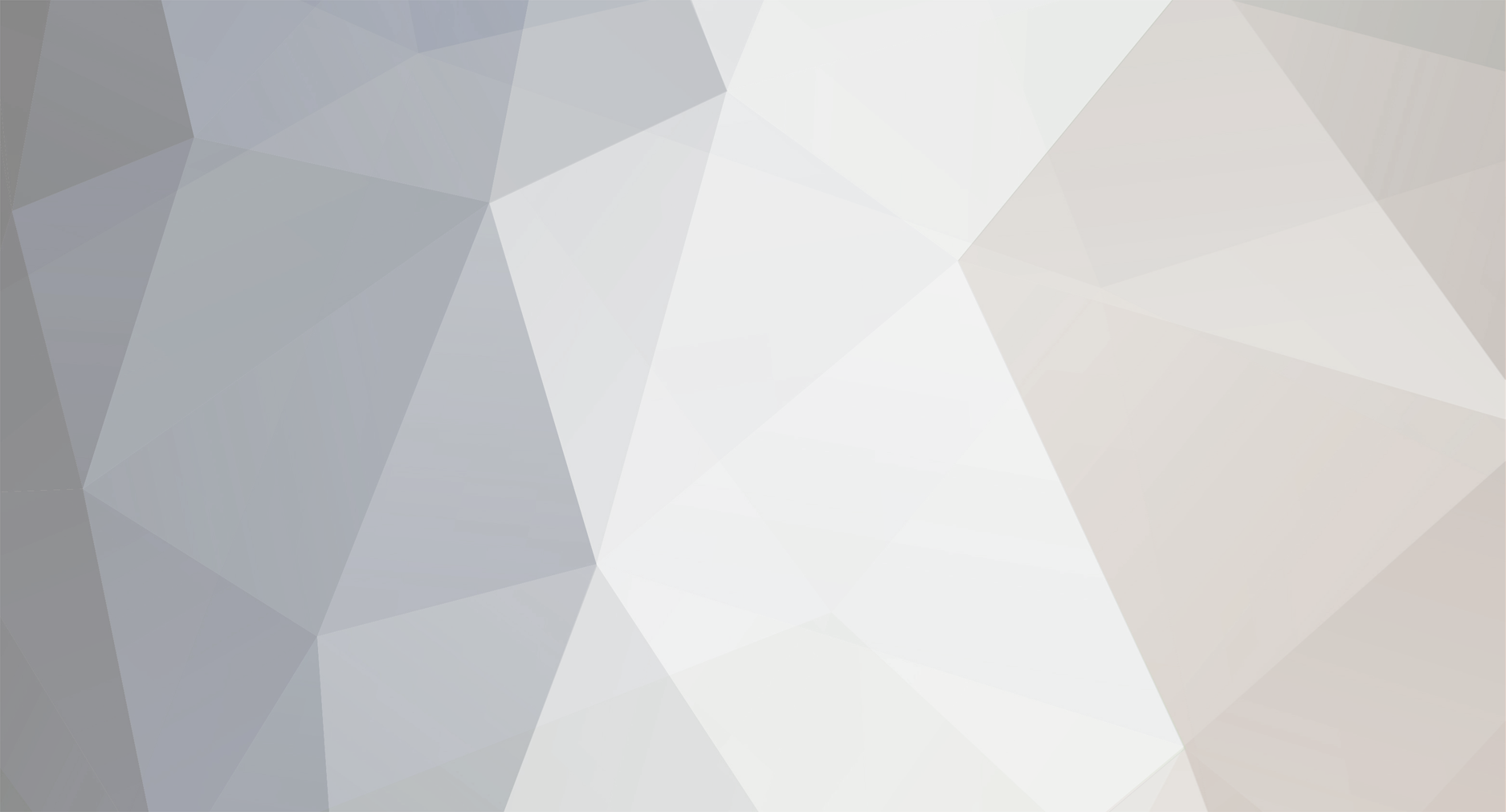
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
how to terminate everything and only show the error message
Psycho replied to et4891's topic in PHP Coding Help
$directory = "./aaa"; if(!(is_dir($directory))) { die("Must create a <strong>aaa</strong> folder first, sorry"); } $aaa_files = scandir($directory); $selectOptions = ''; foreach($aaa_files as $file) { if(($file == ".") || ($file == "..")) { continue; } $file = strtolower($file); if(strlen($file)<5 || substr($file, -4) == '.txt') { continue; } $name = basename($file, '.txt'); $selectOptions .= "<option value=\"{$name}\">{$name}</option>\n"; } if(empty($selectOptions)) { echo "Must create text files first, sorry"; } else { echo "<form action=\"./page2.php\" method=\"get\">"; echo "<select name=\"aaa\">"; echo $selectOptions; echo "</select>"; echo "<br/><input type=\"submit\">"; echo "</form>"; } -
Well, one question I have is why are you using a while() loop to get the result from the query? You are apparently only expecting one result so a while loop() is unnecessary and unintuitive. But, your problem is simple. Here is what the manual states about mysqli_fetch_row() In other words it makes the keys of the array 0, 1, 2, etc. I never use fetch_row because of that. Use mysql-_fetch_assoc() instead so the keys of the array are the names of the fields in the query - as you apparently expect them to be. By the way, you could have verified this very easily by doing some simple debugging such as using print_r() or var_dump() on $retrieve
-
This query will update all the values for the column 'hot_job' to be a 1 or 0 based upon whether the current value is "YES" or "NO", respectively. After running that, you should change the field type to an INT. UPDATE jobs SET hot_job = (hot_job = 'YES') When creating your form, you want to create the fields as an array (use [] at the end of the field name) using the ID of the record as the value. <?php $formFields = ''; while ($row = mysql_fetch_array($sql)) { $id = $row['id']; $checked = ($row['hot_job']) ? ' checked="checked"' : ''; $formFields .= "<tr>\n"; $formFields .= " <td><strong>{$id}</strong></td>\n"; $formFields .= " <td><input type=\"checkbox\" name=\"hot_job[]\" id=\"ONOFF{$id}\" value=\"{$id}\" {$checked} /></td>\n"; $formFields .= "</tr>\n"; ?> <form name='hotbox' action='hot_update.php' method='POST'> <table> <?php echo $formField; ?> <button type="select">Submit change</button> </form> To process you can take all the submitted values (the Record IDs) and create a single query to update the entire table if(isset($_POST['hot_job'])) { //Convert all values to integers and remove invalid values $hotJobIDs = array_filter(array_map('intval', $_POST['hot_job'])); //The var $hotJobIDs will be an array of all the records that should be set to 1 //All other records should be set to 0 //Create ONE query to update the table $hotJobsIDsList = implode(', ', $hotJobIDs); $query = "UPDATE jobs SET hot_jobs = (id IN ($hotJobsIDsList))"; $result = mysql_query($query); //The following lines for debugging only if(!$result) { echo "Query Failed<br><br>Query: $query<br><br>Error: " . mysql_error(); } }
-
I see lots of problems: First of all you should change the values you are storing to 1 or 0 instead of "YES" or "NO". A 1 or 0 can be logically interpreted as Boolean TRUE/FALSE. Second, you should NOT be running queries in loops. Create one query to update the values. Third, there is no error handling on your query - so if there are errors you will never see them Fourth, you appear to be opening a new form for each checkbox - but never closing the form. If you have a closing form tag later in your script you would have only one valid for that would include only the last checkbox. Fifth, you are not creating the form fields as an array, but your processing code is trying to process them as an array Sixth, the values in your DB are YES or NO, but you are trying to update the value to 0 or 1. I'm sure there are other problems I do not see. There is a VERY easy way to do this - will respond momentarily with some code
-
That is something I would never do. I never use something such as if($var) { //do something if $var is an 'unknown' quantity - i.e. I cannot be sure if it exists or what value it may contain. This is along the lines of what I would typically do: $foo = (isset($_POST['foo'])) ? trim($_POST['foo']) : ''; if(!empty($foo)) { //Do something } But, that would not work if a user can submit an empty value and I want to perform the "Do something" process on it as well. In that case I might use $foo = (isset($_POST['foo'])) ? trim($_POST['foo']) : false; if($foo !== false) { //Do something } It all depends on understanding what the possible inputs can be (or not be) and building the functionality accordingly.
-
Yes and No. You are correct that isset() will return false in that condition. But, it is not because $var is not set - it is. In that scenario $var is set to a value of NULL. I forgot that isset() checks to You can verify that $var is set by turning on error reporting to E_ALL and doing an echo $var; which will not report an Undefined Variable warning.
-
Hmm. . . if you are just going to set $dimension on each loop - no need to use the ternary operator and make the PHP processor do more work by doing a comparison check. Just set the value to 200 before the loop and to 100 at the end of each iteration. $query = "SELECT image_location AS src FROM size_chart WHERE prod_size_id = $GET_ID"; $result = mysql_query($query); $size = '200'; //Set size for first iteration while($row = mysql_fetch_assoc($getSize)) { echo "<a href=\"{$row['src']}\"><img src=\"{$row['src']}\" width=\"{$size}px\" height=\"{$size}px\" /></a>\n"; $size = 100; //Set size for subsequent iterations }
-
The problem is your question only states that IF(isset($var)) is having problems and you want to know what is a better replacement. You need to state exactly what you are trying to verify. isset() has been around since PHP4 and has had only 1 update Which is really an edge case scenario in my opinion and wouldn't cause it to start behaving differently for you. Most likely, some logic has changed such that the condition is not returning the same results as before because the preconditions have changed. So, you need to determent what it is you want to verify. Are you wanting to know if the variable is set, if it is empty, if it is FALSE, or what? FYI: I see a lot of people do something like this $var = $_POST['foo']; if(isset($var)) { //Do something } which will always result to true because $var was set immediately before the condition check.
-
Join multiple tables and group multiple columns to the same alias
Psycho replied to Roland_D's topic in MySQL Help
You could also use an IF() statement in the SELECT portion of the query SELECT m.ID, IF(m.pID<>0, p.title, c.name) AS myTitle FROM menu m LEFT JOIN pages AS p ON p.ID = m.pID LEFT JOIN categories AS c ON c.ID = m.cID -
I think you need to talk to your instructor. If we are simply telling you what code to use and where to put it you aren't learning anything.
-
<?php function outputDepartmentList($deptName, $userList) { if(!$deptName) { return false; } $userCount = count($users); $output = "{$currentDept} ({$userCount})<br>\n"; $output .= " implode("<br>\n", $users); return $output; } $query = "SELECT Department, Fname, Lname FROM table_name ORDER BY Dept, Lname"; $result = mysql_query($query) or die (mysql_error()); $currentDept = false; while($row = mysql_fetch_assoc($result)) { if($currentDept != $row['Dept']) { echo outputDepartmentList($currentDept, $users); $users = array(); $currentDept = $row['Dept']; } $users[] = ucfirst($row['Fname']) . ' ' . ucfirst($row['Lname']); } echo outputDepartmentList($currentDept, $users); ?>
-
On a side note, you have a lot of unnecessary code in there. You set $_SESSION['lang'] to a default of 0 to start. Then you have a if() condition that will set it to 0 if a condition is met. Why, you already set it to 0 to begin with right? Then you put a default case in your switch statement and also duplicate the code for the 1 condition. Anyway, that 0 or 1 values are a waste. It makes it more difficult to read in your code and is not intuitive. As to why you are not seeing the spanish content, my guess would be that you aren't actually sending 'span' on the query string as you think you are. Try echoing out $_GET['lang'] to the page. This code will work much better and I've included a couple debugging lines <?php session_start(); if(isset($_GET['lang'])) { $_SESSION['lang'] = strtolower(trim($_GET['lang'])); } ?> <center><a href="index.php?lang=eng"><img title="English" src="images/english.png"></a> <a href="index.php?lang=span"><img title="Spanish" src="images/spanish.png"></a></center> <?php //Debugging lines (Uncomment to debug) //echo "\$_GET['lang']: {$_GET['lang']}<br>"; //echo "\$_SESSION['lang']: {$_SESSION['lang']}<br>"; switch($_SESSION['lang']) { case 'span': include("NavMenuSpan.php"); break; case 'eng': default: include("NavMenuEng.php"); break; } ?>
-
sum of two row from 2 different table(is this correct)
Psycho replied to Taku's topic in PHP Coding Help
You never really provided an adequate explanation of your table structure - I thought you needed a GROUP BY. But, if every ID exists in all tables AND there is one record for each ID then use this: SELECT t1.id, t1.quantity AS qty1, t2.quantity AS qty2, t3.quantity AS qty3, t4.quantity AS qty4, (t1.quantity + t2.quantity + t3.quantity + t4.quantity) as total FROM table1 AS t1 INNER JOIN table2 AS t2 ON t2.id = t1.id INNER JOIN table3 AS t3 ON t3.id = t1.id INNER JOIN table4 AS t4 ON t4.id = t1.id -
sum of two row from 2 different table(is this correct)
Psycho replied to Taku's topic in PHP Coding Help
What does "and it seems not working" mean? Is it failing? Are you getting results but not what you expected, or what? -
I wouldn't think so, but I couldn't get it working otherwise. Perhaps Barand can provide the more efficient solution.
-
sum of two row from 2 different table(is this correct)
Psycho replied to Taku's topic in PHP Coding Help
If ALL ids exist in both tables then you can do the following to get the quantity totals for each ID from both tables in a single query as follows: SELECT t1.id, totalCafe1, totalCafe2, totalCafe1 + totalCafe2 as totalCafe FROM ( SELECT id, SUM(quantity) AS totalCafe1 FROM test_req_concepcion GROUP BY id ) t1 JOIN ( SELECT id, SUM(quantity) AS totalCafe2 FROM test_noval GROUP BY id ) t2 USING (id) That will provide a result with columns for: the ID, the total from the first table, the total from the second table, and the total from both tables. But, if either table may have an id that is not in the other table, those records won't be returned. If one table will absolutely have all the IDs and only the other table may be missing some IDs then that can be resolved by doing a LEFT/RIGHT join as needed. But, if both tables may be missing an ID that is in the other it gets a little more complicated. I'm not willing to do any more until you have clarified -
sum of two row from 2 different table(is this correct)
Psycho replied to Taku's topic in PHP Coding Help
OK, now I am more confused. You now state you want multiple rows to be returned but your queries are constructed to return only 1 row each. Are you wanting a separate row for each unique ID? So, ID | total1 | total2 | total 1 1 2 3 2 4 5 9 -
sum of two row from 2 different table(is this correct)
Psycho replied to Taku's topic in PHP Coding Help
Hmm . . . , that's not making sense to me. You have a POST variable called 'select' which I would think is used to select the appropriate records in your query. But, there is nothing in the query to filter the records. So, you would be selecting ALL the records from both tables. And, I doubt that query would run anyway. And, you know, it takes 2 seconds to drop a query into your database admin panel to test. Why would you take the trouble of asking "does this work" rather than testing it? Of course, if what you think might work doesn't then absolutely post here for help. But, when you simply ask "will this work" it appears you are just being lazy. Please provide some more information as to what is in the tables and what you need to get. -
Use the modulus operator: Use the modulus operator: for(i=0; i<length; i++) { if(i%2) { //Even code } else { //Odd code } } In case you are not familiar with modulus, it is the mathematical operator to get the remainder of a division. Do, the modulus of 11 divided by 3 would be 2: 11 / 3 = 3 (3 x 3 = 9) with a remainder of 2. So, the modulus of 2 as i increases will alternate between 1 and 0 (no remainder). Since 1 and 0 are interpreted as the logical true/false you can just use the result of the modulus in the if statement rather than if(i%2 == 1)
-
Why are you running two queries: 1 to get the count and another to delete. And the delete query is in a loop no less. Never run queries in loops. All you need is ONE query $query = "DELETE FROM tblTemp WHERE LocationID IN ( SELECT LocationID FROM (SELECT LocationID FROM tblTemp GROUP BY LocationID HAVING count(*) < $nchecked ) t )";
-
copying code from the windows clipboard
Psycho replied to help_me_with_php's topic in PHP Coding Help
Again, what "risk" are you asking about. You allude to their being a purpose behind the question but didn't provide any details. So you were a developer at one point? If so, I am really confused as to why you are even asking the question. The question would only make sense from someone who doesn't understand basic operations of a computer. If you are going to ask a question - especially one of such an odd nature - in a public forum you have to accept that people may provide responses that you weren't looking for. Besides, all too often we see people asking a question on how to perform some type of process which doesn't make sense. After we get more information we are able to identify that the person is trying to do something that is counter-productive to what they are really trying to achieve. I'm still trying to figure out what prompted such an odd question. -
Also, don't use LIKE in your query unless it is a search within a string - just use the equal operator. $sql = "SELECT * FROM $tbl_name WHERE (Colour = '$colourselect') AND (Pattern = '$patternselect') LIMIT $offset, $rowsperpage";
-
copying code from the windows clipboard
Psycho replied to help_me_with_php's topic in PHP Coding Help
Risk of what? You are simply copying text from one application to another. -
Well, first of all you should NOT use an ENUM type if the values are Yes/No (i.e. TRUE/FALSE). Use a Boolean (or Tiny INT) type and store a 1 or 0. If you store a string such as "Yes" or "no" then you have to add additional logic to your code to process, such as if($active=='yes') { //Do something } instead, if you use 1 or 0 you can use those values directly because they are interpreted as logical TRUE and FALSE if($active) { //Do something } But, the reason you are getting the error is probably because you have some text type field (possibly the ENUM) for which you have not supplied a length.
-
Then that will be more difficult to implement. Using just spaces to determine words would be very close. If your intent is just to get the content broken out into pieces that are relatively 1,000 words I would think that would suffice. But, if you want something that will split into exactly 1,000 words based upon specific requirements you will have more work to do. I guess preg_split() using space or line break for the split expression would be a good option.