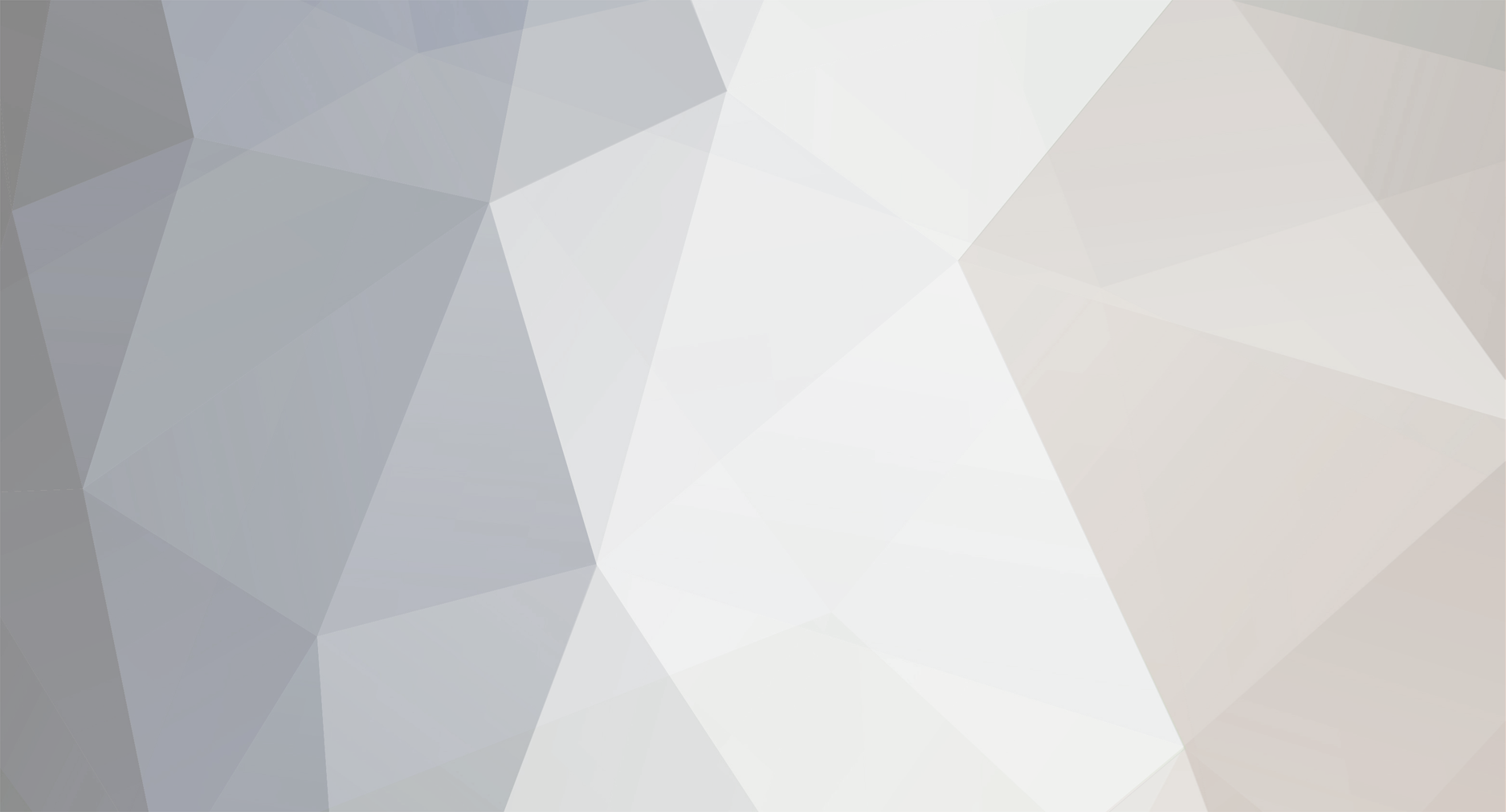
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Php To Select Different Column For Option Value
Psycho replied to ncurran217's topic in PHP Coding Help
$serverName = 'SRB-Nick_Desktop\SQLEXPRESS'; $connectionInfo = array('Database'=>'cslogs', 'UID'=>'cslogslogin', 'PWD'=>'123456'); $connection = sqlsrv_connect($serverName, $connectionInfo); $query = "SELECT ForteID, Rep FROM Reps ORDER BY Rep"; $result = sqlsrv_query($connection,$query); if (!$result) { return 'ERROR: ' . sqlsrv_errors(); } echo "<select name='ForteID' id='ForteID'>\n"; while ($row = sqlsrv_fetch_array($result, SQLSRV_FETCH_ASSOC)) { echo "<option value='{$row['ForteID']}'>{$row['Rep']}</option>\n"; } echo "</select>\n"; sqlsrv_free_stmt ($result); sqlsrv_close( $connection); -
Even if there was a built in function that allowed you to pass arrays, such as preg_replace allows in HP, how do you think that function would actually perform the logic? it would use loops.
-
Looks like you mis-typed your explanation of the result - not sure what you meant. I think you meant that you are seeing HTML code in the text area. If so, then that means there was HTML code in the content saved to the database (which would be correct in my opinion) OR you are appending HTML code either before saving or before using htmlentities(). You don't want to be using the raw unescaperd content from the database, because if any of the saved data had characters that would be interpreted as HTML code it would screw up the page - which is the reason you would use htmlentities()
-
Just to add to what Muddy_Funster first stated. You do not want to be duplicating data across multiple tables (such as customer name). The purpose of a relational database is to relate data across tables. This is typically accomplished by using the ID (or another unique value) from one table as a foreign key in another table of records to relate them. In this case you only want to use the Client ID in the tasks table. You can then reference the client name (or any other data in the client record) by JOINing the records in the two tables. I suggest you look for some database tutorials on database design and how to do JOINs.
-
Here is a rewrite of your code to output the tasks. The only thing you did not provide was the name of the customer's table - so change that as needed in the query below. I also broke up the code to separate the logic from the presentation. This makes maintenance a whole lot easier. Put all of the code to generate the output at the top of your script (or even in a separate file) and then put the output at the bottom of the script using just echo statements. <?php print_dbconn(); $query = "SELECT c.name, t.taskid, t.eta, t.description FROM customers AS c LEFT JOIN `cms_tasks` AS t ON c.cid = t.cid ORDER BY `taskid` DESC"; $result = mysql_query($query) or die("Query: <$query<br>Error: ". mysql_error()); $taskOutput = ''; if(!mysql_num_rows($result)) { $taskOutput .= "<tr style='background-color:#f6fabd;'>\n"; $taskOutput .= "<td colspan='4'><p align='center'>Oops, it appears you don't have any tasks created yet!</p></td>\n"; $taskOutput .= "</tr>"; } else { while($row = mysql_fetch_array($result)) { $description = limit($row['description']); $tasksOutput .= "<tr>\n" $tasksOutput .= " <td class='taskhead' width='120'><b>TASK ID: {$row['taskid']}</b></td>\n"; $tasksOutput .= " <td class='taskhead' width='260'><b>{$row['name']}</td>\n"; $tasksOutput .= " <td class='taskhead' width='150'><b>ETA: {$row['eta']}</b></td>\n"; $tasksOutput .= " <td class='taskhead' width='120'>\n"; $tasksOutput .= "<a class='edit' href='?module=/tasks/edit&taskid={$row['taskid']}'>edit</a>\n"; $tasksOutput .= "<a class='delete' href='?module=/tasks/delete&taskid={$row['taskid']}' onclick='return confirm('Are you sure you want to delete this task?');'>delete</a>\n"; $tasksOutput .= " </td>\n"; $tasksOutput .= "</tr>\n"; $tasksOutput .= "<tr>\n"; $tasksOutput .= " <td colspan='4' style='border-bottom:solid 1px #666666; line-height:16px; color:#333333; padding:10px'>{$description}</td>\n"; $tasksOutput .= "</tr>\n"; } } ?> <table width="700px" border="0" cellspacing="0" cellpadding="0"> <?php echo $tasksOutput; ?> </table>
-
I'm not very familiar with JQuery to be able to write the solution, but I can provide the framework for the solution. Instead of appending the text for each checked value you need to save the values to variable(s) associated with each page. Then, once you've processed all of the checkboxes - them create the output from those variables. Here is some sample code - not sure if it is functional function update_eShow() { $("#eShow").html(''); var values = new Array(); $(".hidden_edtype").each(function() { if(showPage!==true) { //On first iteration append page description var page = $(this).attr("name"); $("#eShow").append('page:' + page + ' value:'); showPage = true; } else { //On 2+ iterations append comma separator $("#eShow").append(','); } //Append value to output var value = $(this).attr("custom"); $("#eShow").append('page:' + page + ' value:' + value +'<br>'); }); }
- 7 replies
-
- checkboxlist
- javascript
-
(and 3 more)
Tagged with:
-
Srsly? Why?? Hmm, maybe I didn't state that quite right. You can have multiple timestamp fields in a table, but only ONE can use a default value or auto-update to populate the current timestamp value. Why? I have no idea. Like I said, makes perfect sense to me to support a creation date and a last modified date. But, if you try you will get an error such as this #1293 - Incorrect table definition; there can be only one TIMESTAMP column with CURRENT_TIMESTAMP in DEFAULT or ON UPDATE clause
-
Right, but you can only have ONE timestamp field in a table. So, you would use the timestamp field for the date_modified field. For the date_created field you would have to use a datetime type which does not allow you to set a default of CURRENT_TIMESTAMP. I don't know why this is not supported as it would seem to be a common usage. So, the best solution, IMHO, is to use the timestamp with an auto-update of CURRENT_TIMESTAMP and then manually set the date_created value in the method to add records.
-
Actually I meant both. I could have sworn you can use a timestamp with an autoupdate value AND a datetime with a default - but apparently not.So, what you should do, is create the date_updated field as a timestamp with the trigger to update with the current timestamp. Then you should NEVER need to touch that field it will be set automatically when the record is created and whenever the record is modified. Then create the date_created field as a date_time and in the function to create the record use the value of NOW() within the query for that field. You shouldn't need to pass the current date/time to the function to create the record Here is an example of how the table structure might look like CREATE TABLE `category` ( `cat_id` int(11) NOT NULL auto_increment, `type_id` int(11) NOT NULL, `cat_name` varchar(25) NOT NULL, `date_created` datetime NOT NULL, `date_modified` timestamp NOT NULL default '0000-00-00 00:00:00' on update CURRENT_TIMESTAMP, PRIMARY KEY (`type_id`) ) Then the method/function to create the record might look like this class cats { public var $name; public var $type_id; public function save() { $query = "INPUT INTO category (`type_id`, `cat_name`, `date_created`) VALUES ('{$this->type_id}', '{$this->name}', NOW())"; $result = mysql_query($query); return ($result!=false); } }
-
You should turn off magic quotes. But, you should not use addslashes. You should use the appropriate method based upon the database you are using AND the type of data. For MySQL, when escaping string data you should use mysql_real_escape_string() or mysqli_real_escape_string(). If you were to use addslashes AND mysql_real_escape_string you would actually escape the escaped characters ending up with corrupt data (which is why you are having to use stripslashes when usign the data from the database!). Taht should answer your first question. For data that should be an integer you should either cast the value as an integer or use intval(). For floats or dates or whatever you need to use the appropriate method of validating/sanitizing the data. However, you can remove the need for sanitizing the input (e.g mysql_real_escape_string) by using prepared statements. But, you should still run processes to validate that the data is of the right type (e.g. intval). Typically htmlspecialchars() is sufficient. The important thing to remember is that you need to prepare the data appropriate based upon the intended output. You maybe outputting to an HTML page today, but tomorrow you may want to output the data to a PDF file or something else. So, I never recommend running functions such as htmlspecialchars() on the data before saving it to the database. You are outputting to a web page - so use htmlspecialchars() or htmlentities(). Nothing else special needed
-
You are giving all the checkboxes in a group the same ID - that is not valid for HTML. All elements need a unique ID. Also, since you have different "groups" of checkboxes named as arrays - you should give each group a different index for that array. You will then use that index to identify which group each checkbox belongs to.
- 7 replies
-
- checkboxlist
- javascript
-
(and 3 more)
Tagged with:
-
And, just to add a side comment, I see the following in the code // INPUT DATE & TIME $date = date('Y-m-d'); $DT = date("Y/m/d H:i:s"); $cats->created = $DT; $cats->modified = $DT; I'm assuming you are creating those values for insertion with the record. If so, you are going about it the wrong way. You should instead create those fields in the database to automatically set those values when you insert/update records.
-
Now that is a good follow up question! Sometimes I put in more advanced code than I think the person asking the question is familiar with. The intent is for that person to use it as a learning opportunity. In the foreach loop you may have noticed that I used an ampersand before the variable $pattern. Without the ampersand PHP will assign the value of each array element to the variable in the foreach statement. You can then use that variable within the loop as needed. But, if you modify that variable in the loop it doesn't affect the value in the array. however, if you put the ampersand there it passes the value "by reference". This means that it is not simply assigning the value to the variable - the variable is a reference to the actual value in the array. So, if you modify the value in the loop, you are modifying the value in the array. This can be a confusing to understand at first. As Christian stated, the \b is a word boundary when doing regular expressions (another thing that can be confusing at first). So, if you have a regular expression such as \bave\b, the expression is looking for the string 'ave' that starts with a word boundary or ends with a word boundary. What does that mean? In basic terms it means that it can't be within another word. So, a word boundary can be the absolute beginning or end of a string, a space, a tap, a period or other punctuation mark. Some examples might help. Using the regular expression \bave\b: "125 fifth ave." would be a match "125 fifth ave suite #5" would be a match "125 fifth avenue" would NOT be a match "talk to dave" would NOT be a match
-
You still have copies of the original, pre-modified files, right? There are plenty of utilities that will do search/replace in many files. I wouldn't bother with trying to "fix" this issue and instead of getting the end results you need. Here is just such a utility I used many years ago - so I can't comment on it's current viability: http://www.softpedia.com/get/File-managers/Search-and-Replace-98.shtml
-
I don't know the answer to your question, but is there a reason you can't add an id to that field and make this simple?
-
I'm guessing that the example output is wrong. Your input has four 'indicies' of 1, 4, 7, and 2. The variable you are trying to add up to is 3. The sample output is 0 and 1? 0 + 1 = 1, 1 != 3. I would think the correct output is 1 & 2. Those are two if the indicies from the input that add up to the variable 3.
-
Seriously? That wasn't a "tutorial" it was a working example. I even included comments at each step to state what that code was doing. So, let's go through it. First section was to define an array for all of the replacements. Is there something you don't understand about this or how you would need to create the array for your needs? Next section was an array of test values - since that is only used for testing you don't need it. Then there is a section to convert the master array of replacements into a usable array for the regular expression. You would want to do the same thing with the master array you create Lastly you run the regular expression and get the results. In the script above I used the test array. Instead you will want to use whatever value you want to run the replacements on. I.e. the text-area input.
-
## I apologize in advance for the following rant. ## Even if it could I would avoid your site like the plague. My own company does that with several of our web applications and it annoys the hell out of me. It's my freakin' browser, if I want it maximized I'll do it myself. It strikes me as pure arrogance that a site feels that their application is so important that it should be viewed full screen for me. WTF? I have very large monitors - depending on what system I'm using - and if the site is built to work with moderately sized monitors (as it should) then making it full screen for me is going to mostly display empty space. It would be like having a TV that uses PIP (Picture In Picture) and when a commercial comes on it detects that it is running in a small window and decides for me that it is more important and should take up the full screen. Leave my freakin' browser window alone.
-
Can't Display Books According To Category - Help Debug
Psycho replied to magzaloo's topic in PHP Coding Help
Well, I'm not really sure what you were trying to accomplish. You were outputting a header for categories and then it looks like you were attempting to output book titles. But, the following query will return a list of category names and the associated book titles. The last line can be used to only get the books from a particular category SELECT tcategory.name, tBook.title FROM tcategory LEFT JOIN tBook ON tBook.category = tcategory.id -- WHERE tcategory.id = $id -
I would use regular expression which can look for word boundaries, make the replacement case-insensitive, and add an optional period at the end. Here is an example script. Note: I couldn't get the optional period to work. It normally has a special meaning in regular expression and I couldn't seem to properly escape it - and I'm too busy to research at the moment. //Master aray of replacements $replacements = array ( 'st' => 'street', 'av' => 'avenue', 'ave' => 'avenue' ); //Array of test values to modify $test_values = array ( "1st st", "1st St", "1st St.", "1st ave", "Averton av", "Averton ave", "Averton Ave." ); //Create separate array for search patterns $patterns = array_keys($replacements); //Modify patterns to: // 1 - include word boundaries // 2 - make case insensitive // 3 - add optional period NOT IMPLEMENTED foreach($patterns as &$pattern) { $pattern = '#\b' . $pattern . '\b#i'; } //Perform replacement for all test values $results = preg_replace($patterns, $replacements, $test_values); //Output the before and after results echo "<br><br>Before:<pre>" . print_r($test_values, 1) . "</pre>"; echo "After:<pre>" . print_r($results, 1) . "</pre>"; Results Before: Array ( [0] => 1st st [1] => 1st St [2] => 1st St. [3] => 1st ave [4] => Averton av [5] => Averton ave [6] => Averton Ave. ) After: Array ( [0] => 1st street [1] => 1st street [2] => 1st street. [3] => 1st avenue [4] => Averton avenue [5] => Averton avenue [6] => Averton avenue. )
-
I was going to agree with Stooney until I notices a problem: 'st'. In your example you state the input could be something like "1st st" and you want that converted to "1st Street". But, str_replace would actually change that to "1street street". Now, regular expression *might* be a better solution. But, the thing is that it is nearly impossible to programatically modify "text" as a human would do it. As in the example above, you really only want to replace 'st' with 'street' based upon the context that it is used. Humans are great at deciphering the meaning of something based upon the context of how it is used. To have a machine do it requires programming an understanding of all those possible contexts. For example, you and I would understand the following inputs as 'logically' the same thing: "1st st", "First St.", "first street", etc. So, your best bet is to create a programatical solution that will meet a large number of possibilities, but will never be fool proof. For somethign like this I would think it would be better to not modify a value that perhaps should be than to erroneously modify a value that shouldn't.
-
Can't Display Books According To Category - Help Debug
Psycho replied to magzaloo's topic in PHP Coding Help
The error is exactly as it states "Can't use function return value" A foreach loop is used to iterate over the elements in an array, correct? You used mysql_fetch_array() as the array parameter in the foreach() loop. mysql_fetch_array() is a function As the error states you cannot use the return value from a function for the array parameter in a foreach loop. But, looking at your code there wouldn't be anything returned from mysql_fetch_array() anyway since you never ran a query. You have a query to run in the class, but you never even initiated the class. EDIT: after looking at your code a little closer, it is full of problems. The constructor of the class assumes there are some values to assign to the properties of the class. But, it seems you are expecting to get those properties after running the method get_category_name(). You are putting the cart before the horse! I was going to try and rewrite it for you, but there isn't enough information. Please provide the details of the category table and the book table. -
You say you've added a "field" into the database. That implies you've added that field as part of some existing table - so I'm not understanding what you mean by that. Based upon how you want to use this data I think you need a table with fields for clerk_id, clerk_name, and last_used. The last_used field will be a timestamp value to indicate the last time the clerk was used on the form. So, on each page load of the form you would run two queries: one to get the clerk with the oldest "last_used" date and another to update their "last_used" date to the current timestamp.
-
Call To Undefined Function Themeheader()
Psycho replied to jmeyers's topic in PHP Installation and Configuration
I'll add the following: OK, so you've found the file where the function is defined. So, somewhere that file was previously being included. There are a few things you can to figure out the cause: 1. The file was moved from it's previous location so the include() can't find it. You can check this by doing a search of the files for the file name. If you find any includes see if the path matches the file's actual location 2. The file name was changed so, again, the include() cannot find the file. I would suggest removing the at symbol from the front of every include() in all your files. 3. The last possibility is that the line of code to include the file has been removed. If this is the case, well, I don't know. It would be difficult to figure out where the include should be unless there are appropriate comments in the code. -
Call To Undefined Function Themeheader()
Psycho replied to jmeyers's topic in PHP Installation and Configuration
I *assume* that the user is not typing www.yourdomain.com/header.php into their browser but is instead going to www.yourdomain.com/somepage.php and it is the somepage.php that is including some files and it either directly including header.php or one of the files that it is including is ultimately including header.php. When a user requests a page in their browser the server could be processing one file or hundreds - which are chained together through includes or requires. Somewhere in the processing of all those files the function themeheader() needs to be defined before you call the function. There is no way for us to know where that function is defined or how the page where it is defined was/is included. It could be that the function was removed or renamed or that the file which defines that function was removed, renamed or moved to a different location. Ok, so I said ampersand instead of apetail (i.e. at symbol). But seriously, you couldn't deduce that is what I meant? Every single one of the include statements begins with the same character. Here is another suggestion. Find a utility that will search the contents of all of the files and look for "function themeheader" and find out what page that file is defined in. Then do a search for any includes for that file. That should get you going in the right direction.