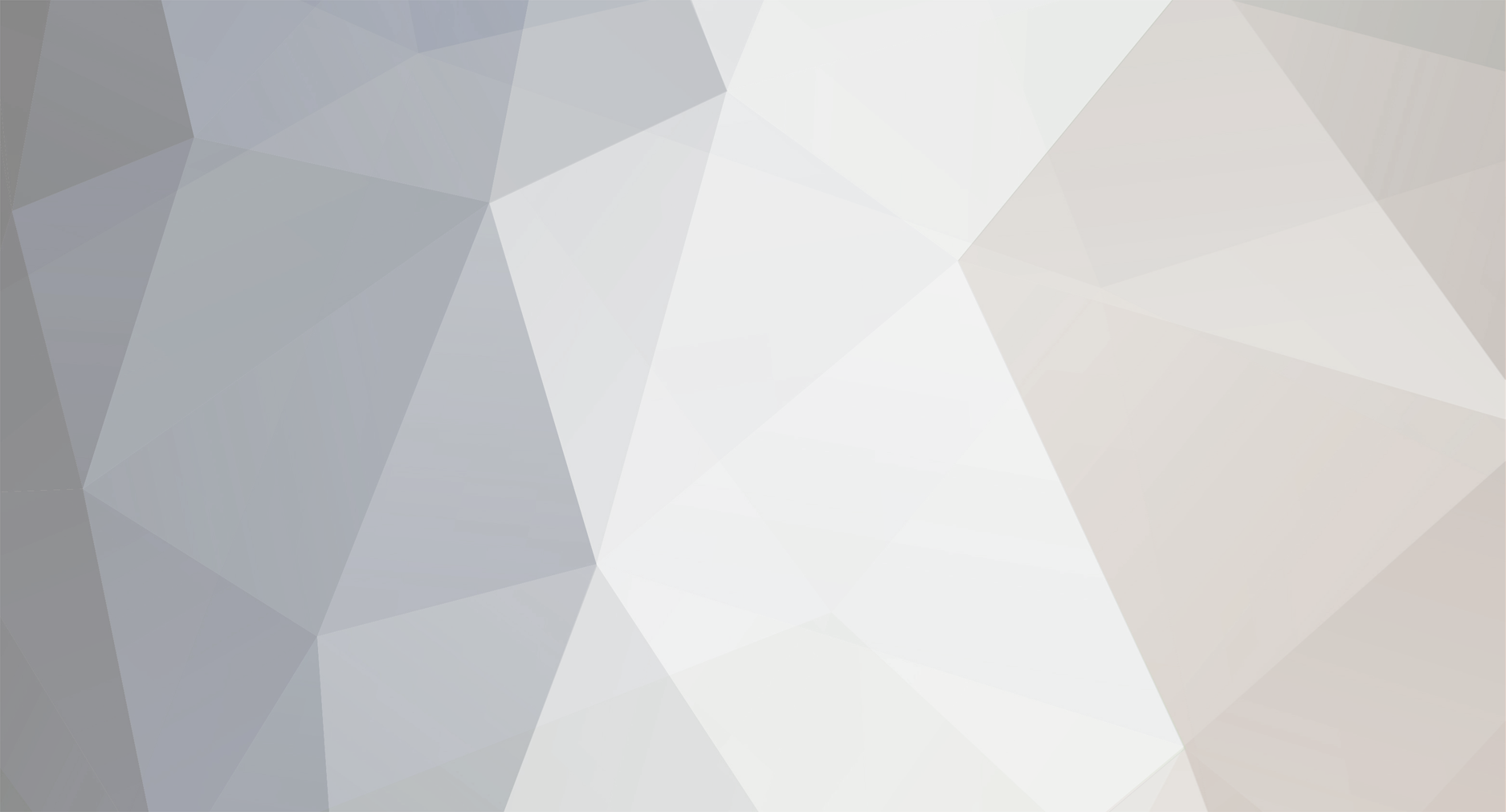
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
@Nodral: You shouldn't replicate code that does not change int he alternating logic. It will lead to bloated, hard to maintain code. Keep it to the core values. @ncurran217: The ternary operator is a great solution for this // each array key is the database column name, the corresponding value is the legend/heading to display in the HTML table // the order of the items in this array are the order they will be output in the HTML table $fields = array( 'RefNumber'=>'Reference Number', 'Status'=>'Status', 'Rep'=>'Rep', 'Disposition'=>'Disposition', 'appNumber'=>'appNumber', 'Con_Number'=>'Contract Number', 'Finance_Num'=>'Finance Number', 'Phone_Num'=>'Phone Number', 'Disc_Amount'=>'Discount Amount', 'Total_Cost'=>'Total Cost', 'Total_MP'=>'Total Monthly Payments', 'New_MP_Amt'=>'New MP Amount', 'New_DP_Amt'=>'New DP Amount', 'Notes'=>'Notes'); $color1 = "#FFFFFF"; $color2 = "#A4A4A4"; // start table and produce table heading echo "<table>\n<tr>"; foreach($fields as $legend) { echo "<th>$legend</th>"; } echo "</tr>\n"; // output table data $rowCount = 1; while($row = sqlsrv_fetch_array( $result,SQLSRV_FETCH_ASSOC)) { $rowcolor = ($rowCount % 2) ? $color1 : $color2; //Ternary operator echo "<tr bgcolor='{$rowcolor}'>"; foreach(array_keys($fields) as $key) { echo "<td>{$row[$key]}</td>"; } echo "</tr>\n"; $rowCount++; //Increment Row Counter } echo "</table>\n";
-
At first it looked like you were running a separate query for each word in the array, but I was mistaken on that. What you ARE doing is redefining the query for each word in the array. Then, after you iterate over each word in the array you run the query (which was created for the last word of the array). In other words, the query does not contain anything for the words before the last one. The tutorial you linked to has the exact same problem. The code you posted above won't work because you are not sanitizing and preparing the values appropriately. Go find a better tutorial.
-
You need to first test your query in your database admin pane. Once it is getting the correct results - THEN implement it in the code. Trying to debug problems queries that are dynamically generated in PHP can be difficult when you don't know where the problem is. But, I see one glaring problem in the above code. You are apparently running multiple queries in loops. Not only is this a very poor implementation, but it makes no sense how you've done it. In the loop you are running the query and assigning the result set of the query to $result - then you loop again running the next query. You never use the contents of $result until all the queries in the loop have been executed. So, you are only left with the result from the last query executed in the loop.
-
You simply need create a script to perform the process as you described. Then create a scheduled task to run every seven days to execute that task. On a Linux server this is typically handled through a CRON job. Your host should have a way of scheduling these
-
I think you jumped to a conclusion. The sample DB records and his text seem to clearly indicate that his DB design is appropriate.
-
Is there a way to set up an automated printing in PHP?
Psycho replied to eldan88's topic in PHP Coding Help
I believe we've already given you an option. Build a web app that will run on an internal server. The app will check the status of orders and print them out as they are placed. The trick is that the printing has to be done from the server - not the client. -
Do a print_r() on $_FILES to ensure it contains what you think it does
-
That's because you are defining the size as false/0. $size = filesize($_FILES['file']['name']); The 'name' index is just the name of the file - not the location. Use 'tmp_name' to get the filepath to the file.
-
You are making it more complicated than it needs to be. Simply store the group and point amount change (e.g. 5 or -5) no need to store a separate field to say weather the value is being added or subtracted. Second, there is no need to use these individual records to update records in another table. You can simply get the total for a group/house by doing a query on the table that will sum up all the additions and subtractions. SELECT groupName, SUM(points) AS totalPoints FROM tableName GROUP BY groupName
-
The file is index.php - I put that on the end of the URL and got the same result. I also loaded up the include files (both .php) and only saw source code as well (including the DB password!). So, PHP files are definitely not being parsed.
-
All of your conditions must be contained within a single set of parens (but you may have sub-parens inside) E.g. if ($Hoff == 'Nelspruit' || $Hoff == 'Hoedspruit' || $Hoff == 'Klaserie' || $Hoff == 'Bushbuck Ridge' || $Hoff == 'Hazyview' || $Hoff == 'Graskop' || $Hoff == 'Sabie' || $Hoff == 'Lydenburg' || $Hoff == 'Pilgrims Rest' || $Hoff == 'Barberton' || $Hoff == 'Waterval Boven' || $Hoff == 'Komatipoort' || $Hoff == 'Malelane') { $addon = $addon +2; } else { $addon = $addon -100; } Or if ( ($Hoff == 'Nelspruit') || ($Hoff == 'Hoedspruit') || ($Hoff == 'Klaserie') || ($Hoff == 'Bushbuck Ridge') || ($Hoff == 'Hazyview') || ($Hoff == 'Graskop') || ($Hoff == 'Sabie') || ($Hoff == 'Lydenburg') || ($Hoff == 'Pilgrims Rest') || ($Hoff == 'Barberton') || ($Hoff == 'Waterval Boven') || ($Hoff == 'Komatipoort') || ($Hoff == 'Malelane') ) { $addon = $addon +2; } else { $addon = $addon -100; } But, a much better way to handle this is by using an array. You can replace all of the above code with just this $hoffList = array( 'Nelspruit', 'Hoedspruit', 'Klaserie', 'Bushbuck Ridge', 'Hazyview', 'Graskop', 'Sabie', 'Lydenburg', 'Pilgrims Rest', 'Barberton', 'Waterval Boven', 'Komatipoort', 'Malelane' ); $addon += (in_array($Hoff, $hoffList)) ? 2 : -100;
-
Debugging is not hard - you just need to determine where the different conditions/branches are in the logic and then TEST before and after the condition to see what is actually happening. You say that the code has not changed, you are not getting any errors, and everything is working except for the data not getting in the database. If you are checking for errors and none are occurring there are two possible conclusions that I can think of: 1. Your script is inserting records into a different database/table than you think it is. 2. The code to do the insertion is not getting executed because of same conditions/logic in the code So, it is simple to check to see if the insert query is being executed at all. just find where you have the insert query and create a couple of echo statements to validate $query = "INSERT INTO table (fiel1, field2, field3) VALUES ('$value1', '$value2', '$value3')"; echo "Executing query: $query<br>"; $result = mysql_query($query); if(!$result) { echo "Error: " . mysql_error(); } else { echo "Successfully inserted " . mysql_affected_rows() . " records"; } Either you will see that text on the page and will know for certain what is happening or you won't see anything - which means that code is not getting executed and you need to look at your branching logic.
-
But, what if what? That would never work for two reasons. First, as I stated before, when the browser requests a PHP page from the server. The server will retrieve the document and process it (and any included files) in its entirety. Once all the processing is complete the server will THEN send the completed page to the browser. The server does not send bits and pieces of the page to the browser as it encounters each echo. Second, a header() call cannot be made AFTER content is sent for output. Therefore, the echo statement above would cause the header() to produce the error: So, the end result of that script is that the user would be looking at a blank page for 5 seconds (because the script has not completed processing), then the user will see the error message. The user would never see the echo statement at all and wouldn't even get redirected. So, that script not only fails - it fails twice.
-
I would definitely go with the Meta tag approach rather than Javascript. It is a feature implemented in the core HTML and wouldn't have any problems due to Javascript being enabled or not. However, this Would definitely not work. A page is not delivered to the browser until processing is complete. Therefore, that sleep() command would simply cause the user to view a blank page for 10 second before ultimately being redirected to the second page - i.e. they would never see the first page with the message.
-
Well, the problem I pointed out does not exist in the original script.
-
I've never seen this before - is this even legit - defining a variable inside a call to run a query? $stmt = $adopts->query($query = "SELECT * FROM {$prefix}adoptables, {$prefix}"."owned_adoptables WHERE owner='".$loggedinname."' AND currentlevel<'6' AND isfrozen='no'");
-
I would also advise reviewing your logic in general. There are a ton of nested if conditions in that code. That makes it very difficult to debug and maintain your code. If you have an if/else condition for an error - create the condition to check for the error - not the success - so you can put the error logic right after the condition. Otherwise the code is disconnected This is poor format, in my opinion if(SUCCESS_CONDITION_1) { if(SUCCESS_CONDITION_2) { //Success logic } else { //Error condition 2 logic } } else { //Error condition 1 logic } This is better/cleaner if(ERROR_CONDITION_1) { //Error condition 1 logic } elseif(ERROR_CONDITION_2) { //Error condition 2 logic } else { //Success logic }
-
all possible combinations for scheduling pattern
Psycho replied to jenkins's topic in PHP Coding Help
Instead of just providing the answer, let me give you a review of the analysis I went through. You should be able to get the solution yourself and it will help you to think critically. After the previous issue identified, I made the following analysis: The duplications was, as you identified, due to days of similar lengths being swapped. Why was this? Well, the 4 hour query was looking for one day of 2-hours and 2 days of 1-hour. Obviously there is no problem with the single 2-hour day being duplicated since it is only looking for one. So, the problem was just the two 1-hour days. In the query I had a condition to ensure that the two 1-hour days would not fall on the same day AND time2.day <> time3.day That worked, but did not prevent the days from being swapped and creating results that were, logically, the same result. The solution that came to me was to only pull results where the 1st 1-hour day was before the 2nd 1-hour day. That prevents the swapping. At first I was going to create some additional fields so Mon = 1, Tue = 2, etc. and then do a comparison where day1 < day2. But, I realized it really didn't matter which day cam before another - so I just used STRCMP on the day names. So, the logical conclusion is that when creating a query where you need classes on multiple days of different lengths: 1. To ensure no two classes fall on the same day, you need to have conditions such as time1.day <> time2.day But, for each additional class these conditions need to expand. So, for day three you need two conditions to ensure it doesn't fall on the same day #1 or day #2. For a fourth class day you would need three conditions, etc. 2. Additionally, if you have multiple class days of the same length you need to create a condition such that they days are not swapped - which would create a duplicate. For this you would use STRCMP. This is only needed for days of the same length. So, for a 5hour class that you want over 5, 1-hour days I think the query could look like this (not tested) SELECT time1.day, time1.hour, time2.day, time2.hour, time3.day, time3.hour, time4.day, time4.hour, time5.day, time5.hour FROM timeBlocks AS time1 JOIN timeBlocks AS time2 ON time2.day <> time1.day AND STRCMP(time1.day, time2.day) < 0 JOIN timeBlocks AS time3 ON time3.day <> time1.day AND time3.day <> time2.day AND STRCMP(time2.day, time3.day) < 0 JOIN timeBlocks AS time4 ON time4.day <> time1.day AND time4.day <> time2.day AND time4.day <> time3.day AND STRCMP(time3.day, time4.day) < 0 JOIN timeBlocks AS time5 ON time5.day <> time1.day AND time5.day <> time2.day AND time5.day <> time3.day AND time5.day <> time4.day AND STRCMP(time4.day, time5.day) < 0 WHERE time1.length = 1 AND time2.length = 1 AND time3.length = 1 AND time4.length = 1 AND time5.length = 1 EDIT: OK, I've tested it and there are 32 combinations returned. -
Everything before "<?php" is output to the browser. You cannot have any output before you start the session.
-
all possible combinations for scheduling pattern
Psycho replied to jenkins's topic in PHP Coding Help
I tested these somewhat and they appear to produce the correct results For a 4 hour class (2 + 1 + 1) - 120 total combinations. SELECT time1.day, time1.hour, time2.day, time2.hour, time3.day, time3.hour FROM timeBlocks AS time1 JOIN timeBlocks AS time2 ON time1.day <> time2.day JOIN timeBlocks AS time3 ON time1.day <> time3.day AND STRCMP(time2.day, time3.day) < 0 WHERE time1.length = 2 AND time2.length = 1 AND time3.length = 1 For a 5-hour pattern (2 + 2 + 1) - 60 total combinations. SELECT time1.day, time1.hour, time2.day, time2.hour, time3.day, time3.hour FROM timeBlocks AS time1 JOIN timeBlocks AS time2 ON time1.day <> time2.day AND STRCMP(time1.day, time2.day) < 0 JOIN timeBlocks AS time3 ON time1.day <> time3.day AND time2.day <> time3.day WHERE time1.length = 2 AND time2.length = 2 AND time3.length = 1 -
all possible combinations for scheduling pattern
Psycho replied to jenkins's topic in PHP Coding Help
Yes, I think all the time. -
I thought that is what you meant at first - but then your first question makes no sense That would never return a match. For example, let's say the values within the IN clause are like this WHERE field IN ('apple', 'banana', 'cucumber') It is impossible for any ONE field value to match multiple comparison values. It could be 'apple' OR it could be 'banana', but it could never be 'apple' AND 'banana' If those vars are PROPERLY formatted comma separated strings - then go back to using the IN clause. If that does not get the results you want then you need to try and explain what you want in a different way (perhaps provide some examples). $query = "SELECT h.id, h.fname, h.lname, h.lhname, h.cnum, h.exnum, GROUP_CONCAT(s.sname SEPARATOR ', ') AS snames FROM hire AS h LEFT JOIN skill AS s USING(id) WHERE sname='$sname' AND lhname IN ($ser2) AND exnum IN ($ser3) GROUP BY h.id"; $result = mysql_query($query); if(!$result) { echo "Query Failed!<br>\n"; echo "Query: {$query}<br>\n"; echo "Error: " . mysql_error(); } else { while($row = mysql_fetch_assoc($result)) { echo "<tr>\n"; echo "<td>{$row['id']}</td>\n"; echo "<td>{$row['fname']}</td>\n"; echo "<td>{$row['lname']}</td>\n"; echo "<td>{$row['lhname']}</td>\n"; echo "<td>{$row['cnum']}</td>\n"; echo "<td>{$row['exnum']}</td>\n"; echo "<td>{$row['snames']}</td>\n"; echo "</tr>\n"; } }
- 4 replies
-
- mysql
- fetch_array
-
(and 2 more)
Tagged with:
-
$query = "SELECT h.id, h.fname, h.lname, h.lhname, h.cnum, h.exnum, GROUP_CONCAT(s.sname SEPARATOR ', ') AS snames FROM hire AS h LEFT JOIN skill AS s USING(id) WHERE h.sname='$sname' AND h.lhname = '$ser2' AND h.exnum = '$ser3' GROUP BY h.id"; $result = mysql_query($query); if(!$result) { echo "Query Failed!<br>\n"; echo "Query: {$query}<br>\n"; echo "Error: " . mysql_error(); } else { while($row = mysql_fetch_assoc($result)) { echo "<tr>\n"; echo "<td>{$row['id']}</td>\n"; echo "<td>{$row['fname']}</td>\n"; echo "<td>{$row['lname']}</td>\n"; echo "<td>{$row['lhname']}</td>\n"; echo "<td>{$row['cnum']}</td>\n"; echo "<td>{$row['exnum']}</td>\n"; echo "<td>{$row['snames']}</td>\n"; echo "</tr>\n"; } }
- 4 replies
-
- mysql
- fetch_array
-
(and 2 more)
Tagged with:
-
That error is a sure sign that your query failed. the result will be a Boolean false and not a DB result resource - thus the error. You need to check for errors - don't assume you write the code correctly the first time. Plus, there is absolutely no reason to use the IN clause if you are only going to have one value. And, you do not have quotes around the search values. Unless these are number values it will cause this type of error. Lastly, never run queries in loops - there is always a better way. In this case the better way is with a JOIN query the GROUP_CONCAT function.
- 4 replies
-
- mysql
- fetch_array
-
(and 2 more)
Tagged with:
-
And where is the code? Are you sure there are no spaces or line breaks before the opening PHP tags and that it is not included in another file that outputs content before it is included?