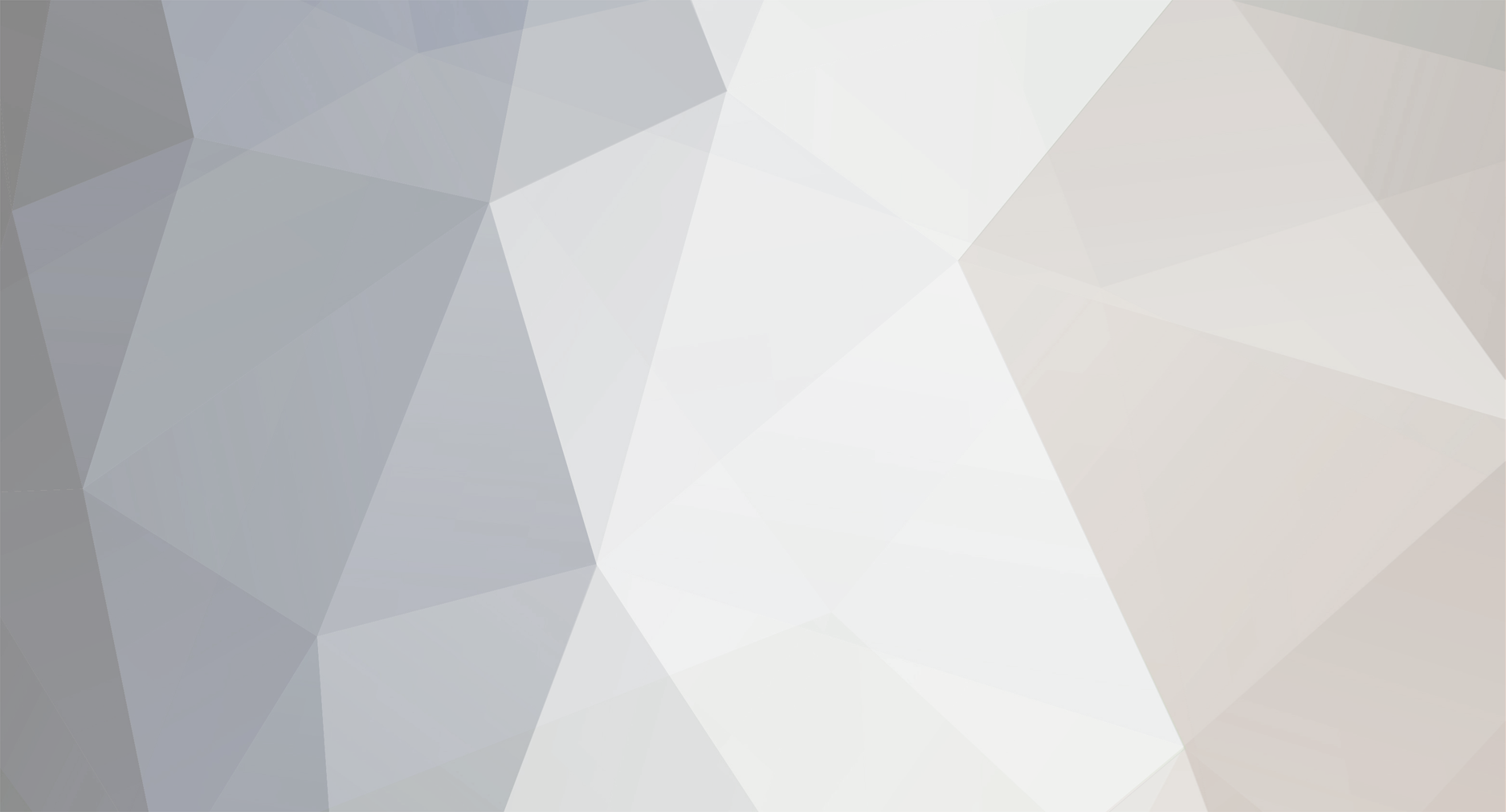
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Assuming you start the session at the beginning of your script(s) if(!isset($_SESSION['viewed'])) { mysql_query("UPDATE videos SET views = $views WHERE code = '$video'"); $_SESSION['viewed'] = 1; }
-
FWIW: http://www.php.net/manual/en/datetime.formats.relative.php
-
Also, what version of PHP are you using. Some of the possible formats are not supported in earlier versions.
-
all possible combinations for scheduling pattern
Psycho replied to jenkins's topic in PHP Coding Help
Umm . . . I based my proposed solution on the example you posted in your original post. If the requirements are more complicated than that it would have been nice that you at least stated as much. I don't have my test table to verify, but I think you can do this for other conditions - but the query would get more complicated. For a 4 hour class (2 + 1 + 1) I think this should work: SELECT time1.day, time1.hour, time2.day, time2.hour, time3.day, time3.hour FROM timeBlocks AS time1 JOIN timeBlocks AS time2 JOIN timeBlocks AS time3 WHERE time1.length = 2 AND time2.length = 1 AND time3.length = 1 AND time1.day <> time2.day AND time1.day <> time3.day AND time2.day <> time3.day For a 5-hour pattern (2 + 2 + 1), I think this would work SELECT time1.day, time1.hour, time2.day, time2.hour, time3.day, time3.hour FROM timeBlocks AS time1 JOIN timeBlocks AS time2 JOIN timeBlocks AS time3 WHERE time1.length = 2 AND time2.length = 2 AND time3.length = 1 AND time1.day <> time2.day AND time1.day <> time3.day AND time2.day <> time3.day -
Try putting the file in a different location
-
The problem is simple. Step through each line of code and figure out what would happen and/or echo the values of key variables before and after changes are made. Those are key debugging techniques and would allow you to find the problem yourself. But, I'll be nice and just tell you the problem. The loop is executing twice and it does replace the first occurrence of 'apple'. But, look how you get your $new_string $new_string = substr_replace ($string, $replace, $string_position , $find_length); It defines $new_string as the ORIGINAL string with the current replacement. So, on the first loop it defines $new_string as the original string with the first occurrence of 'apple' replaced. Then on the second iteration of the loop it REDEFINES $new_string as the ORIGINAL string with the second occurrence of 'apple' replaced. So, you lose the first replacement. $string = 'That\'s a simple string. I want to replace a word in this string. I am very fond of eating apples and what about you? I think you are also fond of eating apples right?'; $find = 'apples'; $replace = 'banana'; $find_length = strlen($find); $offset = 0; $new_string = $string; while($string_position = strpos($new_string, $find, $offset)) { $new_string = substr_replace ($new_string, $replace, $string_position , $find_length); $offset = $string_position + $find_length; } echo $new_string;
-
I think you are making it more difficult than it needs to be. Assuming you may want to conduct different polls you will want three tables. One table will be for the Poll question. Another will be for the poll answers. Each record will be a different answer and will have a foreign key reference back to the poll record. When creating a new poll the data will be added to both of those tables before the poll can be used. Then when a poll is ready you simply add a record to the third table (poll_results) when a user answers the poll. But, here is the trick: the query should be an INSERT ON DUPLICATE KEY UPDATE query. Then a user can answer the poll again, but it will only update their previous answer instead of creating a new result. To do this you need to make the COMBINATION of the poll_id and the user_id a unique key.
-
all possible combinations for scheduling pattern
Psycho replied to jenkins's topic in PHP Coding Help
If the data is in a database do a simple JOIN query to get all the combinations. SELECT time1.day, time1.hour, time2.day, time2.hour FROM timeBlocks AS time1 JOIN timeBlocks as time2 WHERE time1.length = 2 AND time2.length = 1 EDIT: After rereading the post I think you want it so the 2hour and 1hour blocks are not on the same day, so you would use this SELECT time1.day, time1.hour, time2.day, time2.hour FROM timeBlocks AS time1 JOIN timeBlocks AS time2 WHERE time1.length = 2 AND time2.length = 1 AND time1.day <> time2.day -
Are you sure you really want to store the value and NOT the ID? That's sort of the point of why you have a relational database - so you can define a value (or values) in one place and reference that values in others without having to duplicate the data. Looking at the code in your first post I don't see why it would be saving the ID as opposed to the value (unless the values in that table are the same as the IDs). But, that code is very inefficient.You should NEVER run queries in loops. If you do store the IDs (as I think you should) you can create ALL the records with just ONE query. But, if you do need to store the values then you can at least replace those four queries in the loop with a single query. foreach($_POST['fields'] as $items) { //Process values for DB use $name = mysql_real_escape_string($_POST['name']); $number = mysql_real_escape_string($_POST['number']); $address = mysql_real_escape_string($_POST['address']); $other = mysql_real_escape_string($items['other']); $hardwareid = mysql_real_escape_string($items['hardwareid']); $replacement = mysql_real_escape_string($items['replacement']); $hardwarerep = mysql_real_escape_string($items['hardwarerep']); $caseID = intval($items['case']); $hmodelID = intval($items['hmodel']); $errorID = intval($items['error']); $query = "INSERT INTO `customer_data` (`name`, `number`, `address`, `other`, `hardwareid`, `replacement`, `hardwarerep`, `case`, `hmodel`, `error`) SELECT '$name', '$number', '$address', '$other', '$hardwareid', '$replacement', '$hardwarerep', (SELECT value FROM fields WHERE id='{$caseID}' LIMIT 1), (SELECT value FROM fields WHERE id='{$hmodelID}' LIMIT 1), (SELECT value FROM fields WHERE id='{$errorID}' LIMIT 1)" $result = mysql_query($query) or die(mysql_error()); echo "<br> Din registrering har ID : ".mysql_insert_id(); } But, if you do only store the IDs (as I think you should) then you only need to create one query by looping over the records. It will be much, much more efficient. Something like this $values = array(); foreach($_POST['fields'] as $items) { //Process values for DB use $name = mysql_real_escape_string($_POST['name']); $number = mysql_real_escape_string($_POST['number']); $address = mysql_real_escape_string($_POST['address']); $other = mysql_real_escape_string($items['other']); $hardwareid = mysql_real_escape_string($items['hardwareid']); $replacement = mysql_real_escape_string($items['replacement']); $hardwarerep = mysql_real_escape_string($items['hardwarerep']); $caseID = intval($items['case']); $hmodelID = intval($items['hmodel']); $errorID = intval($items['error']); $values[] = "('$name', '$number', '$address', '$other', '$hardwareid', '$replacement', '$hardwarerep', '$caseID', '$hmodelID', '$errorID')"; } //Create and execute ONE query $query = "INSERT INTO `customer_data` (`name`, `number`, `address`, `other`, `hardwareid`, `replacement`, `hardwarerep`, `case`, `hmodel`, `error`) VALUES " . implode(', ', $values); $result = mysql_query($query) or die(mysql_error());
-
Another consideration: Are you sure the value you *think* is in $mail actually exists in the DB? Could be that the original value and the value in $email were escaped, trimmed, etc. differently which would also explain why you get 0 results.
-
I think you need to show the entire query. A SUM() needs a GROUP BY in order to actually sum anything. And, I believe, it is the GROUP BY that will need to have the logic to group by the age groups. It would also be helpful if you were to show the age groups you will have.
-
And what verifications have you done? 1. You say you have executed the script from a command prompt, but are you manually typing the string in or did you try the dynamically generated string? You are using variable in constructing that string so they may not contain what you think they do. The if() condition is only verifying that those POST variables exist - not that they actually have a value. Echo the command string to the page and then run THAT from the command line. 2. Is the path to the vbs file explicit (i.e. "C:\folder\") or relative (i.e. "\folder\")? If it is relative, it will need to be relative from the folder that the web server is running from (not the folder that the PHP file is executed from). At least this is what I found from my testing. So, in my XAMPP installation my path to the VBS file was relative from the root of the "xampp" root folder not the "htdocs" folder.
-
Managing MySQL INSERTS & UPDATES Along with Queries
Psycho replied to n1concepts's topic in Application Design
To be honest, what you are asking doesn't make sense to me. Either you aren't aware of some basic database handling or the questions are not accurate. What is this supposed to mean? Are you saying you will do a SELECT query to see if a record already exists before deciding to do an UPDATE vs INSERT query? If so, you are doing it wrong. You should be using an INSERT with an ON DUPLICATE KEY clause. This isn't making any sense. I see no performance gain and it would only cause data to get out of sync. -
Ok, so Google has a sitemap of your site. And . . . ? As already stated, the "context" of the content of your pages matter. By formatting the content AFTER the page is loaded you are ensuring that any scanning engine will not read the data as you intend for it to be consumed. Thereby making it impossible for appropriate relevance to be given to any data. As for links pointing to external sites - again so what? Google has complicated algorithms for determining page ranking, but one of the key criteria is page links. I'm sure they use the links pointing to a page as well as what that page links to in order to determine the relevance of the page. We've given you several reasons why formatting the page in PHP is the right decision and you only try to defend your current implementation. Why ask a question if you are only interested in validating your own decision instead of getting honest responses?
-
I'm not going to read through all of your code. You need to learn how to TEST your code. If there is a problem, narrow down the section of code where the error occurs and, if necessary, use appropriate data to expose the root cause. In this case, you are having a problem with multiple uploads and large uploads. So, parse down the code where the problem occurs and try single uploads of varying sizes and then try multiple uploads incrementally. However, I am guessing this is the problem with the single large uploads if($size < 1000000) { uploadImages($tmp, $file_name, $extension, $album_id); } else { } What was the purpose there? Else what??? That size check is for just less than 1MB. So, any file over that size would apparently not be uploaded Also, why do you use a for loop to iterate over an array???
-
???? Why are you not just writing the formatting logic in PHP? Doing this in Javascript is, in my opinion, much more difficult and introduces many issues that would not exist if done with PHP. As for SEO, it could affect the results - but whether it hurts the results I can't say. I'm no SEO expert, but I would assume that HTML formatting for heading tags (e.g. <h1>, <h2>, etc.) and other elements would definitely affect how the page is indexed. I just don't see any good reasons to do this with Javascript.
-
I tried something similar (using COUNT to increment the dynamic var) on a simple table and got the same results. I'm guessing it has something with how the COUNT and GROUP BY operations and the order of precedence. I would suggest just handling that logic in the processing logic. However, I was able to make it work with a sub-query - but I think that would be inefficient. SET @runtot := 0; SELECT *, (@runtot := @runtot + Adjustments) AS RT FROM (SELECT COUNT(adjustment_id) AS Adjustments, DATE(FROM_UNIXTIME(shifts.outtime)) AS 'Month' FROM adjustments INNER JOIN shifts ON (shifts.shiftID = adjustments.shiftID) INNER JOIN employees ON (shifts.idnum = employees.idnum) WHERE YEAR (FROM_UNIXTIME(shifts.outtime)) = '2012' GROUP BY MONTH(FROM_UNIXTIME(shifts.outtime)) ORDER BY MONTH(FROM_UNIXTIME(shifts.outtime)) ASC ) AS sub
-
When you are testing if something is equal to something else you need to use DOUBLE equal signs. A single equal sign is an assignment operator. Although, I would expect incorrect results - I wouldn't expect the results you are getting. As for your code, you should not duplicate a lot of redundant code int he if/elseif/else statements. In this case you should only use that logic to DEFINE the image. Additionally, if the number of images will be dynamic, instead of hard-coding the number of images, create an array of images and let the code figure it out programatically. Since all your images/links have a set format you could just use code to set the "root' word (e.g. 'internship', 'research', or 'classes'), but I would define all the elements separately so you are not tied to that in the future. Lastly, rather than use the day of the month, a better option (IMHO) is the day of the year (i.e. 0 to 365) <?php //Define the elements of the various slides //This is the ONLY code that would need to be modified if slides are added in the future. //Also, this can be stored in a separate file and included OR stored in a DB $slides = array( array( //internships 'page' => 'learning_internships.php', 'text' => 'link to undergraduate internship FAQ page', 'top' => 'learning_outside_internships_top_mouseover.jpg', 'bottom' => 'learning_outside_internships_bottom.jpg', 'over' => 'learning_outside_research_top_mouseover.jpg' ), array( //research 'page' => 'learning_research.php', 'text' => 'link to undergraduate research FAQ page', 'top' => 'learning_outside_research_top.jpg', 'bottom' => 'learning_outside_research_bottom.jpg', 'over' => 'learning_outside_research_top_mouseover.jpg' ), array( //class based 'page' => 'class_based.php', 'text' => 'link to undergraduate hands on learning in classes page', 'top' => 'learning_outside_class_based_top.jpg', 'bottom' => 'learning_outside_class_based_bottom.jpg', 'over' => 'learning_outside_class_based_top_mouseover.jpg' ) ); //Determine which slide to use $slideNum = date('z') % count($slides); //Define the slide to use $slide = $slides[$slideNum]; //Create the output echo <<<EOL <div id="learning_outside_top"> <a href="learning_outside/learning_outside_main.php" title="link to hands on learning page" alt="link to hands on learning page"> <img src="images/{$slide['top']}" onmouseenter="this.src='images/{$slide['over']}'" onmouseleave="this.src='images/{$slide['top']}'"/> </a> </div> <a href="learning_outside/{$slide['page']}" title="{$slide['text']}" alt="{$slide['text']}"> <img src="images/{$slide['bottom']}"/> </a> EOL; ?>
-
Is there a way to set up an automated printing in PHP?
Psycho replied to eldan88's topic in PHP Coding Help
I get free food occasionally by placing an order online and expecting that a human on the other end will do their job correctly. (Hint: humans fail. A lot.) Right, but ultimately a human is required to process the order (at least in the case of a food order). Even if the order is printed it still requires a person to check the printer. You could have a web page that routinely checks for new order and changes the color of the page if there are new, unprocessed orders. That doesn't require creating a second webserver or any way out solutions. At least that's my opinion. -
SELECT * FROM table WHERE number IN (SELECT number FROM table WHERE date <= '2012-05-03')
-
Is there a way to set up an automated printing in PHP?
Psycho replied to eldan88's topic in PHP Coding Help
You could set up the app in the remote server as it is now and set up a local server in the restaurant that will query the application every x minutes for new orders and, if any are found, print them out. But, it seems like a lot of complexity for something that can be easily resolved by a human simply checking for the orders -
Is there a way to set up an automated printing in PHP?
Psycho replied to eldan88's topic in PHP Coding Help
I'm fairly certain those functions only work on the server. So, unless you are hosting the site on a server in the restaurant they won't work. Trying to automatically print on a client browser would be a significant security risk. Could you imagine? Instead of all the popup advertising, all those internet advertisers would be trying to print to our printers. -
3.3m USD to build a website: what are your thoughts?
Psycho replied to peppericious's topic in Miscellaneous
I have to second what .josh stated. I used to work for a corporation where the core business was not technology. I was in a small company that built web products used by the other companies within the corporation. We had just over a dozen people and we were able to produce good quality and quantity. Now I work for a very large organization that specializes in software and we have hundreds of people working on many pieces of a suite of products. It can take weeks of meetings and designs to make a simple decision. -
OK, here is some sample code to get you started. Your original HTML markup that was being created was very bloated. If you want all the TDs in a table to use a specific class, there is no need to create a span tag around the contents in each cell with a class name. You can do it with a single line in the style sheet. Also, use the table header tag (<th>) instead of using additional markup (i.e. the bold tag). So, I took the liberty of removing a lot of the cruft. Below are two sample scripts. One to create the form with the input fields created with array names and a second script to process the form. Form script <?php include("connection.php"); $query = "SELECT title, subtext, nameid, category, price FROM starters"; $result= mysql_query($query); $formFields = ''; while($row = mysql_fetch_array($result)) { //Make values safe to use in HTML form $id = intval($row['nameid']); $title = htmlspecialchars($row['title'], ENT_QUOTES); $subtext = htmlspecialchars($row['subtext'], ENT_QUOTES); $category = htmlspecialchars($row['category'], ENT_QUOTES); $price = htmlspecialchars($row['price'], ENT_QUOTES); $formFields .= " <tr>\n"; $formFields .= " <td><input type=\"text\" name=\"title[$id]\" value=\"$title\"></td>\n"; $formFields .= " <td><input type=\"text\" name=\"subtext[$id]\" value=\"$subtext\"></td>\n"; $formFields .= " <td><input type=\"text\" name=\"category[$id]\" value=\"$category\"></td>\n"; $formFields .= " <td><input type=\"text\" name=\"price[$id]\" value=\"$price\"></td>\n"; $formFields .= " </tr>\n"; } mysql_close($con); ?> <form action="chstarterprice.php" method="post"> <br><br> <table>"; <tr> <th width="70px">Title</th> <th width="120px">Subtext</th> <th width="80px">Category</th> <th width="80px">Price</th> </tr> <?php echo $formFields; ?> </table> <input name="Submit" type="submit" value="Change" /></p> </form> Processing script: if(isset($_POST['title'])) { foreach($_POST['title'] as $id => $title) { $id = intval($id); $title = trim($title); $subtext = isset($_POST['title'][$id]) ? trim($_POST['title'][$id]) : ''; $category = isset($_POST['category'][$id]) ? trim($_POST['category'][$id]) : ''; $price = isset($_POST['price'][$id]) ? trim($_POST['price'][$id]) : ''; //Insert code to validate the data. //Are certain values required or must be of a certain type? // e.g. price would be expected to be a number //Preepare values for use in query $titleSQL = mysql_real_escape_string($title); $subtextSQL = mysql_real_escape_string($subtext); $categorySQL = mysql_real_escape_string($category); $priceSQL = floatval($price); //Create an run update query //NOTE: Running queiries in loops is very inefficient //if this is only for a relatively small number of records (e.g. 50) //this should be OK. $query = "UPDATE starters SET title = '$titleSQL', subtext = '$subtextSQL', category = '$categorySQL', price = '$priceSQL' WHERE nameid = $id"; $result = mysql_query($query); } }