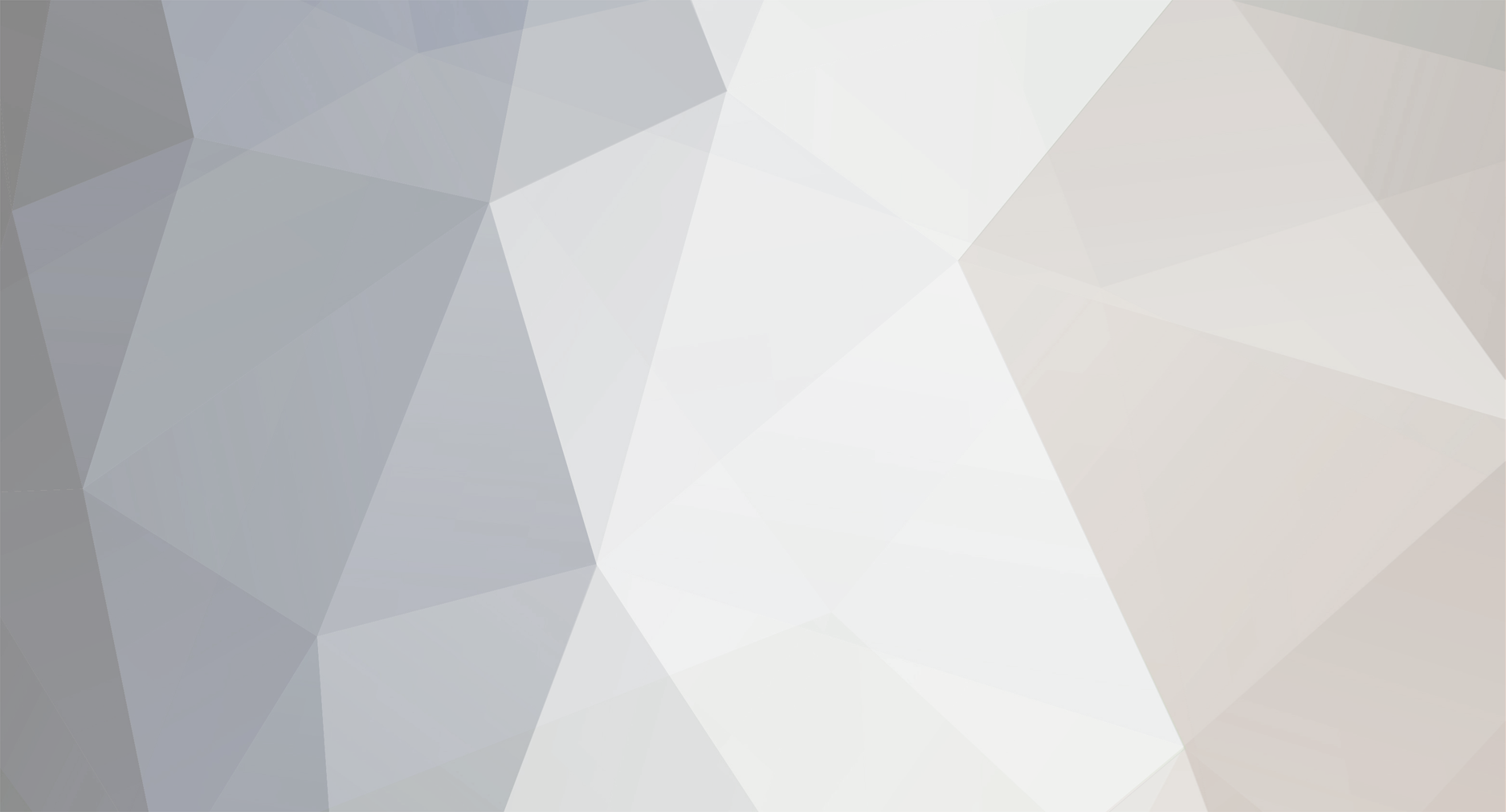
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Find file from folder with latest date modified stamp
Psycho replied to john_doemoor's topic in PHP Coding Help
It would be some pretty easy code to write. Do you have any experience with PHP? If not, then you should post in the freelance forum. If you do have some experience, give it a try then post back with any specific problems you have. Here is the process I would follow: 1. Create two variable to hold 1. the file namepath with the newest date and 2) the file's date. Set the first to false and the latter to 0. 2. Use glob() to get all the csv files from the specific directory into an array. 3. Loop through all files (using foreach) returned by glob. 4. Use filemtime() to get the last modified date of the file. 5. Test if that value is greater than the variable that holds the date of the recent file (created in step 1). If so, then set the two variables to the current file and date respectively. When the loop ends you will either have the most recent file and time in those two variables or (if there were no csv files) the file variable will be false. -
Lasha, This forum is intended for people to get help on code they have written. It is expected that the person asking for help has the basic knowledge necessary to take advice given and implement it or only needs very specific help. The probable reason no one has responded is that, based on your comments, you don't have the necessary knowledge to implement an appropriate solution and we would either have to write it all for you or this thread would turn in to a long tutorial. Having said that I will at least provide some guidance: 1. Remove the cat_id field from the table. 2. Create a new table called categories with two fields: cat_id (auto_increment) and cat_name 3. Create a new table called user_cats with two field: user_id and cat_id 4. When creating the form, run a query on the category table and create the checkboxes using something like this while($row = mysql_fetch_assoc($result)) { echo "<input type='checkbox' name='cat_ids[]' value='{$row['cat_id']}'> {$row['cat_name']}<br>\n"; } 5. When the user submits the form you will create the record as I assume you are already doing for all the fields that are in that table. After running the insert query use mysql_insert_id() or mysqli_insert_id() to get the auto-increment id that was created for that record (i.e the user_id). 6. Using the user_id from the previous step and the submitted categories, create an insert statement to create the records in the user_cats table. Example code: $values = array(); foeach($_POST['cat_ids'] as $cat_id) { $cat_id = int_val($cat_id)); $values[] = "('$user_id', '$cat_id')"; } $query = "INSERT INTO user_cats (user_id, cat_id) VALUES " . implode(', ', $values); $result = mysql_query($query); Then, when you need to get the user's data along with their categories you can run a query such as this SELECT user.name, user.surname, user.facebook_link, categories.name FROM users LEFT JOIN user_cats ON user.user_id = user_cats.user_id LEFT JOIN categories ON user_cats.cat_id = categories.cat_id
-
this was offered to me elsewhere and so ive now put the captcha_answer in a SESSION variable. but youre saying there could be problems with that? how so? The main problem that comes to mind is concurrency. A user could have two windows open for your form at tone time. However, only the last value would have been saved to session. So, if the user submits the first page their response would fail. I'd actually go with using a hash. When creating the form determine the answer. Create a hash of that answer and populate that into a hidden field. Then when the for is submitted, take the response and hash it in the same was as you did for the answer. If the two are the same you pass the validation.
-
Well, your captha is What do you mean "despite" the captcha? Have you verified that the responses are not entering the correct response to the captcha and are getting processed anyway or are you saying that these submissions are entering the captcha and have content you don't want. If the responses are not entering the correct captcha response you need to look for loopholes in the system. For example, I see that you are having the captcha response AND the answer included in the POST data. That's kinda worthless since you cannot control what is subitted in the POST data. Even if you have a hidden field with a value you set, it is child's play to modify that value when the form is submitted. It would be very easy for someone to create a custom form submission that would bypass that validation by simply submitting the same values for those two fields. You should NOT include the answer in the form - at least not directly. you could store it in a session value, but there are some problems with that. Or you could store a hash of the solution in the form. Then compare a hash of the answer against the answer value. But, if the answers are getting submitted it could be a human that is entering the captcha and there is no good way to prevent that except through an approval process, blocking IPs, etc. etc. Or it could be a script that is reading the form to calculate the response. Either someone built one specifically for your site or it is one that has some "intelligence" built in to deduce what you are doing.
-
Correction: It seems like natcasesort () does indeed do what you want, as evidenced by this result. (Though, upon thinking about it, I really should have realized it right away. ) . . . However, do not that it does not change the keys for the values so a for () loop would yield the unsorted result. You'll need to use foreach (). You do realize that he is dealing with a multidimensional array, right? natcasesort() only works on one-dimensional array. I already provided a solution back on page two but apparently some chose to ignore it. Query the records and put into an array using the file name as the key. Then use ksort() with the SORT_NATURAL flag.
-
For an alphanumerical sort - yes they are in the right order. I already provided you a solution to get them sorted in a natural order, as you are requesting. You will just have to do it using PHP code after you query the results.
-
@Barand, I'm thinking that table already exists and the OP is not aware of that @not_sure, why did you omit the one line that may actually help us? Without seeing your DB structure or the query in question it is impossible to say. But, my guess would be that the numerical references are foreign key references to an associative table where the actual State name or abbreviations are stored. If that's the case the query, which you deemed irrelevant, would need to be modified to JOIN onto the other table to get the results you want. I would be curious to knwo the actual name of the field that holds the value you are currently getting.
-
You don't, you have another table with relations of products to categories. To build upon what Jesirose has stated, your tables might look something like this: Categories: cat_id | cat_name 1 Cat 1 2 Cat 2 3 Cat 3 Users user_id | name 1 Adam 2 Bob 3 Carl user_cats (this associates users with one or more categories) user_id | cat_id 1 2 2 1 2 3 3 1 3 2 3 3 So, from the above data you can see that: User Adam is associated with Category 1 User Bob is associated with categories 1 & 3 User Carl is associated with categories 1, 2 & 3
-
I don't believe MySQL has a natural sorting capability. So, you might need to query the records, dump into an array and then sort using PHP's natural sorting capability $query = "SELECT * FROM files WHERE `prod_id` = '{$this->curProduct}'"; $result = mysql_query($query); $data = array(); while($row = mysql_fetch_assoc($result)) { $data[$row['file_name']] = $row; } //Perform natural sort on array key ksort($data, SORT_NATURAL); print_r($data);
-
I take that back, I don't think you can use a datetime to auto set with creation. So, a timestamp is still the solution I would use.
-
Not true, a timestamp field is the only one that *can* be configured to be updated with any change of the record, but you don't have to. You can just set it up to only populate the timestamp when the record is created. Set at creation and updates `topics_date` timestamp NOT NULL default CURRENT_TIMESTAMP on update CURRENT_TIMESTAMP, Set only on creation `topics_date` timestamp NOT NULL default CURRENT_TIMESTAMP, But, yeah, you could also use a datetime field if the need is only to set the value on record creation.
-
The solution is not a php one. you can set up the default value to be the Current Timestamp. As for only having the data you can use a Date type instead of a Datetime or Timestamp. but it will store it as YYYY-MM-DD. But, that is fine you should not be using the date values directly from the database anyhow. You'll almost always want to conver the date to a format that you specify using the PHP date() function OR you can have the date formatted to your liking within the query using similar functions in MySQL Personally I would use a timestamp anyhow - you can always just use the date part of the value.
-
Hmm, I wouldn't use regular expression at all. It seems you only want files anyway, right? Are you aware that your regex (if it did work) would allow folders if they had names that ended in '.pdf' for example? I'd use glob() along with is_file() and check the extension against an allowed array. [removed some lines for brevity] $dir = "/sync/www/html/ohx/training"; $allowedTypes = array('pdf', 'xlsx', 'xls', 'doc', 'docx'); foreach(glob($dir.'/*') as $filePath) { # Eliminate directories if(!is_file($filePath)) { continue; } # Eliminate disallowed filed $fileInfo = pathinfo($filePath); if(!in_array($fileInfo['extension'], $allowedTypes)) { continue; }
-
This topic has been moved to PHP Freelancing. http://forums.phpfreaks.com/index.php?topic=364897.0
-
OK, so what values are entered into all of your variables and which branch of logic would you expect to occur? You have a lot of condition checks in there, so without knowing what the incoming values are I can't say what it should or shouldn't do. But, if that message is displayed that tells me that the very first condition if($_GET['m'] == "link") is not met, because the block of code associated with that condition ends with an exit command.
-
After looking at the code a little closer some things don't make sense. if($_GET['m'] == "link") { $query = mysql_query("SELECT * FROM organisermembers WHERE userid = '".mysql_real_escape_string($_GET['i'])."' LIMIT 1"); You first check if the variable 'm' was set to a particular value then use the value for the variable 'i' in your query. You should really use more descriptive variable names because I don't see why you don't just check if 'i' is set. mysql_query("UPDATE organisermembers SET password = '".mysql_real_escape_string(md5($password))."' WHERE userid = '".$row['userid']."'"); It's useless to use mysql_real_escape_string() on a hash. $r = md5($row['companyname'].$row['password']); if($r == $_GET['r']) { You are taking two values from the database, hashing them, and then seeing if they match a value that was sent in the POST data. Is that a hidden field value that you set? Because I can't believe a user would know what that hash value should be. $password = trim($_POST['password']); if(!isset($password) || empty($password)) { Why are you checking if $password is set right after you just set it? You should be checking if the POST value was set. There's a lot of logic that I cannot make sense of because I don't know what the variables represent or what they would be expected to contain. It could be that the values you think are being passed are not what you expect or don't exist. I would suggest changing the logic from if(!error_condition_1) { if(!error_condition_2) { //Do somthing } else { //error message 2 } } else { //error message 1 } To something like: if(error_condition_1) { //error message 1 } elseif(error_condition_2) { //error message 2 } else { //Do something } Makes it much easier to follow
-
What problems are you experiencing? Errors anything?
-
No, that should not be an issue based upon the values you have (it might with accented characters based upon the collation used on your table). You need to provide exact details for us to help. Please provide the exact query you are using and a var_dump() of the results. If the results are too numerous to post, then provide a subset for us to view.
-
White-spaces have some idiosyncrasies when displayed in a browser which is why you need to verify the content of variables accordingly. If you aren't going to use var_dump or something like echo "[" . $var . "]"; you definitely need to be looking at the source of the HTML and not the display. So, having said all that you should do two things: 1. You should almost always trim data before storing it. It should be standard procedure and you should only NOT trim when you have made a conscious decision that it makes sense not to. So, any code you have now that creates those records you should go back an implement appropriate trim()'s. In fact, since you aren't trimming you might have some validations that are broken. E.g. if you are testing if a field was empty using $_POST['var'] == '' or empty($_POST['var']), etc. that would allow the value to pass with just spaces entered. So, do your trim before any validations. 2. Once you know that any new values entered into the DB will be appropriately trimmed you should go back and run a single query to trim any existing values using the MySQL function of the same name as used in PHP.
-
The problem is most likely as suggested earlier - there are probably white-space characters before some of the values that is causing the problem. You won't see that unless you look at the source code and if you copied/pasted this from the browser (and not the code) it isn't apparent from what you just posted. Do a var_dump() on the results and post the output here. $query = "SELECT * FROM files WHERE `prod_id` = '". $this->curProduct ."' ORDER BY `file_name` ASC"; $files = $wpdb->get_results($query, ARRAY_A); var_dump($files);
-
My apologies if I offended you, but I believe the whole reason you are having to post so many topics is the fact that you are chasing the wrong solution. Several people have tried to provide the same guidance, yet it seems to fall on deaf ears. That is the problem! You are making decisions based upon your preconceived assumptions and not based upon actual understanding or experience. Just because you are JOINing many tables with a complicated structure does not mean you can't also limit and order the results as you need them. This is infinitely more efficient than running multiple queries. It sounds like you really are making this more difficult than it needs to be. What I see you doing is making the same mistakes I see a lot of people using when they first start. We're trying to help you by learning the right way to do it instead of ingraining bad habits. But, I will state that learning to really use a database is not a trivial task. So, I understand your inclination to continue with what makes sense to you rather than trying to learn some new skills that might be a little intimidating. When you post a help request you have already decided upon a specific process which may be flawed. But, your request is so specific to the point in the process that you are stuck on we can only reply along the lines of "you're doing it wrong" and perhaps throw you a bone about using a single query. On the other hand, if you were to provide more information about the data structure you have and what you are trying to get from that data we could, perhaps, provide a much better solution. Again, the concept is flawed. You shouldn't need to do one big query and store the data into an array to use later. You query for the data you need when you need it. In fact, in most cases you shouldn't even need to store DB results in an array. You should only need to process the data ONE TIME when you extract the records from the result. But, that should not be taken to the extreme. If you were going to display different tables of data for each position, for example, you would still probably do only one query and then create the multiple tables as you process the data. So, if you want some real help, let's start over. If you can provide 1) The table structures that you have, 2) Explain what data you are wanting to display, and 3) The code you are currently using to get the data - then I will try to provide a query/process to meet your needs.
-
Then you ushould do ONE query using appropriate JOINs, but I think you were given that advice previously and ignored it as well. So, if you are going to plow ahead with this method in the face of multiple assertions as to why it is a terrible idea, then I would say you should use usort() instead of array_multisort() it is much easier and much more flexible IMHO: //Custom function to use with usort() function sortPlayers($a, $b) { //Position ID is not the same, sort on that value if($a['positionid'] != $b['positionid']) { return $a['positionid'] - $b['positionid']; } //Ranking is not the same, sort on that value if($a['ranking'] != $b['ranking']) { return $a['ranking'] - $b['ranking']; } //PositionID and ranking are the same - no sort pref return 0; } usort($fullrosterafter, 'sortPlayers'); You may need to reverse the $a['index'] - $b['index'] to get the order you want them sorted in.
-
Didn't we go through this already in one of your other posts? You should do the sorting using your query rather than processing the data multiple times. $sql = "SELECT * FROM players ORDER BY positionid, ranking"; Also, if you are not going to use ALL of those fields in the output do not use '*' in your SELECT and instead list out the fields you will use.
-
Can you show the directory structure and where - exactly - you are storing the files? Are the folders with the files in directories below the root? If not, you cannot allow the user to access them directly. But, there are solutions. So, please show the folders with the files in relation to the root and show what one of the URLs to the files that you output are.
-
Younger? Not necessarily. But, no one is saying you can't or shouldn't store the images in the DB, only that saying it is more secure is a subjective statement. It is quite possible to store images in the DB that would be less secure than storing them as files. But, this question got way off base because I don't believe the OP ever mentioned anything about these being sensitive images. There are benefits and drawbacks to storing images in either the DB or in the file system. Each problem requires appropriate analysis to determine the best solution. FYI: Regarding the reference to "Post #17", I think you misunderstood. There is no post #17 on that page. At least not one that stands out. The numbers to the left of the posts is the number of "Votes" the post received. The comment was just a clarification/confirmation.