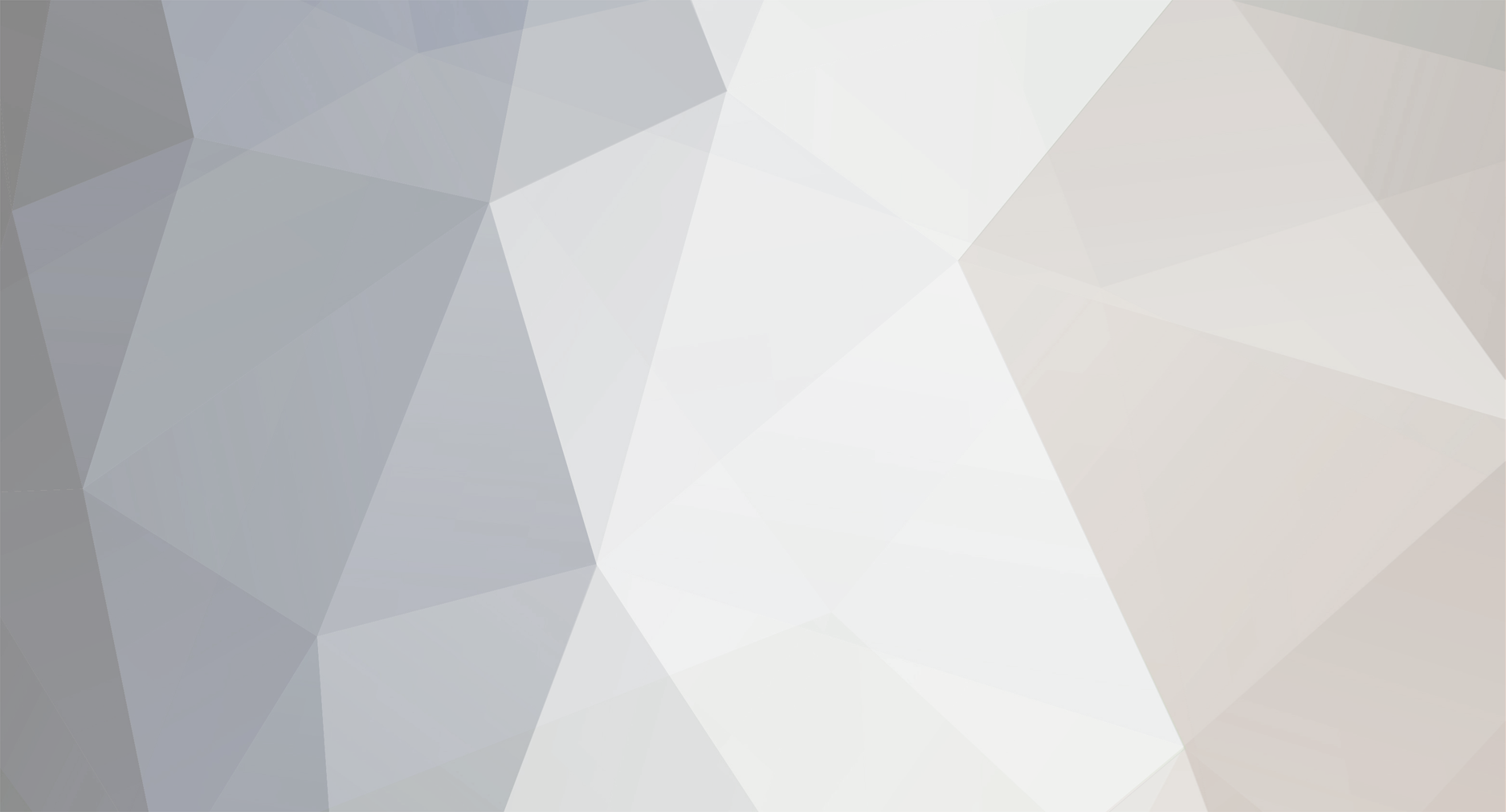
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Strange problem with post data not showing
Psycho replied to Skylight_lady's topic in Javascript Help
Right, the AJAX query is pulling cached data as I stated in my first post. You can either add a random parameter to the URL of the AJAX query as I suggested or research the other solution that is available. Here are a couple of links on the issue (I just googled them just now - I did not read them) http://www.codecouch.com/2009/01/how-to-stop-internet-explorer-from-caching-ajax-requests/ http://ajaxian.com/archives/ajax-ie-caching-issue http://weblogs.asp.net/pleloup/archive/2006/06/08/451583.aspx -
You first need to get the saved value from the database. Then simply add a "switch" to detemine if an option should be selected or not and add selected="selected" to the option. In this example code the variable $selectedValue is assumed to be the selected value you extracted from the DB <?php $result = mysql_query("SELECT Salutation FROM salutations"); $options = ''; while ($row = mysql_fetch_assoc($result)) { $selected = ($row['Salutation']==$selectedValue) ? ' selected="selected"' : ''; $options .= "<option value=\"{$row['Salutation']}\"{$selected}>{$row['Salutation']}</option>\n"; } ?> <select name="Salutation_1"> <option>< select salutation ></option> <?php echo $options ?> </select>
-
str_replace() would be much more efficient for a simple replacement such as this $text = str_replace("Product", "<br />\nProduct", $text); But why is all that text in a single database field. You should have each of those pieces of data in unique fields.
-
Strange problem with post data not showing
Psycho replied to Skylight_lady's topic in Javascript Help
Alert the response back from the AJAX call. Is it what you expect? If not, then it is probably a caching issue. Otherwise, it is probably a problem with populating the response in the existing DIV if (req) { req.onreadystatechange = function() { if (req.readyState == 4) { // only if "OK" if (req.status == 200) { //ADD THIS NEXT LINE alert(req.responseText); document.getElementById('userDataEdit').innerHTML=req.responseText; document.getElementById('userDataEdit').style.display = 'inline'; document.getElementById('userDataList').style.display = 'none'; } else { alert("There was a problem while using XMLHTTP:\n" + req.statusText); } } }; req.open("GET", strURL, true); req.send(null); } -
Um, yeah, I "mentioned" it that way because that is the correct terminology. From the manual:
-
Any problem with writing a cookie with ASP/VB but deleting with PHP?
Psycho replied to benphp's topic in PHP Coding Help
Well according to the PHP manual for cookies So, I'm guessing the browser has to be closed before the cookie gets unset? EDIT: Also in the manual So, if the aspx process is setting any of the optional parameters automatically you would need to include those in the call trying to unset the cookie. Also, in the line where you try to delete the cookie try setting the value to boolean false instead of NULL as there is another refernce that using boolean false can also delete a cookie. -
Here is a rewrite of that entire script. I added logic to clean/parse the data and validate that there is something (at least 1 character) in the values. I also re-organized the logic so you don't need any ELSE statements by putting the error conditions first. If anything fails it takes appropriate action and exits the script. It may be a little more verbose, but definitely more intuitive. Lastly, I commented out all the header redirect lines and inserted echo statements to state the result. Once you get the correct results you can remove the echo statements and uncomment the header() functions. <?php session_start(); require("db_connect.php"); //Parse user input $username = mysql_real_escape_string(trim($_POST['uname'])); $password = mysql_real_escape_string(trim($_POST['pword'])); $npass = mysql_real_escape_string(trim($_POST['nPass'])); $cnpass = mysql_real_escape_string(trim($_POST['cnPass'])); //Verify all required fileds are entered //NOTE: Should also test for minimum field lengths / valid data etc if(empty($username) || empty($password) || empty($npass) || empty($cnpass)) { //Not all required fields have values $_SESSION['error1']= "Missing required fields"; echo "Missing required fields"; // header("Location: change_pass.php"); die(); } //Create and run query to authenticate user $sql = "SELECT * FROM login_details WHERE username='$username' AND password='$password'"; $result = mysql_query($sql, $connect); //Check if query failed if(!$result) { //Query failed $_SESSION['error1']= "There was a problem running the authentication query"; echo "There was a problem running the authentication query"; // header("Location: change_pass.php"); die(); } //Check if there was a match if (mysql_num_rows($results) == 0) { //No match for username password $_SESSION['error1']= "Icorrect Username Or Password"; echo "Icorrect Username Or Password"; // header("Location: change_pass.php"); die(); } //Check that new passwords match and are valid if ($npass != $cnpass) { $_SESSION['error2']= "Passwords Do Not Match"; echo "Passwords Do Not Match"; // header("Location: change_pass.php"); die(); } //Create/run query to change password $sql = "UPDATE login_details SET password='$npass' WHERE username='$username'"; $result = mysql_query($sql, $connect); //Check if query failed if(!$result) { //Query failed $_SESSION['error1']= "There was a problem running the update query"; echo "There was a problem running the update query"; // header("Location: change_pass.php"); die(); } //Password was updated successfully echo "Password was updated successfully"; //header("Location: secure_page.php"); die(); ?>
-
Well, let's see what some of the problems are: 1. You don't validate any of the user input (e.g. trim the data, don't allow empty values, ensure there are values for the new password, etc) 2. You don't escape the input for the database leaving yourself open for sql injection 3. You don't hash the password which can compromise the users 4. You have your IF/ELSE conditions backwards 5. You create a query to update the password - but you never run it. Also, both results of the page do a redirect. That's not necessarily a problem (assuming you actually completed everything you should before doing so). But, the fact that you get a page that never loads, it is quite possible that you have an infinite loop problem. Look at the two pages that get redirected to, do either of those redirect to other pages or back to the one above?
-
Strange problem with post data not showing
Psycho replied to Skylight_lady's topic in Javascript Help
The DOM solution will only work if the problem is due to the client side JavaScript display code. If it is a caching problem the DOM "solution" will not work. You need to identify the cause of the problem and then implement the correct solution. To determine if it is a caching problem you can have the javascript alert() the response back from the AJAX call. If that response is the same every time when it should be different - then it is a caching problem. If the data returned back from the AJAX call is correct - but is not getting displayed in the DIV, then it is a DOM issue. -
This topic has been moved to JavaScript Help. http://www.phpfreaks.com/forums/index.php?topic=325313.0
-
Strange problem with post data not showing
Psycho replied to Skylight_lady's topic in Javascript Help
I'm ot an AJAX expert by any means, But, I recall there is a well know problem in IE that when you make a call via AJAX if the query string has not changed you will get back the exact same results every time because IE caches the results. WHen I encountered this in the past I worked around it by adding a random value to the AJAX request. That forces IE to get fresh data. For example, I think strURL is the URL you are passing through AJAX. Just add something like this to add a timestamp to the end of the url strURL += 'timestamp='+getTime() I recall reading something a while ago that had a more elegant solution. So, you might want to do some more research into it. But, the above should be very easy to try EDIT: This is probably either a JavaScript or AJAX problem. Moving to JS forum for now. -
On each iteratin of the loop, you would only increment the one matching the result of the roll.
-
There is no reason to define $side1, $side2, etc. There is no purpose for them - as you have them now. SO, you have a for() loop that runs 5,000 times and generates a random number between 1 and 6. So, based upon your statements I don't think you want to display the results of each roll. Instead you need to store the results of each roll then display a table showing the results. So, your next step is to store the results of rand(). You could use $side1, $side2, etc. to store how many times each side is rolled. But, then you have to create multiple IF/ELSE statements or a switch. That's too much work. Just create an array called $results. Then use the index of the array for each possible result (i.e. $result[1] is for when a 1 is rolled). Increase the value for the appropriate index by 1 based the value of the roll. $results[$roll ]++; Then when you are complete with the for() loop you have an array something like this array( 1 => 785, 2 => 924, 3 => 1022, 4 => 852, 5 => 935, 6 => 482 ) You can then calculate how often a particular number was rolled and display the results.
-
Well, the answer to your problem is pretty simple: $UserName and $Password are either not set or have values that result in empty strings. You need to check that you don't have a typo in the variable names and that the values are getting properly set. As for debugging techniques, that is a complex conversation that doesn't fit into a forum post. But, I typically like to have a switch to turn debugging on/off. That way I can leave all my debugging code in place when my pages go to production and just turn the debugging mode off (you can even make it so you can turn on debug mode on a user-by-user basis if you have to trace down a problem only occuring in production). So, instead of using echo, I will create a function with the text to display in debug mode. You can even pass the line number to make it easier to trace down where the problem occured. Example //Set the debug mode. You can do this statically in the file //Or dynamically by username, IP address, $_GET parameters, etc. $_SESSION['debug'] = true; function debug($msg, $lineNo) { if($_SESSION['debug']!=true) { return false; } echo "Debug [{$lineNo}]: {$msg}<br />\n"; return; } //Usage $query = sprintf("INSERT INTO Users (UserName, Password) VALUES ('%s', '%s')", mysql_real_escape_string($UserName), mysql_real_escape_string($Password)); debug("Query: $query", __LINE__); That is just a rudimentary example. But, by using a function to handle the debugging you can make the process more elaborate without having to change all the lines where you have debugging. For example, you can have the debug function store all the degug info and echo them all at the end of the page since echoing them at the point where they occur could cause problems with the HTML output. Or, you could echo the debug messages as HTML comments, write to a log file, etc. The possibilities are endless.
-
Ok, the root of the problem is that the $whenClauses array doesn't have any values. So, just start at the beginning and work through each process that could affect those values. Step 1: Reading the file when you read the file, with file(), and echo the results with //Read input file into an array $data = file($org); echo $data. "<br />"; You are getting Array If it wasn't reading the file the function file() will return false. So, the fact that $data is an array means that it is succesfully reading the file. Instead of echo, you should use print_r() to check the contents of the array. So, since the file is being read into an array it could be that it is an empty array, but assuming it is not then we need to check the next step. Step 2: Validating the lines (records) So, looking further on, there is the block of code to reject any lines (i.e. records) that do not consist of three values after being exploded. It could be that there is an extra tab character or something else that is causing all the lines to be rejected. You should add some debugging code to check that. Step 3: Assuming the validation is not rejecting valid records the next block of code is used to trim the values and add the values to the arrays $barCodes and $whenClauses. Since those array elements will get created even if the record values have empty strings AND $whenClauses has some static text we can be certain that these lines are not being run - otherwise the query created with those arrays would have some of that data. The only reason the query would not have any of that data is if there was a typo between the variable names - and that is not the case. So, I have to assume that either the file is not being read correctly OR the validation is rejecting the records. Try running the following code with some additional debugging added to help determine where the problem is. <?php //Create DEBUG switch and function $debug = true; function debug($msg) { global $debug; if(!$debug) { return false; } echo "<b>Debug</b>: {$msg}<br />\n"; return; } //Define input file / variabelen vastleggen $org = "/full/path/to/the/file/public_html/splash/sd-voorraad-edit.txt"; // Connectie met mysql $host = 'localhost'; $user = 'username'; $pass = 'password'; $db = 'dbname'; $dbconnect = mysql_connect($host, $user, $pass); if (!$dbconnect) { die('Could not connect: ' . mysql_error()); } //Read input file into an array $data = file($org); //Add debug message to check file read if(!$data) { debug("Unable to read data file {$org}"); } else { $numberOfLines = count($data); debug("Data file read succesfully with {$numberOfLines} lines.", ); } //Create temp arrays for query $barCodes = array(); $whenClauses = array(); //Process the data foreach($data as $lineNo => $line) { //Parse line $partRecord = explode("\t", $line); //Check that it is a valid record if(count($partRecord) != 3) { debug("Line $lineNo has ".count($partRecord)." elements. NOT VALID<br />\n'{$line}'"); //Not a valid record, do not process //Proceed to next record continue; } else { debug("Line $lineNo has ".count($partRecord)." elements. VALID<br />\n'{$line}'"); } //Record appears valid, continue with processing //Remove any leading/trailing white-space in values array_map('trim', $partRecord); //Populate data array into descriptive variables // to make processing code more intuitive list($barcode, $qty, $price) = $partRecord; //Add data to temp arrays $barCodes[] = $barcode; $whenClauses[] = " WHEN {$barcode} THEN {$qty}"; } //Create the single query to update ALL records $query = "UPDATE {$db}.products SET voorraad = CASE barcode " . implode('', $whenClauses) . " END WHERE barcode IN (" . implode(',', $barCodes) . ")"; echo $query; ?>
-
Yes, there are "many" ways, but that ^^ is not one of the "right" ways. $stringOfIDs = implode(',', $arrayOfIDs); $query = "SELECT * FROM [table_name] WHERE id IN {$stringOfIDs})";
-
query2 = "INSERT INTO jobnumbers (jobnumber) VALUES ('$jobnum') WHERE jobid='$id' "; INSERTS don't use a WHERE clause. I think you meant to do an UPDATE of the query you ran to initially insert the record. But, YOU DON'T NEED TO RUN THE SECOND QUERY! If the Job Number will always be the Category and the Job Number, then you don't need another field to add that concatenated value. It is a waste. When you need to look up a job using the complete job number, just split the value based on the dash and look up the job using something such as SELECT * FROM jobnumbers WHERE category='$cat' AND id='$id'
-
Shouldn't that be a dollar sign and not an ampersand??? Have you at least tried it? As I said the performance will increase greatly. Not, 10%, 20%, or 50%, but more like 1,000 - probably more, especially if you have many records.
-
So, a user can only have one seat? That would seem to be the case based upon your code. Oh well, denno020 has the right idea, but I think the below would be more efficient. Plus that code will not work as written sice it is trying to run queries before connecting to the DB. I also added code to prevent sql injection <?php session_start(); /* connect to data base */ require ('./secure/connect.php'); /* get user id */ $user_id = mysql_real_escape_string(trim($_SESSION['username'])); /* get the seat number */ $seat_id = mysql_real_escape_string(trim($_GET['seat'])); //Check if seat is already taken $query = "SELECT taken FROM seats_table WHERE seat_id = $seat_id AND taken = TRUE"; $result = mysql_query($query, $myConnection) or die (mysql_error()); //Check if seat is taken if(mysql_num_rows($result)>0) { echo "That seat is taken, please go back and select another"; exit(); //Could also do a header to redirect to appropritate page } /* create query */ $query = "UPDATE seats_table set taken = FALSE, user_id = '0' WHERE user_id = '$user_id'"; $result = mysql_query($query) or die (mysql_error()); $query = "UPDATE seats_table set taken = TRUE, user_id = '$user_id' WHERE id = '$seat_id'"; $result = mysql_query($query) or die (mysql_error()); /* $query3 = "UPDATE users set signed_up = '3' WHERE username = '$user_id'"; */ /* $result3 = mysql_query($query3); */ /* advise user their seat has been reserved */ include 'seating.php'; ?>
-
OK, you can create the next ID for the unique category with a single query. Still, you wouldn't let the auto-increment do it. Here is how: INSERT INTO `job_num` (`id`, `category`, `name`) VALUES ( (SELECT MAX(id)+1 FROM `job_num` WHERE `category` = '$category' GROUP BY `category`), '$category', '$name')
-
Ok, I just tested that and it doesn't work the way I think you are stating. It does allow for duplicate IDs with records that have different names. But the auto-increment is still "global" - i.e. the value will be 1 greater than the last auto-increment value regardless of the secondary primary key. The combinated primary key just allows the same "key" value to be used mutliple times as long as different secondary primary values are used - but you have to do so manually. The auto-increment parameter will not do it automatically. Using your table example above (note third record) INSERT INTO job_num ('category', 'name') VALUES ('A', 'some text'), ('A', 'some text'), ('B', 'some text'), ('A', 'some text') Assuming these are the first four records, the auto-increment ID values will be 1, 2, 3, 4. Not, 1, 2, 1, 3 Still, this is a viable approach, but getting the next ID for each category will require a two-step process to query the DB for the highest current ID of the category, increment the value by 1, then insert the new record specifying the ID (i.e. don't let auto-increment do it).
-
Never used that before. Good to know. Do you know how to set that property for a table in phpmyadmin after the table is created?
-
I made the comment previously I have no idea what you are setting the value as. If you are setting the value as the boolean true/false then trying to compare with the string values of 'true'/'false' is not correct. Plus, you have TWO conditions on that IF statement. The second is a function call. I have no idea what is being returned from that function call and whether it is what you expect or not. Put these line right before that last IF statement. To see what is being compared: echo "ACCOUNT_COMPANY: "; var_dump(ACCOUNT_COMPANY); echo "<br />\ntep_not_null(company_tax_id): "; var_dump(tep_not_null($company_tax_id));
-
You stated you wanted separate job number lists for each category, but your code shows one table. If you only want to maintain one list (which will be the easiest solution) then just set up the ID field to be an auto-increment field and set the type as a separate field. Then don't specify a job ID when inserting and the DB will create it for you. Very easy solution as the database will do all the work for you. Below is some sample code (I also moved the logic for parsing the post data and connecting to the database. There is no need to do that if nothing was posted since you aren't going to do anything with it) <?php if(isset($_POST['submit'])) { // Connect to server and select database. $host = ""; // Host name $username = ""; // Mysql username $password = ""; // Mysql password $db_name = ""; // Database name mysql_connect("$host", "$username", "$password")or die("cannot connect"); mysql_select_db("$db_name")or die("cannot select DB"); //Parse & escape POSTed values $category = mysql_real_escape_string(trim($_POST['category'])); $name = mysql_real_escape_string(trim($_POST['project-name'])); //Create & Run insert query $query = "INSERT INTO job-numbers (category, name) VALUES ('$category', '$name')"; $result = mysql_query($query); if (!$result) { //Query failed, display error echo"Error: <br />\n" . mysql_error() . "<br />\nQuery:<br />\n{$query}\n";; } else { //Display job id of inserted record. $id = mysql_insert_id(); $cat = htmlspecialchars((trim($_POST['category'])); echo"The record {$cat}-{$id} was created."; } } ?> <html> <body> <form action="#" method="post"> <select id="category" name="category"> <option>Web</option> <option>Print</option> </select> <input type="text" name="project-name" id="project-name" /> <input type="submit" name="submit" id="submit" /> </form> </body> </html> If you do need separate consequtive numbers for each category it can be done, but will be more complex