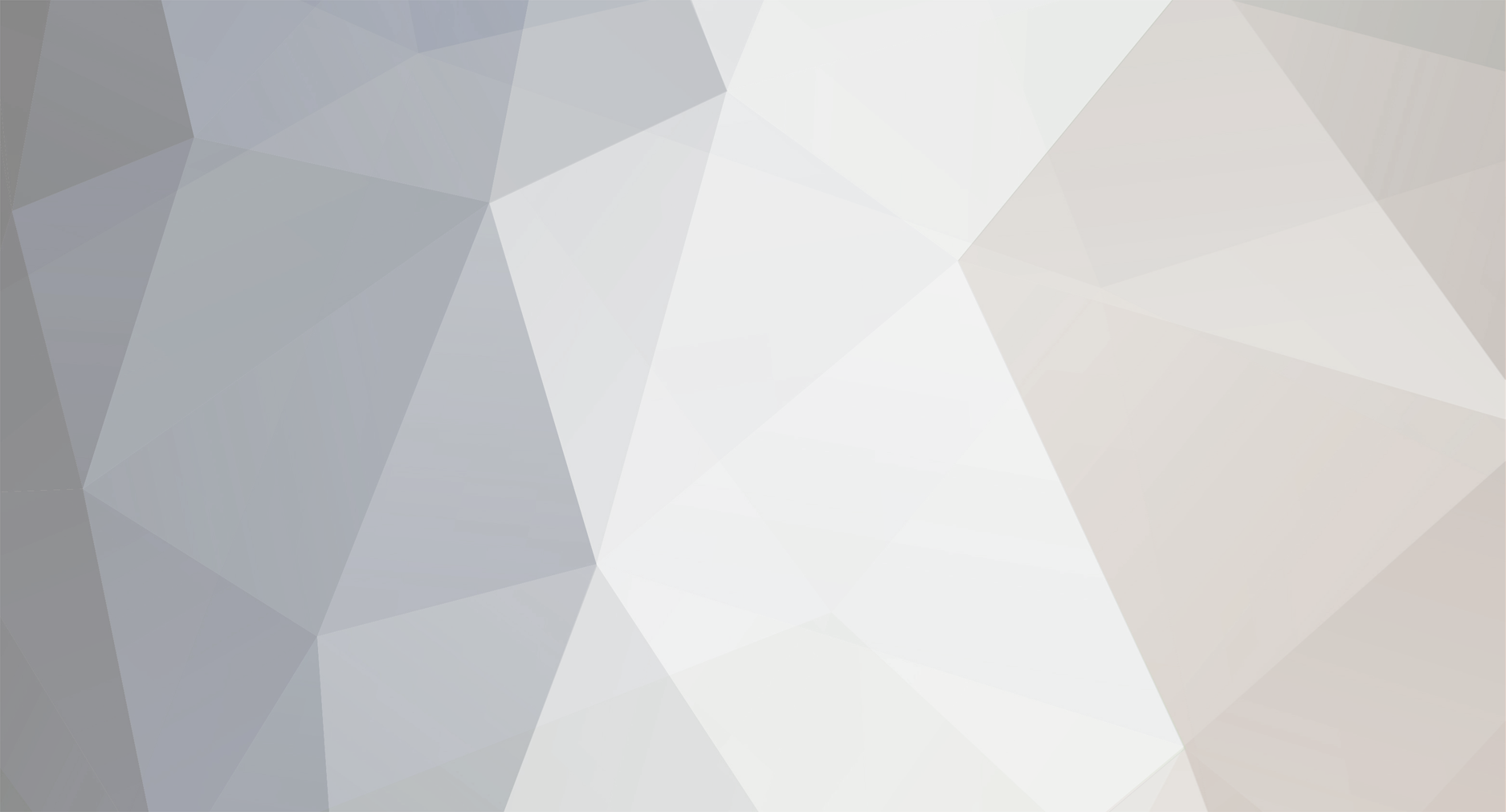
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I mean a completely different approach. It doesn't make sense, to me at least, to loop through consecutive numbers to try and find the number to generate the rounded result. Plus, why calculate the solution, test if the solution matches teh round, then IF the solution doesn't match the rounded solution run a function. Just use the function to do everything. To be honest though, I don't see how that function is supposed to work for anything other than division. Assuming the input numbers are whole numbers the result for addition, subtraction, and multiplication would always be whole numbers as well. Only divisioin would result in decimal numbers. By the way, your function is called round_up, but you are only doing a simple round, which means it could be rounded down. If you want to get the rounded up value you should use ceil(). Here is a simple solution that handles division operation. I would do the others, but as I said, I don't see how that would ever be applicable function returnExactRoundUp($x1, $x2, $op) { switch($op) { case '/': if(($x1/$x2)<ceil($x1/$x2)) { $x1 = $x2 * ceil($x1/$x2); } $result = ceil($x1/$x2); break; } return array('num1' => $x1, 'num2' => $x2, 'op' => $op, 'solution' => $result); } $num1 = 3; $num2 = 5; $op = '/'; $result = returnExactRoundUp($num1, $num2, $op); print_r($result);
-
<?php //Dummy array for testing $list_array = range(1, 500); //Define how many records you want on a page $records_per_page = 20; //Cacluate total records and total pages $total_records = count($list_array); $total_pages = ceil($total_records/$records_per_page); //Get/define current page $current_page = (int) $_GET['page']; if($current_page<1 || $current_page>$total_pages) { $current_page = 1; } //Get records for the current page $records = array_splice($list_array, ($current_page-1)*$records_per_page, $records_per_page); //Create ouput for the records of the current page $list_ouput = ''; foreach($records as $value) { $list_ouput .= "<li>{$value}</li>\n"; } //Create pagination links $first = "First"; $prev = "Prev"; $next = "Next"; $last = "Last"; if($current_page>1) { $prevPage = $current_page - 1; $first = "<a href=\"test.php?page=1\">First</a>"; $prev = "<a href=\"test.php?page={$prevPage}\">Prev</a>"; } if($current_page<$total_pages) { $nextPage = $current_page + 1; $next = "<a href=\"test.php?page={$nextPage}\">Next</a>"; $last = "<a href=\"test.php?page={$total_pages}\">Last</a>"; } ?> <html> <body> <h2>Here are the records for page <?php echo $current_page; ?></h2> <ul> <?php echo $list_ouput; ?> </ul> Page <?php echo $current_page; ?> of <?php echo $total_pages; ?> <br /> <?php echo "{$first} | {$prev} | {$next} | {$last}"; ?> </body> </html>
-
Please show your code for processing and inserting the text into the database and the code for selecting the text from the DB and outputting to the browser. Also, what collation are you using for the database field?
-
You defintely need to take a different approach - that is going to be a headache. But, your problem is the fact that you are using a for loop and increasing $x1 in the third parameter of that loop. From the manual At the end of each iteration, expr3 is evaluated (executed). So, on the last iteration of the loop where the condition $sol < round($sol) is no longer true, the third expression ($x1++) is executed again - increasing the value one more than you intended. You should use a while() loop and increment $x1 in the loop. However, I think that code is pretty bad to start with. function round_up ($sol, $x1, $op, $x2) { while($sol < round($sol)) { $x1++; switch ($op) { case '+' : $sol = $x1 + $x2; break; case '-' : $sol = $x1 - $x2; break; case '*' : $sol = $x1 * $x2; break; case '/' : $sol = $x1 / $x2; break; } } return (array ($sol, $x1, $op, $x2)); }
-
This topic has been moved to PHP Regex. http://www.phpfreaks.com/forums/index.php?topic=326002.0
-
$text = preg_replace("#<script[^>]*>.*?</script>#i", '', $text); $text = preg_replace("#<style[^>]*>.*?</style>#i", '', $text); Not tested, but I think it is right.
-
define( 'DS', DIRECTORY_SEPARATOR ); That line is defining a constant as the value for the PHP system variable DIRECTORY_SEPARATOR. The DIRECTORY_SEPARATOR variable will be a backslash or forwardslash based on the operating system of the server. This ensures that a file path will work whether the application is running on Windows or Linux. They are simply redefining the value as DS as it is much simpler to write DS when you need the directory separator than writing out the full system variable name. I see your edit - but if you had really read that page you would understand why two were used. It was kind of the whole point of the article.
-
Your problem is on the very first line of code $comp_name = abc; That line is trying to assign the value of a constant named abc as the value of $comp_name. I assume you mean to assign a string value of "abc". So, you need to enclose the value in quotes (either single or double) $comp_name = "abc"; EDIT: Also no need for two separate IF statements: $comp_name = "abc"; if(isset($_POST['company_name']) && $_POST['company_name']==$comp_name) { header("Location: http://www.hotmail.com"); } else { header("Location: http://www.yahoo.com"); } exit();
-
If $var = $_POST['text']; is telling you that $_POST['text'] is not defined then, guess what, it is not defined. Either the data is being sent with a different name than you think or it is not being sent. You should do a print_r($_POST) to verify what data is in the POST data. If the data is there, but with a different name/structure than you think then you can make the necessary adjustments. If the data isn't there, then the problem is likely in your form. Perhaps the text fields are not inside the start/end FORM tags. But, some simple debugging will point you in the right direction. When you have problems such as these add some logic to display any variables to the page to validate what you are getting and what you are doing with it.
-
You need to change this to a while loop ($row = mysql_fetch_assoc($result)) ;{ It was in your original code, so I don't know at what point you changed it. (Also, remove the semicolon) while ($row = mysql_fetch_assoc($result)) { Lastly, I inadvertently left off the column names in the dynamic query, which flolam corrected. But, you can make this line $where_parts[] = str_replace("search", "user", $name)." = '".mysql_real_escape_string(trim($_POST[$name]))."'"; much simpler by naming your input fields the same as your db fields. Doing that makes it much easier on you in the future when you have to make changes to your code. That line would look something like this $where_parts[] = "{$name} = '".mysql_real_escape_string(trim($_POST[$name]))."'";
-
I changed your original IF statement because this if($_POST) will always return true. So, I changed it to check if one of the form inputs was set, but inadvertantly set it to the wrong name. So, it was always returning false and the search code wasn't run. Change it to this: if(isset($_POST['searchage']))
-
<?php if(isset($_POST['username'])) { // Connects to your Database mysql_connect("localhost", "user", "pass") or die(mysql_error()); mysql_select_db("DB") or die(mysql_error()); //Create where clause $postNames = array('searchage', 'searchlocation', 'searchgenre', 'searchinstrument', 'searchexperience'); $where_parts = array(); $where_clause = ''; foreach($postNames as $name) { if(isset($_POST[$name]) && trim($_POST[$name])!='') { $where_parts[] = mysql_real_escape_string(trim($_POST[$name])); } } if(count($where_parts)>0) { $where_clause = " WHERE " . implode(' AND ', $where_parts); } $query = "SELECT * FROM table_user {$where_clause}"; $result = mysql_query($query) or die(mysql_error()); $num = mysql_num_rows($result); echo "$num results found!<br>"; while($row = mysql_fetch_assoc($query)) { echo " Name: {$row['username']}<br> Email: {$row['useremail']}<br> Age: {$row['userage']}<br> Location: {$row['userlocation']}<br> Genre: {$row['usergenre']}<br> Instrument: {$row['userinstrument']}<br> Experience: {$row['userexperience']}<br> Biography: {$row['userbiography']}<br><br> "; } } ?>
-
Typically you wouldn't have a bunch of confirmation statements for all the validations. If you really want all those messages for each validation, then you need separate logic for each. But, if you only want to provide messages when there is a problem - as is typical - then here is another method: Put the validation logic at the topo of the page. Then you can provide any error messages at the top of the page and repopulate form fields with the entered values if appropriate. <?php //verify that the update button is pressed if (isset($_POST['update'])) { //Parse submitted data $password = (isset($_POST['password'])) ? $_POST['password'] : ''; $confirm = (isset($_POST['password'])) ? $_POST['confirm'] : ''; //Perform validation and determine if errors exist $error = false; //Verify that required fields have values if(empty($password) || empty($confirm)) { $error = "All fields are required"; } //Verify length elseif(strlen($password) < 6) { $error = "Passwords must be at least 6 characters long."; } //Verify content elseif(preg_match('/[0-9]/', $password)==0) { $error = "Passwords must contain at least one number."; } //Verify match with confirmation elseif($password != $confirm) { $error = "Passwords do not match"; } //Validation complete, check if error occured if(!$error) { //Do something with the data and redirect to confirmation page } } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "[url=http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd]http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd[/url]"> <html xmlns="[url=http://www.w3.org/1999/xhtml]http://www.w3.org/1999/xhtml[/url]"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Untitled Document</title> </head> <body> <div style=\"color:red;\"><?php echo $error; ?></div> <form name="form1" method="post" action="<?PHP $_SERVER['PHP_SELF'] ?>"> <table width="100%" border="1"> <tr> <th scope="row"> <strong>New password: </strong> <input type="password" maxlength="15" name="password"></th> <th scope="row"> <strong>New password confirmed: </strong> <input type="password" maxlength="15" name="confirm"></th> <th scope="row"><input type="submit" name="update" value="Update Password" /></th> </tr> </table> </form> </body> </html>
-
No need to make this harder than it needs to be. When you loop through the results, instead of putting them back into the array just to implode them into a string - ust build the string within the loop. And why in the worl would you use a while() loop instead of foreach()? $output = ''; foreach ( explode(',', $other_form) as $chapter => $href) { $output .="<a href=\"{$href}\"> Chapter {$chapter} </a>\n"; }
-
based upon the OP comments he needs to search the array VALUES against the user input and return the index. The above would work if you were searching the array indexes and wanted the value. array_search() is the correct solution.
-
Ah, I didn't catch that. You would never use an or die() after a num_rows() call (assuming you already validated that the query didn't fail). Since the value was 0 - the code interpreted that as false and performed the on die() action. Since there was no error with the query there was no output displayed from the die()
-
That is what you want. You have the eoptions in thier own table, so you want to store the ID from the sm_options table into the mission table. That is how you should reference data between table (using a foreign ID). Then when you pull data from the mission table you do a JOIN on the sm_options table to get the textual value. With the above process if you were to ever change a description in the sm_options ns table it applies to all the records that use that option. If you try to store the textual value in the mission table you woul dhave to update all the records when you want/need to make a change So, in your mission table, I would change the "event" column name to "sm_options_id". Then store the numerical value in that column. You can then get the values from the mission table along with the textual value for the option using a JOIN query Example: SELECT m.*, sm.eoptions FROM mission AS m JOIN sm_options AS sm ON m.sm_options_id = sm.id
-
Not sure I understand your confusion. You have a form which contains a select list. Select a value from the list and click the submit button. Your processing page, "pentry.php", will need to access that POSTed value ($_POST['eoptions']) and do something with it.
-
array_search(): http://www.php.net/manual/en/function.array-search.php You will need to take into account the "case" of the input. It will need to be the same case as the value in the array. I.e. "One" != "one"
-
You can't "... return the selected option to mySQL". You need to have a form that POSTs to a PHP page. That PHP page will take the POSTed values (perform any necessary validations) and run a query to insert the values. Assuming your select list is being generated and you can select a value, have you determined if the value is being passed to the processing page? There are some minor issues with your existng code that may or may not be part of your problem. 1) You are not enclosing the "vlaue" of the options in quotes. If the values have a space this will cause a problem. 2) Your select statement is malformed. It is always preferable, in my opinion, to generate your dynamic code in the beginning of your script and then produce all the output at the end - instead of trying to put php code within the output portion of your code. Makes it much easier to see those types of errors and gives you a lot of flexibility. <?php // Create and run query to get list of event options $query = "SELECT eoptions,id FROM sm_options"; $result = mysql_query($query); // Loop through results to create the options list for events $eventOptions = ''; while($nt=mysql_fetch_array($result)) { /* Option values are added by looping through the array */ $eventOptions .= "<option value=\"{$nt['id']}\">{$nt['eoptions']}</option>\n"; } mysql_close(); ?> <form name="pentry" method="post" action="" action="pentry.php"> <input name="username" type="hidden" value="<?php echo $username; ?>"> Date of Practice: <input type="text" id="dates" name="practdate" datepicker="true" datepicker_format="YYYY-MM-DD" maxlength="100"> <br> <select name="eoptions">Event Options</option> <option value="">Event Options</option> <?php echo $eventOptions; ?> </select> <br> Event: <input type="text" name="event" maxlength="100" value="<?php echo "test $output" ?>"> <br> Session Time: <input type="text" name="practtime" id="practtime">min. <input type="button" value=" + " onClick="addmin(practtime);"> <input type="button" value=" - " onClick="submin(practtime);"> <br> Practice Content: <input type="text" name="practnotes"><br> <input type="submit" name="submit" value="Submit"><br> </form>
-
If no results are returned from a SELECT query, mysqli_num_rows() WILL return 0. If your echo statement is not displaying then something else is causing your problem. Since that code is wrapped in an IF statement, I would assume that the condition for that IF statement is returning false and the queries are never being run at all. if ($con_submit && isset($user_id)) {
-
You are not using the correct parameters for a for() loop - perhaps you meant to use a while() loop. You can do one of the following: for() loop for ($i=1; $i<26; $i++) { //Run code inside loop //$i will be incremented automatically } while() loop //Define $i $i = 1; while($i<26) { //Run code inside loop //$i need to be incremented in the loop $i++; }
-
$query = "SELECT source, COUNT(*) as cnt FROM states GROUP BY source"; $result = mysql_query($query) or die(mysql_error()); while($row = mysql_fetch_array($result)) { echo "State: {$row['source']}, Count: {$row['cnt']} <br />\n"; }
-
Your problem is that you are missing the underscore right after the dollar sign: if(isset($POST['addclub'])){ Should be if(isset($_POST['addclub'])){ To ensure all the variables are passed in the POST/GET variables, use print_r() to see them echo "<pre>"; print_r($_POST); echo "</pre>";
-
The code you posted only contained the information needed to generate the select list. As I stated you need to acquire the selected value in order to auto-select it. As I also stated the $selectedValue variable is assumed to contain the, well, selected value. Since you did not provide any information on how to obtain that value I left it to you to do. If the selected value is in the records table, then you need to query that table to get it and use it in the code I provided.