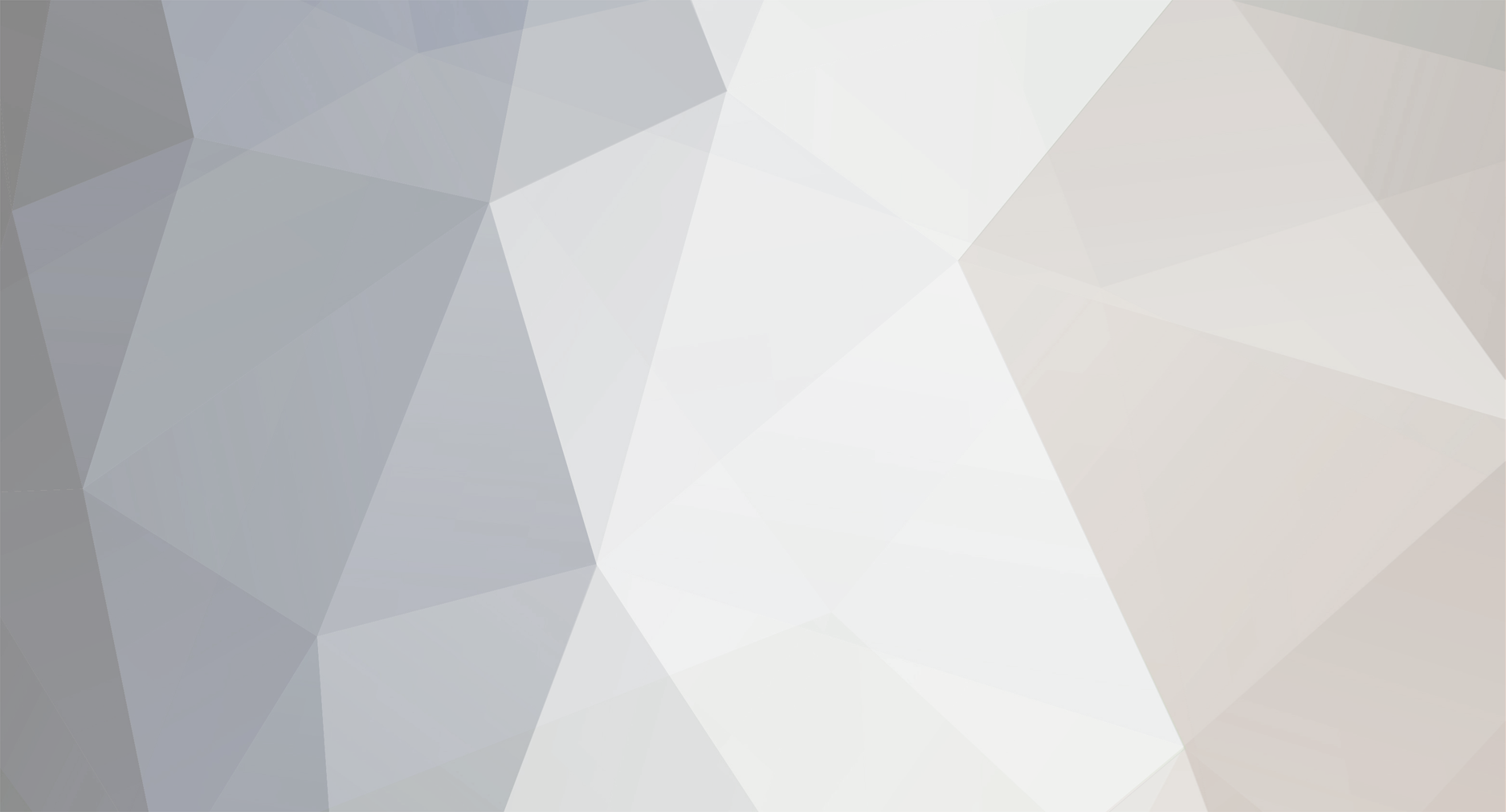
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Arrays have many useful functions that are rarely used. One example is array_shift() which will remove and return the first value int he array. You could use that (or array_pop() for th elast element) after randomizing the array. I think WildTeens' solution might be more efficient but I supply this as an alternative. //Import file as an array by lines $usernames = file('usernames.txt'); //Randomize the array shuffle($usernames); //Remove the first element $random_username = array_shift($usernames); echo 'Random Username: ' . $random_username; //Rewrite remaining array back to file $handle = fopen('usernames.txt', 'w'); fwrite($handle, implode('\n', $usernames); fclose($handle); Note, the previous solution used implode() without the parameter for the joining string. I don't think the rewritten values would be on separate lines in that instance. I modified it in my solution. If you go with the previous code you may need to modify that line.
-
I identified the exact problem back on reply #14 and I provide an example of how a correct query might look. You have a book? Most programming books are riddled with errors and should not be considered definitive by any means. Case in point: That statement is false. You CAN use double quotes in double quotes - if you know what you are doing and properly escape the quotes. $str = "Here is a string with \"double quotes\" inside \"double quotes\"."; echo $str; //output: Here is a string with "double quotes" inside "double quotes". I just don't want to just "let this die" if there is inaccurate information that someone else looking at this post for a similar issue may take as fact.
-
Exactly! That is the precise reason to use strtotime()! It will do the work for you.
-
Nice use of a double negative. Anyway, I didn't say your advice wouldn't work only that saying it HAD to use single quotes was a false statement. You can create any string with single or double quotes (or even the heredoc method). You just have to be aware of how variables and escaped characters are handled differently between the two.
-
What are you smoking? This thread is over a month old and your comment is completely false. There is no reason you can't define the query using double quotes.
-
Not tested, so there may be some syntax errors //Run query to get categories $query = "SELECT cat_id, cat_title FROM category ORDER BY cat_id"; $result = mysql_query($query) or die(mysql_error()); if (!mysql_num_rows($result)) { $categoryList = "<p><em>Sorry, no categories to browse.</em></p>"; } else { //Set the selected category (if exist) $selected_cat = (isset($_GET["cat_id"])) ? $_GET["cat_id"] : false; //Create category select list $categoryList = "<select name=\"cat_id\" class=\"blackfont\">\n"; //Display the categories while ($cats = mysql_fetch_array($result)) { $selected = ($cats['cat_id']==$selected_cat) ? ' selected="selected"' : ''; $categoryList .= "<option value=\"{$cats['cat_id']}\"{$selected}>{$cats['cat_title']}</option>\n"; //Set first category as selected if none selected, so we can determine subcategories to display if($selected_cat===false) { $selected_cat = $cats['cat_id']; } } $categoryList .= "</select>\n"; //Run query to get subcategories $query = "SELECT id, cat_id, sub_title FROM sub_category WHERE cat_id = '{$selected_cat}'"; $result = mysql_query($query) or die(mysql_error()); if (!mysql_num_rows($result)) { $subCategoryList = "<p><em>Sorry, no categories to browse.</em></p>"; } else { //Create subcategory select list (if needed) $subCategoryList = "<select name=\"sub_id\" class=\"blackfont\">\n"; while ($subcats = mysql_fetch_array($result)) { $subCategoryList .= "<option value=\"{$subcats['cat_id']}\">{$subcats['sub_title']}</option>\n"; } $subCategoryList .= "</select>\n"; } }
-
You are making this way harder than it needs to be. Can you give some insight as to the source and purpose of the variables $nextMonth and $year2 and how they are modified? If "next month" is literally supposed to be next month, then you could simply use strtotime() $year2 = date('o', strtotime("next month");
-
Here is a bit of a hack, but it works in both IE and FF, did not try in other browsers: function setFocusToEnd(fieldID) { var inputObj = document.getElementById(fieldID); inputObj.focus(); inputObj.value = inputObj.value; } Then just call the onload event using onload="setFocusToEnd('t_description') Assuming you gave the field an ID and not just a name.
-
var toObj = document.getElementById("TO"); var toVal = toObj.options[toObj.slectedIndex].value;
-
This should meet your needs. I did some testing, but suggest you do some as well to ensure it meets your needs. function isValidPassword($password) { //Validate that 1st char is alpha, ALL characters are valid and length is correct if(preg_match("/^[A-Z][A-Z0-9\!\@\#\$\%\^\&\*\(\)\+\=\.\_\-]{5,15}$/i", $password)===0) { return false; } //Validate that password has at least 3 of 4 classes $classes = 0; $classes += preg_match("/[A-Z]/", $password); $classes += preg_match("/[a-z]/", $password); $classes += preg_match("/[0-9]/", $password); $classes += preg_match("/[!@#$%^&*()+=._-]/", $password); return ($classes >= 3); } Note the RegEx uses {5, 15} for the length because it doesn't include the first character that has different requirements than the rest.
-
I doubt your error has anything to do with that line since all your parens are within a string. The error is most likely somewhere above that line. As to your particular requirement I don't think it is possible to do it with a single regex expression due to your last requirement to require 3 out of four different "classes" of characters. You can validate that all classes are present, but I can't think of a way to validate that at least 3 out of the 4 are present. Well, not without creating an exceptionally long expression. I would suggest just using RegEx to validate that ALL the characters are withint he acceptable classes and do the other validations with non regex.
-
http://en.wikipedia.org/wiki/Greenwich_Mean_Time JavaScript time functions include functions that do not include GTM or UTC in their name. in those instances the time is simply the time from the computer running the code, whereas the UTC functions will return the UTC/GMT time. The one exception are the two functions: toGMTString() and toUTCString() which I believe are causing your confusion. They are simply the same function under different names. According to several sources, the toGMTString() function is http://www.w3schools.com/jsref/jsref_obj_date.asp
-
If that code is to be put into an AJAX request then it's not HTML, is it?
-
You need to use an actual ampersand ("&") and not "&" in the URL. Otherwise the GET variables are interpreted as 'input' and 'amp;inputvalue'
-
Although, this has nothing to do with your error, I would advise using strlen($_POST['fieldname']) to validate the input. Unless there is a valid reason not to, you should always trim() the input. Otherwise a user can enter spaces and it will be considered valid. That assumes that you are also using trim when the values are created and stored in the DB. Also, using empty() is a little more compact and is more "obvious" to someone reading the code: Example: $username = trim($_POST['username']); $password = trim($_POST['passwd']); if (empty($username)) { $errors[] = '<p>You forgot to enter your username!</p>'; } if (empty($password)) { $errors[] = '<p>You forgot to enter your password!</p>'; }
-
Sure you can, he just has the syntax wrong. I suspect you could make the function name dynamic but a simpler solution is to just use an array: <html> <head> <script type="text/javascript"> var validate = new Array(); for (var i=0; i<64; i++) { validate[i] = function() { var teamName = document.getElementById("teamName").value; alert(teamName); } } </script> </head> <body> <input type="text" name="teamname" id="teamname" /><br /> <button onclick="validate[2]();">Invoke function validate[2]</button> </body> </html>
-
Why on earth would you want to programatically create functions? If you can do that programatically, then you could just as easily create one function that takes the appropriate parameters. The functions you are apparently trying to create above do EXACTLY the same thing, so there is no purpose at all for that. Otherwise I would provide an example to your specific situation.
-
Um yeah. For testing purposes I didn't want the form to submit even if validation passed. So, I had a "return false;" even for the success scenario and forgot to change it when done. Just change the very last line in the function to "return true;". I'd also put a comment above that line to the effect "//No errors" I should also point out that I change the functin to take three parameters - the form object (which is passed by simply including 'this' in the trigger call), the first question number and the last question number. You will need to set those two values on every page inthe onsubmit trigger call based upon the questions being displayed on the page.
-
See that, changed it, no effect. Why is that an issue? Well, it has no effect if you don't fix the other problems too. But, it is a problem nonetheless. A form can be submitted by the user by clicking a submit button OR by pressing the enter key (depending where the focus is). The form already has an onsubmit trigger. That trigger will fire regardless of how the form is submitted. The onclick trigger in the submit button is onclick="location.href='process-assessment.php?num=0&num1=5'" So, assuming everything else is functioning correctly, if the user clicks the submit button, the onsubmit trigger is fired and validation is done. If validation fails then the form won’t submit. But THEN the onclick event in the submit button is triggered so the page will redirect to that URL – and not even submit the data. It is not needed and is detrimental to what you are trying to achieve. It needs to be removed. Can you elaborate? Well, I can tell you what the results of my testing were. When you have a single element, such as a text input you could reference the value using formObject.Elements[textFieldName].value When you have a radio group you give all elements the same name. So, Javascript sees them as an array and to reference a particular radio button you would need to use: formObject.Elements[radioFieldName][radioElementIndex].value. In my testing I tried alerting formObj.elements[1].length. That SHOULD have alerted with “3” because the radio group with the name “1” is an array of three elements. But, instead I received “undefined”. I spent a lot of time scratching my head and trying different things. Although the “1” is valid for an HTML field name, many programming languages do not allow variable name to being with a numeric character. So, I changed the name of the radio inputs to “Q1” and then my test ran successfully. Here is a working page: <html> <head> <script type="text/javascript"> function checkForm(formObj, firstNo, lastNo) { var errors = new Array(); //Cycle through each question for(var qIdx=firstNo; qIdx<=lastNo; qIdx++) { var groupObj = formObj['Q'+qIdx]; //Cycle through each answer to see if one is checked var hasAnswer = false; var answerLength = groupObj.length; for(aIdx=0; aIdx<answerLength; aIdx++) { hasAnswer = groupObj[aIdx].checked; if(hasAnswer) { break; } } if(!hasAnswer) { errors[errors.length] = ' - Question ' + qIdx + ' has not been answered.'; } } if(errors.length>0) { var errorMsg = 'The following errors occured:\n\n'; errorMsg += errors.join('\n'); alert(errorMsg); return false; } return false; } </script> </head> <body> <form id="myForm" action="process-assessment.php?num=0&num1=5" method="post" onsubmit="return checkForm(this, 1, 5);"> 1. How many beers can a person have while eating dinner, and still be able to drive?<br /><ul> <li style="list-style-type:none;"><input type="radio" name="Q1" value="1" />1-3</li><br /> <li style="list-style-type:none;"><input type="radio" name="Q1" value="2" />4-6</li><br /> <li style="list-style-type:none;"><input type="radio" name="Q1" value="3" />None</li><br /> </ul> 2. Whether or not you drink every day, are there times you drink more than 5 drinks in one sitting?<br /><ul> <li style="list-style-type:none;"><input type="radio" name="Q2" value="4" />Yes</li><br /> <li style="list-style-type:none;"><input type="radio" name="Q2" value="5" />No</li><br /> </ul> 3. In the past six months, have there been days when you have had to cut down or limit your usual activities because of your health?<br /><ul> <li style="list-style-type:none;"><input type="radio" name="Q3" value="7" />Yes</li><br /> <li style="list-style-type:none;"><input type="radio" name="Q3" value="8" />No</li><br /> </ul> 4. Is your blood pressure greater than 120/80 mmHg?<br /><ul> <li style="list-style-type:none;"><input type="radio" name="Q4" value="9" />Yes</li><br /> <li style="list-style-type:none;"><input type="radio" name="Q4" value="10" />No</li><br /> <li style="list-style-type:none;"><input type="radio" name="Q4" value="11" />I don't know</li><br /> </ul> 5. Is your total cholesterol under 200mg/dl and/or controlled by medication?<br /><ul> <li style="list-style-type:none;"><input type="radio" name="Q5" value="12" />Yes</li><br /> <li style="list-style-type:none;"><input type="radio" name="Q5" value="13" />No</li><br /> <li style="list-style-type:none;"><input type="radio" name="Q5" value="14" />I don't know</li><br /> </ul><br /> <div><button type="submit" name="next">Next</button></div><br /><br /> You have <b>83</b> out of <b>88</b> questions left<br /><br /></form> </body> </html>
-
Your biggest problem is that you have an onclick event for your submit button and it is scewing with the submit functionality. You don't need that in your submit button - remove it. Second. Don't give the radio options names with just numbers. I did some testing and found that the fields are not referenced properly with numeric only names. Try using Q1, Q2, Q3, etc.
-
Here is a complete rewrite (not tested) that puts all the coding in a more logical order and solves the problem <?php @session_start(); if(!isset($_SESSION['SESSION'])) { require ("sess.php"); } //Set page parameters $records_per_page = 2; //Set the search criteria if (isset($_POST['record_id'])) { $record_id = $_POST['record_id']; ; $file_number = $_POST['file_number']; $name_land = $_POST['name_land']; $location = $_POST['location'] ; $_SESSION['record_id'] = $record_id; $_SESSION['file_number'] = $file_number; $_SESSION['name_land'] = $name_land; $_SESSION['location'] = $location; } else { $record_id = $_SESSION['record_id']; ; $file_number = $_SESSION['file_number']; $name_land = $_SESSION['name_land']; $location = $_SESSION['location'] ; } //Create common WHERE clause $WHERE = "file_number = '$file_number' || Record_id = '$record_id' || land_name = '$name_land' || location = '$location'"; //Connect to database @$connect = mysql_connect($_SESSION['server'],$_SESSION['un'],$_SESSION['pass']) or die ('Error : Unable to connect to the MYSQL Server');; @mysql_select_db($_SESSION['db']) or die ('Error : Unable to connect to the Data Base'); //Get total number of records and determine total pages $query = "SELECT COUNT(Record_id) as total FROM {TABLE} WHERE {$WHERE}"; $result = mysql_query($query); $total_records = mysql_result($result, 0, 'total'); $total_pages = ceil($total_records / $records_per_page); //Determine curernt page and start limit $page = (isset($_GET['page'])) ? $_GET['page'] : 1; if($page<1 || $page>$total_pages) { $page = 1; } $start_limit = ($page-1) * $records_per_page; //Get the records for the current page $query = "SELECT Record_id,file_number,Land Name from TABLE WHERE {$WHERE} ORDER BY Record_id ASC LIMIT {$start_limit}, {$records_per_page}"; $result = mysql_query($query); //Process the results $tableBody = ''; while ($row = mysql_fetch_array($query1)) { $tableBody .= "<tr>\n"; $tableBody .= "<td>{$row['Record_id']}</td>\n"; $tableBody .= "<td>{$row['file_number']}</td>\n"; $tableBody .= "<td>{$row['land_name']}</td>\n"; $tableBody .= "<td>\n"; $tableBody .= "<form action=\"dlt_grid.php\" method = \"GET\">\n"; $tableBody .= "<input type=\"submit\" value=\"Delete\">\n"; $tableBody .= "<input type=\"hidden\" name=\"hf\" value=\"{$row['Record_id']}\">\n"; $tableBody .= "</form>\n"; $tableBody .= "</td>\n"; $tableBody .= "</tr>\n"; }; //Create pagination menu $page_links_ary = array(); for($p=1; $p<=$total_pages; $p++) { $page_links_ary[] = ($page==$p) ? "<b>{$i}</b>" : "<a href=\"searching.php?page={$i}\">{$i}</a>"; } $page_links = implode('', $page_links_ary); ?> <html> <body> Page: <?php echo $page; ?> <br /><br /> <table border="1"> <thead> <tr> <th>Record ID</th> <th>file_number</th> <th>Land Name</th> </tr> </thead> <tbody> <php echo $tableBody; ?> </tbody> </table> <br /><br /> Total Records: <?php echo $total_records; ?> <br /><br /> <?php echo $page_links; ?> </body> </html>
-
I'm too lazy right now to check every line of code right now. But, I do see you are using POST & GET values in that page. I suspect that what is happening is that you have a form that is used to first access the page. So, the first load of the page has those POST values that are used in the query. But, when you click one of the links to access another page the POST values do not exist. Therefore your query is not returning any results. You will need to save the POST values in session or cookie values on the first page load so you can use them on subsequent loads for the other pages.
-
When a PHP script is run it doesn't send the page to the browser until it is done processing. You can't send a page tothe browser then have PHP interact with that page by itself. THe user needs to initiate some action to ask the server for new information such as the user clicking a link or JavaScript running in the client's browser that will initiate the request. So, you need a client-side solution. But, in this case, even JavaScript is not needed since there is a simple HTML solution. The meta refresh tag. <meta http-equiv="refresh" content="10;url=http://www.domain.com/pagetoredirect.php"> Put a line like that in the HEAD section of the HTML page and after 10 seconds it will refresh the page by opening the URL http://www.domain.com/pagetoredirect.php
-
Your form has TWO fields named "mfg" <select id="mfg" name="mfg"> <input type="hidden" id="mfg" name="mfg" value="<?php echo $_GET['mfg']; ?>" /> That might be causing the problem.
-
The manner in which you create teh HTML (loops, if statements, etc) will have absolutely no bearing on whether the javascript will work or not. If the javascript code works, which I assume it does since I could see it working on the page linked above, then there is something wrong in the output you are creating. Specifically, I would be looking at the structure of the table. Are all cells and row tags properly opened and closed? EDIT: Yep, a quick look at your code shows that the tabletags are fugged up. This line makes no sense echo "<td></tr></td>"; You don't seem to be using nested tables so there can't be a closing TD tag after a closing TR tag. Try this: $max_col = 100; $query = "SELECT * FROM events"; $result = mysql_query($query) or die(mysql_error()); echo "<table>\n"; echo "<tr>\n"; echo "<th style=\"text-align:left\">Event ID</th>\n"; echo "<th style=\"text-align:left\">Event Name</th>\n"; echo "<th style=\"text-align:left\">Sport</th>\n"; echo "<th style=\"text-align:left\">State</th>\n"; echo "<th style=\"text-align:left\">Venue</th>\n"; echo "<th style=\"text-align:left\">Date</th>\n"; echo "</tr>\n"; $col = 0; while($row = mysql_fetch_array($result)) { extract($row); $col++; //Open row if needed if ($col%$max_col==1) { echo "<tr>\n"; } echo "<td>{$id}</td>\n"; echo "<td>{$eventname}</td>\n"; echo "<td>{$sport}</td>\n"; echo "<td>{$state}</td>\n"; echo "<td>{$venue}</td>\n"; echo "<td>{$month} {$day}, {$year}</td>\n"; //Close row if needed if ($col%$max_col==0) { echo "</tr>\n"; } } //Close last row if not already done if($col%$max_col!=0) { echo "</tr>\n"; } echo"</table>";