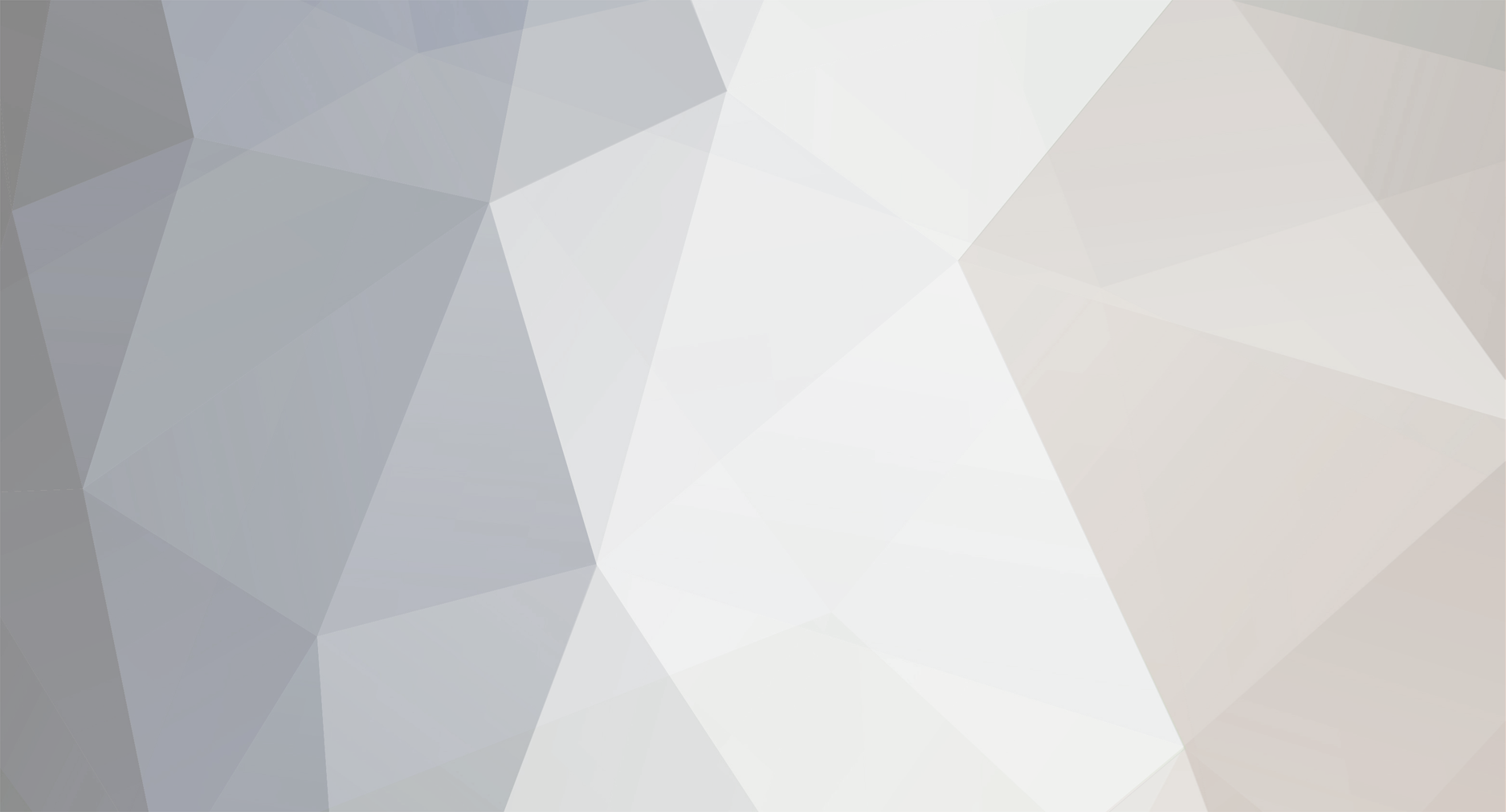
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I still don't understand what you really want to do and I suspect there is a VERY easy solution with the proper query. But, I'll give you a solution based on what you already have. while ($player1 = mysql_fetch_array($update) && $player2 = mysql_fetch_array($update)) { //Each iteration of the loop will pull two records from the //results and assign to $player1 and $player2. //Note: if uneven number of records are returned //the last record won't be processed //Insert //Delete }
-
I'm confused too because I'm not understanding what you are trying to accomplish. Are you just wanting to match up all the players in pairs randomly? I would assume the insert is used to save a record for the association of each pair, but I'm not understanding what the delete would be for. It should be possible to create all the pairs records with a single query - i.e. no PHP processing. But, I'm hesitant to invest any time into this since I'm not sure if what i think is what you really want.
-
If you don't want to refresh the page you will have to use JavaScript. There are some javascript only solutions that will allow you to sort a table. http://www.kryogenix.org/code/browser/sorttable/ However, you could also use JavaScript + PHP (i.e. AJAX). This wouldn't be an optimal solution in my opinion because there can be a delay with AJAX, but the one benefit is that if the records changed since the user first loaded the page they would get the most up to date results when sorting the table.
-
New HTML table within a while loop foreach $row['sectionid'] ?
Psycho replied to RJP1's topic in PHP Coding Help
I would suggest using a single table with intermediary headers for each section. It will make this much easier. Or, put an empty row between sections. Here's a quick rewrite (not tested) public function listArticle() { require_once('../includes/DbConnector.php'); $connector = new DbConnector(); $result = $connector->query('SELECT * FROM article ORDER BY section, title'); echo '<h2>Articles:</h2>'; if ($result === false) { $output = "Unable to query results."; } else if(mysql_num_rows($result) < 1) { $output = "There were no results."; } else { $header = "<tr>\n"; $header .= "<th scope=\"col\">Section</th>\n"; $header .= "<th scope=\"col\" style=\"text-align:center;\">Edit?</th>\n"; $header .= "<th scope=\"col\" style=\"text-align:center;\">Delete?</th>\n"; $header .= "</tr>\n"; $output = "<table id=\"table\">\n"; $output .= "<tbody>\n"; $current_title = ''; while ($row = $connector->fetchArray($result)) { if($current_title != $row['title']) { $current_title = $row['title']; $output .= "<tr><td colspan=\"3\"> </td></tr>\n"; $output .= "<tr><td colspan=\"3\"><b>{$current_title}</b></td></tr>\n"; $output .= $header; } $output .= "<tr>\n"; $output .= "<td>{$row['section']}</td>\n"; $output .= "<td>[Edit Link]</td>\n"; $output .= "<td>[Delete Link]</td>\n"; $output .= "</tr>\n"; } $output .= "</tbody>\n"; $output .= "</table>\n"; } echo $output; return; } -
Your expression is greedy. Do some research for greedy vs. non-greedy regular expressions. But, the simple fix if to add a question mark as indicated to make it non-greedy: preg_match_all('%<!-- message -->(.+?)<!-- / message -->%si',$x,$y)
-
Here's my two cents: First query (in readable format): SELECT manufacturer.manufacturerid AS mfgID, manufacturer.name AS mfgName FROM manufacturer JOIN product ON manufacturer.manufacturerid = product.manufacturerid JOIN categorynames ON product.categoryid = categorynames.categoryid WHERE categorynames.name LIKE ('%printer%') GROUP BY manufacturer.manufacturerid ORDER BY manufacturer.name ASC Are the category names very diverse - i.e. are they a fixed list of values or do they change constantly? If they are a fixed list, but you have several that have "printer" in the name, it might be more efficient to explicitly retrieve the records exatcly matching those values instead of using a LIKE statement that has to interpret every value. WHERE categorynames.name IN ('Laser Printer', 'Color Printer', 'Duplex Printer')
-
No. You are using a class for your database operations. I suspect that either an error is occuring during one of the database connection/query commands here: $this->db->select('lang_code, lang_name'); $this->db->from('languages'); $langQuery = $this->db->get(); Or, you are not calling the num_rows function correctly onthis line $langQuery->num_rows() > 0 However, you are using a custom class for these database operations not the common PHP functions. So, it is impossible for anyone to determine the answer from what you have provided unless we understand the 3rd party class that you are using.
-
I think your expected results are wrong. According to your explanation the actual results you show appear to be correct. In your example your starting two numbers are 1 and 1. You state that the two number should be added and that the first number will be removed and the seconf number will move to the first position while the result will go to position 2. Since you start with 1 and 1, the first sum would be 2. What are you really trying to achieve? Because what you are asking doesn't seem to make sense based upon the context of the code where you seem to be trying to calculate proce.
-
My god, this is frustrating. I don't want to feel that all the time I've invested has been wasted. If I was able to "unfollow" a thread I would, so I wouldn't be tempted to see these responses. I just handed you the perfect solution and you didn't take 2 seconds to even understand or implement it. All you need to do is use the function I provided and add the additional patterns for the other skill levels. You could have easily modified the one I provided for skill-0 to get the others. In fact, you could create them programatically function parser($data) { //Temp array for the output $output = array(); //Create array of the keys and patters to extract data $patterns = array( 'username' => '/character-username = (.*)/', ); //Create skill patterns programatically since they are the same for($skillIdx=0; $skillIdx<25; $skillIdx++) { $patterns["skill-{$skillIdx}"] = '/character-skill = '.$skillIdx.'\t([^\t]*)/'; } //Iterate through pattern array to extract data and assign to $ouput var foreach($patterns as $key => $pattern) { preg_match($pattern, $data, $matches); $output[$key] = $matches[1]; } //Return the output array return $output; } $data = file_get_contents('Matthew.txt'); print_r(parser($data)); That function will extract ONLY the data you are after so there is no need to strip unnecessary data afterward. Output: Array ( [username] => Matthew [skill-0] => 99 [skill-1] => 99 [skill-2] => 99 [skill-3] => 99 [skill-4] => 99 [skill-5] => 99 [skill-6] => 99 [skill-7] => 99 [skill-8] => 99 [skill-9] => 99 [skill-10] => 99 [skill-11] => 99 [skill-12] => 99 [skill-13] => 99 [skill-14] => 99 [skill-15] => 99 [skill-16] => 99 [skill-17] => 99 [skill-18] => 99 [skill-19] => 99 [skill-20] => 99 [skill-21] => 1 [skill-22] => 1 [skill-23] => 1 [skill-24] => 0 )
-
Here is my last submission to this thread. This solution uses - surprise, surprise - regular expressions. Because, well, it makes sense for this problem. But, no one is forcing you to use it. With this solution you can add additional keys and expressions to the $patterns array to have whatever informaiton you want returned in the output array: function parser($data) { $output = array(); $patterns = array( 'username' => '/character-username = (.*)/', 'skill-0' => '/character-skill = 0\t([^\t].)/' ); foreach($patterns as $key => $pattern) { preg_match($pattern, $data, $matches); $output[$key] = $matches[1]; } return $output; } $data = file_get_contents('Matthew.txt'); print_r(parser($data)); Using the text file you attached, this is the output: Array ( [username] => Matthew [skill-0] => 99 )
-
Use whatever you want to use. No one is forcing you to use anything. We have provided code that works on the file you have provided it. I tested the code I posted back on reply #5 and it worked. You were the one asking for help using a regular expression. If you don't have the mental capacity or the initiative to learn something then don't post on a forum asking for people to help.
-
$userList = array(); while($user_fetch = $db->fetch_assoc($chars_fetch)) { $userList[] = $user_fetch['username']; } echo implode(',', $userList);
-
As I posted way back on page one, you need the "S" flag to tell the regex parser to look for the pattern across multiple lines $pattern = "/username = (.+?)character-password/s";
-
Here is a quick mock-up of one possible solution: <html> <head> <style> .post { background-color: #ccccff; } .post_title { font-weight: bold; float: left; } .quote_link { text-align: right; } </style> <script type="text/javascript"> function addQuote(postID) { var replyObj = document.getElementById('reply'); var quoteAuth = document.getElementById('post_'+postID+'_author').innerHTML; var quoteText = document.getElementById('post_'+postID+'_text').innerHTML; var replyContent = '[quote author='+quoteAuth+']'+quoteText+'[/quote]'; replyObj.value += "\n" + replyContent; return false; } </script> </head> <body> <div id="post_1" class="post"> <div id="post_1_header" class="post_title">Post By: <span id="post_1_author">Bob</span></div> <div class="quote_link"><a href="#" onclick="return addQuote('1');">Quote</a></div> <div id="post_1_text">Hey how many feet are in a mile?</div> </div> <br /> <div id="post_2" class="post"> <div id="post_2_header" class="post_title">Post By: <span id="post_2_author">Jane</span></div> <div class="quote_link"><a href="#" onclick="return addQuote('2');">Quote</a></div> <div id="post_2_text">There are 5,280 feet in a mile.</div> </div> <br /> <div id="post_3" class="post"> <div id="post_3_header" class="post_title">Post By: <span id="post_3_author">Bob</span></div> <div class="quote_link"><a href="#" onclick="return addQuote('3');">Quote</a></div> <div id="post_3_text">Great, thanks.</div> </div> <br /> Reply:<br /> <textarea name="reply" id="reply" style="width:600px; height:250px;"></textarea> </body> </html>
-
Again, you need to read the manual for preg_match_all(). You won't get the results in the format you are wanting because it doesn't have any option for doing so. The default behavior of preg_match_all() is the same as if you used the PREG_PATTERN_ORDER flag. In that format, the results are returned in a multi-dimensional array where the first indexc contains an array of the "full" pattern matches and the second index contains an array of the values matched by the first parenthesized subpattern. That is what I did with the example I provided. I included a full pattern that matches everything from the opening A tag to the ending A tag. But, I included a sub-pattern in parenthesis that matched ONLY the exact data you are wanting - the URL. The results are contained in a multi-dimensional array as I showed above. So, if you want to reference just the URLs you would do so like this: first URL = $mulinks[1][0], second URL = $mulinks[1][1], third URL = $mulinks[1][2], etc. If you want just a single dimensional array of just the URLs, then you can define a new variable using: $justURLs = $mulinks[1] Assuming you are usng a parenthesised sub query such as I provided.
-
Not tested: SELECT cust.field1, cust.field2, cust.field3, COUNT(Orders.id) as order_Count, COUNT(Deals.id) as deals_count FROM Customers as cust, LEFT JOIN Orders ON Orders.d_id = cust.id LEFT JOIN Deals as d ON Deals.d_id = cust.id GROUP BY Orders.d_id, Deals.d_id
-
You should read the manual for preg_match_all() - specifically on how the matches are assigned to the $matches parameter. There are a number of optional flags you can set to determine the format of the results. http://us3.php.net/manual/en/function.preg-match-all.php Also, you can improve your pattern so you don't get the leading and ending <> characters: preg_match_all('/<a[^>]*>(http:\/\/www.link\.com\/[^<]*)<\/a>/', $data, $mulinks); Output: Array ( [0] => Array ( [0] => <a href="http://www.link.com/?d=E8ON0ESE" target="_blank">http://www.link.com/?q=E8ON0ESE</a> [1] => <a href="http://www.link.com/?d=LVZTY0TE" target="_blank">http://www.link.com/?q=LVZTY0TE</a> [2] => <a href="http://www.link.com/?d=8ZYJY3MC" target="_blank">http://www.link.com/?q=8ZYJY3MC</a> [3] => <a href="http://www.link.com/?d=FJ3W6QAB" target="_blank">http://www.link.com/?q=FJ3W6QAB</a> ) [1] => Array ( [0] => http://www.link.com/?q=E8ON0ESE [1] => http://www.link.com/?q=LVZTY0TE [2] => http://www.link.com/?q=8ZYJY3MC [3] => http://www.link.com/?q=FJ3W6QAB ) )
-
Regular expressions are, in my opinion, one of the more difficult aspects of programming to learn. Trying to intelligently explain it in a forum post is not achievable. Here's a good site with a tutorial and lots of reference info to get you started: http://www.regular-expressions.info/
-
What does that mean? Do you want to replace "P" with "WR" in the string or do you have a different string that uses "WR" insterad of "P" and you need the number in that string? The space and "P" is trying to match those characters. The "\b" matches a word boundry such as a space, tab, period, etc. The "/" is the closing delimited for the expression. Try giving a better explanation of what you are trying to achieve.
-
SELECT IFNULL(lo.log_time, 0) AS isOnline, IFNULL(a.id_attach, 0) AS ID_ATTACH, a.filename, a.attachment_type, mem.personal_text, mem.avatar, mem.id_member, mem.member_name, mem.real_name, mem.last_login, mem.website_title, mem.website_url, mem.location, mem.posts, mem.id_group FROM ". $db_prefix."members AS mem LEFT JOIN ".$db_prefix."log_online AS lo ON (lo.id_member = mem.id_member) LEFT JOIN ".$db_prefix."attachments AS a ON (a.id_member = mem.id_member) WHERE mem.id_group IN (". implode(",", $groupIds) .") GROUP BY mem.id_group ORDER BY mem.id_group
-
regular expression that matches the time without the am/pm
Psycho replied to kee2ka4's topic in Regex Help
That won't work for 24:00 as requirested by the OP. Although, I do see I had an error in my original code for the hour "20". And, that does give me an idea on shortening the original pattern to: return (preg_match("/^(([0-1]?\d|2[0-3]):[0-5]\d)|24:00$/", $timeStr)>0); - not tested -
OK, I meant to use explode() not split() - I was thinking of Javascript. Anyway, I think there are tow problems. 1. The preg_quote is escaping the control characters in the expression. It should be run on the variables only. 2. The expression will need to use the "s" flag to have the expression work across multiple lines. $delimiters = array( "username = |character-password", "skill = 0 | " ); foreach($delimiters as $delimSet) { $delimPair = explode('|', $delimSet); $startDelim = preg_quote($delimPair[0], '*'); $endDelim = preg_quote($delimPair[1], '*'); $pattern = "/{$startDelim}(.+?){$endDelim}/s"; preg_match_all($pattern, $data, $matches); print_r($matches[1]); }
-
Here's my function which seems to be more efficient and flexible. Just pass the string and the max characters to allow. It will reduce it to the first space character before the max limit and add ellipses if needed. The third parameter is optional for setting a custom string for the ellipses function truncateString($string, $length, $ellipse='...') { if (strlen($string) <= $length) { return $string; } return array_shift(explode("\n", wordwrap($string, $length))) . $ellipse; } $text = "This is a long string with many, many words and it should be truncated"; echo truncateString($text, 45); //Output: "This is a long string with many, many words..." You should probably trim() the string beforehand.
-
Or for easier reading: $a = '/{$firstcol[$i]}(.+?){$secondcol[$i]}/"; Also, $matches will be an array, so you can't echo it out. You will want to use the second index (i.e. $matches[1] to see the results of the matches). So, use print_r($matches[1]) to see the results. Check the documentation to see what is included in the $matches parameter. Here is what I would do: $data = file_get_contents('Matthew.txt'); $delimiters = array( "username = |character-password", "skill = 0 | " ); foreach($delimiters as $delimSet) { $delimPair = split('|', $delimSet); $pattern = preg_quote("/{$delimPair[0]}(.+?){$delimPair[1]}/", '*'); preg_match_all($pattern, $data, $matches); print_r($matches[1]); }