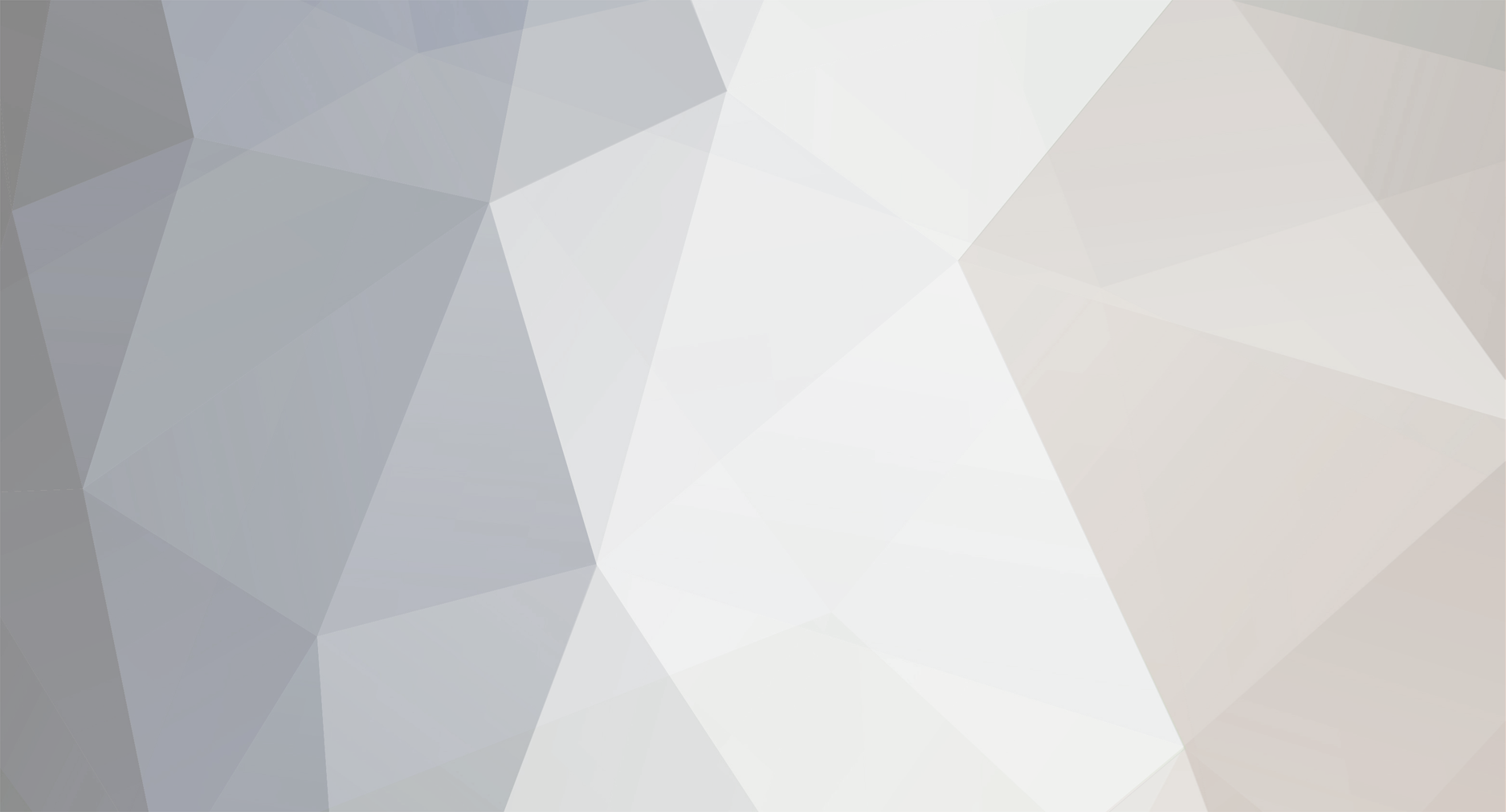
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
That function will not work. It simply returns true if ANY ONE character matches the pattern! The pattern needs to be exclusionary (Besides, why would you test if something is true, just to return true or false? Just return the result of the test. See example below) function validCharacters($input) { //Returns true if all the characters are letters (upper and lower case) return (preg_match("/[^\w]/", $input)==0); } //Usage if (!validCharacters($_POST['username'])) { echo "Username can only contain letters"; } else { //Input was valid }
-
echo "<title>" . ucwords(str_replace('-',' ',$r['Testing'])) . " </title>";
-
substr_count() returns an integer. The IF statement above is taking three integers and trying to && them together? You should be adding those results: if ((substr_count($forname, ' '))+(substr_count($surname, ' '))+(substr_count($city, ' ')) < 1) Or even simpler: if ((substr_count($forname.$surname.$city, ' '))< 1) Of course I'd use regular expression myself using preg_match()
-
You haven't provided enough information. You state there is a table that holds each poll as a single record with separate fields for each question. I assume that will have the text of the question. And, hopefully, each "poll" record has a unique id. But, you have not explained how the results are stored and how those records relate back to the poll records. However, you really should have a separate tables for 1) the polls, 2) the questions, and 3) the results.
-
Well, first off, DON'T do looping queries!!! The whole point of having a relational database is to be able to extract records that are related. Without knowing the exact database layout, this is only a guess as to what your query should look like: SELECT * FROM jobs JOIN customer_info ON jobs.customer_id = customer_info.id JOIN bus_info ON jobs.bus_id = bus_info.id JOIN sides ON jobs.side_id = sides.id
-
// Make the query: $q = "UPDATE users SET money=(money-$price) WHERE username='{$_COOKIE['username']}'"; $r = @mysqli_query ($dbc, $q) or trigger_error(mysqli_error($dbc)); if (mysqli_affected_rows($dbc) == 1) { // If it ran OK. Your query doesn't seem to be too restrictive. If it is updating more than one row then the IF statemetn will return false. That's just a possibility. Try echoing mysqli_affected_rows($dbc) to the page after that query to see what it is returning.
-
This works for me <html> <head> <script type="text/javascript"> function setBackgroundColor(color) { //remove current background setting document.body.style.background = ''; document.body.style.backgroundColor = color; return; } function setBackgroundImage(imageURL) { //remove current background setting document.body.style.background = ''; document.body.style.background = "url("+imageURL+")" return; } </script> </head> <body> <button onclick="setBackgroundColor('#0000ff')">Blue Backgrouond</button><br /> <button onclick="setBackgroundColor('#ff0000')">Red Backgrouond</button><br /> <button onclick="setBackgroundColor('#00ff00')">Green Backgrouond</button><br /> <button onclick="setBackgroundImage('image1.jpg')">Image 1 Background</button><br /> <button onclick="setBackgroundImage('image2.jpg')">Image 2 Background</button><br /> <button onclick="setBackgroundColor('')">Reset</button><br /> </body> </html>
-
Probably not. But that code should be modified. You are creating a random number from 0 to 10 (i.e. 11 possible options) whereas you only have 10 records from your query. Besides, why hard code the limit for the random number? If you were to ever change your query to a different number of records you would have to remember to change that too. Better to write the code so it self-adjusts: while ($row = mysql_fetch_row($request)) { $products[] = $row; } $randomProduct = $products[array_rand($products)];
-
Kind of hard to debug without your database. I've done a simple test within my own database and the logic provided above should work. I have also reviewed Buddski's posted query and compared it to your original query and see no reason it should not work. The only thing I can think of is that the database structure is not normalized and/or the original query is not as efficient as it could be. As I stated above you could do it in PHP if you can't figure out a workable solution using a query.
-
I don't think "background" is a standard object. You should be using "document.body.style.backgroundColor" and to reset the value you would use a null string not a string with a space. This test page works fine for me in IE and FF <html> <head> <script type="text/javascript"> function setBackgroundColor(color) { document.body.style.backgroundColor = color; return; } </script> </head> <body> <button onclick="setBackgroundColor('#0000ff')">Blue</button><br /> <button onclick="setBackgroundColor('#ff0000')">Red</button><br /> <button onclick="setBackgroundColor('#00ff00')">Green</button><br /> <button onclick="setBackgroundColor('')">Reset</button><br /> </body> </html>
-
The code doesn't work "how"? Are you getting errors, if so what are they? Did the original query work? If so, I don't see why sasa's query wouldn't work for what you want. He basically used your query as a sub query to get the last 10 records, then the outer query would select one of those records. yes, of course you could do this through PHP, but why? Learn to use the power of databases instead of rebuilding the wheel. But, if you must know, if you wante to do it in PHP you could just dump the results into a multi-dimensional array and then randomize the array. But, using a query is really the way to go. EDIT: I just realized the code you posted in your reply is NOT the same code sasa posted. Did you TRY the code he posted or did you not realize it was modified?
-
Just do the whole table in a single query. No mess, no fuss: UPDATE table SET prior_status = current_status, current_status = 'inactive'
-
Depends on what you mean. If you are referring to three tables that are related and you need data for a total of three recordsets of combined data from those three tables then you would need to use joins. However, if you need three different sets of records each from each table then just run three queries.
-
Help Required on PHP & Mysql Login Security Check Script
Psycho replied to j.smith1981's topic in PHP Coding Help
It is simply a matter of escaping the user input based upon the usage. If you are using the input in a mysql query, then you want to use mysql_real_escape_string(). If you are going to use as text within an html page then you can use htmlentities() to escape an user input that might otherwise be interpreted as HTML code. For example, if the user has a username of "<BOB>" the browser would interpret that as an opening tag and could disrupt the output or not display it visually (although there are more malicious uses of such things). The htmlentities() function will convert the <> to the appropriate codes so they are not interpreted as HTML. E.g. "<Bob>" However, if repopulating user input into a form field you should only need to escape the quote marks. But each usage is different. -
You mean the latest row(s) not columns. Yes, it is as easy as sorting by a field and getting the first n records. Here is an example which you would need to modify according to your particular database: //Create query to get last 5 records based upon date field $query = "SELECT field1, field2, field3 FROM table ORDER BY date DESC LIMIT 0, 5"; $result = mysql_query($query); while($record = mysql_fetch_assoc($result)) { echo "{$record['field1']}, {$record['field2']}, {$record['field3']}<br />\n"; }
-
You figured it out? Isn't that what I said in my first response? Just giving you crap. As long as you solve your problem - that's the goal.
-
Help Required on PHP & Mysql Login Security Check Script
Psycho replied to j.smith1981's topic in PHP Coding Help
Ah, yes... Change this else if(count($result)!=1) To this else if(mysql_num_rows($result)!=1) -
Well, that code has an error in that it appears you are wanting to concatenate those stings but you have a semicolon after each one. Your question is way too vague to provide any meninginful response. If this is a one-time event where you need to concatenate those variables, then just do it that one time. Personally, I would define the string in the logic of the code and just echo the value in the HTML content like so: <?php $domain = "http://test.com"; $imageSrc = $domain . $image . $var3 . $var4 . $var5 . $var6; ?> <img src="<?php echo $imageSrc; ?>" /> But, assuming you need to do this for many different images, then I would suggest a function. I would create parameters for the function based upon what values are variable. I would assume $domain is constant and that image name is variable. But, not sure about the other variables. Here is an example: <?php function imageSource($image) { global $domain, $var3, $var4, $var5, $var6; $imageSrc = $domain . "/" . $image . $var3 . $var4 . $var5 . $var6; return $imageSrc; } $domain = "http://test.com"; ?> <img src="<?php echo imageSource('photo.jpg'); ?>" /><?php function imageSource($image) { global $domain, $var3, $var4, $var5, $var6; $imageSrc = $domain . "/" . $image . $var3 . $var4 . $var5 . $var6; return $imageSrc; } $domain = "http://test.com"; ?> <img src="<?php echo imageSource('photo1.jpg'); ?>" /> <img src="<?php echo imageSource('photo2.jpg'); ?>" /> <img src="<?php echo imageSource('photo3.jpg'); ?>" />
-
That makes no sense, you would be redefining the variables ($details[1], $details[2], etc.) on each iteration. I *think* this may be what you are after: //Create the array variable $locations = array(); //Iterate through the result set while($row = mysql_fetch_array($result)) { //Add to the multidim array using the record id as the index $locations[$row['id']] = $row; }
-
OK, I don't use JQuery so some of this is conjecture. But, it appears that in the update() function within the IF statement you are creating a function? If so, that probably explains what is going on. How does that created function get triggered? I think what is happening is that the first time update() is called it is creating the function, but the function is not being run (at least not within the context of update(). So, the return within that created function doesn't apply to the update function. That explains why you need to put the subsequent code within the else block. The return of the created function is not within the scope of update() so the code execution in update() would continue and always run the code after the IF statement (when it is not within an else statement). Again, since the returns within the created function are probably not run within the scope of update() you are getting an undefined error when the IF section of update is run because there is no return value sent back to changeISBN.
-
I have never had such a problem. Why do you assume it is JavaScript that is illogical and not your code? No offense, but everytime I have code that is doing something wacky it always turns out to be my own fault. In any event, it is impossible for anyone to help you if you do not post the code in question.
-
How to use javascript to check if at least one radio button is selected?
Psycho replied to ghurty's topic in Javascript Help
The following function will return the value of the selected or will return false if none of the values are selected function radioGroupValue(groupObj) { //Check if radio group is an array (i.e. has multiple options) if (!groupObj.length) { //Only one option in group return (groupObj.checked) ? groupObj.value : false; } //Multiple options, iterate through each option for (var i=0; i<groupObj.length; i++) { //Check if option is checked if (groupObj[i].checked) { //Return value of the checked radio button return groupObj[i].value; } } //No option was selected return false; } Just pass the radio button object to the function, such as: if (radioGroupValue(document.formName[radioGroupName])==false) { alert("You must select a value"); return false; } -
Hmm... I just tested and a framed form always POSTed in the frame it was called from. I could only get the form to POST to another frame or the entire window by specifically assigning a value for the other window or page in the form target. Not sure why you are getting different results.
-
try target="menu" FYI: This is just one example of why using frames is not generally used. If you are using PHP then you have the ability to manage menus and such independantly from the content. So why use frames?
-
Absolute path for images and how it affects load time...
Psycho replied to webmaster1's topic in PHP Coding Help
Yeah, I got it backwards. So sue me. Personally, I don't get a wrapped up in these types of details. If I'm writing new code and it doesn't work it takes two seconds to see that the path was defined incorrectly and to fix it. Sorry for the confusion.