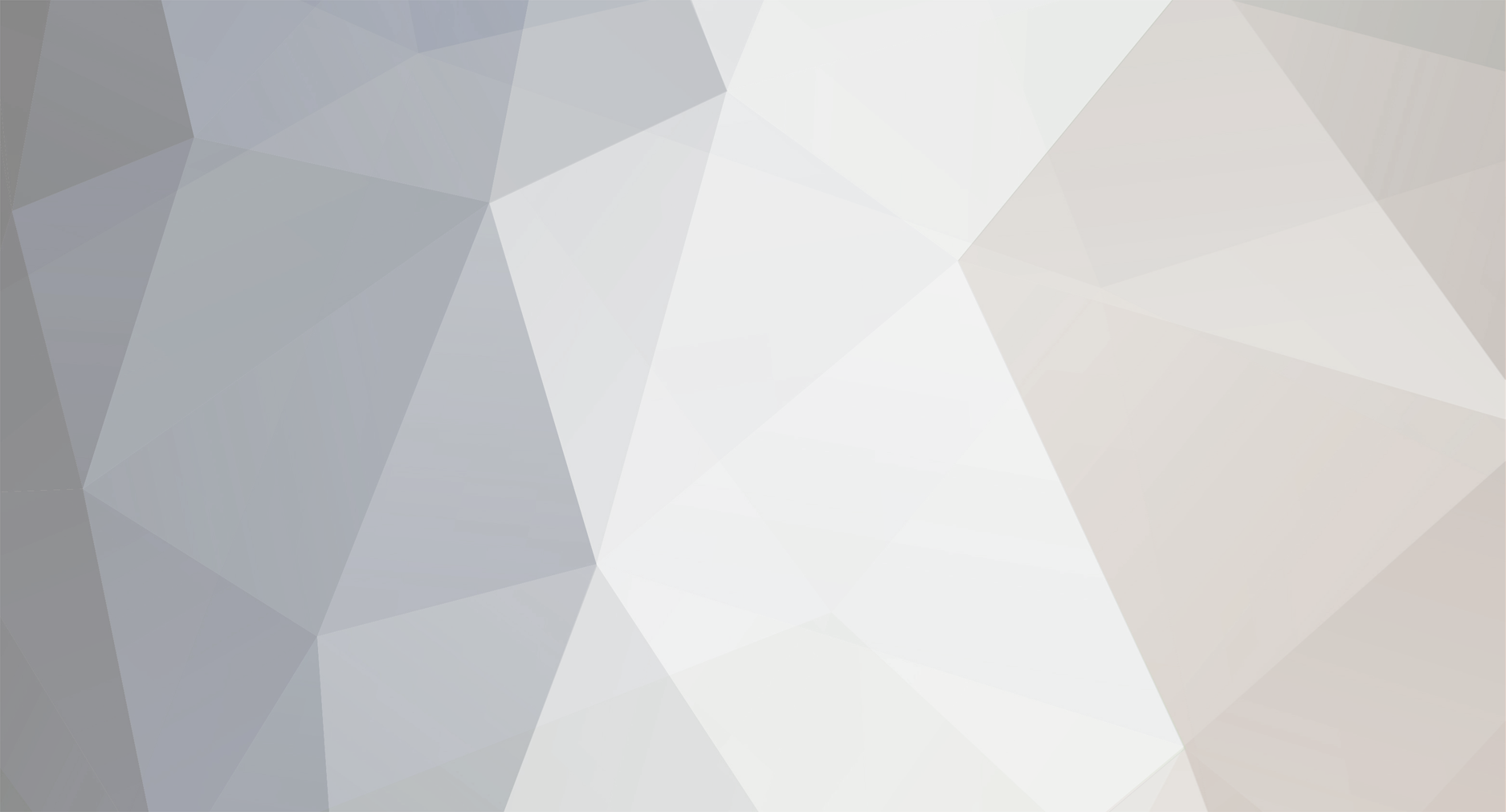
Psycho
Moderators-
Posts
12,160 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Comparing Two Arrays and Merging Values That Are a Near Match
Psycho replied to mkc's topic in PHP Coding Help
And can you provide the details of how the phone numbers are stored? What are the field types? Are they stored with formatting (e.g. (123) 456-7890), etc. Exactly how many characters are the ones that are cut off? -
Help required to fully and properly parameterize a SELECT query
Psycho replied to excelmaster's topic in PHP Coding Help
I use this approach all the time //Force all passed IDs to be integers and filter out 0/empty values $IDnumbersAry = array_filter(array_map('intval', $_POST['IDnumber'])); There is zero difference in the results of doing this than in adding all the values as parametized values in the query. however, with respect to the rest of the code, you have a check to see if the array contains any values before running the query. But, that check is buried under two switch statements and an if(). Assuming all the logic under those two switch statements require this array of values, you could do that check first. I'll add one last comment. I typically wrap my queries within functions or methods. The sanitizing of the input data would typically occur before the function/method is called. But, I don't want to risk passing potentially unsafe values to the function/method at some later point. So it would make sense to parametize the values anyway. I just prefer to run intval/array_filter before that so I can implement and error handling/messaging to the user. -
Comparing Two Arrays and Merging Values That Are a Near Match
Psycho replied to mkc's topic in PHP Coding Help
Just to be sure we aren't taking the long road, I have a question. Why not just update the phone numbers without the comparison. Now, I *think* what may be the issue is that you have the original DB and the current DB - and that the current DB has since been modified: phone numbers added, edited, deleted. So, you may just be wanting to correct those phone numbers which were copied from the original DB and cut off. Can you confirm if that is the case or what the situation is? That might help to create an easier solution. Second, we might not have to query the two databases, dump the results into arrays, process the arrays, and then update the database. This might be able to be handled direction through a query or two. Please provide details about the two tables along with the relevant fields. For example, what fields are used to determine that it is the same record, what is the format of the phone number data, etc. -
Maybe. I based my decision on the fact that he has two records in the users table that reference the same record in the adventures table. But, the two records in the user's table have different 'codes'.
-
OK, but - as I stated - the real problem seems to be with the table structure. That "solution" is really a hack. Is there some reason you need the 'code' defined in both tables? Here is how I would define those tables. adventure_questions q_id q_number question 1 1 DTCAP question 1 2 2 DTCAP question 2 26 1 CNROW question 1 27 2 CNROW question 2 51 1 MONFW question 1 52 2 MONFW question 2 users_adventures ua_id ua_username ua_code q_number ua_score 1 kjb31ca DTCAP 1 0 2 kjb31ca CNROW 2 10 3 kjb31ca MONFW 1 0 Note the use of q_number in BOTH tables. The query then becomes very simple SELECT * FROM adventure_questions q INNER JOIN users_adventures u USING(q_number) WHERE u.ua_username = '$_SESSION[username]' AND u.ua_code='$_SESSION[code]'
-
Assuming $result is a valid MySQL result set (with records) you would get crap for output. In this loop you are storing the records into a multi-dimensional array with the id as the index and then TWO values in the sub-array. $data = array(); while ($row = mysqli_fetch_assoc($result)) { $data[$row['id']] = array( 'title' => $row['title'], 'pris' => $row['pris'], ); } You then loop through that array as follows: foreach($data as $key => $value): echo '<option value="'.$key.'">'.$value.'</option>'; //close your tags!! endforeach; $value with be the subarray - but you are trying to echo it out. Do you want to echo out 'title' or 'pris' or some concatenation of the two?
-
I think I see the problem. You are only joining the two tables using the q_number/ua_question and that is joining records with different "codes" - which I don';t think you want. [PRO TIP: Give your field names values that make it obvious where foreign keys are used. You can even give them the same field name. What you have now is unintuitive]. So, using the example tables you provided above, this would be the result of the JOIN - before the WHERE clause is applied q_id | q_code | q_number | question | ua_id | ua_username | ua_code | ua_question | ua_score ----------------------------------------------------------------------------------------------------- 1 DTCAP 1 DTCAP question 1 1 kjb31ca DTCAP 1 0 >1 DTCAP 1 DTCAP question 1 3 kjb31ca MONFW 1 0 2 DTCAP 2 DTCAP question 2 2 kjb31ca CNROW 2 10 26 CNROW 1 CNROW question 1 1 kjb31ca DTCAP 1 0 >26 CNROW 1 CNROW question 1 3 kjb31ca MONFW 1 0 27 CNROW 2 CNROW question 2 2 kjb31ca CNROW 2 10 >51 MONFW 1 MONFW question 1 1 kjb31ca DTCAP 1 0 51 MONFW 1 MONFW question 1 3 kjb31ca MONFW 1 0 52 MONFW 2 MONFW question 2 2 kjb31ca CNROW 2 10 On the rows preceeded with '>' the q_code in the adventure_questions table doesn't match the ua_code in the users_adventures table. Perhaps you want to JOIN on both the ids and the code? If the code in the WHERE caluse is any of the three possible values I see above, then at least one of the first three records would be included in your result set (which all contain DTCAP). You are filtering out the records that don't contain the code in the second table, but not those from the first. You then, use the value from the first table. So, if my hunch is correct, this might be the correct query: $query = "SELECT * FROM adventure_questions q INNER JOIN users_adventures u ON q.q_number = u.ua_question AND q.q_code = u.ua_code WHERE u.ua_username = '$_SESSION[username]' AND u.ua_code='$_SESSION[code]'"; However, if that is the case, then your database is likely not structured correctly. It doesn't make sense to duplicate the code in both tables. It probably only needs to apply to the users_adventures table. So, in my opinioin, your current problem is a result of a bad database design.
-
No, create your query as a string and echo that. Don't put the query directly in the query function $query = "SELECT * FROM adventure_questions q INNER JOIN users_adventures u ON q.q_number = u.ua_question WHERE u.ua_username = '$_SESSION[username]' AND u.ua_code='$_SESSION[code]'" echo $query; $query_question=mysql_query($query) or die(mysql_error()); I think I see the problem, but will take me a couple minutes to write out.
-
Not when the array value is parsed within a double quoted string as the OP has. there are some scenarios where the array must be included within curly braces (e.g. multi-dimensional array). But, for a simple array value with a textual index it is perfectly value to not use quotes around the index inside a double quoted (or HEREDOC syntax) string. However, I personally don't do that. I always enclose my variables within curly braces and use quotes around the array indexes. See third echo in example #8: http://www.php.net/manual/en/language.types.string.php#language.types.string.syntax.double And later on that page is a reference that states
-
I didn't state to set it manually. I only stated that you should use numerically based indexes to ensure the "sets" of fields are logically grouped. You can do that programatically. You have a lot of different things going on to make this functionality work and, sorry, but I'm not going to take the time to rewrite it all. Even trying to tell you how to do it would likely take even more time. If you want to take the easy way out, keep your field names as you already have them (e.g. name="fieldname[]". There are downsides to that, but it *should* work with what you have now. Then you can process the form submissions like this: foreach($_POST['child-salutations'] as $index => $salutation) { //Get the other variables for this index $fname = $_POST['child-fname'][$index]; $lname = $_POST['child-lname'][$index]; $cday = $_POST['cday'][$index]; // etc. for all other fields //Process the data for this record }
-
You don't know how the site was built or what language it is even built in. How can we provide any help since we know even less than you do? You need to find out what the backend of the site is built in. Then you can determine if you want to (or can) build an interface into changing that data outside of the application that was used to build it.
-
Displaying information when image is clicked
Psycho replied to cerberus478's topic in Javascript Help
This is a JavaScript issue, and should be in the JavaScript forum. Second, if you are wanting help on a JavaScript issue then you need to post the relevant HTML source of the page. If you post the PHP that generates the page it makes it very difficult for us to backward engineer the code to determine what the output will be like. In fact, when trying to create JavaScript functionality such as this, you should start with a hard-coded page with a couple of representative records. Once you get it working - THEN create the PHP code to create the page dynamically. I haven't read through all of the code you posted - but it looks awfully complicated based upon what you say you want to do. << Moved to JS forum >> -
A few other comments: It doesn't make sense to have a Team object that only contains the name. It should contain all of the relevant details about the team (such as the results). No need to run multiple queries. Just decide what properties (if any) should be directly accessible as opposed to using setter/getter methods. I also agree that the separate classes probably aren't needed. // spawn a new Team object if query is valid, if not throw exception and end via try/catch... if ($result = $this->database->single()) { return new Team($result); // create new Team passing $result to Team constructor } else { throw new exception ('No Valid Team returned'); } The else block is unnecessary here. If the if() condition passes, the 'return' will exit the method. Also, I think it is good practice to have a failure return false. So, this could be simplified as: // spawn a new Team object if query is valid, if not throw exception and end via try/catch... if ($result = $this->database->single()) { return new Team($result); // create new Team passing $result to Team constructor } throw new exception ('No Valid Team returned'); return false;
-
OK, let's step back a moment. I would advise against using non-named indexes in your form field names when you have "grouped" form fields. The reason is that you can't reply upon the ones with the same index being from the same group. A checkbox field that is not checked is not sent in the post data. Even if you have no checkbox fields, it's just good practice. I would suggest the following - create the 'groups' of fields as a multi-dimensionsal array. Make the first dimension the index of the group and the second dimension the field names. For example: The first group would look like this (NOTE: ids MUST be unique): <select name="contact[0][child-salutations" id="child-salutations_0"> <input type="text" name="contact[0][child-fname]" id="child-fname_0" class="style" /> <input type="text" name="contact[0][child-lname]" id="child-lname_0" class="style" /> <select name="contact[0][cday]"> <select name="contact[0][cmonth]"> <input name="contact[0][cyear]" type="text" maxlength="4" size="4" class="year"> <input name="contact[0][living]" type="radio" id="living-other_0" class="living-other"/> <input name="contact[0][living]" type="radio" id="living-own_0" class="living-own"/> <input name="contact[0][child-line1]" type="text" id="child-line1_0" size="20" class="style" /> <input name="contact[0][child-mobile]" type="text" id="child-mobile_0" class="style" /> <input name="contact[0][child-office]" type="text" id="child-office_0" class="style" /> <input name="contact[0][child-email]" type="email" id="email_0" class="style" /> The second set would look like this <select name="contact[1][child-salutations" id="child-salutations_1"> <input type="text" name="contact[1][child-fname]" id="child-fname_1" class="style" /> <input type="text" name="contact[1][child-lname]" id="child-lname_1" class="style" /> <select name="contact[1][cday]"> <select name="contact[1][cmonth]"> <input name="contact[1][cyear]" type="text" maxlength="4" size="4" class="year"> <input name="contact[1][living]" type="radio" id="living-other_1" class="living-other"/> <input name="contact[1][living]" type="radio" id="living-own_1" class="living-own"/> <input name="contact[1][child-line1]" type="text" id="child-line1_1" size="20" class="style" /> <input name="contact[1][child-mobile]" type="text" id="child-mobile_1" class="style" /> <input name="contact[1][child-office]" type="text" id="child-office_1" class="style" /> <input name="contact[1][child-email]" type="email" id="email_1" class="style" /> You can then iterate through each group of fields like this foreach($_POST['contact'] as $contact) { //Process the contact record }
-
Building upon mac_gyver's response: $inputArray = json_decode($string, true); $uuVals = array_map(function ($var) {return $var['uu'];}, $inputArray); echo '<pre>' . print_r($uuVals, 1) . "</pre>"; Output Array ( [0] => en.wikipedia.org/wiki/HTTP_cookie [1] => en.wikipedia.org/wiki/HTTP_cookie [2] => en.wikipedia.org/wiki/HTTP_cookie [3] => en.wikipedia.org/wiki/HTTP_cookie [4] => en.wikipedia.org/wiki/HTTP_cookie [5] => en.wikipedia.org/wiki/United_States [6] => en.wikipedia.org/wiki/United_States [7] => en.wikipedia.org/wiki/United_States [8] => en.wikipedia.org/wiki/United_States [9] => en.wikipedia.org/wiki/United_States [10] => en.wikipedia.org/wiki/United_States [11] => en.wikipedia.org/wiki/United_States [12] => en.wikipedia.org/wiki/United_States [13] => en.wikipedia.org/wiki/United_States [14] => en.wikipedia.org/wiki/United_States [15] => en.wikipedia.org/wiki/United_States [16] => en.wikipedia.org/wiki/United_States [17] => en.wikipedia.org/wiki/United_States [18] => en.wikipedia.org/wiki/United_States [19] => en.wikipedia.org/wiki/United_States [20] => en.wikipedia.org/wiki/United_States [21] => en.wikipedia.org/wiki/United_States [22] => en.wikipedia.org/wiki/United_States [23] => en.wikipedia.org/wiki/United_States [24] => en.wikipedia.org/wiki/United_States ) You can remove the duplicates, if needed, using array_unique()
-
Simple Problem: Class Syntax error results in parsing error
Psycho replied to Chezshire's topic in PHP Coding Help
Using the editing tools, click the <> button to add code. Or, you can manually enter the BBCode around the code content: [ code ] and [ /code ] (without the additional spaces). And, maybe you do have indentation in your code to display the structure. BUt, if you do, you missed something: You have this: if(mysqli_num_rows($result) > 0) {#there are records - present data while($row = mysqli_fetch_assoc($result)) {# pull data from associative array $this->Questions[] = new Question((INT)$row['QuestionID'],$row['Question'],$row['Description']); } }//end of constructor }//end of survey class When indented properly, it is obvious there is an ending bracket missing. if(mysqli_num_rows($result) > 0) {#there are records - present data while($row = mysqli_fetch_assoc($result)) {# pull data from associative array $this->Questions[] = new Question((INT)$row['QuestionID'],$row['Question'],$row['Description']); } }//end of constructor }//end of survey class The end constructor bracket matches up with the if() opening bracket. You also have an extra closing bracket at the end which is out of place. Do not be afraid of line breaks in your code. I see some lines where it appears you are trying to combine a couple of things for brevity. It's not worth it. Add plenty of line breaks, indentation and comments to your code. You will save many more hours in debugging than it takes to add those things. There are plenty of things that need improvement in this code, but I didn't bother changing them. Here is the code with the corrections made to the brackets <?php require '../inc_0700/config_inc.php'; #provides configuration, pathing, error handling, db credentials $config->titleTag = smartTitle(); #Fills <title> tag. If left empty will fallback to $config->titleTag in config_inc.php $config->metaDescription = smartTitle() . ' - ' . $config->metaDescription; //END CONFIG AREA ---------------------------------------------------------- get_header(); #defaults to header_inc.php $mySurvey = new Survey(1);//all our code happens in here if ($mySurvey->isValid) { echo ' The title of the surevis is ' . $mySurvey->Title . ' <br />'; echo ' The title of the surevis is ' . $mySurvey->Description . ' <br />'; } else { echo ' No such survey exists'; } dumpDie($mySurvey); //like var_dump inside/available this app because of common file get_footer(); #defaults to footer_inc.php class Survey { //class creates one object at a time, creates as many as you want //create properties public $SurveyID = 0; public $Title = ''; public $Description = ''; public $isValid = FALSE; public $Questions = array();// how we add an array of questions to a survey function __construct($id) { $id = (int)$id; //cast to an integer - stops most sequal injection in this case if(!$id) { return false; //don't bother asking database, there is no frog, don't ask - no data no dice } $sql = "select Title, Description, SurveyID from sp14_surveys where SurveyID = $id"; $result = mysqli_query(IDB::conn(),$sql) or die(trigger_error(mysqli_error(IDB::conn()), E_USER_ERROR)); //echo '<div align="center"><h4>SQL STATEMENT: <font color="red">' . $sql . '</font></h4></div>'; if(mysqli_num_rows($result) > 0) { #there are records - present data while($row = mysqli_fetch_assoc($result)) { # pull data from associative array $this->SurveyID = (INT)$row['SurveyID']; $this->Title = $row['Title']; $this->Description = $row['Description']; } $this->isValid = TRUE; } @mysqli_free_result($result);//at symbol surpresses multiple copies of an error silences a line basically $sql = "select * from sp14_questions where Survey14 = $id"; $result = mysqli_query(IDB::conn(),$sql) or die(trigger_error(mysqli_error(IDB::conn()), E_USER_ERROR)); if(mysqli_num_rows($result) > 0) { #there are records - present data while($row = mysqli_fetch_assoc($result)) { # pull data from associative array $this->Questions[] = new Question((INT)$row['QuestionID'],$row['Question'],$row['Description']); } } ### <==== THIS IS WHAT WAS MISSING }//end of constructor }//end of survey class //BEGIN new question object class Question { public $QuestionID = 0; public $Text = ''; public $Description = ''; function __construct($QuestionID, $Question, $Description) { $this->QuestionID = $QuestionID; $this->Question = $Question;// field is named Question $this->Description = $Description;// the context info about the question asked }//END question Constructor }// END question Class //} ### <==== THIS WAS NOT NEEDED ?> -
You should add each child contact information as a separate record instead of trying to concatenate all of them into a single record. You can't store an array - as an array - into the database. You are trying to store an array into a field meant to hold a string value. (There is no array type of DB field). You *could* concatenate all of the arrays into single values, but that's a bad idea. Process each record individually as stated above.
-
You didn't show the query you used so this may not work out-of-the-box. But, you should be able to change your query to do the calculations for you. Give this a try SELECT dayname, hour, SUM(IF(widtype='type1', Output, 0)) AS type1_total, SUM(IF(widtype='type2', Output, 0)) AS type2_total FROM table GROUP BY dayname, hour
-
First off, there is no reason to need to run 16 queries to get the counts of 16 different "fruit" or "electronics". Assuming these two types of objects need two different tables, you can get the counts you want with ONE query. Second, if your host is limiting you to 999 connections per hour you will need to implement some type of caching to work around this problem - or get a new host. But, fixing the code to use only one query would alleviate the problem considerably.
-
could somebody tell me how to correct this
Psycho replied to computernerd21's topic in PHP Coding Help
Give your variables meaningful names. $i and $k don't tell you what they are or what their purpose is. It may not seem like a big deal when you are actively working on the code. But, if you have to go back to the code in the future it will take longer to decypher what it is doing to make whatever bug fixes or enhancements you want to make. -
could somebody tell me how to correct this
Psycho replied to computernerd21's topic in PHP Coding Help
Try this <?php function myt($border, $total_cols, $total_rows, $words) { $borderParam = ($total_cols==1) ? "border='{$border}'" : ''; $table = "<table {$borderParam}>\n"; for($row=0; $row<$total_rows; $row++) { $table .= "<tr>\n"; for($col=0; $col<=$total_cols; $col++) { $table .= "<td>{$words}</td>\n"; } $table .= "</tr>\n"; } $table.="</table>"; return $table; } ?> <html> <head></head> <body> <?php echo myt(1, 2, 4, 'lalalala'); ?> </body> </html> -
Yes, it is possible, but probably not with fpdf. I don't have any experience with fpdf but I have extensive experience with dynamic PDF creation via a browser application. In a prior company we provided a web form for the user to enter data for a specific document. Once submitted, the back end would utilize PostScript to merge the static content with the content the the user entered to generate a PDF using the Acrobat engine. That allows a huge amount of flexibility, and I'm sure it would allow the user of editable fields to be added to the final PDF since it is using the Acrobat engine - but was not something that we needed. That is probably something that is well beyond what you need. Not to mention, it would require you to learn several new technologies and the cost would be prohibitive. But, I have some ideas you could look into: First, see if there are any third party tools that allow you to create a PDF with editable fields. There may be, but such a full-featured API will likely come with a cost. Plus it may require additional installs to the web server. If you are on a shared host, that may be an issue. Second, see if you can find a utility to create PDFs that can utilize an existing PDF as a template. You could have a blank page with just the editable fields then add your variable content. However, if the editable fields are going to be variable in size and position this would not server your purpose. Third, instead of an editable PFD, provide the users a web page to enter their content and then use that content, along with the CSV content, to generate the PDF
-
There is one thing you can try. 1. Use a RegEx to find valid records as you are now 2. Use the file() function on the text file to read the contents of the file into an array (each line is an element in the array). 3. Use array_diff() between the two arrays to get all the lines that do not contain valid email addresses.
-
Why would no rows be returned for a SELECT DISTINCT query?
Psycho replied to excelmaster's topic in PHP Coding Help
If your tables are properly structured why do you need to use DISTINCT? Your store_master should only contain one record for each unique store and the products table should contain one record for each unique product. My guess is that your query is failing. You need to add proper code to debug the DB errors. As for your second request, that is quite a lot to ask in a forum post. There are a multitude of things that are required to accomplish that. First off you need to determine what the UI will look like. You could have a drop-down list with an optional text field or you could implement a custom JavaScript UI element that works like a drop-down but allows custom values to be entered. I would suggest the former to get a working solution, then you can add the latter if you want. Second is you need to add the back end functionality to accept the values. You'll need to detect if a new value was sent. If so, attempt to save the value, but add handling in case it is a duplicate. -
How is the content provided? Is it a text file, a form post, or what? If this isn't provided from some programatic output that you can 100% rely upon then you should account for variability. If user entered, they may enter a comma or semi-colon between each record. It would not be hard to create a process to catch those scenarios. Also, the RegEx you have will not work for some email addresses. It can result in false positives and false negatives.