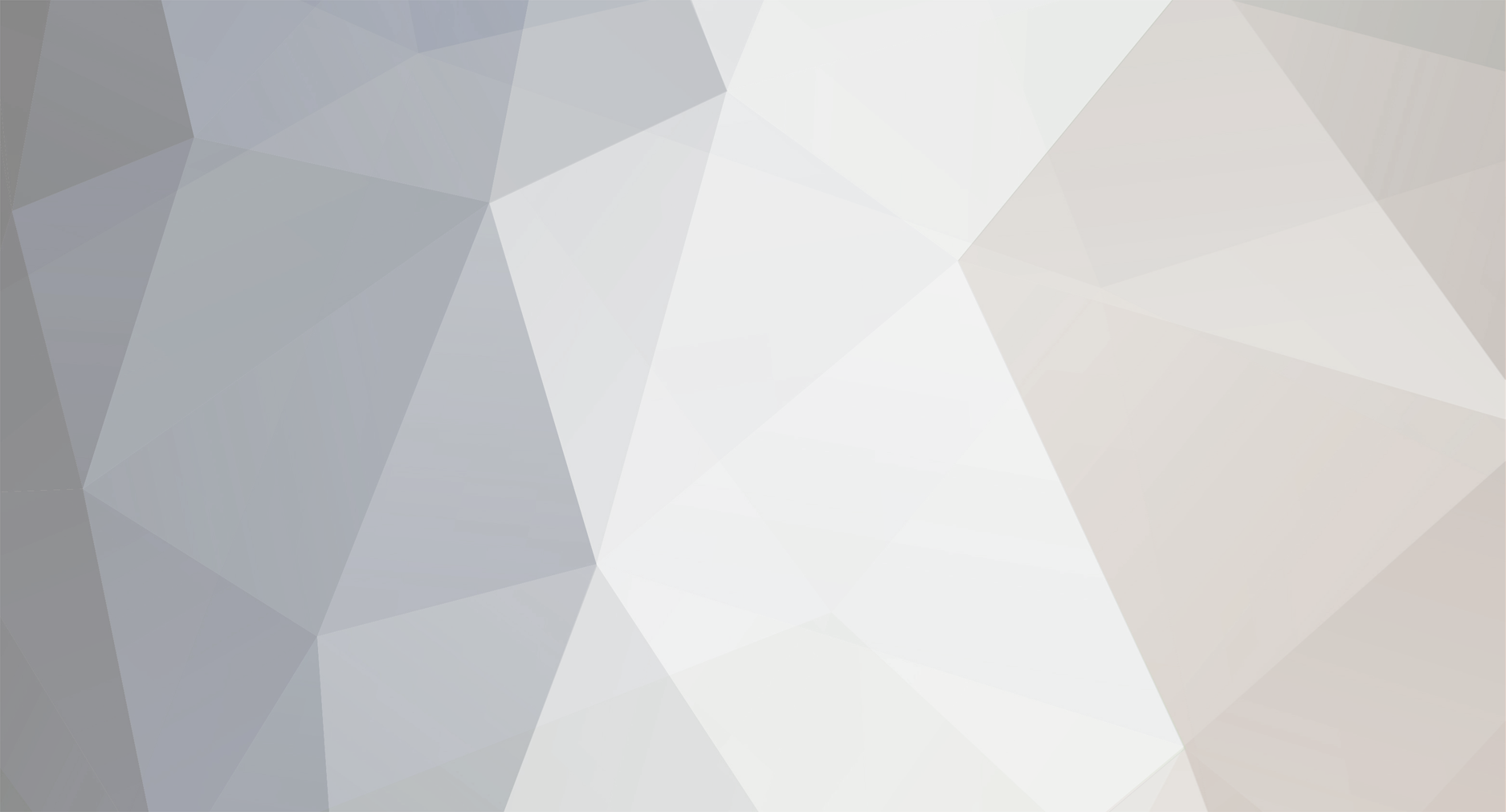
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
You only need to determine which button the user selected. Then take the passed IDs from the checkboxes and run the query. The 'case' in you example code was for 'update-delete' which didn't seem logical for the specific process you were asking about. But, I would think all/most of your processes would be using the selected check boxes - so I would get those values before the switch statement. I don't know what logic you have to determine the value used for the switch(), but here's a quick example of how you can do everythign pretty simply. I made up my own switch values for illustrative purposes <?php //Force all passed IDs to be integers and filter out 0/empty values $prodIDsAry = array_filter(array_map('intval', $_POST['prodIDs'])); //Format list into a comma separated string $prodIDList = implode(', ', $prodIDsAry); //Use your logic to determine the value to be used for the switch statement $switchValue = '??????'; //Detemine the process to run switch($switchValue) { case "toggle_purchased": //Toggle purchased flag for selected records where the //purchase_later_flag is NOT 'Y' if(!empty($prodIDList)) { $query = "UPDATE shoplist SET purchased_flag = IF(purchased_flag='Y', 'N', 'Y') WHERE prod_id IN ({$prodIDsAry}) AND NOT purchase_later_flag <> 'Y'"; $statement = $conn->exec($query); } break; case "toggle_purchase_later": //Toggle purchased flag for selected records where the //purchased_flag is NOT 'Y' if(!empty($prodIDList)) { $query = "UPDATE shoplist SET purchase_later_flag = IF(purchase_later_flag='Y', 'N', 'Y') WHERE prod_id IN ({$prodIDsAry}) AND NOT purchase_later_flag <> 'Y'"; $statement = $conn->exec($query); } break; case "delete_selected": //Delete only the selected records if(!empty($prodIDList)) { $query = "DELETE FROM shoplist WHERE prod_id IN ({$prodIDsAry})"; $statement = $conn->exec($query); } break; case "delete_all": //Delete all the records $query = "DELETE FROM shoplist"; $statement = $conn->exec($query); break; } ?>
-
Two other things: 1. That is not the correct way to get the selected value of a select list. You will need to use the selected index 2. If you are adding parameters to the URL, you start with a question mark and then use an ampersand between each additional item. <SCRIPT type="text/javascript"> function getSelectvalue(selID) { selObj = document.getElementById(selID); return selObj.options[selObj.selectedIndex].value; } function search() { //Get values of options var color = getSelectvalue('colour'); var material = getSelectvalue('material'); var countryoforigin = getSelectvalue('countryoforigin'); var budget = getSelectvalue('budget'); //Define default variables var search = "/products/mens/t-shirts/"; var params = new Array(); //Determine optional parameters if(color != "(Any)") { params[params.length] = "colour=" + color; } if(material != "(Any)") { params[params.length] = "material=" + material; } if(countryoforigin != "(Any)") { params[params.length] = "countryoforigin=" + countryoforigin; } if(budget != "(None)") { params[params.length] = "budget=" + budget; } //Add selected parameters to search URL if(params.length) { search += '?' + params.join('&'); } //Return results alert(search); window.location = $search; } </SCRIPT>
-
What do you base that comment on? I've never seen any information that states an IP address is considered PII (personally identifiable information) that has to be safeguarded. Although it would be a bad business decision to expose IP addresses, they are 'public'. This page, supposedly a Google blog seems pretty clear that it is not confidential information but that they take the conservative approach to protect the information. While I agree that blocking by IP is not foolproof, there really isn't much that is. Even large sites use IP blocking - my company (a very large world-wide organization with thousands of employees) has been struggling with Google detecting us as potentially malicious. Many times Google will require us to enter in a captcha to perform a search. If the problems are with automated processes, those can usually be thwarted with UI preventatives (such as captcha or capturing JavaScript events - e.g. onclick). But, if it is a malicious user, then sometimes an IP block is the only way. Yes, they can spoof their IP address, but it may not be worth that persons trouble to do that and they will turn their efforts elsewhere.
-
Guru Barand has a class for doing this called baaSelect. I'm not sure if the class uses AJAX or not. If the lists of options are relatively small, I would suggest querying all the options and outputting them as JavaScript arrays so all the logic can happen on the client side. If you use AJAX there will typically be a delay when making a selection in order to populate the other list(s). In some cases it is possible for errors to occur if you don't enable/disable things to prevent them. If it is all done client-side it takes place nearly instantaneously. Only if the lists are very extensive and loading them into the HTML source will be a performance issue would I make these AJAX enabled.
-
"pull through"? If you want those graphs you would need to reverse engineer the code to figure out what code is being used to create the graphs and then implement that in your pages. Or, build your own graphs. A very poor hack would be to use some sort of scraping of the MySQL pages to copy the code out, but it would probably open you up to potential malicious issues and could even be more complicated than just doing it the right way. Edit: There *could* be some sort of API built into MySQL that I am not aware of, but I doubt it.
-
EDIT: Ch0cu3r beat me to it and covered most of what I state below. But, I think he missed the point that the values need to be toggled. If I am reading the above query correctly it only changes the value from 'Y' to 'N'. But, the OP also needs the 'N' values changed to 'Y'. I would agree with that statement. I think the core of the problem is how the UI workflow is built. As a user I would be thoroughly confused on how to use that page. I think you are approaching the problem from a "programatic" perspective. You should think about the problem that the user is trying to solve and the workflow that would make sense to them. But, I don't know enough about your application or the users in order to provide any suggestions, so we'll deal with what you have. Regarding the two columns for already purchased and items to purchase later you could combine those into a single column with three possible values to simplify things. Or, at the very least, it would be better to change the current possible values to 0 and 1, because those can be interpreted as True/False. I'm not going to comment on your current code, but rather provide what I think is the solution. First of all, you only want to run the query against the records that the user has selected (we'll address the first record later).The checkboxes should be named as an array with the value being the ID of the records such as this (Don't create your checkboxes with a name like 'ckeckboxes'! That's just stupid (sorry to be blunt). Give all variables, fields, etc. names that give you an idea of what they contain. In this case, the checkboxes should contain the ID of the products, so 'prodIDs' would make more sense): <input type="checkbox" name="prodIDs[]" value="5" /> So, to ensure the query is only run against the selected products you would use an IN condition as part of the WHERE clause. Of course you would do some pre-processing on the passed IDs. WHERE prod_id IN (1, 2, 3) Second, since you don't want to run this for records where the "items to be purchased later" is true, you would add an exclusion to the where clause WHERE prod_id IN (1, 2, 3) AND NOT purchase_later_flag -- I.e. value is false Lastly, for the records that do match the where clause you want to toggle the current value. You can do that simply set the value based upon the current value. If the values are 0/1 this is VERY easy SET purchase_now_flag = NOT purchase_now_flag Everything put together: //Force all passed IDs to be integers and filter out 0/empty values $prodIDsAry = array_filter(array_map('intval', $_POST['prodIDs'])); if(count($prodIDsAry)) { //No valid IDs passed - add error condition } else { //Create comma separated list of selected product IDs $prodIDList = implode(', ', $prodIDsAry); //Create query to update selected records $query = "UPDATE shoplist SET purchase_now_flag = NOT purchase_now_flag WHERE prod_id IN ({$prodIDList}) AND NOT purchase_later_flag"; } If you don't change the columns to use 0/1 and instead continue to use Y/N (which I would advise against) this query should work $query = "UPDATE shoplist SET purchase_now_flag = IF(purchase_now_flag='Y', 'N', 'Y') WHERE prod_id IN (1, 2, 3, 4) AND NOT purchase_later_flag <> 'Y'";
-
<?php print "Hello world, I need a good brain!"; ?>
Psycho replied to phpallwinner's topic in Introductions
If these sites don't have an API to allow you to perform a search such as you need, then it's a pretty good chance that trying to scrape their sites is against the TOS for those sites.- 4 replies
-
- php search
- php search engine
-
(and 1 more)
Tagged with:
-
<?php //Start session and enable error reporting session_start(); error_reporting(E_ALL | E_STRICT | E_NOTICE); ini_set('display_errors', '1'); //Check if the user had voted in the last 24 hours if(isset($_COOKIE['voted'])) { $expireString = date('m-d-Y h:i:s', $_COOKIE['voted']); $output = "Sorry, you can only vote once every 24 hours. You can vote again after $expireString"; } else { //Connect to DB $host ="localhost"; $user ="jingleko_reload"; $pwd ="*******"; $dbname ="jingleko_reloader"; $link = mysqli_connect($host,$user,$pwd,$dbname) or die(mysqli_error()); //Update count for selected song: THIS IS WHERE I MADE THE CHANGES $number = intval($_GET['Nr']); $query = "UPDATE voting SET Votes = Votes+1 WHERE Nr = $number"; $result = mysqli_query($link, $query); if (!$result) { //Query failed #die(mysqli_error()); //Uncomment for debugging only $output = "There was a problem processing your request."; } elseif(!mysqli_affected_rows($link)) { //No records were updated $output = "The song you selected doesn't exist." } else { //Vote was registered $query = "SELECT Song FROM stemming WHERE Nr = $number"; $result = mysqli_query($link, $query); $row = mysqli_fetch_assoc($result); $songSafeHtml = htmlspecialchars($row['Song']); $output = "You voted for <b>$songSafeHtml</b><br> U het gestem vir <b>$songSafeHtml</b></br>"; //Set cookie to prevent multiple votes $expire = time() + (60 * 60 * 24); //Set expiration for 24 hours setcookie('voted', $expire, $expire); //Send confirmation email $to = "beheer@vlaamseradio.tk"; $subject = "There was a vote"; $message = "Someone voted for $songSafeHtml."; $header = "From: systeem@jinglekot.cu.cc \r\n"; $retval = mail($to, $subject, $message, $header); } } ?> <html> <head></head> <body> <?php echo $output; ?> </body> </html>
-
$query="SELECT Song FROM stemming WHERE Nr=$songSafeHtml"; You aren't using $songSafeHtml anymore - that shouldn't be in your query.
-
Why do so Many Beginners Use a lot of Bad Practices?
Psycho replied to mogosselin's topic in Miscellaneous
My thoughts: Assuming the user does know how to write functions and what they are for, this is probably due to a lack of planning. They know what they want for the end result and just start writing code not knowing "how" they are going to get to the end result and just figure it out as they go. That's why we see so many people who have coded themselves into a corner, so to speak. Either they will have to implement a "hack" to fix their issue or rewrite their code from scratch to fix the issues they created. I have to admit I do this sometimes - especially when I have an idea on how to code some specific process that I want to get working before I forget. But, I will always go back and refactor the code to pull out parts of code that belong in separate functions or classes. I think this is due to some people not being able to conceptualize the process of separating the logic from the presentation. They think in a linear fashion. It just doesn't make "sense" to them to write the logic to create the output without actually echoin'g the output right then and there. Many of these people probably don't use a full functioning IDE (e.g. Notepad++) and even if they do, don't know how to use 90% of the features. It's much more rewarding to write code to do something new than to read a manual about an IDE on how to use it properly. Echoing Jacques1 comments, I think many of these users are copy/paste programmers that don't know what have of the code they put together even does. They may not even understand simple basics such as parameters (optional vs. required). Heck, I've seen posts where a person passed a value to a function that was completely opposite of what should have been passed. When I asked him why he passed that value he hadn't looked at the manual, but that he thought it made sense. I work with professional developers that don't remember to do this! But, you can group this into lots of different security risks that are not appropriately handled. Without having knowledge of the different risks it's difficult for someone to know how to handle it. And, I also somewhat agree with Jacques1 response that PHP attracts the wrong people. I wound't say they are the wrong people so much as it attracts the completely uninitiated people that have no programming background. My guess is many of these people start with just HTML and then want to add some functionality. They may have some experience with JavaScript which is similar to PHP. But, pretty much every host out there supports PHP and there is a huge support community (such as this site). So, the natural progression is to start with PHP. They don't want to take a few months to learn programming basics let alone learn PHP. It's just easier to find a tutorial around a feature they want to implement and copy/paste it and try to tweak it for their needs. -
<?php //Connect to MySQL Server include 'Connect.php'; mysql_connect($host, $dbusername, $dbpassword); //Select Database mysql_select_db($dbname) or die(mysql_error()); // Escape User Input to help prevent SQL Injection $first_name = mysql_real_escape_string(trim($_GET['first_name'])); // Retrieve data from Query $query = "SELECT id, LRN, first_name, last_name, grade, section, FROM student_information WHERE first_name LIKE '%{$first_name}%'"; $result = mysql_query($query) or die(mysql_error()); //Generate the output $searchResults = ''; if(!mysql_num_rows($result)) { $searchResults = "<tr><td colspan='8'>No results found</td></tr>\n"; } else { // Insert a new row in the table for each person returned while($row = mysql_fetch_array($result)) { $student_id = $row[id]; // - ??? Not sure if this is the correct field name $searchResults .= "<tr>\n"; $searchResults .= " <td>{$row['LRN']}</td>\n"; $searchResults .= " <td>{$row['first_name']}</td>\n"; $searchResults .= " <td>{$row['last_name']}</td>\n"; $searchResults .= " <td>{$row['grade']}</td>\n"; $searchResults .= " <td>{$row['section']}</td>\n"; $searchResults .= " <td><a href='View_Profile.php?id={$student_id}'>View</a> </td>\n"; $searchResults .= " <td><a href='Admin_Edit_Student_Info.php?id={$student_id}'>Update</a></td>\n"; $searchResults .= " <td><a href='Delete.php?id={$student_id}'>Delete</a></td>\n"; $searchResults .= "</tr>\n"; } } ?> <html> <head></head> <body> <table border='1' cellpadding='10' cellspacing='1' align='center'> <tr align='center'> <th>LR Number</th> <th>F i r s t N a m e</th> <th>L a s t N a m e</th> <th>Grade</th> <th>Section</th> <th>View</th> <th>Update</th> <th>Delete</th> </tr> <?php echo $searchResults; ?> </table> </body> </html>
-
You should ONLY be passing the ID of the record. After incrementing the vote count you should do a SELECT query to get the name of the song for display purposes.
-
-
@Jacques1: I wouldn't beat him up too bad about that - I see it all the time, even by advanced users. But, that is a pet peeve of mine. Even worse is when I see someone use an if/else condition where there is no logic included for the if() condition and only the else condition because the user wasn't able to properly create the condition.
-
C'mon man. issest is not the same as isset
-
You need to sloooooooow down. I'm sure there are plenty of things you want your application to have. But, you have to learn how to differentiate things you MUST have, things you really WANT to have and things that would be NICE to have. First, build the ability for the user to post comments using htmlentities() or htmlspecialchars() to prevent any malicious content from making it's way into the output. Then, once you have that working and have implemented any other high value features you can go back and figure out how to properly allow clickable hyperlinks.
-
I agree that strip_tags() should not be used to prevent cross-site scripting, and removing tags from user input is typically a stupid idea. In fact,it always irks me when I see people trying to prevent certain characters in fields such as name and end up disallowing characters that people use inthier names (dash, apostrophe, accented characters, etc.) But, strip_tags() is a useful function. There are many scenarios where you might have content that is used for multiple purposes - browser output being only one. For example, you might have content for a page which includes hyperlinks and formatting code. If you wanted to re-purpose the content for output to a text file or PDF that doesn't support those tags you would want to remove them. You don't want users creating their own links. Let them put in a URL in plain text then programatically make it a hyperlink when you display it. That's what this site does.
-
No it does not work. A value of "<body>", for example, would pass the test when it should fail. Be sure you understand the order of operations. Same concept as when you have a mathematical expression - if you do things out of order you will get different results. In this case, the expressions are run from the inside-out. So, first you are executing empty($username). That function ONLY returns a Boolean TRUE or FALSE. Then you are executing strip_tags() on the result of the above. Well, strip_tags() won't do anything on a Boolean value. Plus, if you don't want to allow tags in the username, then you should strip them from the value that you end up saving as well. $username = strip_tags(trim($_POST["username"])); if(empty($username)) { echo "username is required"; } else { //Continue However, there is no technical reason you can't support tags in the username (or most values). You should be properly escaping the values when you output to HTML anyway. For example, there are plenty of people on this forum that have HTML tags in their names.
-
Then, most likely, there are no matching records in the database.Echo the query to the page and then run it in PHPMyAdmin, or whatever DB management app you are using. You could also add some debugging code to verify this $result = mysqli_query($link,$sql); //Debugging lines $rowcount = mysqli_num_rows($result); echo "There were {$rowcount} record returned for the query:<br>{$sql}";
- 7 replies
-
- mysqli
- fatalerror
-
(and 1 more)
Tagged with:
-
Look at the response in this forum post: http://stackoverflow.com/questions/21020294/preventing-session-fixation-by-ensuring-the-only-source-of-creating-a-session-sh It seems that a malicious attack isn't about the user trying some random session ID. The malicious user would trick an unsuspecting user to visit an unsecured site using a session ID that the malicious user has defined. once the user logs in the malicious user could then hijack their session.
-
To follow up on Jacques1's response: Yes, fetch_row will work but, as the manual states, it will return a numerically based index. So, you would reference the records using numbers based on the order of the fields in the select clause (0 for the 1st field, 1 for the second, etc.). Whereas, fetch_assoc returns an array using the names of the fields (or aliases if you use them) as the index of the array. It has nothing to do with whether you used a JOIN in your query or not. So, if the other uses of it are working for you, it is because you are referencing the fields using numerically based indexes. I encourage you to always use associative arrays when possible. It makes your code easier to read and problems can occur if you use numerical indexes and make any changes in table structures or in the fields returned in the query. I agree, though, that the manual was pretty clear on the explanation. And, it kinda irks me when people come on here asking for help, we point to the entry in the manual that explains their problem, then they state that it's too "complicated". If the PHP manual is too complicated (which is one of the best technical manuals I've ever used) for someone then maybe programming isn't what they should be doing. I'm not meaning that as a derogatory comment to you. Instead, I encourage you to pick a single function that you already know pretty well and read the entire entry in the manual for that function. Figure out what the different sections on the page are for and how they are laid out. Once you understand how the manual is constructed it becomes easier to read an entry for something that is new to you. Anyway, I'll be generous and provide some examples on three flavors of the _fetch functions. Assuming you run this query: $query = "SELECT name, address, phone FROM people WHERE id = 22"; $result = mysqli_query($link, $query); Three of the _fetch functions would create arrays as follows fetch_row (numerically based indexes) $row = mysqli_fetch_row($result) array ( 0 => David Smith 1 => 123 main Street 2 => 213-456-7890 ) i.e. to reference the name you would use $row[0] fetch_assoc (associative based indexes) $row = mysqli_fetch_assoc($result) array ( name => David Smith address => 123 main Street phone => 213-456-7890 ) i.e. to reference the name you would use $row['name'] fetch_array (return BOTH a numerical and associative based array) $row = mysqli_fetch_array($result) array ( 0 => David Smith 1 => 123 main Street 2 => 213-456-7890 name => David Smith address => 123 main Street phone => 213-456-7890 ) i.e. to reference the name you could use $row[0] or $row['name']. That is the default behavior for this function. But, it takes an optional second parameter to determine whether the results are returned as BOTH numerical and associative or only one or the other. There is also the fetch_object which returns an object that has properties named for each field. $row = mysqli_fetch_object($result) To reference the name you would use $row->name
-
That almost always mean that the query failed and the result of the query is a Boolean (i.e FALSE). You need to check your query calls for errors since those errors occur in MySQL and not in PHP. This is the quick and dirty way to check it, but should not be used when used in a production environment $result = mysqli_query($link,$sql) or die("Query: $sql<br>Error: " . mysqli_error($link);
- 7 replies
-
- mysqli
- fatalerror
-
(and 1 more)
Tagged with:
-
Read the two files into separate arrays. I assume each product has something such as a unique ID along with additional data about the product (name, description, etc). Use the unique identifier as the index for each array element and then make each element a subarray with all the other data E.g. $newCSV = array( '1' => array('name' => 'Prod 1', 'Desc' => 'Description for product 1'), '4' => array('name' => 'Prod 4', 'Desc' => 'Description for product 4'), '7' => array('name' => 'Prod 7', 'Desc' => 'Description for product 7') ) Once you have the two arrays $newCSV and $currentCSV (or whatever you want to name them) you can use the function array_diff_key() to determine the new records and the deleted keys - just depends on the order of the parameters $deletions = array_diff_key($currentCSV, $newCSV); $additions = array_diff_key($newCSV, $currentCSV); Look ma - no loops!
-
From the manual When you have these types of problems, take a breath and determine what data you can inspect to determine what the problem may be. As Ch0cu3r proposed, checking the results of the query is a good first step. Once you verify that you could have inspected the HTML code and you would have seen multiple lines of the table rows with no content. The next logic steps would have been to inspect what the value of $img2 is using either print_r() or var_dump(). Either one would have made the problem perfectly evident.
-
Something to consider: If you were to use PDO instead of mysqli_ you can create a prepared statement and pass the entire array to the statement. You do need to specify the named indexes in the prepared statement, which you would have to write out in the code or do it programatically as Ch0cu3r did above. But, you can then create multiple records by passing the arrays. Example //Arrays with values to insert $record1 = array('name' => 'John Smith', 'addr' => '123 Main Street', 'city' => 'New York'); $record2 = array('name' => 'Jane Doe', 'addr' => '425 Elm Street', 'city' => 'Los Angeles'); $record3 = array('name' => 'Alex Williams', 'addr' => '623 Delta Ave', 'city' => 'Austin'); //Create database handle $dbh = new PDO("mysql:host=$host;dbname=$dbname", $user, $pass); //Create prepared statement handle $sth = $dbh->prepare("INSERT INTO my_table (name, addr, city) value (:name, :addr, :city)"); //Insert records using the arrays $sth->execute($record1); $sth->execute($record2); $sth->execute($record3);