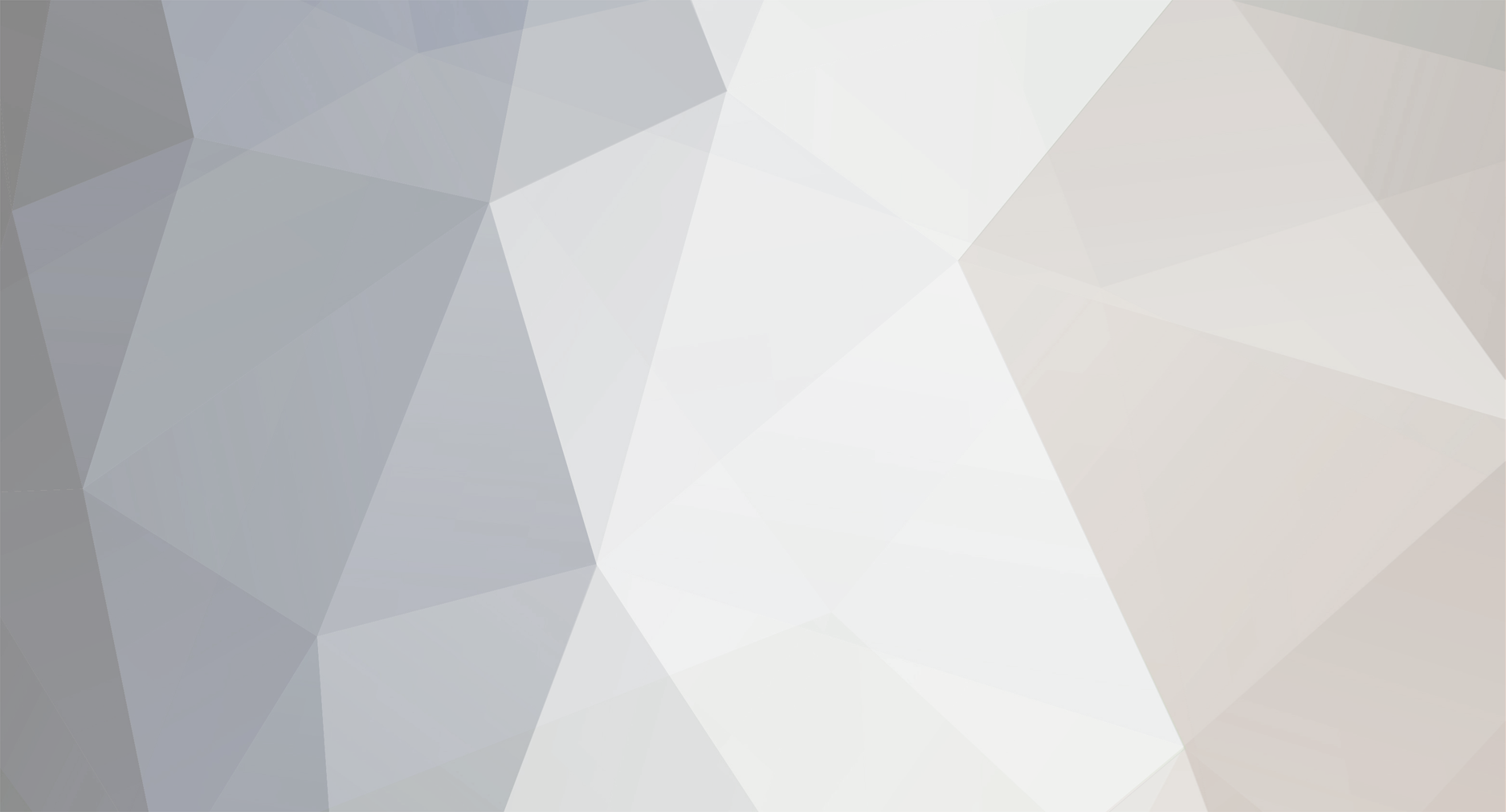
Psycho
Moderators-
Posts
12,160 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
php script restarts randomly with same POST data
Psycho replied to holdorfold's topic in PHP Coding Help
There may be two different types of timeouts at play here. Typical "session" timeouts are set for 20 minutes. So, if you have stores information in the session it will expire if the user is inactive for 20 minutes. That is completely different from the execution timeout. That is how long a script can run before the webserver gives up. This is to prevent situations where the script may have an infinite loop. Otherwise, one bad script would bring down the server. I'm no web server guru, but I though that 300 seconds was the maximum amount that the max execution time could be set to (i.e. 10 minutes), but the default setting is much lower (maybe 30 seconds). At least that's true in Apache servers (I think). So, let's go back to what you are trying to achieve. You want to put a gap in between each email. I'm assuming to stop from it being triggered as spam? You could use a service to send the emails which would not have that limitation. Plus, why the random 2-20 second delay? Why not just set it to 1 or 2 seconds for all of them? In any event, I have a better idea to achieve what you are trying to do (although I think there is a better solution altogether). Instead of trying to send the emails when you are processing the data - separate that logic. Have the script run ONE TIME to completely process ALL the data and store it. A database would be best, but you could use a log file. That should reduce a lot of the complexity - especially around the kill switch and other triggers you had to use. Now, once you have all the processed data in a single format you can call a script with a delay that will pull N records from the repository, send the emails, and then remove those records from the repository. Then you don't have to have any logic to remember what was or was not processed! In fact you could build one script to do both the processing and the emailing. Here is a VERY basic mock example of how the framework might look <?php $dataFile = 'path/name.txt'; if(isset($_POST['value_to_determine_there_is_a_new_input'])) { //Insert logic here to process the entire input file //and create a data file with the processed results //Create file $dataFile with the processed data } else { if(file_exists($dataFile)) { //Remove a record (or records) from the data file //send the email(s) //If no more records exist in data file - delete it } else { //There are no records to process exit(); } } sleep(2); ?> -
SalientAnimal, I think you need to slow down just a tad. Get your scripts working without all the JavaScript. If you are going to go to the trouble of making things react immediately, then you should rally use AJAX rather than just dynamically submitting the page. But, don't do that until you have everything working without JavaScript. So, create your page with a simple Submit button for testing. Plus, you should use POST data as opposed to GET since you are sending so much data. This is really a simple process. On every page load you check for the selected value for any of the fields on the form. Then, query the database for the possible values and use any POSTed values to determine which options are selected when building the form fields. So, just like I provided a function to create the select list options which takes parameters for the possible values and the optional selected value, you can create similar functions for other types of form fields - such as a radio button group, checkboxes, whatever.
-
Why isn't this Regex capturing everything between the <body> tags?
Psycho replied to MySQL_Narb's topic in Regex Help
1. The content you are matching against doesn't have a closing body tag 2. You need the 's' parameter to tell the expression to traverse multiple lines - otherwise it looks for a match on single lines only The page you are using doesn't allow you to add that flag. Try a different one (e.g. http://regex101.com/) or test it yourself. -
php script restarts randomly with same POST data
Psycho replied to holdorfold's topic in PHP Coding Help
You are making this more complicated than it needs to be. If you need to process it in chunks as you say, then you were already there. The problem was you were implementing the sleep() on each record in the chunk. Seems you should put it at the end of the script before you process the next chunk! But, I'm really not understanding the point of this script. It doesn't seem to do anything except make log files. If you are having timeout issues I think it is because of the manner that you are creating those log files. Every time you want to add a line to the log file you are opening the file, reading the entire contents into memory, appending a new line, and the writing the entire contents back tot he file. You can simply open the file in "append" mode and just send the new line to be appended to the content. Plus, you should only open the file ONCE per script execution instead of opening it each time you want to add a line to the log file. Based on the type of processing I see in this script you should be able to process many thousands of records without having to break it into chunks. If you can provide a complete input file I might be able to help. -
php script restarts randomly with same POST data
Psycho replied to holdorfold's topic in PHP Coding Help
I hear you. But, I can tell you from experience that what may seem obvious may not always be. I've chased many problems down "knowing" that the problem had nothing to do with "X" and wasting a lot of time researching other possibilities. Only to find out the issue was staring right at me. Is there some reason you are processing the data in chunks like this? Also, the file you uploaded is just a CSV with firstnames, lastnames and email addresses. Based on your code I should see things such as "<!asplit!>" in there. EDIT: OK, I think this is another one of those times. I didn't even consider how long the "sleep" time might be IN TOTAL. You are making it a random number from 2 to 20. I think you are executing 10 records on each page execution. SO, the time would be anywhere from 20 to 200 seconds (with 1 or 2 for actual execution). The 200 seconds shouldn't be a problem, Now, the 200 seconds should never really occur - the changes are pretty rare. So, even in the middle of 100 seconds you are looking at the page taking a minute and a half to complete. I'm guessing the script is timing out. You should really test this without JavaScript if possible (just put the URL into the browser passign the appropriate parameter) then you can see any errors - if they do occur. That all makes sense now as to why it does it and why it does it randomly. -
Change the file name from somefile.htm to somefile.php and it's a PHP file. Now, it will still just output whatever you had before and you would still need to implement some code to initiate the check for login though. Besides those two files won't do anything just by putting them together. FYI: many of us regulars get a little peeved by people posting homework on here and trying to get people to do it for them. I find it unlikely your DB is named "Assignment" for any other reason. No. If you want someone to do this for you, look at posting in the freelance forum. Although I doubt anyone would be willing to do it for free.
-
Yes Typically you will want all of your pages to be PHP. Any page that is requested from the user would need to perform a check to see if the user is logged in. If yes, display the requested content. If no, redirect to the login page. If you have any pure HTML pages and the user can type in the URL to that page you can't restrict them via any login functionality. Well, technically you can, but it gets complicated and not worth discussing at this time.
-
OK, so what's your question? Where is the code you have attempted and what is/is not happening and/or what errors are you getting?
- 1 reply
-
- php
- pagination
-
(and 1 more)
Tagged with:
-
php script restarts randomly with same POST data
Psycho replied to holdorfold's topic in PHP Coding Help
Can you attach the file that is being processed? -
php script restarts randomly with same POST data
Psycho replied to holdorfold's topic in PHP Coding Help
Ok, so you see that packet #9 had a problem. Did you inspect the full contents of that packet? Could be some odd character in the input that is causing the problem. Also, your initial post made it seem as if it was reprocessing the same chunk of code. That's not the case, so I will assume there is something in the data that is causing that chunk to fail. -
I would also suggest using the named indexes of the value from the database instead of 0, 1, 2, etc. You will save yourself a ton of problems when you have to modify/debug the code.
-
php script restarts randomly with same POST data
Psycho replied to holdorfold's topic in PHP Coding Help
OK, looking through the PHP code, it is very difficult to follow because you use cryptic variable name (e.g. $q) and you reuse them. So, kind of difficult to know what the variable contain or what their purpose is. Plus there are plenty of inefficiencies. For example, you open the log file each time you want to write to it. You should only open it once during the script execution and close it at the end. Plus, many times you are echo'ing the same thing you are sending to the log function. Just add a parameter to the log function to determine if you want the log entry to be output to the page. Or, have the log function return the log entry and then just echo the result of the log function where you want to do so. Plus, you are reading the entire contents of the log file and then writing the entire thing back with the new line. You can simply append a new line to the content. Another problem I see is the 'killswitch' logic. You are storing a value in a flat file. So, if the PHP script happens to be executed concurrently multiple times, there will be problems since the multiple concurrences will be reading from the same file. Not sure why you can't store the killswitch in memory as a variable or as a session value. -
php script restarts randomly with same POST data
Psycho replied to holdorfold's topic in PHP Coding Help
And how are you making that determination? If that was the case I would think it would get caught in an infinite loop. Or, perhaps you are seeing some of the same data processed multiple times in the output. If that is the case, how do you know the input data doesn't have some duplication? -
Not sure what you are really saying. Your code shows you have defined a variable $places as an array. What does the $global variable have to do with it? Create a list echo "<ul>\n"; foreach($places as $place) { echo "<li>{$place}</li>\n"; } echo "<ul>\n";
-
The second two are typos
-
Not that I know of. Unlike a computer virus, PHP code is meant to be executed. It would only do what it is programmed to do, so how would a scanner know what is and is not supposed to do? If you are buying code, make sure it is from a reputable source. Also, I would not be so concerned with code having an obvious malicious intent as opposed to code that has been built with a back door to allow someone to compromise your server. And, if there were a scanner to find such holes there wouldn't be instances of legitimate code having such flaws - e.g. heartbleed.
-
As for only getting the first five blogs with all of their comments, you would likely need to use a subquery with the limit. [Note the second to last line int he query below] SELECT blog.blog_id, blog.dateposted, blog.content, blog.title, blog.published, user.login, user.img, bc.username, bc.comment FROM blog JOIN user ON blog.user_id = user.user_id LEFT JOIN blog_comments bc ON blog.blog_id = bc.blog_id WHERE blog.published = 1 AND blog.blog_id IN (SELECT blog_id FROM blog ORDER BY dateposted LIMIT 5) as L ORDER BY blog.blog_id DESC
-
When you are querying data with JOINs where there could be multiple records JOINed on a single record you get results such as this: blog_id | blog_text | comment_id | comment_text ------------------------------------------------- 2 Blog2Text 4 comment4text 2 Blog2Text 7 comment7text 2 Blog2Text 8 comment8text 5 Blog5Text 9 comment9text 5 Blog5Text 11 comment11text There are two unique blogs. The first has 3 comments and the second has two comments. This is normal. Now if you wanted to display each blog one time along with the associated comments you do this in the code. Example <?php $blogID = false; //Usa a flag to check when the parent record changes while($row = mysql_fetch_assoc($result)) { //Check if this is a new blog //This is skipped for a record if the blog is same as the last iteration if($blogID != $row['blog_id']) { //This is a new blog, let's display it $blogID = $row['blog_id']; echo "<br><b>" . $row['blog_text'] . "</b><br>"; } //Display the comment echo "Comment: " $row['blog_text'] . "<br>"; } ?> The output of the above should look something like this: Blog2Text Comment: comment4text Comment: comment7text Comment: comment8text Blog5Text Comment: comment9text Comment: comment11text
-
Your GROUP BY is "grouping", i.e. collapsing, the records. That needs to be taken out. Also, the JOIN on the comments should be a LEFT JOIN. This assumes you want all of the blog posts - even if there are no comments. Lastly, you have a LIMIT with will only allow 5 records to be returned. Because of the JOINs you may get five records all for the same blog post with different comments. Or you could get five blog posts with one comment each. Unless you can explain what you were trying to achieve with that limit, it's difficult to know how it needs to change. You reference a field called username in the SELECT clause that you don't mention as being in the comments table and it doesn't make sense being in that table to me. I removed the aliases from the first two tables. The point of using aliases is to make it easier to read the query. When you use something like 'a' which has no correlation to the table name it has the opposite effect. I wouldn't use an alias on a table that is only four characters long anyway. SELECT blog.blog_id, blog.dateposted, blog.content, blog.title, blog.published, user.login, user.img, bc.username, bc.comment FROM blog JOIN user ON blog.user_id = user.user_id LEFT JOIN blog_comments bc ON blog.blog_id = bc.blog_id WHERE blog.published = 1 ORDER BY blog.blog_id DESC
-
I prefer to let the counter just increment by one on each iteration and not reset it. Instead, you can just use the modulus operator. Example: $columns = 5; $count = 0; while($row = mysql_fetch_assoc($result)) { $count++; //Open new row if needed if($count%$columns == 1) { echo "<tr>\n"; } //Display the record echo "<td>"; //Record output goes here echo "</td>"; //Close the row if needed if($count%$columns == 0) { echo "</tr>\n"; } } //After loop, close row if it didn't end with full last row if($count%$columns != 0) { echo "</tr>\n"; }
-
Um, no. That is a NOT how you should be storing the records. Based upon this and your other post I see that you really, really need to do some research on database normalization. The things you are trying to do are only going to make your life harder. Yes. But, it should be done differently than you think it should. No idea. Your explanation above makes no sense. You select a time period and retrieve a list of users based on what? You then select checkmarks next to the users' names that does what? You then want to store records associated for the user based on whether they are present, absent or late. How is this determined, the checkbox? A checkbox only has two possible states: checked or not checked. So, how do you determine the three statuses? Ok, from this it appears you are now wanting three checkboxes per user even though you clearly stated previously you would only have one. Why checkboxes? They are not mutually exclusive and makes your life harder. I.e. a user could check the Present AND the Absent checkboxes for the same user. So you would have to add additional validation logic. Just provide a single select list with the three options for each user. And the fields should be something like this: <select name="present[34]"> <option value="0">Absent</option> <option value="1">Present</option> <option value="2">Late</option> </select> The 34 in the name would represent the user ID of the user that the field is associated with. You POST data would then contain an array with the values for all the user.
-
Ok, but if you fix your database structure as I've suggested, which you should really do, the queries become simpler. In fact, you can create ONE SINGLE QUERY to use for all of the lists. Just create a function to pass an optional parameter To query the Primary Categories would look like SELECT category_id, category_name FROM categories WHERE parent_id = 0 To query the secondary categories based upon a specific primary category SELECT category_id, category_name FROM categories WHERE parent_id = $primary_category_id To query the tertiary categories based upon a specific secondary category SELECT category_id, category_name FROM categories WHERE parent_id = $secondary_category_id Note the only difference in the parent_id value. So, you can create a function to use for all of them. Here is the code I posted revised on how it could be done if the DB was properly built. As you can see it is so, so much simpler. There are just two functions and then only two lines of code to build each select list! <?php mysql_connect('localhost', 'root', ''); mysql_select_db('tab_test'); //Functions to create HTML for options list function createOptions($optionList, $selectedValue) { $options = ''; foreach ($optionList as $id => $label) { $selected = ($id==$selectedValue) ? ' selected="selected"' : ''; $options .= "<option value='{$id}'{$selected}>{$label}</option>\n" } return $options; } //Function to get a list of categories function getCategories($parent_id=0) { $query = "SELECT category_id, category_name FROM categories WHERE parent_id = {$parent_id} ORDER BY category_name"; $results = $dbo->query($query); $categories = array(); foreach($results as $cat) { $categories[$cat['category_id']] = $cat['category_name']; } return $categories; } //Determine selected options passed on query string $selected_primary = isset($_GET['primary_category']) ? intval($_GET['primary_category']) : false; $selected_secondary = isset($_GET['secondary_category']) ? intval($_GET['secondary_category']) : false; $selected_tertiary = isset($_GET['tertiary_category']) ? intval($_GET['tertiary_category']) : false; //Generate options for primary category $primary_categories = getCategories(); //Get the primary categories $primary_category_options = createOptions($primary_categories, $selected_primary); //Generate options for secondary category $secondary_categories = getCategories($selected_primary); //Get secondary categories based on selected primary $secondary_category_options = createOptions($secondary_categories, $selected_secondary); //Generate options for tertiary category $tertiary_categories = getCategories($selected_secondary); //Get secondary categories based on selected secondary $tertiary_category_options = createOptions($tertiary_categories, $selected_tertiary); ?> <!doctype html public "-//w3c//dtd html 3.2//en"> <html> <head> <title>Demo of Three Multiple drop down list box from plus2net</title> <meta name="GENERATOR" content="Arachnophilia 4.0"> <meta name="FORMATTER" content="Arachnophilia 4.0"> <script language="JavaScript"> function getSelectValue(selectID) { var optionObj = document.getElementById(selectID); return optionObj.options[optionObj.selectedIndex].value; } function reload(form) { //Adding the unselected options should work fine var locationURL = 'dd3.php?'; locationURL += 'primary_category=' + getSelectValue('primary_category'); locationURL += 'secondary_category=' + getSelectValue('secondary_category'); locationURL += 'tertiary_category=' + getSelectValue('tertiary_category'); //Perform the reload self.location = locationURL; } </script> </head> <body> <form method=post name=f1 action='dd3ck.php'> <select name='primary_category' id='primary_category' onchange="reload(this.form)"> <option value=''>Select one</option> <?php echo $primary_category_options; ?> </select> <select name='secondary_category' id='secondary_category' onchange=\"reload(this.form)\"> <option value=''>Select one</option> <?php echo $secondary_category_options; ?> </select> <select name='tertiary_category' id='tertiary_category' onchange=\"reload(this.form)\"> <option value=''>Select one</option> <?php echo $tertiary_category_options; ?> </select> <input type=submit value='Submit the form data'> </form> <br><br> <a href=dd3.php>Reset and Try again</a> <br><br> </body> </html>
-
What are the actual values you are working with? Are you using values such as '428.13 ft' or is is just '428.13'? I will assume the former. function calcLevel($current, $normal) { //Get float values from strings $current = floatval($current); $normal = floatval($normal); //Calculate the difference $calcLevel = $current - $normal; //Format difference to two decimal places and add 'Ft' $calcLevelStr = (string) number_format($calcLevel, 2) . ' Ft'; //If vlaue is positive add a + to beginning if($calcLevel>0) { $calcLevelStr = '+'.$calcLevelStr; } //Return the calculated formatted value return $calcLevelStr; } echo calcLevel('445.5 Ft', '428.13 Ft'); //Output +17.37 F echo calcLevel('405.5 Ft', '428.13 Ft'); //Output -22.63 Ft
-
Huh? The query ONLY returns records where the values are equal. There is no need to do any comparison int he code. In other words, you need to send an email to every record in the result set of that query.
-
If both tables will not always have matching records, which table has all the records? That is the one you will want to run the query from initially (I am assuming deals). Also, need to know which table the email address is in (again assuming deals) This will return a result set of the email addresses and remaining visits where the admin value and the visits count is the same SELECT deals.email, deals.visits FROM deals LEFT JOIN customers ON deals.admin_id = customers.admin_id WHERE deals.visits = customers.visits