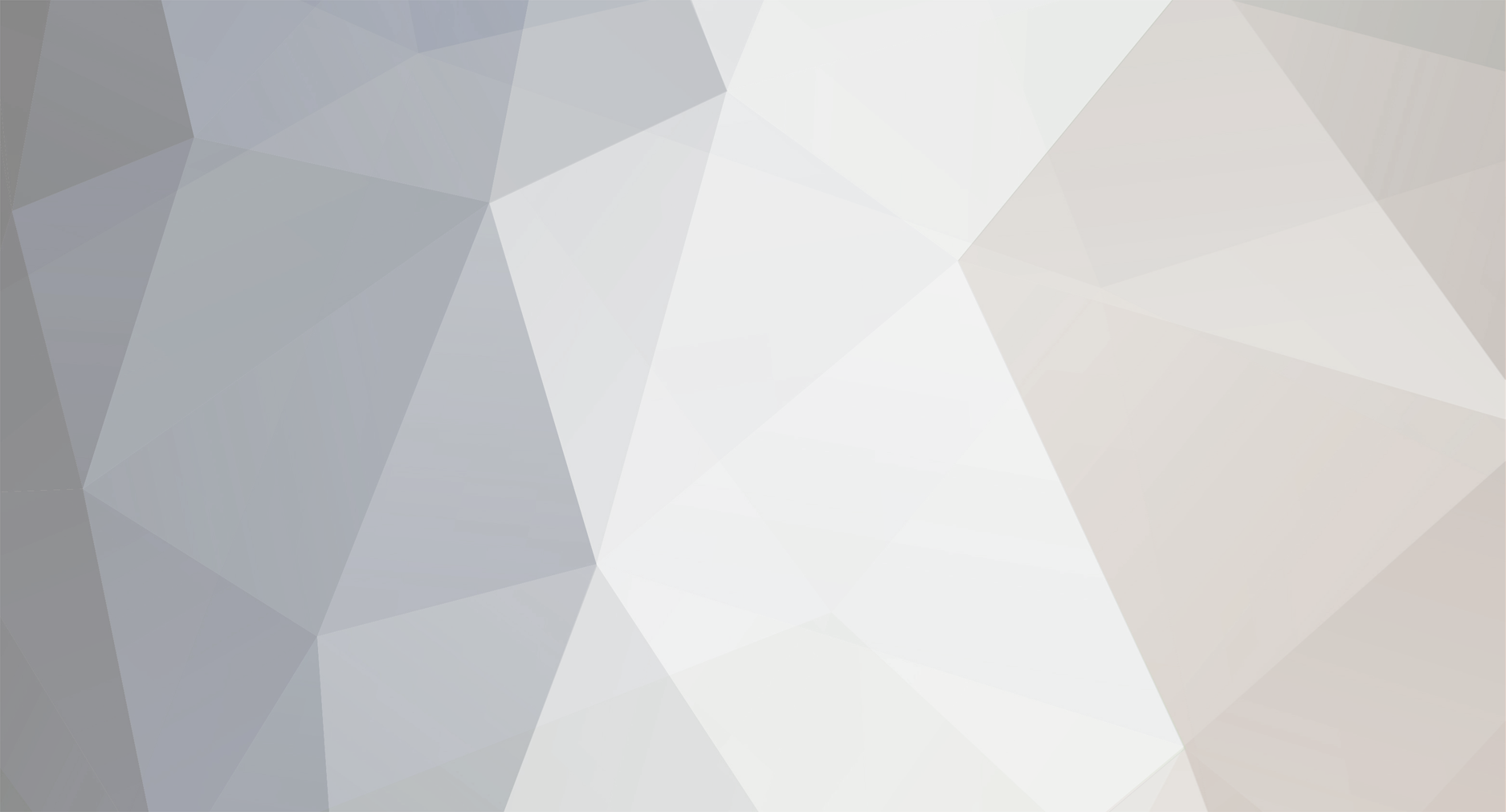
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I don't think it is feasible to do this in the query. Just create the "months" in the output using PHP. Just be sure to "normalize" the start date to the first of the month. Because if the start date is on Jan 31 and you increase it using "+1 month" you will get something like March 3rd.
-
how to retrieve data from many table in database
Psycho replied to Spraban9's topic in PHP Coding Help
You need to JOIN the tables in your queries. Trying to teach you how to use JOIN in a forum post is counter-productive. Go find some tutorials and start learning. -
Sessions are not stored on a user's PC. The only thing that is stored on the user's machine is a cookie with the session ID. All the session data is stored on the server. The sessions are only valid for a limited time (I think 20 minutes by default). When you close your browser the cookie is deleted. So, the security risk would be someone copying that cookie while you have the browser open and while the session is active. But, I would guess the session ID may also be based on the IP address, so that may not work either.
-
Advice on php code and what process i should take
Psycho replied to benjahnee's topic in PHP Coding Help
Your question is too general to really provide a response. There are many ways to accomplish the same thing. Plus, we do not know what your knowledge base to even give some guidance. You should talk to your instructor. But, whenever you approach something like this you can start by breaking down all the requirements into small pieces of functionality, do an analysis of how they can all work together, and then build each piece of functionality one at a time. Generally, I would approach it in this fashion: Based upon the requirements, determine what data would need to be stored and set up the database. Then create the CRUD processes (create, update, delete). Then build any "display" features for the data. And, lastly, build any other features. -
I could, but I won't. I love helping people, but at some point you need to teach a man to fish rather than giving him a fish. You should be able to see where I implemented logic to handle co.uk. There's only one line to worry about which uses two functions and the ternary operator. You should be able to break down what that line is doing and figure out how to change it for multiple scenarios. Of course, you will need to break it out to multiple lines in a normal if/else condition rather than using the ternary operator. So, give it a try and post back if you run into problems showing the code you have.
-
What you need is a FULL OUTER JOIN which, unfortunately MySQL does not support. So, you have to be creative SELECT teamID, SUM(homePoints + awayPoints) AS totalPoints, SUM(homePoints) as homePoints, SUM(awayPoints) AS awayPoints, SUM(pointsAgainst) AS pointsAgainst FROM (SELECT homeTeamID as teamID, SUM(homePoints) as homePoints, 0 AS awayPoints, SUM(awayPoints) as pointsAgainst FROM results GROUP BY teamID UNION ALL SELECT awayTeamID as teamID, 0 as homePoints, SUM(awayPoints) AS awayPoints, SUM(homePoints) as pointsAgainst FROM results GROUP BY teamID ) as t GROUP BY teamID ORDER BY totalPoints DESC, pointsAgainst ASC The key is the UNION of the two inner queries in the FROM clause. The first gets all the appropriate values where a team was the home team (note that awayPoints is hard coded for 0). The second get all the appropriate values where a team was the away team (note that homePoints is hard coded for 0). Then after those two result sets are combined with UNION ALL, the outer queries combines the home values and away values for each team. NOTE: I originally used just UNION, but couldn't figure out why the results for all the teams was correct except for team ID 96. It was only coming up with 10 for the points against and not 20. The reason is that UNION will drop any exact duplicates between the two queries. And the results for team ID 96 in both those sub queries was 96, 0, 0, 10. So, it dropped one. The UNION ALL was the solution.
-
SELECT t1.art_id, t1.artist_id, t1.file, t1.competition_id, t2.score FROM table1 AS t1 LEFT JOIN table2 AS t2 ON t1.art_id = t2.art_id AND t1.artist_id = t2.artist_id You want ALL the records from table 1, so you should not use a WHERE clause to filter out any records. However, if you want to restrict which records from table 2 are joined on table 1 on those fields you can do that in the JOIN condition. Since some records from t1 do not have corresponding records from t2, the first condition in the where clause will exclude all of those records. SELECT t1.art_id, t1.artist_id, t1.file, t1.competition_id, t2.score FROM table1 AS t1 LEFT JOIN table2 AS t2 ON t1.art_id = t2.art_id AND t1.artist_id = t2.artist_id AND t2.juror_id = 5 AND t1.competition_id = 1
-
The logic in that script doesn't make sense to me. Why do you bury the login check at the very end after all the code has been run to display the content that shouldn't be displayed to a non-logged in user? In any event, there is nothing in that script that would tell us why the user is being incorrectly identified as not logged in. I *assume* your login script is setting a value for $_SESSION['loggedIn']. So, a couple things come to mind. I don't see a session_start() on the above script - which is mandatory to reference session variables. But, you may have that in one of the included files. The other thing is, what is the value being set for $_SESSION['loggedIn']? If you are setting a value that is evaluated as false, your condition check will fail.
-
MySQL select where record does not exist in other table
Psycho replied to john-formby's topic in PHP Coding Help
You have to execute the query and then extract the results - just like you would when running any other query. <?php mysql_connect('MySQL_Server_URL', 'username', 'password'); mysql_select_db('database_name'); $dayQueries = array(); for($i=0;$i<5;$i++) { $bookDate = date('Y-m-j', strtotime("Monday +{$i} days")); $dayQueries[] = "SELECT roomID, roomNum, '{$bookDate}' FROM rooms WHERE rooms.roomID NOT IN (SELECT roomID FROM roomsbooked WHERE roomsbooked.event_date = '{$bookDate}')" } $SingleQueryToRun = implode("\nUNION\n", $dayQueries); $result = mysql_query($query) or die(mysql_error()); echo "<table border='1'>\n"; while($row = mysql_fetch_assoc($result)) { echo "<tr><td>" . implode("</td><td>", $row) . "</td></tr>\n"; } echo "</table>\n"; ?> -
I would never assume that someone's post count is indicative of their ability or competence in some skill-set. Only that their post count should be indicative of how much experience they have posting in that particular forum.
-
MySQL select where record does not exist in other table
Psycho replied to john-formby's topic in PHP Coding Help
Here's a solution using UNION (tested) $dayQueries = array(); for($i=0;$i<5;$i++) { $bookDate = date('Y-m-j', strtotime('Monday +'.$i. ' days')).'<br />'; $dayQueries[] = "SELECT roomID, roomNum, '{$bookDate}' FROM rooms WHERE rooms.roomID NOT IN (SELECT roomID FROM roomsbooked WHERE roomsbooked.event_date = '{$bookDate}')" } $SingleQueryToRun = implode("\nUNION\n", $dayQueries); -
MySQL select where record does not exist in other table
Psycho replied to john-formby's topic in PHP Coding Help
No, you do not need to run the query five time. As jcbones stated you should NEVER run queries in loops. 95% of the time you can run ONE query to get what you need. In the other 5% of the times you probably have a bad database design. Based on your code above you want all the vacancies that occur on each of the days in the date range. This is a little more difficult, but still does not require a loop. You could use PHP to dynamically create the multiple queries ad use a UNION or you could take a different approach entirely and instead just query the list of all room IDs and the booked rooms by date and put the logic of determining what is vacant in the PHP logic. -
Make the WHERE clause dependent upon any being selected. If none are selected do not use it. You should first process the list first to remove any invalid values. //Get values passed in POST data $hoodAry = isset($_POST['hoodselect']) ? $_POST['hoodselect'] : array(); //Trim the values $hoodAry = array_map('trim', $_POST['hoodselect']); //Remove empty values $hoodAry = array_filter($hoodAry); //Create WHERE caluse for query $WHERE = ''; //Set default as empty string if(count($hoodAry)) { //Prepare for DB query $hoodAry = array_map('mysql_real_escape_string', $hoodAry); //Implode into comma separated list (with quotes) $hoodList = "'" . implode("', '", $hoodAry) . "'"; $WHERE = "WHERE location IN ($hoodList)"; } $query = "SELECT * FROM available LEFT JOIN land ON available.building = land.building {$WHERE}";
-
MySQL select where record does not exist in other table
Psycho replied to john-formby's topic in PHP Coding Help
Well, if what you have above is representative of your data, then that is your problem. In the "rooms" table you have a roomID field with values of 1, 2, and 3. In the "roomsbooked" table you also have a roomID field which SHOULD be the same roomID value from the "rooms" table. But, instead you have a room NUMBER: "12". If you start storing the correct value for the roomID in the "roomsbooked" table you will get the correct results using either of the two queries I provided. Using your sample data, but changing the "12" to a "1" in the rooms booked table I ran this query: SELECT roomID, roomNum FROM rooms WHERE rooms.roomID NOT IN ( SELECT roomID FROM roomsbooked WHERE roomsbooked.event_date = '2013-03-25' ) And got these results: roomID | roomNum 2 14 3 18 -
. . . and don't put extraneous spaces between the percent signs and the value - otherwise the data will only match on records where the search value exists with spaces on either side. Plus, I always trim such data from the user as well. $searchValue = trim($_GET['Value']); stmt->execute(array(":Value" => "%{$searchValue}%" ));
-
Based upon a couple quick searches I did, putting the parameter between percent symbols in the prepared statement does not work. The solutions I found were to either append the percent symbols to the value being passed as the parameter OR to concat the percents in the query. http://stackoverflow.com/questions/1786436/php-pdo-prepared-statement-mysql-like-query http://board.phpbuilder.com/showthread.php?10368514-RESOLVED-mysqli-gt-prepare%28-WHERE-LIKE-%29 Since you are using the term multiple places, I would add the symbols to the value before passing it in as a parameter $query = " SELECT Id,Yr,ProjectName,Department,Owner,MaplibraryName, Description,MaplibraryPath,PageSize,Scale,LastModified, Notes,ModifiedBy,FullPath,Path_jpg,PDF_Path,JPG_Path FROM tbl_maplibrary WHERE Department LIKE :dept OR Field1 LIKE :dept OR Field2 LIKE :dept ORDER BY Yr DESC"; $prep = $dbh->prepare($query); $ret = $prep->execute(array(':dept' => "%{$department}%"));
-
And this is in the PHP forum why . . . ? You have over 7,000 posts. If we cannot trust people who have been around a long time to follow the rules it's kind of hard to expect new users to follow them.
-
MySQL select where record does not exist in other table
Psycho replied to john-formby's topic in PHP Coding Help
Why would you want to apply that filter later when you can simply apply it in the SELECT query? That only creates more overhead. Besides, the OP clearly stated his intent -
MySQL select where record does not exist in other table
Psycho replied to john-formby's topic in PHP Coding Help
The OP needed all unbooked rooms - for a specific date. Both of my solutions would do that. And why would you want to return the room ID from the booked table? It would be NULL and of no value. -
MySQL select where record does not exist in other table
Psycho replied to john-formby's topic in PHP Coding Help
Since you don't need any data from the roomsbooked table, this might be more efficient since a JOIN is not needed. SELECT rooms.roomID, rooms.roomDescription FROM rooms WHERE rooms.roomID NOT IN ( SELECT roomID FROM roomsbooked WHERE roomsbooked.event_date = '$currentDate' ) -
MySQL select where record does not exist in other table
Psycho replied to john-formby's topic in PHP Coding Help
Close, but pretty sure you have to use "IS NULL" or "IS NOT NULL" and not "= NULL". Also, you will need to use the date to limit the records being joined based on the date being checked. This should work SELECT rooms.roomID, rooms.roomDescription FROM rooms LEFT OUTER JOIN roomsbooked ON rooms.roomID = roomsbooked.roomID AND roomsbooked.event_date = '$currentDate' WHERE roomsbooked..roomID IS NULL Put the fields you need returned in the SELECT clause - don't use '*'. -
Um, yeah, that's to be expected. A hash has a VERY large number of possible combinations. So a measly 1000 codes would almost certainly not have a duplicate. I ran a test generating the 8 character substring of the MD5() hash for each number from 1 to 50,000 and there were no duplicates. But, again, that is to be expected. But, to make a statement that you want an 8 character "hash" that could NEVER have a duplicate is ridiculous. Assuming you don't think you will need 50,000 codes, just have an incremental value in the DB and take the first 8 characters of the hash on that value. I would have tested it out further, but the script timed out and I'm not willing to invest additional time.
-
Every HASH will repeat at some point! Even a 32 bit hash will repeat because the number of things that can be hashed is infinite and the number of hashes is finite. If you are not having 10's of thousands of users to manage with these codes a substr() of a normal hash should do just fine
-
Every HASH will repeat at some point! Even a 32 bit hash will repeat because the number of things that can be hashed is infinite and the number of hashes is finite. If you are not having 10's of thousands of users then using a substr of a hash should work just fine.