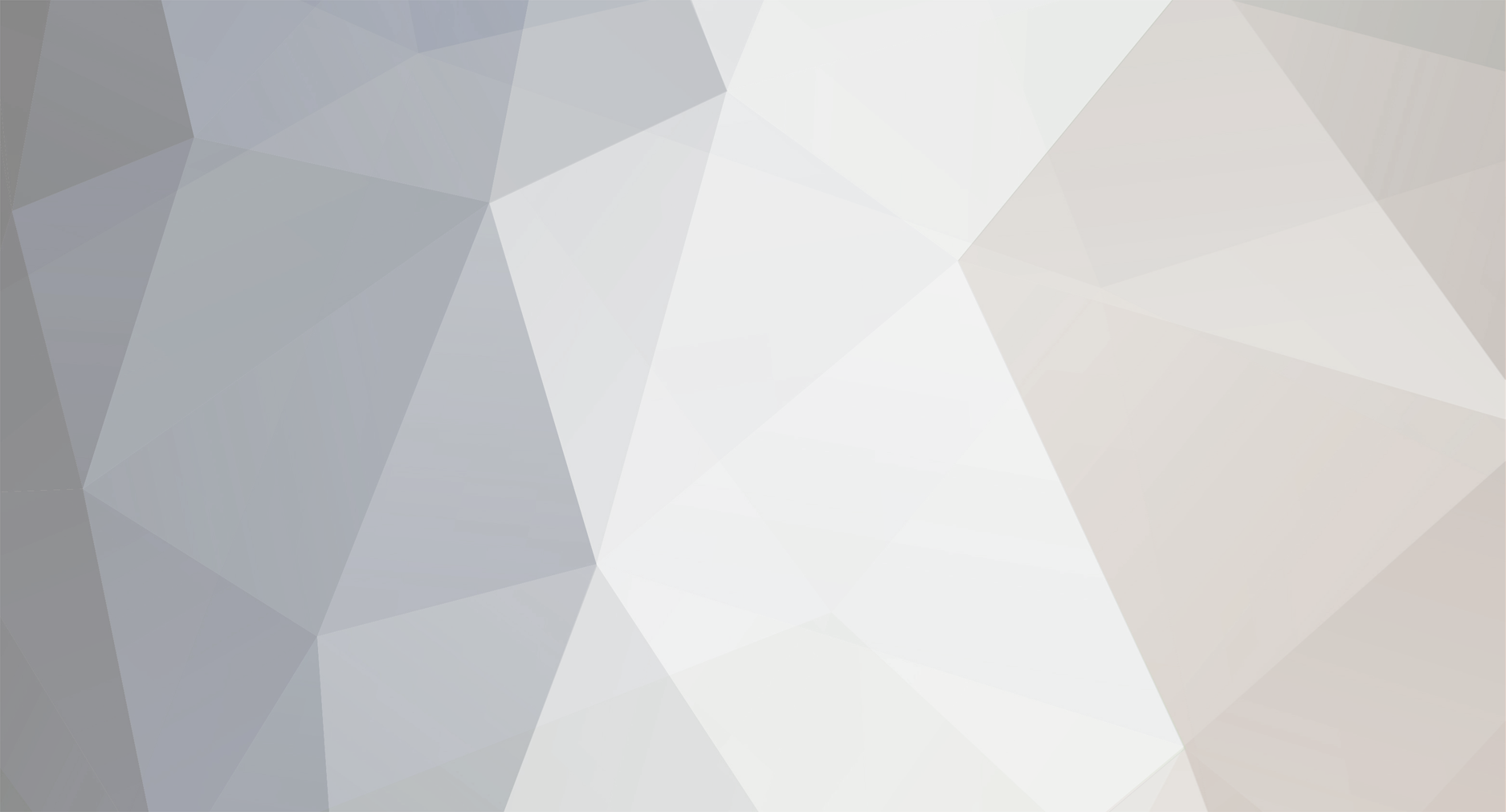
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
Your markup is invalid. You're mixing HTML and XHTML syntax. You need to either enclose attribute values in single or double quotes, or you need to remove the self closing part of the input tag.
-
Both. I sometimes work on a freelance/consultant basis.
-
Sure: <?php class Timer { private $start; public function __construct() { $this->start = (float) microtime(true); } public function getTime() { return microtime(true) - $this->start; } } $numbers = array(); for ($i = 0; $i < 10000; $i++) { $numbers[] = rand(1,1000); } $timer = new Timer(); // START ALGORITHM $group1 = $group2 = array(); // create two empty arrays to store the numbers in sort($numbers); // sort numbers ascendingly. this will put the highest numbers as the end. // we need that for maximum precision so we can do the more fine grained // grouping at the end while (count($numbers)) { // loop through the $numbers array as long as it contains elements $topVal = array_pop($numbers); // remove the last element from $numbers and store it in $topVal // doing this each iteration will mean we iterate over each // element exactly once because the while loop will end once // $numbers has been depleted $delta = array_sum($group2) - array_sum($group1); // we need the current difference of the lists' sums // to determine where to put the current number if ($delta <= 0) { // if the $delta <= 0, then $group1 has the largest sum and as such we need to put $group2[] = $topVal; // the new value in $group2 to even it out... } else { // ...otherwise we do the opposite and place it in $group1 $group1[] = $topVal; } } // we have now split the $numbers list into two lists $group1 and $group2. they are, however, not necessarily // of same size, but the following will take care of if (($g2s = sizeof($group2)) != ($g1s = sizeof($group1))) { // check if their same size and store their size in $g1s and $g2s if ($g2s > $g1s) { // if $group2 is largest then we make $from store a *reference* to $group2, and $to store a reference $from = &$group2; // to $group1. we are going to move elements from $group2 to $group1... $to = &$group1; } else { // ... and we'll do the opposite if $group1 is largest $from = &$group1; $to = &$group2; } while (sizeof($from) > sizeof($to)) { // this loop runs as long as $from is larger than $to $to[] = array_pop($from); // because we grouped the smallest elements in the first part of the algorithm, // this means that $group1 and $group2 will have the smallest elements in the end // as well. we need to move the smallest element because those will have the least // significant change on the diff sum of the lists, so we remove the smallest from // the largest list and move to the smallest list until the lists are evenly sized } } // END ALGORITHM echo $timer->getTime() . PHP_EOL; echo array_sum($group2) - array_sum($group1); //var_dump($group1, $group2); ?> I left over some debugging and benchmarking code in case you're interested.
-
Hmm... my algorithm can split 10000 random integers between 1 and 1000 in about 1.27 seconds on my computer. That's fairly decent I suppose. If we assume you're not going to have more than 100 elements then it has a running time at about 0.3 ms. That should be sufficiently fast.
-
Also, if you have a really large data set then it might be faster to use SplStack than an array. You cannot ensure minimal difference otherwise. If you let x={7,20,4,4,3,5}, then you need y1={7,5,4,4} and y2={20,3} for minimal difference. 7+5+4+4=20, 20+3=23, 23-20=3. There is no other way you could group that data set such that you both have minimal difference between their sums while still making the lists contain an equal amount of elements. Edit: Well, I guess you could. You could move one of the 4s from y1 to y2. That would get a difference of 11 instead of 3 though. Edit 2: If you do want that then you can do this: <?php $numbers = array(7,20,4,4,3,5); $group1 = $group2 = array(); sort($numbers); // i know THIS array is already sorted... while (count($numbers)) { $topVal = array_pop($numbers); $delta = array_sum($group2) - array_sum($group1); if ($delta <= 0) { $group2[] = $topVal; } else { $group1[] = $topVal; } } if (($g2s = sizeof($group2)) != ($g1s = sizeof($group1))) { if ($g2s > $g1s) { $from = &$group2; $to = &$group1; } else { $from = &$group1; $to = &$group2; } while (sizeof($from) > sizeof($to)) { $to[] = array_pop($from); } } ?> However, if you have an odd number of elements then you'll still be stuck though.
-
Hmm... try this: <?php $numbers = array(1,2,3,4,5,6,7,8,9,10,11,12); $group1 = $group2 = array(); sort($numbers); // i know THIS array is already sorted... while (count($numbers)) { $topVal = array_pop($numbers); $delta = array_sum($group2) - array_sum($group1); if ($delta <= 0) { $group2[] = $topVal; } else { $group1[] = $topVal; } } ?>
-
Then you need to read through the Language Reference chapter of the manual. It's required reading for anyone. No excuse.
-
$url .= substr($url, 0, 7) != 'http://' ? 'http://' : null;
-
Like this? $numbers = array(/* [...] */); $groups = array(); foreach ($numbers as $num) { $group = floor($num / 5); $groups[$group][] = $num; } (not tested) That should group the numbers in intervals of five.
-
dynamic community sites- message board vs bulletin board forum
Daniel0 replied to yamahammer's topic in Miscellaneous
I think it's important that the UI is logical and easy to use. I'm mostly concerned with the admin/mod side of the features though. Essentially the core user functionality is the same in all forums. As a user I just need an easy way to track replies to topics I've participated in. -
Well, you can catch regular errors using set_error_handler as well.
-
That's what abstraction is for. PDO will throw an exception that you can catch and pass to another place that handles errors in different ways depending on whether you're in a production or development environment.
-
Then send a 301 response code and tell them the new URI using the Location header.
-
One of the advantages it has if you are going to run the query repeatedly then you'll see a performance boost because it's already prepared. So it's excellent for things like batch processing. The parametrized queries do not have any advantage over escaping values and interpolating them into the query string in terms of security. Of course if you make a habit of using parametrized queries then you will not accidentally forget escaping tainted data, so I suppose that it in that sense is more secure. No.
-
You can use Zend Server or Zend Server CE.
-
You might also want to setup load balancing between multiple servers and perhaps run a more lightweight web server such as lighttpd instead of Apache for serving static assets like those.
-
Also set a far future Expires header, configure ETags, and send a Last-Modified header.
-
"You're f-ed* in T minus 10, 9, 8, 7 […]" * Trying to be PG-13 here...
-
I don't mean to break it for you, but your class is entirely redundant. It would be easier and more efficient to just call the mail function directly unless you are going to give your class more advanced functionality.
-
What is the Difference Between MYSQLi and MYSQL Extenstion
Daniel0 replied to binumathew's topic in Application Design
Yeah I'm really looking forward to the 5.3 release. Screw lame hosts that are slow to update. I've got my own server and I'll update immediately after the stable release. This timetable says that it's scheduled for release in Q2. I hope that's true. -
Well, I guess you can replace all your files with goatse, tubgirl, et al. That should get people to go away.
-
There is no such thing as unlimited anything, and anyone who claims to offer unlimited anything are overselling by the definition that overselling constitutes selling more than you have. Let x be DreamHost's hard disk capacity/bandwidth/requests, and let y be the amount they are selling. To satisfy the "not overselling condition" then [tex]x\geq y[/tex] [tex]\forall{x\in\mathbb{R}}, x<\infty[/tex] [tex]y = \infty \Rightarrow x < y[/tex] [tex]\therefore[/tex] DreamHost is overselling. QED. Anyone who thinks otherwise fails at math. I've said it before, and now I'll say it again. Do business with an honest host that makes it clear exactly how much you are and are not allowed to use.
-
What's the big deal? I thought you had "unlimited"...
-
Why did you choose to ignore my advise of using your distro's LAMP specific packages?
-
What is the Difference Between MYSQLi and MYSQL Extenstion
Daniel0 replied to binumathew's topic in Application Design
That's not entirely accurate. MySQLi supports some things that the old MySQL extension doesn't. Things like prepared statements, multiple statements, and transactions on top of my head.