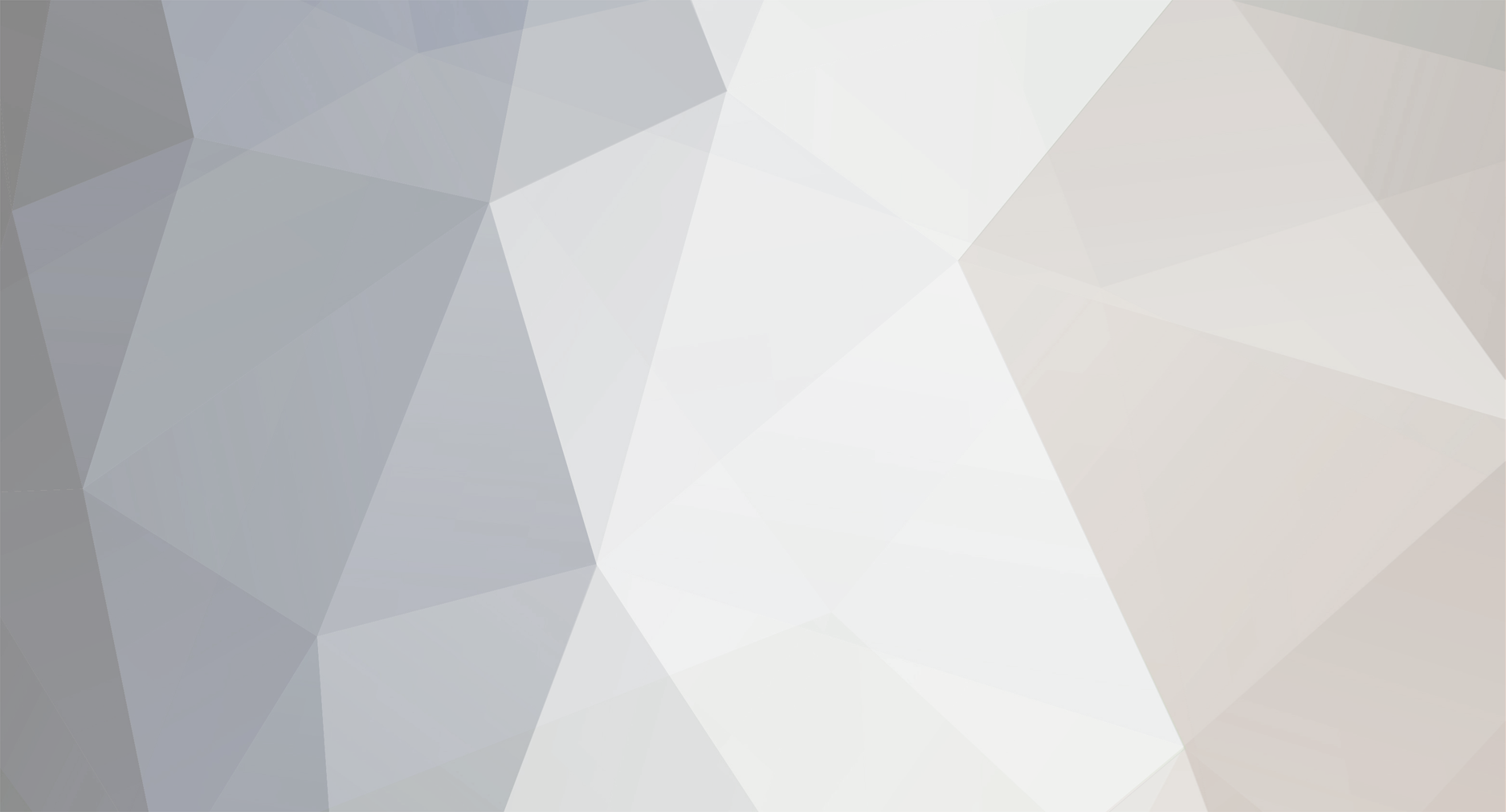
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
Yes it will, the single quote is part of the SQL syntax, not part of PHP's syntax. The variable appears inside a PHP double quoted string, so it will be substituted with its value before it's given to mysql_query(). As for the problem, you can't do like foo.php?book_title=C++ because a + will be decoded as a space.
-
You would want to use md5_file() on $_FILES['file']['tmp_name'] instead. It needs a path to a file on the filesystem and it returns the MD5 hash of its contents. Either you can store MD5 hashes for files in a database and do a query to check if it already exists, or you can iterate through all the files in your upload directory, get their MD5 hash and compare it to the new one. A file can still be a duplicate even though it has a different name. On your computer, try to make file and then copy-paste it in the same folder with a different name. Do we agree it's a duplicate? One heuristic for checking whether a file is a duplicate or not can be comparing hashes of their contents, in this case using MD5.
-
Single quoted strings do not have variable interpolation.
-
No, we'll not help you break the law if that's what you're asking. Personally, I don't care whether you pirate things or not, but you won't get help doing it here. Check out Can You Run It? to check if your computer satisfies the system requirements for a particular game. It obviously doesn't contain all games, but it contains a lot. Otherwise, find the system requirements on the publisher's website and compare them to your own hardware. Generally, built-in graphic cards aren't really suited for playing games.
-
http://www.google.com/search?q=toshiba+m35x+specs -> http://www.mobilewhack.com/reviews/toshiba_m35xs163_review.html As I said:
-
Well, you cannot send an email to [email protected]. How do you imagine your script should catch that? That's why the typical way of checking the existence of an email address (as opposed to its validity) is to send an email to the user and have them follow a link with a unique, random code. If they got the code, they must have gotten the email.
-
You should use prepared statements if you are using PDO or MySQLi. Rough, untested example: <?php $db = new PDO('mysql:host=localhost;dbname=test', 'user', 'password'); if ($_SERVER['REQUEST_METHOD'] == 'POST') { $errors = array(); if (!($name = filter_input(INPUT_POST, 'name'))) { $errors[] = 'Missing name.'; } if (!($email = filter_input(INPUT_POST, 'email', FILTER_VALIDATE_EMAIL))) { $errors[] = 'Invalid email address.'; } if (count($errors)) { echo 'Errors:<br>' . join('<br>', $errors); // make this better of course } else { $stmt = $db->prepare('INSERT INTO people (name, email) VALUES (?, ?)'); $stmt->execute(array($name, $email)); } } else { // show form } No, that's a perfectly valid email address. The validity does not refer to its existence, but its potential existence, i.e. is the format valid.
-
Could you please submit a bug report at http://bugs.php.net then?
-
Buy a better computer.
-
How does filter_var() not work for emails? if (!filter_var('[email protected]', FILTER_VALIDATE_EMAIL)) { echo 'invalid email'; } if (!filter_input(INPUT_POST, 'email', FILTER_VALIDATE_EMAIL)) { echo 'invalid email'; } Have you checked out the manual? http://php.net/pdo http://php.net/pdo.connections http://php.net/pdo.prepared-statements
-
Finding a Char from string and make that Link
Daniel0 replied to binumathew's topic in PHP Coding Help
I think you've got some misconceptions about both the purpose of this forum and how learning works. This forum is not made so people can write code for you on-demand, and learning does not work by having others do it for you. -
Also take a look at filter_input and filter_var.
-
Right, so in average it's 0.000000247 seconds slower on your system? You better watch out it doesn't kill your server
-
But that's also the binary representation for 253, how can you tell the difference? You cannot represent 253 with a signed 8-bit int. Half the range goes to the negative integers so the max number is 2^7-1=127.
-
Yeah but not when you nest them. Readability should never be sacrificed in favor of fewer lines. The code is for you and other humans to read after all.
-
42
-
It's fairly easy. <?php function myip2long($ip) { list($o1,$o2,$o3,$o4) = explode('.', $ip); return ($o1 << 24) + ($o2 << 16) + ($o3 << + $o4; } var_dump(myip2long('4.5.6.7'), ip2long('4.5.6.7')); function mylong2ip($n) { return sprintf('%d.%d.%d.%d', $n >> 24, ($n & 16711680) >> 16, ($n & 65280) >> 8, $n & 255 ); } var_dump(mylong2ip(67438087), long2ip(67438087));
-
You typically use "two's complement" for representing negative integers in binary. Go look it up. It's fairly easy though. To flip the sign you just flip all bits and say +1. So if you have +3 using 8 bits: 00000011 Flip all bits: 11111100 Add 1 and you now have -3: 11111101 Now flip all the bits: 00000010 And add 1 and you're back at +3: 00000011
-
Uh... ever heard about HTML5?
-
ip2long, long2ip
-
Sorry, but I don't see how that would protect you. How is it more difficult doing POST /foo/bar.php HTTP/1.1 rather than GET /foo/bar.php HTTP/1.1 in your request? Using $_POST instead of $_GET or $_REQUEST is at best security theater. There is nothing inherently wrong with register globals. The problem begins when people don't initialize their variables before using them. The only reason why register globals would be bad is because it makes it easier for stupid people to screw up. The only place where register globals would be a security issue is when you otherwise would have gotten an E_NOTICE. That being said, I don't like using register globals either, but that's just a matter of style.
-
If you're vulnerable to CSRF you're vulnerable regardless of whether you use $_GET, $_POST, $_REQUEST or filter_input.
-
You can do like this: <?php $arr1 = array(1,2,3,4,5,6); $arr2 = array('a','b','c','d','e','f'); $shuffle = array(); for ($i = 0, $size = min(sizeof($arr1), sizeof($arr2)); $i < $size; ++$i) { $shuffle[] = array($arr1[$i], $arr2[$i]); } shuffle($shuffle); foreach ($shuffle as $i => $v) { list($arr1[$i], $arr2[$i]) = $v; } var_dump($arr1, $arr2); Of course you need them to have equal length.
-
Can't you just use bindec?