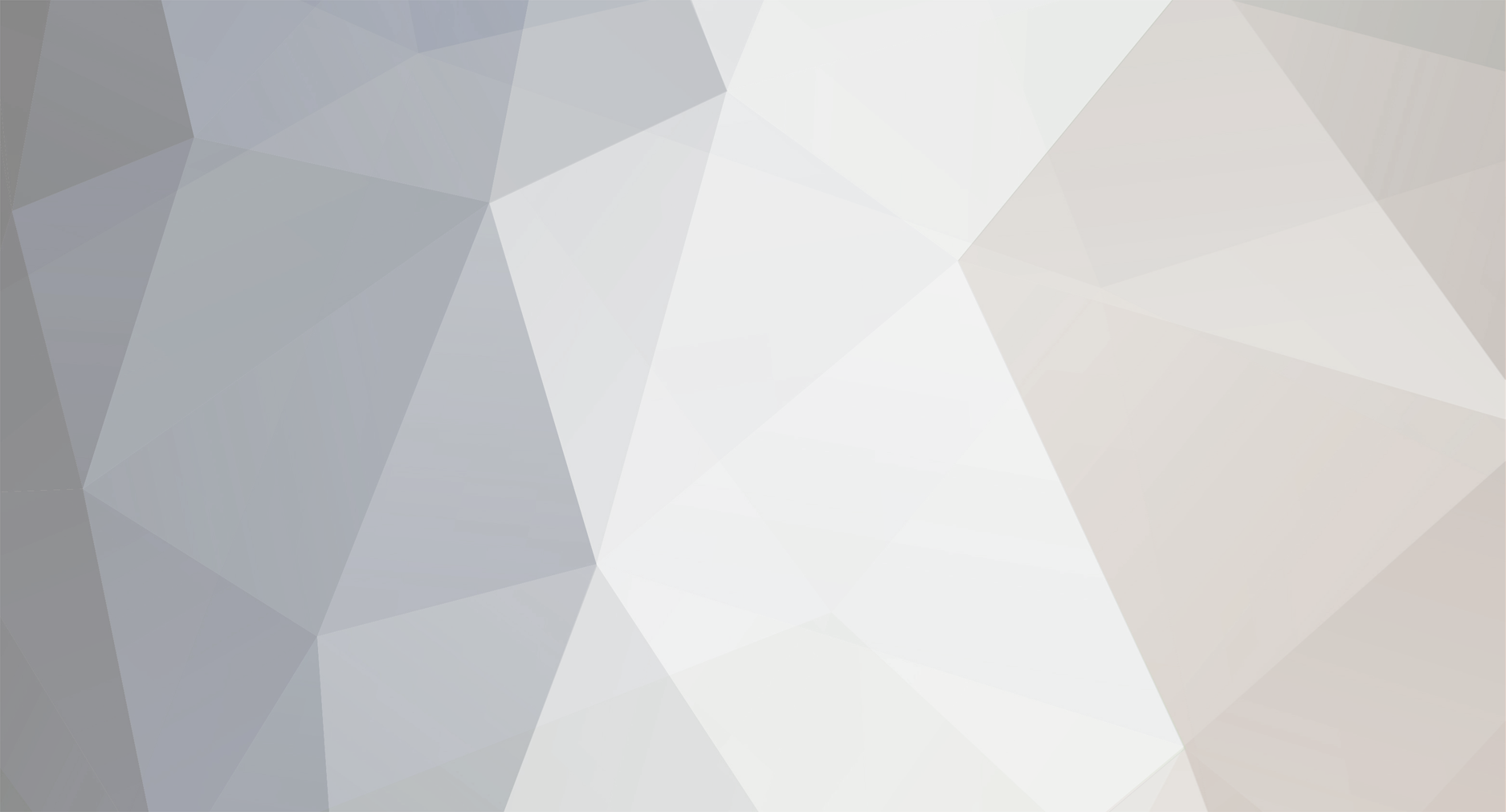
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Don't make separate threads for the same problem. Take a look at your logic: You're always going to be posting your form to manage.php?serv=del&id=&del=true because of this line: <form action="manage.php?serv=del&id=<?php echo $serverid; ?>&del=true" method="post"> Why? Because when your page loads, $serverid is not initialized, so it will contain a default value. In this case, either an empty string or 0. Your if-conditionals are failing because of this: While($server_b = mysql_fetch_array($server_a)) { $serverid = $server_b['id']; $servername = $server_b['servername']; $banned = $server_b['ban']; $datemade = $server_b['added']; $premium = $server_b['premium']; $serverhost = $server_b['serverhost']; $serverport = $server_b['serverport']; } You actually have a couple things going on here: 1. Since you're looping over your results, $serverid will only contain the $server_b['id'] value of the last row of your result set. 2. That value likely won't be equal to $_GET['id'], which is, like I said before, either an empty string or 0 since $serverid isn't initialized when you output your form's action.
-
Can you show that code? $serverid is a key part of your conditional statements. If it's not being set properly, then nothing will show.
-
I also like the idea. I think you could transform what you've already done with your existing site into a series of lessons and/or tips, perhaps in parallel with Maq's idea. Sort of like: "Here's what I originally had. Here's how I changed it. Now, let's look at trying it from scratch for a new layout."
-
I also think you should keep an old version of your site online. It would be a great way to show the before and after for the newbie tutorial you're thinking of writing (which is also a good idea). Simply put the old site's folder/file structure in a top-level folder named 'archive'. That will keep relative file paths the same while keeping index.html from interfering with index.php. The old site could then be accessed through bayarearcsociety.com/archive/index.html
-
Again, where is $serverid coming from? It's not a value coming from your form, so where are you initializing it?
-
Trying to build a drop-down menu that appears on mouse-over.
KevinM1 replied to AdrianRobinson's topic in PHP Coding Help
For compatibility with older browsers (read: IE6), JavaScript is necessary. The bulk of it, however, can be done with HTML and CSS. Google 'Son of Suckerfish' for code examples. -
GET and POST are supposed to be used according to what the words mean in English. GET is used for non-mutable requests, such as retrieving data. POST is used for mutable requests, such as, well, posting data. Sessions aren't part of HTTP, but are used to keep information 'alive' between HTTP requests. Things get a lot simpler when you code with semantics in mind.
-
Where's $serverid coming from? Is its value set anywhere upon page refresh?
-
Does inc_theme.php render any output?
-
You validate according to the kind of data you expect. Is a field only supposed to contain numbers? Check to see if it does. If not, display an error. Is a field supposed to contain letters and certain particular non-alphanumeric characters? Use regex to enforce the format and display an error for incoming data that doesn't comply.
-
Global variables. Yay or nay? Alternatives maybe?
KevinM1 replied to bachx's topic in PHP Coding Help
What advantages do sessions have over global variables? I was thinking along the lines of data retrieval, as that was your main concern. Apps live on HTTP requests. Storing your data in sessions will mitigate having to retrieve this data from the db after each request. As far as passing the data into functions, yes, use the argument list. I agree with MrAdam about adopting an OO approach. You can easily encapsulate db data in an object and then store that object in sessions to keep it 'alive' for as long as you need. You also gain the added benefit of having the data object being able to handle its own updating and persistence. -
Hidden fields are only 'hidden' in that they don't appear on screen. They're still visible in your source code. Also, mysql_real_escape_string is only part of the battle. You still need to validate incoming data.
-
Global variables. Yay or nay? Alternatives maybe?
KevinM1 replied to bachx's topic in PHP Coding Help
Why not store it in sessions? -
PHP doesn't have the concept of a main function/method. Essentially, whatever is in the outermost/global scope is main. Your code would be written as: function subA(/* argument list */) { // function code } function subB(/* argument list */) { // function code } $localVar = "I'm a local variable."; subA(/* passed in parameters */); You can 'insert' with include, but there's no functionality for deleting or appending functions.
-
Can you show an example of how/where you're including it?
-
Error: Using $this when not in object context
KevinM1 replied to chaseman's topic in PHP Coding Help
The error is clear in what it's saying. The 'this' keyword can only be used in an object context, which means only when you're writing a class definition. If you're not writing code which is part of a class, you can't use 'this'. -
VS PHP for Visual Studio
KevinM1 replied to aabruzzese's topic in Editor Help (PhpStorm, VS Code, etc)
I was actually curious about this myself. I currently use Notepad++ for my PHP needs, but I love VS 2010. Does the PHP extension have intellisense? -
Do you ever actually invoke your epn_submit functions? All I see in your second file is you directly accessing epn_class' data members. Since these are hidden input values, you need to: 1. Display your form (which is done by echoing the return value of one of your epn_submit functions) 2. Submit your form 3. Capture the $_POST values in the second file. Just writing a function definition does NOT make the function run. You need to invoke it by: $ps = new epn_class(); echo $ps->epn_submit(); Depending on which function you actually need to run. A class definition is simply a blueprint. It's up to you to create an object of that class and invoke its functions.
-
Which variables, specifically, are you trying to pass? General tips: Never, ever, ever use the 'global' keyword. Pass parameters to a function through its argument list. That's why argument lists exist. You also don't need to pass $_POST in through an argument list. $_POST is always available to you. Finally, rather than manually include-ing your class files, look into autoload-ing them instead.
-
Can't pass DOM object to function without breaking everything
KevinM1 replied to a topic in Javascript Help
Use Firebug while you develop. It has nice JavaScript errors. -
Looking for a more efficient to find and process multiple IDs
KevinM1 replied to cyberRobot's topic in Javascript Help
It's mostly a stylistic thing. I've heard some others say it's a bit faster than the post-increment, but I'm not entirely sure about that. For for-loops, since the index simply needs to be incremented without any kind of value check until after the incrementation, it doesn't really matter when the incrementation takes place. I figure why not do it as soon as possible? So, yeah, mostly for irrational, "it's different" reasons. -
Instead of trying to call the method several times, why not simply pass in an array of scripts you want to have on each page? class Page { public function display_js($scripts) { foreach($scripts as $script) { echo "<script type='text/javascript' src='$script'></script>\n"; } } } $scripts = array("js/jquery-1.3.2.min.js", "js/revealClass.js"); $page = new Page(); $page->display_js($scripts); If you have one script that's necessary for every page, you can have it as a data member of your Page class, then add it as the first element of the incoming $scripts array.
-
Yeah, you did it wrong You simply need: $current = $_GET['category']; ?> <ul id="subnav"> <li><a href="gallery.php?category=Wedding" id="wedding" <?php if ($current == 'Wedding' || $current == 'wedding') {echo 'class="selected"';} ?>>Wedding Hair</a></li> <li><a href="gallery.php?category=Men" id="mens-styles" <?php if ($current == 'Men' || $current == 'men') {echo 'class="selected"';} ?>>Mens Styles</a></li> <li><a href="gallery.php?category=Women" id="womens-styles" <?php if ($current == 'Women' || $current == 'women') {echo 'class="selected"';} ?>>Women's Styles</a></li> <li><a href="gallery.php?category=Salon" id="salon" <?php if ($current == 'Salon' || $current == 'salon') {echo 'class="selected"';} ?>>Salon</a></li> </ul> You should really brush up on how to handle the superglobal arrays $_GET and $_POST, as you'll be dealing with them all the time if you stick with PHP.
-
Simple question, how to make javascript work with mutliple rows?
KevinM1 replied to Jason28's topic in Javascript Help
Well, right now you have two spans with the ID of 'demo', so you'll first need to change that. Elements can't share the same ID. And, like I said before, it depends on what you want to do, exactly. You could simply do something like (pseudo-code): var rows = document.getElementsByTagName('tr'); // change depending on what kind of element you need var matches = new Array(); for(var i = 0; i < rows.length; ++i) { if (/* rows[i] matches a regex pattern for your collection of IDs, like demo1, demo2, demo3, etc. */) { matches.push(rows[i]); } } for(var j = 0; j < matches.length; ++j) { matches[j].onclick = function() { // event handling code } } -
Why not simply use $_GET['category']?