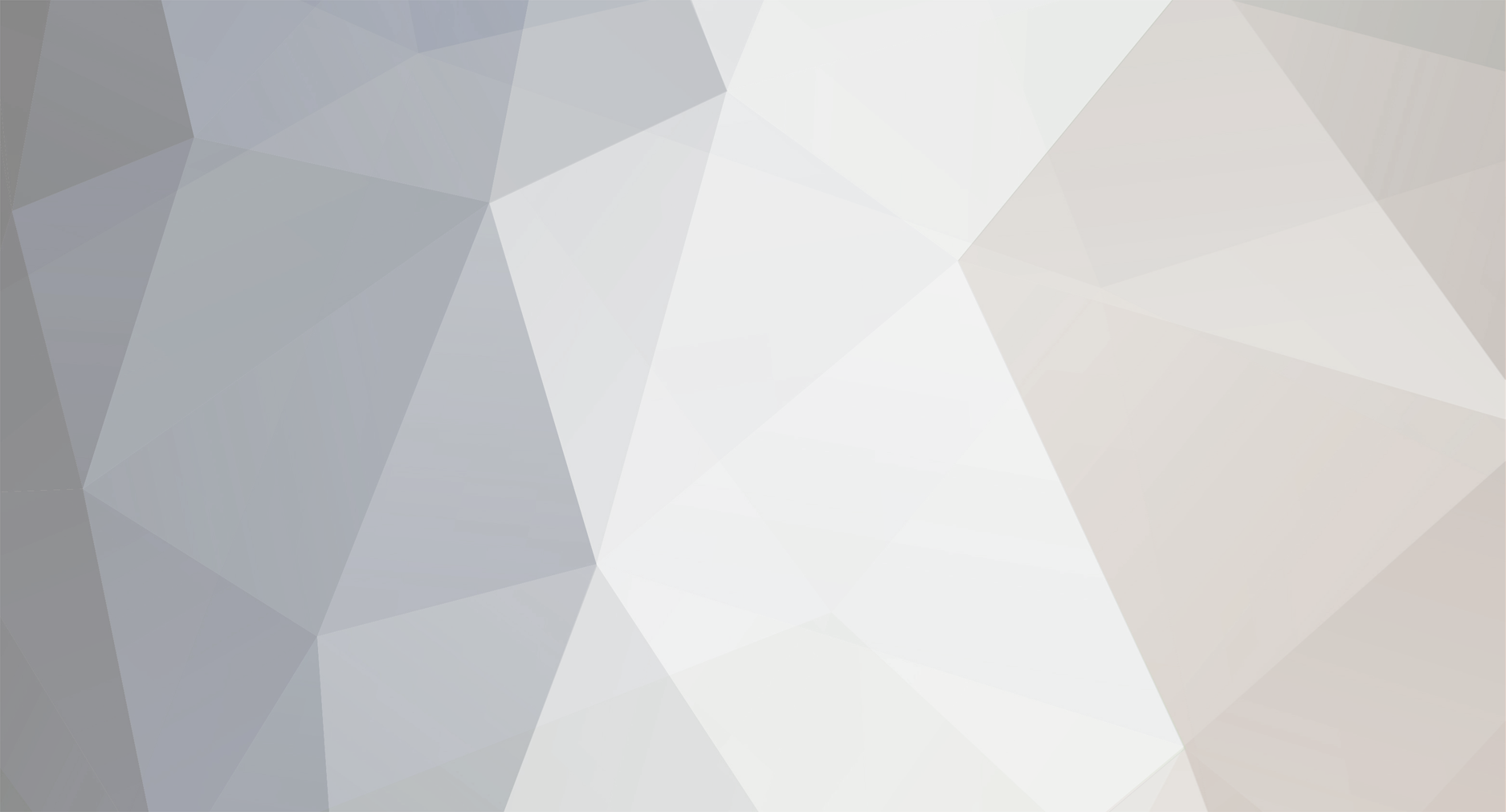
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
To elaborate on some stuff, did you try just calling the getdata.php?id=22 page and see what displays there? View the source and see what shows up. <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> </head> <body> <img src="getdata.php?id=22" /> </body> </html> <?php // display.php error_reporting(E_ALL); var_dump($_GET); ?> That var_dump will be empty, cause you make a remote call out the getdata.php page. Doing this you set the get data inside the image tag. Therefore it would return empty. Check out that http://www.yoursite.com/getdata.php?id=22 page and see if there are any errors and view the source. If there are errors that is the issue. If there are not then we will continue from there.
-
Tried helping, it looks like you are not even reading what was written before hand. I suggest you re-read the entire posts in this thread and take out the information that was supplied by the users. I know I posted a clean version of this code and I see non of that code being implemented or used in this version, it looks like you just reverted back to your original bad code.
-
Is this on an actual site or on Localhost? If on localhost check out this article: http://www.aeonity.com/frost/php-setcookie-localhost-apache Why are you setting the cookie soo many times? I am not sure if this matters, but pHp is CaSe SenSitIve and you are using SetCookie, to be a good coder I would change it to be setcookie just to avoid any possiblity of error now or later. So A: remove the 4 other checks, change the setcookie to lowercase and let us know if you are working on LocalHost as localhost has to have cookies set a different way, unfortunately.
-
You could but I do not think it would help the situation. You want to test if the user inputted a url or a name. If it was a URL (tested by finding either www. or http:// using stristr or eregi). If it is you check it differently, if it does not contain those then verify the username according to your standards. I know there are more elaborate URL checkers online or even in the PHP.net comments portion on either eregi or ereg which can test if it is a URL with any type of form it can be passed in. I do not think stripslashes will be useful with what you are trying to do honestly.
-
[SOLVED] Retrieving values from Associative arrays?
premiso replied to idire's topic in PHP Coding Help
This function is designed wrong. function fetchArray($query) // Return query result as an associative array { $result = $this->query($query); //$result = mysql_fetch_assoc($result); while($r = mysql_fetch_assoc($result)) { $result[] = $r; } return $result; } the fetch_Array will return both assoc and non-assoc, the above will not just return the assoc, saves on array space. Onto QUestion 1: You would generally only use the query function to execute the UPDATE, INSERT and DELETE queries outside of the class unless you are looking to do something different. I would do it like this instead: $result = $con->fetchArray("Select * from `user`"); That way it returns the array, if you want it to and you do not have to do the extra code. The point of the class is to reduce repeat code by having functions do the work for you instead of having to code it each time you need it. Anyhow I hope that helps you with your questions. -
If you want to check contents you can use stristr or eregi If you want to check length you can use strlen You can also let the user know that certain characters are not allowed, and disallow the use of the / : and . in the username using the same functions above. Hope that will help you to get started. I am sure there are a ton of tutorials of form validation online for php that are already created so you can use that as an example and take out the parts you want/need. I know on the eregi page they have zipcode validation procedures etc in the comments.
-
Are you sure that getdata.php?id=22 does not display an error. Given that you have error reporting turned on I would check that the page is actually pulling the data from MySQL and is not displaying any text that it shouldn't, as that would throw off the image and have it display the red X
-
I think your header is wrong. Header( "Content-type: $type"); Header( "Content-type: image/jpg"); echo $data; exit; ?> Another way to check is to browse to the actual image page and make sure there are no errors on the getimage.php page. If there is it would show up there or if not it should show the image.
-
Urgent Advice Needed... PHP, Unix, Firefox, Chrome
premiso replied to MediaMonkey's topic in PHP Coding Help
Yep if that phpinfo(); function cannot even display it is definitely a server issue. I would remove the HTML portion of that and just have the php on a page, test it again and if it just displays the sourcecode email your provider with a link to that page letting them know it is THEIR issue not yours. -
Solving this portion. <?php if (isset($_POST['info'])) { $info = trim($_POST['info']); if ($info == "" || empty($info)) { $message_info = "Please enter data for info!"; } } ?> Trim should trim out any whitespaces at the end/breaks and empty will check if PHP considers the variable to be empty. Untested but I am pretty sure that would work.
-
Basically your GET data is not getting populated due to not checking if the form has been submitted. Sure you have the form output on that page but it has to be submitted first and you are not checking if it was submitted. To do this I added a hidden input with a name of "submitted" and a value of "yes" to the HTML. <html> <head> <title>Loggin DB</title> </head> <body> <body bgcolor="#82CAFA"> <form method="get" action="loggin1.php"> <input type="hidden" name="submitted" value="yes" /> Page: <select name="Page"> <option value="approve.php" >Approve</option> <option value="custquery" >CustQuery</option> <option value="slpnlogin">SlpnLogin</option> </select> User Code: <select name="User Code"> <option >T3</option> <option >J9</option> <option>BL</option> <option >SC</option> <option >MN</option> <option >CA</option> <option>MW</option> <option >TJ</option> <option >E2</option> </select> Date: <input type="text" name="Date"> <p><input type="submit" value="send"/></p></form> <?php echo $_GET['Page']; if (isset($_GET['submitted']) && $_GET["submitted"] == "yes") { $db = $_SERVER['DOCUMENT_ROOT']."/../data/log.db"; //connect to sql $handle = sqlite_open($db); $query = "SELECT * FROM log where id > 85076"; $query .= " and page='" . $_GET["Page"] . "' and userCode='" . $_GET["userCode"] . "' and time='" . $_GET["time"] . "'"; echo $query; $result = sqlite_query($handle, $query); $row = sqlite_fetch_array( $result ); echo "<table>"; while($row = sqlite_fetch_array($result)){ echo " <tr>\n"; echo " <td> " . $row['id'] . "</td><td>" . $row['page'] . "</td> <td>" . $row['desc'] . " </td><td>" . $row['userCode'] . " </td><td>" . $row['codeType'] . " </td><td>". date( "Y-m-d", $row['time'])."</td><td> " . $row['ipaddr'] . "</td>\n"; echo " </tr>\n"; } echo "</table>"; } ?> </html> That way the code that requires the query will only run if the form has been submitted. Also modified the while loop, as how you were pulling the data/calling the data inside the echo statements was wrong. I would read up more on the syntax of PHP and variable usage in echos/strings.
-
You want to look into AJAX, check out the AJAX forum, or do a simple google. You should find mounds of tutorials on it.
-
Urgent Advice Needed... PHP, Unix, Firefox, Chrome
premiso replied to MediaMonkey's topic in PHP Coding Help
The number one check I usually do to verify that my site is running PHP/APACHE fine is invoke the phpinfo function on a test page. If that page shows up just fine, than it is your code, if it does not it is the server. <?php phpinfo(); ?> Check the versions of your development server's PHP and your new server's PHP. If the PHP versions are different that can break code too. -
I did not see where you modified the code to go with PFMa's. So here is another version, that would work also. <?php $query .= "and page='" . $_GET["Page"] . "' and userCode='" . $_GET["userCode"] . "' and time='" . $_GET["time"] . "'"; ?> That is the proper way to use concatenation. In your code about it would have been sql error galore due to no equals sign, no ' and no space between the last statement and "and". As seen above is the correct way to write the sql and use concatenation between variables.
-
file_get_contents with regex is a good way to do it. Given that the url allows hotlinking.
-
Sorry I was not hinting that he should change his server to parse .xhtml as php. Should have been clearer on it. mod_rewrite would be better, that way you would not have to create a file plugins.xhtml, or you could even have the .htaccess do the redirect for you. http://www.isitebuild.com/301-redirect.htm Will help with the .htaccess 301 redirect.
-
Some code would be very helpful.
-
Your server is not setup to parse .xhtml as php.
-
You are mixing PHP with HTML and php cannot parse that you need to exit in and out of PHP or echo the html out. <?php $domesticGoods = "SELECT categoryName FROM categories WHERE categoryType='Domestic' OR categoryType='International' "; $domesticGoodsResult = mysql_query($domesticGoods, $connected); $internationalGoods = "SELECT categoryName FROM categories WHERE categoryType='International'"; $internationalGoodsResult = mysql_query($internationalGoods, $connected); echo '<form name="categoryRegister" id="categoryRegister" method="post" onsubmit="return categoryRegistration()"> <table width="600" border="0" cellpadding="10" cellspacing="0"> <tr> <td>'; while($row = msql_fetch_assoc($domesticGoodsResult)){ echo '<center><input type="checkbox" name= ' . $row['categoryName'] . ' id=' . $row['categoryName'] . ' value=' . $row['categoryName'] . ' />' . $row['categoryName'] . '</center><br/>'; } echo '</td> <td>'; while($row = msql_fetch_assoc($internationalGoodsResult)){ echo '<center><input type="checkbox" name= ' . $row['categoryName'] . ' id=' . $row['categoryName'] . ' value=' . $row['categoryName'] . ' />' . $row['categoryName'] . '</center><br/>'; } echo ' </td> </tr> <tr> <td colspan="2"><center><input type="submit" name="submit" value="Sign-Up"/></center> <input type="hidden" name="type" value="goodsInfo" /> </td>'; ?> Edit also assumed that you wanted it to be just "International" or "Domestic" and changed that portion as well.
-
Your if statement is checking the wrong one. Change the function to this: function dose_user_exist($username) { db_connect(); $query = "SELECT id FROM users WHERE username = '$username'"; $result = mysql_query($query); if (mysql_num_rows($result) == 0) { return false; } else { return true; } } Your if for dose_user_exist was returning true for if the user did not exist and false if he did exist.
-
The first spot I see is that you check mail for true/false this indicator is buggy and often returns the wrong result. Also I do not see the redirection done in the code... Also you are not using Mail Headers, mail has examples of them, although they are not needed if they are not there alot of email apps will block them as spam.
-
Weird stuff, maybe php has the limit and not the OS? not sure. Anyhow you could use the exec and use the Unix commands to rename the file that way. Not ideal, but it should work. A side note I would do this: <?php if (file_exists('./upload/5b4875453cbcb59713701e17e495de4c')) { rename('./upload/5b4875453cbcb59713701e17e495de4c', './upload/32876fc3df25fb68fb9e398b5d7d7977'); }else { echo 'Unable to rename the dang file cause it does not exist.'; } ?> On a lighter side, I am sure it is some stupid little mistep and when you figure it out you will rip your hair out at how easy it was to find.
-
$sql = "INSERT INTO people (`person_id`, `firstname`, `surname`, `from`, `dob`, `bio`, `modifiedby`) " . "VALUES (NULL, '$firstname', '$surname', '$from', '$dob', '$bio', '$editby')"; I am sure from is a keyword in mysql, use backticks ( ` ) to define columns to avoid this issue.
-
Yep, however if you do not re-create the table using data converted to the timestamp you may lose all the dates prior when you changed this. (Assuming this is on a production server and not a development/test server).
-
The timestamp column type is just bad news. If it was me, depending on how many records are currently there, I would do a dump of all the rows via php and convert that time column to a unix timestamp value and put it into a text file as an INSERT statement to the database. Then I would dump all the current data in the table and change the row type to INT(11). But that is just me and it does depend on how many rows you currently have to tell whether that is probable or not.