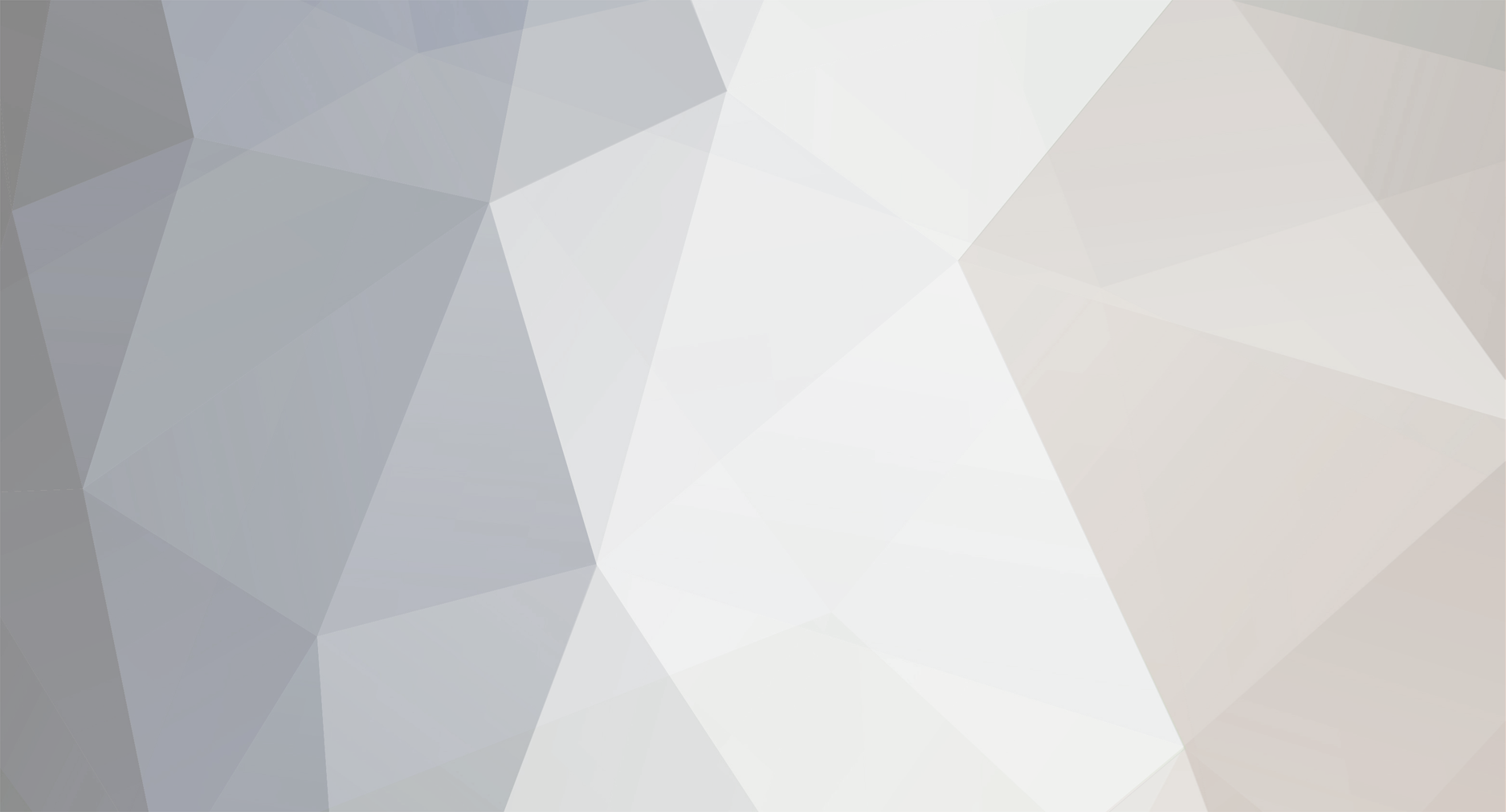
lemmin
Members-
Posts
1,904 -
Joined
-
Last visited
-
Days Won
2
Everything posted by lemmin
-
Why not just check how many home games each team has when you are matching them up and give the home game to whichever team has the fewest?
-
You really should use a database for this, but this code should work: if ($f = fopen('file1.txt', 'r')) { $c=1; while ($line = fgets($f)) { if (preg_match('/^\d+/', $line)) break; $c++; } fclose($f); if (($word1 = getLine('file2.txt', $c)) && ($word2 = getLine('file3.txt', $c))) $string = $word1 . ' ' . $word2; else $string = 'File lines didn\'t match'; echo $string; } function getLine($file, $n) { if ($f = fopen($file, 'r')) { for ($i=1;$i<$n;$i++) { if (fgets($f) === false) return false; //file line not found } $line = fgets($f); fclose($f); return $line; } }
-
PHP keyword search of file names and directories, display results?
lemmin replied to sleepyw's topic in PHP Coding Help
You aren't ever checking the keyword against anything. Add an if statement to check the keyword in your first iteration of the files: foreach ($files as $file) { if ($file!="."&&$file!="..") { $extention = explode('.', $file); if ($extention[1] != "") { if (stripos($extention[0], $keyword) !== false) { echo "<div class='imgwrapper'>"; echo"<a class='fancybox' rel='group'' href='$dir$file' return false' title='$filename'>"; echo "<img src='timthumb.php?src=$dir$file&h=$height&w=$width' alt='$extention[0]' width='$width' height='$height'/>"; echo"</a><br/>"; $file_name = current(explode('.', $file)); echo substr($file_name,0,21); echo "</div>"; } } } } The sample code expects that you are storing the file names in a database (which you should), but this code should work for what you have right now -
My first guess is that you have error reporting off and you've reached the max memory_limit. Try adding this above your code: error_reporting(E_ALL);
-
I'm not 100% sure I understand, but you should be able to load all of the associative information into arrays instead of querying each relationship individually. The goal would be to turn the logic into more of a math problem than a decision tree. Maybe consider the differences between the career numbers and the answers to come up with a linear relationship. For example (using your example data), the student answers differed from the Doctor answers by 4,3,2, and 1 respectively for a total of 10. Let's say a second career has the question numbers that match EXACTLY what the student entered (which would be the highest scored, right?). The differences are 0,0,0, and 0 for a total of 0. Now, if you do the same for all of the other careers and arrange them in ascending order, you have the the careers related to the student's answers. The percentage number depends on what it is based off of. You could use the range of 0 to the highest possible difference per question, or base it off of all the total ranges collected. If I'm wrong about your end goal, maybe post one example scenario with real data to go off of.
-
If it's a public URL, you can use file_get_contents() to load it into memory. It will return a false value if it wasn't able to download the entire file. After you have checked the success, you can save it using file_put_contents().
-
If the file is being uploaded to the server it is being saved on, no. The way you have worded the process is a little confusing. You ARE using a form to upload a file, right?
-
PHP and MySQL Graphing options? Recommendations....
lemmin replied to mdemetri2's topic in PHP Coding Help
You can use the GD library to create images however you want. There are a few libraries out there that provide graphing functions; Here is one: http://pchart.sourceforge.net/ Or, you could generate the graph in HTML and have the client render it. Here is a tutorial on graphing using the canvas tag: http://www.html5canvastutorials.com/labs/html5-canvas-graphing-an-equation/ -
You should really be using move_uploaded_file(). http://us3.php.net/manual/en/function.move-uploaded-file.php Always make sure to include error handling in your code. Check to make sure the file was uploaded successfully before copying it. If you post your code it would be easier to see where the problem may be.
-
The copy() function is what is naming and saving it. You are telling it to name it the original name, so just change that to whatever you want. You could use md5 hash to generate a pseudo-random name. Just extract the file extension first: preg_match('/\.[^\.]+$/', $_FILES['ufile']['name'], $match); $ext = $match[0]; $path = "uploads/" . md5($_FILES['ufile']['name']) . $ext; You might want to look over line 6. You probably meant to put "none" in quotes. Although, $ufile doesn't seem to be set so it probably isn't really doing anything.
-
With your HTML, $_POST['owner'] and $_POST['worker'] will either contain 'on' or not be set at all. Assuming you want to set a boolean flag in your database, you could put this before your query: $owner = isset($_POST['owner']) ? 1 : 0; $worker = isset($_POST['worker']) ? 1 : 0;
-
$_SESSION is a super global associative array. Any index key you set becomes a session-accessible variable. In other words, you can change 'name' to whatever you want. http://php.net/manual/en/language.variables.superglobals.php
-
If you try to output hex values, they will show up as decimals in your browser. echo 0xA5611BCD; If you look at the documentation for dechex(), it actually returns a string, not a number. A number is the same number no matter what base you convert it to. If you actually want the hex string, requinix's solution gives you the exact output you asked for in your first post. If you want the integer values of each hex string, just run hexdec() one time. $strings = str_split($getSKEY, ; foreach ($strings as $string) echo hexdec($string).'<br/>'; I think the answer to your question relies wholly on what you are trying to accomplish.
-
Just to be clear, you DO need to pass the mysqli connection resource when calling mysqli_query(). You do NOT need to if you are using a variable in object context, which he is not. http://us3.php.net/manual/en/mysqli.query.php
-
Like I said, the problem is with the data correlation. Your query has: $id = intval($_POST['question']); [..] WHERE question='$id' You set $id to an integer, then test it against a string. Based on your screenshot, there are no values of "question" that would match an integer comparison. That is why you never get any rows from your query. Assuming $_POST['question'] actually contains an id, you query should be against the id column: WHERE id=$id
-
Is productID in the URL? When you say "nothing," do you mean a completely blank white page? Have you checked your error logs?
-
Your loop is overwriting variables. What exactly are you trying to do?
-
I don't think $_POST['question'] and $_POST['answer'] contain the values that you expect. I don't see anything wrong with your code, so if it is never returning anything from the database, then the criteria probably isn't matching any rows. Either that or you don't have the data you expect in your table. Also, question appears to be an integer, so you don't need quotes around it in the query. It should still work though.
-
I think the lack of response is indicative of the amount of Spanish-speakers on this website. It is hard to look through all your code not understanding the intention of the variables. Could you be more specific about what area in the code is at fault? You say that you are "setting" choices. Is that in the database?
-
You are right; it is always better to take security precautions. My response was based on every-day web security. If you are worried that your sha1 hash will actually be reversed, the salt is irrelevant. If your hashes are being brute-forced, a salt is only going to make it take longer. I guess I never consider any higher-level threats unless there are actual sensitive data being stored.
-
It even references it in the comments hehe: Try changing that function to this: function observed_day($year, $month, $day) { // sat -> fri & sun -> mon, any exceptions? $dow = date("w", mktime(0, 0, 0, $month, $day, $year)); $daystring = $year . '-' . $month . '-' . $day; if ($dow == 0) return $daystring . ' +1 day'; elseif ($dow == 6) return $daystring . ' -1 day'; return $daystring; }
-
It looks to be a glitch in the observed_day() function. The 31st of March 2013 is on a Sunday, so it adds a day and makes it the 32nd of March, which strtotime() can't parse, so it returns a null timestamp.
-
Your star logic seems like it should work just fine, assuming all images are of just one star and you are just printing each out next to each other. You might consider setting a flag in the database for the user, instead. You could do this logic when the gold is updated and set the flag for the star number. That way, you wouldn't have to check it every time a user's information is loaded. As for your hashing, sha1 is completely fine. No hash can be reversed without a rainbow table. The only reason to salt is to prevent someone from using a rainbow table to reverse a hash of a known string. As long as your salt is not a common string of characters, it is unlikely to ever be a part of a known sha1 hash. In this case, you can use the same salt for EVERY password and be safe. A changing salt consoles those who are paranoid of their salt being discovered. Since a static salt is usually in the code and the information about what dynamic salt is used would also be in the code, I find the two methods quite similar in terms of security.