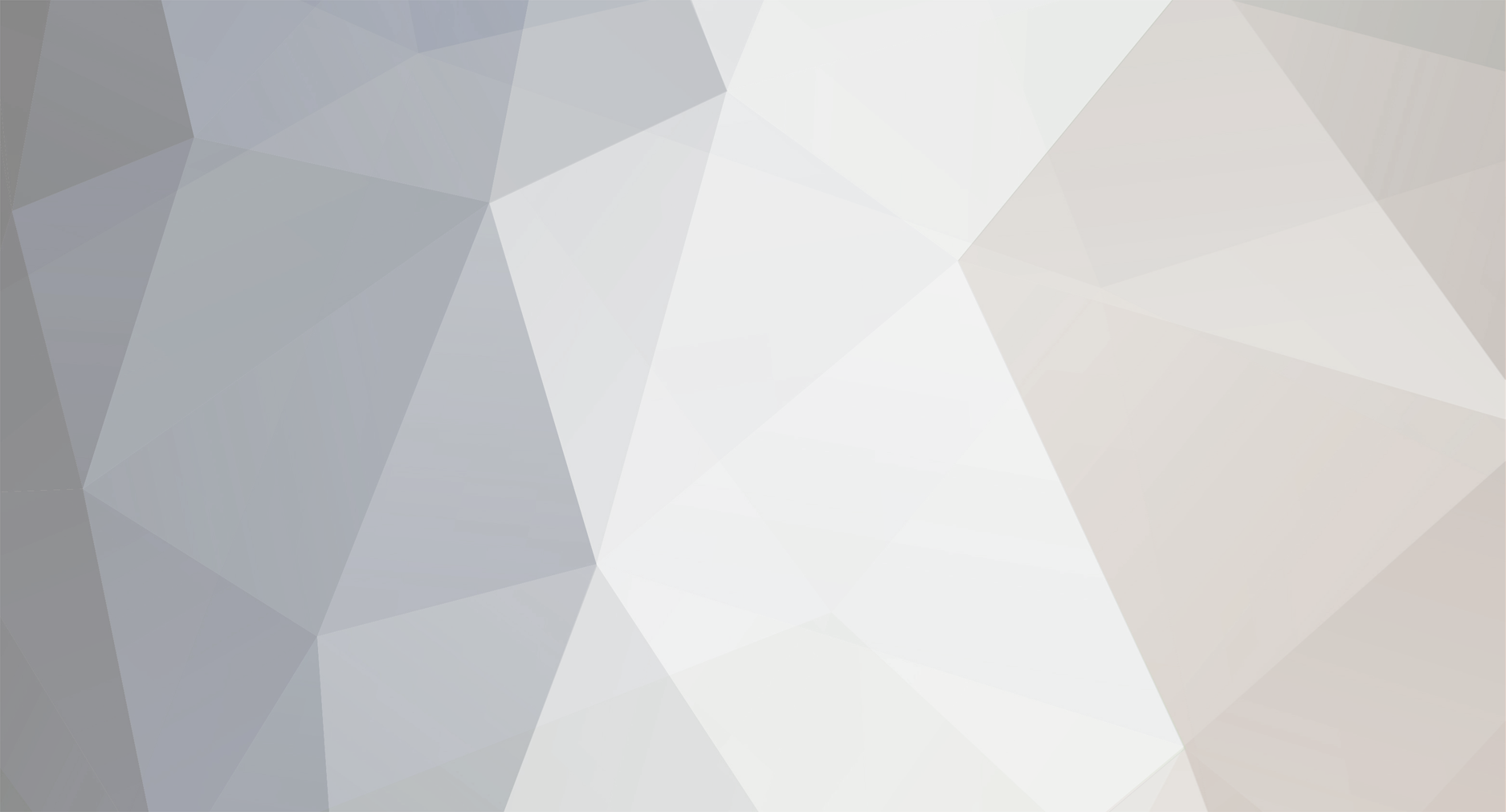
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
With so little code provided, I can only guess. But this snippet will unconditionally set the $data['filter_cust_group'] element, so isset will always be true. You should set some value in the HTML (not zero and not an empty string), then use empty instead of isset. if (empty($data['filter_cust_group'])) { // The box is NOT checked } else { // The box IS checked }Also, it is important to note, this snippet and the fact that you can't find $_POST in the code, would indicate that your code relies on register_globals to be on. This "feature" of PHP has been deprecated and has been removed in the most recent releases. Your code will break if your PHP engine is updated in the future. You need to think about revising your code to NOT depend on this "feature". P.S. Yes, there is a time limit on editing posts on this forum.
-
Checkboxes are only submitted if they are checked. So your logic using isset() should work. The only issue I see is that there is no value provided in the HTML, so there is nothing to submit if it is checked. You might try: <input type"checkbox" name="filter_cust" value="1">This assumes that somewhere you have copied the data from $_POST to $data. You might check the code that does that copy to see if it is only copying specific keys.
-
Please use ... tags for posting. If there are headings in the file, you would need to capture them on the first pass of the loop: <?php /* */ $newname = $_GET['newname']; //The file that has been moved into the Uploads/ folder will need to be stripped here to retreive just the filename... $filename = basename($newname,"/"); //basename function strips off filename based on first forward slash "/" $row =3; if (($handle = fopen($filename, "r")) !== FALSE) { $headings = array(); # MAD - Added while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) { if (empty($headings)) $headings = $data; # MAD - Added $num = count($data); echo "<p> <br /></p>\n"; $row++; for ($c=0; $c < $num; $c++) { echo $data[$c]; echo " "; } } fclose($handle); }
-
If the value is actually a numeric value, you can use number_format, specifying a space as the thousands separator. If the value is a string of characters limited to the digits 0 - 9, there are a couple of options: 1) Reverse the string, split it, then reverse the result; 2) Pad the string so the length is a multiple of 3, split it, remove the padding. # An actual number $int = 123456789; echo number_format($int, 0, '.', ' '); # A String - Reverse it, split it, reverse the result $rev = strrev($int); $split = trim(chunk_split($rev, 3, ' ')); echo strrev($split); # A String - Extend it, split it, remove padding $padded = str_repeat('?', 3 - (strlen($int) % 3)) . $int; $split = chunk_split($padded, 3, ' '); echo trim(str_replace('?', '', $split)); Of course, there is the brute-force method using a loop to build the output one character at a time, but it's much more code, and I'm too lazy to write it right now.
-
Sorting titles without definite article advice
DavidAM replied to Big_Pat's topic in PHP Coding Help
Unless your database is the CSV file, you can restructure (normalize) when you load it. Of course, that means writing a PHP script to load the data rather than using INFILE. One common solution to your original question is to store a "sort" value for certain fields. i.e. titleSort, artistSort, albumSort, album_artistSort. When you load the data, you store the value to be used for sorting/searching in this field. So, the artist field may be "The Ramones" (for display purposes) and the artistSort field would be "Ramones" (for sorting/searching). If you are using a script to load the CSV file, then these values can be calculated just before the insert. If you are using INFILE to load the data, you can write a couple of UPDATE queries to set these fields after the load is complete. You might also look at your music program, it may be that it will export sort fields as well in which case you can maintain them there and send them to the CSV for import without any extra work. -
To the best of my knowledge, an AREA tag does NOT submit a FORM. It is basically an A (anchor) tag that opens a new resource (with a GET request). You have not defined any fields in the FORM tags, so I'm wondering what you are trying to POST? Even when you POST a form, anything in the url after the "?" becomes a GET element, only fields defined in the FORM become POST elements. Here is the code you posted, I just reformatted it a bit -- please use the ... tags for posting code (it's the <> button on the toolbar) <div id="bottom"> <img src="test.png" id="test" usemap="#testmap" width="0" height="0" border="0" style="position: relative; top:-0px; visibility: hidden;"> <img src="test.png" id="test2" usemap="#testmap" width="0" height="0" border="0" style="position: relative; top:-0px; visibility: hidden;"> <map name="test"> <form method="post" target ="top> <area shape="rect" coords="1,1,1,1" onmouseover="display(1);" href="test.php?id=0000"> </map> <map name="test2"> <form method="post" target ="top> <area shape="rect" coords="1,1,1,1" onmouseover="display(1);" href="test.php?id=0000"> </map> </form> </div> I've never used MAPs and AREAs, but from what I've read about them, I don't see how this code could "work" at all. 1. Both of your images refer to the same MAP, and that MAP ("testmap") does not exist 2. Both images have width and height of zero and visibility hidden -- perhaps there is some JavaScript in play here? 3. The FORM tag inside the first MAP tag is never closed 4. The FORM tag inside the second MAP tag is closed outside of the MAP tag -- You should never overlap block-level tags. 5. There are no form fields in either form to be submitted 6. The coordinates in both AREA tags define a single-pixel region (that's going to be difficult to click on) [edit] 7. Forms are submitted to the URL specified in the ACTION attribute of the FORM tag. If no ACTION attribute is specified it will submit to "itself" (the same URL as is displaying it). [/edit]
-
If you are actually going to be storing millions of IP Addresses, you may want to look at mySql's INET_ATON() and INET_NTOA() functions. These convert the dotted-decimal IPv4 address to a single 4-byte unsigned integer. Instead of storing 7 to 17 characters for each address, you store 4 bytes each. Your indexes will also be smaller.
-
Do NOT store dates as VARCHAR. You will not be able to use the mySql date functions, you will have problems with sorting and comparing, etc. etc. Always store dates as DATE (or DATETIME or even TIMESTAMP). The server accepts and returns them as YYYY-MM-DD. You can change the way it displays when you retrieve the data. Would you store numbers as "two hundred fifty-seven and 23/100" just so you can print it on a check? Of course not.
-
I saw that. What i was saying was that you did not pass $headers to the mail() function
-
You need to read up on "variable scope". The $db variable does not exist INSIDE the function since it is defined OUTSIDE the function (there may be others, I did not go through all of the code). You need to pass $db to the function in the parameter list.
-
You did NOT supply the $headers variable to the mail function call. Unless you have the FROM address set correctly in the php.ini, the mail server probably refused to relay the message because the FROM address is not "valid" or is missing. I say refused, because the TRUE response from mail() simply means that PHP ran the function and handed the message to the appropriate agent. PHP does NOT know if the mail server rejects the message (in general). [You handed the envelope to the postman, you did not see the clerk at the office throw it in the floor because he does not like red-crayon-addressed letters] Remember, the FROM address needs to be one that has authority to send mail using the SMTP mail server you are accessing. For instance, if you use the SMTP server at AAA.com and use a from address of BBB.com, the mail server may decide the email is forged (it's not coming from [email protected]) and refuse to relay it, or give it a high spam score. P.S. I have used mail from Linux for some very complex stuff. The first time I did it on a Windows 7 box running WAMP, I was surprised to see the message go with no real problem. I was fully expecting it to fail and was prepared to start searching for a sendmail() alternative, but thankfully that was not necessary.
-
There is no real reason to create a new variable for one that already exists. $_POST[id'] is perfectly valid and usable in this situation. It is IN FACT a variable -- a super-global array variable -- but a variable nonetheless. There is also no real reason to put the variables in a string over using concatenation. Neither of these "answers" address the problem he is having. They are both PREFERENCES of one programmer over another. If you are getting a "white page", use the "View Source" feature of the browser to see if there is output that is not rendered. Turn on error reporting. A white page indicates a fatal error. Probably a syntax error, that prevents PHP from doing anything. You have not shown enough of your code for us to really help. You can NOT mix mysql_* functions with mysqli_* functions. You are either using the standard (now deprecated) mysql module, or you are using the "improved" (hence the "i") module. Whichever one you connect with, is the one you MUST use for the rest of the script. I find it difficult, if not impossible to maintain code that has such long SQL lines built in. You are free to breakup these lines, and I recommend you do so: $sql = "UPDATE articles SET art_title='" . $art_title . "', art_image='" . $art_image . "', image_caption='" . $image_caption . "', image_src='" . $image_src . "', image_src_location='" . $image_src_location . "', article ='" . $article . "' WHERE artnum=" . $_POST['ID'] . "";Since that leaves the "closing" single-quote for each field on the line after the field, and because I find it difficult to pick out the variables, I generally use sprintf to build my SQL statements: $sql = sprintf("UPDATE articles SET art_title='%s', art_image='%s', image_caption='%s', image_src='%s', image_src_location='%s', article ='%s' WHERE artnum=%d", mysql_real_escape_string($art_title), mysql_real_escape_string($art_image), mysql_real_escape_string($image_caption), mysql_real_escape_string($image_src), mysql_real_escape_string($image_src_location), mysql_real_escape_string($article), intval($_POST['ID']));Note: I added escaping for the strings, and sanitation for the integer. I used the mysql version, if you are using mysqli it is a different function.
-
1. Most web-page scripts are very short lived, and should connect to the database only once. The delay caused by opening a connection (a second or third time) is more significant than the savings of closing it between queries. Keep in mind that the Database server is NOT going to immediately release the connection anyway; so closing it can actually cause the database server to do more work managing connections than it will save in resources. PHP will automatically close the connection when the script ends if you don't do it in code. 2. It is generally true that forms will POST data. However, the W3 specification says use POST for "actions" -- things that change data (i.e. add a record), and use GET for "requests" -- things that ask for data. True, if there are a significant number for fields, or size of data, POST is a better choice over filling the URL with data; however, I have written search forms to use GET, especially in cases where I might want to use a link on another page to go directly to specific search results. It simplifies the coding and prevents the need for two scripts that do essentially the same thing. 3. You are spot on about the $searchSubmit never having been assigned. In addition, the OP is using single quotes around the SQL statement, so the variable is not going to be interpreted. Also, the other single-quotes inside the string are going to cause a query error, since the first one will prematurely end the query. It should be:$sql = "SELECT * FROM PROPOSALS p WHERE p.Name LIKE '%$searchSubmit%'"; 4. OP: You need to shift your thought process slightly. Each page request is a separate activity. Putting the search (database) logic AFTER the form shows the implied sequence of events; but when the form is submitted it is a new run of the script. The best approach is to put all of the PHP logic at the top of the script, collecting the data you need. Then the only PHP in the HTML output is to echo the data you have collected. Something along these lines: a. Connect to database b. IF THE FORM WAS SUBMITTED 1. Validate the search criteria (i.e. it cannot be empty, etc) 2. If valid, SELECT the data to be displayed - store it in an array c. Output the HTML to display the form 1. ECHO the data from the PHP array in the appropriate places
-
Usually when an environment variable has PATH in the name, it contains ONLY the path (directory) not a full filename. Maybe try putenv('GDFONTPATH=' . dirname(realpath('cour.ttf')));
-
What the heck is that? Why do you have the condition you want to test in quotes? That IF statement is checking to see if the string is not empty. It is not empty because it contains "$unit == 'Apartment'" (literally). if ( $unit == 'Apartment' ) { header ("Location: $a"); } elseif ( $unit == 'Commercial' )
-
What is the problem? Tell us what it is doing that is should NOT do, or what it is NOT doing that it should. Turn on error reporting (at the beginning of the script) so you can see if there are any error messages error_reporting(E_ALL); ini_set('display.errors', 1); Don't use short tags "<?" use full tags instead "<?php" -- some servers do not support short tags. Make sure there is absolutely no output before the header() calls (like maybe from the included files). Technically, a Location header is supposed to have a full url. i.e. http://www.domain.com/apteditform.php?id=1.
-
Have a look at the mysql_error() when the connection OR the select db fails. If you care going to do it that way (in a single IF statement) it needs to be OR not AND. if(!mysql_connect($mysql_host,$mysql_user,$mysql_pass) OR !mysql_select_db($mysql_db)){ echo 'error: ' . mysql_error(); } with AND, since you are looking for failure, you only get that error condition if BOTH fail. It would appear that the connect succeeds but the select fails.
-
These two lines put text on the image. The first one, according to the comment, is adding shadow text behind the actaul text. Have a look at imagettftext in the PHP manual for an explantaion of the parameters: image resource, position and size, color, font, text.
-
Usually a column named "ID" holds a UNIQUE value that identifies ONE row (record) in the table. Generally it is an Auto-Increment value, so the server sets it on input and you never touch it. That script is assuming ID is a field of this type. There are other issues with that script, including the fact that the ID is not sent with the POST, so the updates are not going to work. Note that as it is, if ID is the PRIMARY KEY of your table, you can only ever have 1 pending recipe. When a second one is entered, it will overwrite the first, or the entry will fail. I would suggest you add a column to your table, perhaps: IsApproved BOOLEAN DEFAULT 0. Then update this column when you approve a recipe. You will have to make sure your scripts that list recipes do NOT include any that have a 0 in this column.
-
I usually do it this way: $username = (isset($_POST['username']) ? $_POST['username'] : ''); $password = (isset($_POST['password']) ? $_POST['password'] : ''); if ( (!empty($username) and (!empty($password)) ) {
-
It may be a Carriage Return ("\r") on the end of the field.
-
Using back-ticks ( ` ) to surround column and table names, is something I have only ever seen in mySql. I don't know if it is supported in any other database server. It is ONLY necessary in mySql if the column/table name matches a reserved word. I never use it unless I am forced to work with a database that I can't change, and then only when the specific column name presents a problem. I would take them out of that code and see where that leads. When working in Windows, I prefer Notepad++, myself. There are plenty of other editors that support highlighting, etc. And there are several IDE's that support debugging and so forth. I don't have any experience with any of them, so I can't make a recommendation.
-
The problem is with the way you have included variables in that string: # This is incorrect VALUES ( '($_POST['dea_number'])' # This is correct -- use curly braces VALUES ( '{$_POST['dea_number']}' You will have to change all of those embedded variables.
-
Are you trying to select the Anchor (A) or the List Element (LI)? Your original post indicated LI. What browser are you testing in? This #product_tabs ul li:last-child { says the last LI in the UL While this #product_tabs ul li a:last-child { says the last A in all of the LI's in the UL. Since there is only one anchor in all of your LI's, that would select all of the anchors in that UL If you want THE anchor in the LAST LI, try this: #product_tabs ul li:last-child a {
-
Then is it: #product_tabs ul li:last-child {