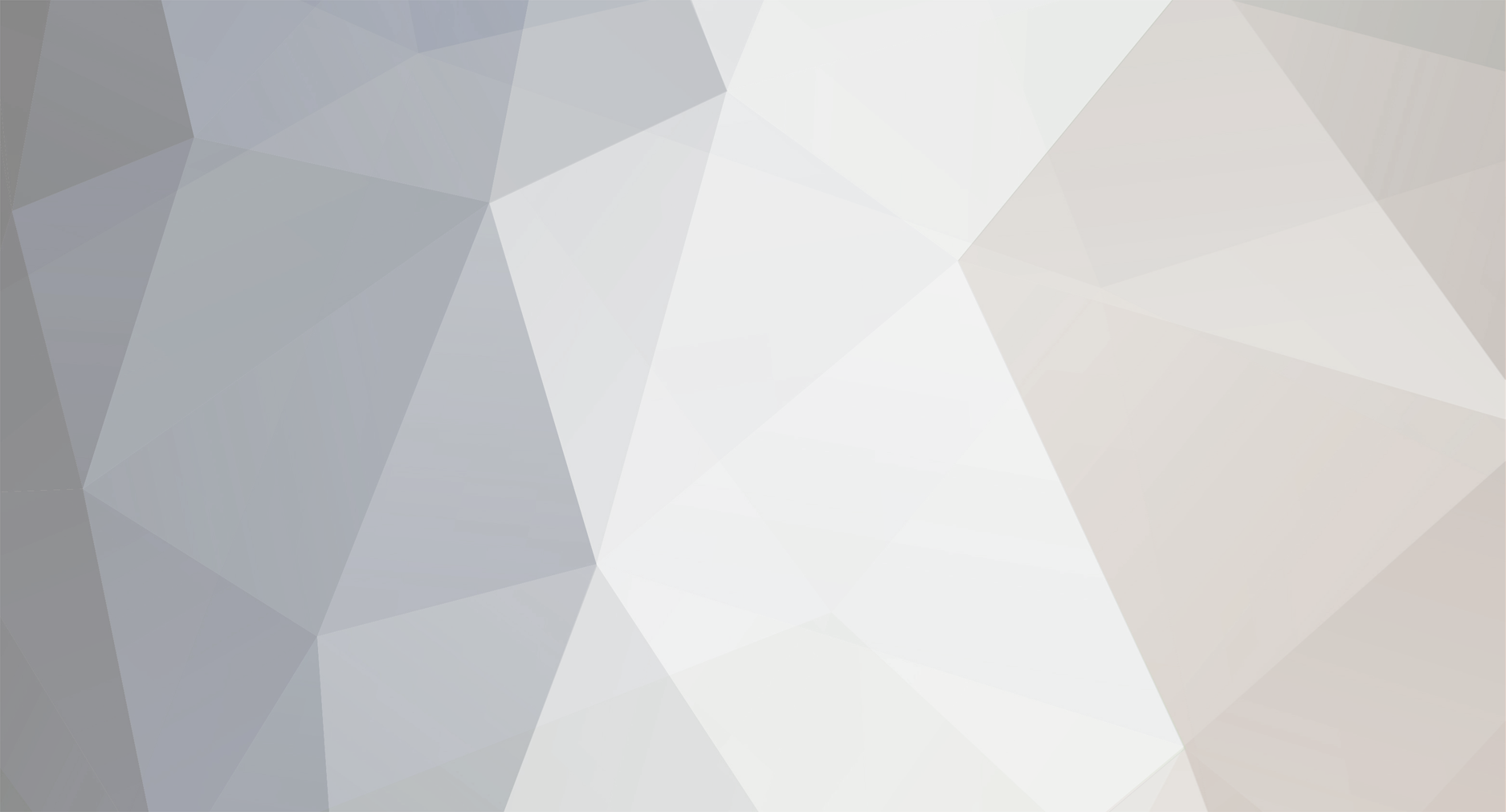
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
You are going to end up with the delimiter left at the beginning or at the end as well as doubled-up in the middle. The most effective way I see to do this is to explode the list (to an array), remove the entries (from the array), and implode the array back into a list ... Untested: $var = 'hello|goodbye|morning|evening'; $list = explode('|', $var); if ( ($ind = array_search('goodbye', $list)) !== false) unset($list[$ind]); if ( ($ind = array_search('morning', $list)) !== false) unset($list[$ind]); $var = implode('|', $list); Altough a function might be cleaner, it incurs the explode/implode overhead for each removal: function removeFrom($psList, $psEntry) { $list = explode('|', $psList); if ( ($ind = array_search($psEntry, $list)) !== false) unset($list[$ind]); return implode('|', $list); } $var = 'hello|goodbye|morning|evening'; $var = removeFrom($var, 'goodbye'); $var = removeFrom($var, 'morning');
-
There is no condition for the ON DUPLICATE KEY phrase. It will trigger if ANY unique index on the table would be duplicated. If there is no uniqueness in the data, how do you know if the data already exists? You are checking if the date exists in the table but then updating based on the route. Without any other condition on the UPDATE, you will be updating ALL records for that route regardless of the date. Your INSERT statement references a column that does not exist (or the UPDATE does) -- train_type vs. train_route. Your test to see if the data already exists is not correct. mysql_fetch_row returns an array. The comparison might work, but you are not always going to get the correct result. The recommended method is to use SELECT COUNT(*) FROM ..., then execute the query, fetch the row, and check the value of the count. You need to define what determines if the data already exists. Is there a combination of columns that define the uniqueness? If there is, then you can define a composite index and use the ON DUPLICATE KEY phrase. If not, then we need to know exactly what you are trying to accomplish so we can recommend an appropriate course of action.
-
To eliminate the error -- and in my opinion this should be considered an error, not just a notice to be hidden -- you should check to see if the GET index is set. Something like: $mid = (isset($_GET['male_id']) ? $_GET['male_id'] : null); $fid = (isset($_GET['female_id']) ? $_GET['female_id'] : null); $serid = (isset($_GET['series_id']) ? $_GET['series_id'] : null); $id = (isset($_GET['id']) ? $_GET['id'] : null); This will require you to change your IF statements, which you should do anyway since all of the variables are set now. You can use if (! empty($_GET['id'])) instead of isset() Of course, you will need to add an ELSE block for when none of them is provided (something you should have in there now, anyway). Having said that, it is NOT a good idea to allow any kind of PHP error message to be displayed on a public-facing website. This can provide hackers information you don't want them to have. If you want information about errors that have occurred, log them to a file -- use the date as part of the filename -- then have a cron job email the file to you each day if it is not empty.
-
How to pass a user's select value to a cookie and maintain it?
DavidAM replied to hitman47's topic in PHP Coding Help
session_start and setcookie are two unrelated actions. You do not need the session for the cookie (I can't tell if you are using a session for something else in the code, but you don't need it for the cookie). The problem is that you are unconditionally setting the cookie. If you come back to the page, through a link, it sends a GET request, and the $_POST array is empty, so you are setting the cookie to an empty string. You need to test for the POST value before setting the cookie: if (isset($_POST['sortid'])) setcookie('sortid', $_POST['sortid']); P.S. Please use tags when posting code. -
It can not be equal to ANYTHING and also be NULL at the same time. You need to use OR there -- and I would use empty($datecsv) instead of equal null. However, this is NOT going to solve the problem with the "bad file" since it will skip ONLY the row in the middle with the different date, the rest of the rows will be blank. If you want to process this file, you will have to capture any non-empty date column that you find, then process all the following rows as that date, until you find another non-empty date column (which you will capture and so on).
-
This is a true statement. Disabled form elements are not posted with the form. Read Only elements are posted. Keep in mind, however, that it is very simple for a visitor to change the value if they try -- the same is true for hidden elements which are posted.
-
It has become a world of glitter and glamor. If it looks good, people buy it. If it turns out to be a UI nightmare, or it doesn't work well, people will just move on to the next app. Those who do research, will never buy an app from that developer again, perhaps. First, and foremost, design and build the app -- including significant testing! Then design the "packaging", that's just marketing anyway and has nothing really to do with programming.
-
If this script is running on your server and you want to save the file on that server, there is no upload involved. You have a couple of options: 1) Instead of echoing the data directly, collect it in a string. When you are done, write the string to a file and write it to the browser (i.e. echo). OR 2) Again, do not echo the data, write it directly to a file on the server. When you are done, send the file to the client (readfile). OR 3) Use the Output buffering functions (see ob_start) to collect what you are outputting and then grab the buffer, write it to a file, and echo it as well. Unless it is a HUGE amount of data, I would choose option 1. If the file is or can be very large, I would use option 2. I do not recommend option 3; eventhough it will be the easiest to implement into what you have, it can create issues and make it difficult to debug. $files = header(...) - The header function sends a header. It does not return a value, so $files there will be null and the header will be sent. The assignment is useless. What are you trying to accomplish there? You never use $files in the code you showed.
-
You are creating a separate TABLE for each product. You need to move the TABLE tag to a place BEFORE the loop, and the closing TABLE tag to a place AFTER the loop. The TH element needs to be INSIDE a table row Your main problem is that you are setting $category to am empty string to stop it from printing. Then you assign $category to $prev_category_name to test the next iteration. Well, now the previous category is NOT equal to the next one. Do not change the category, as Barand showed, just output the heading inside the IF test if the current category does not match the previous one.
-
Caution: Older versions of I.E. (6.0) used to POST all BUTTON elements on the form regardless of which one was pressed. I'm not sure if they have fixed this issue in later versions, but it made it very difficult (i.e. impossible) to determine which button was used to submit the form. Other than that, I like the BUTTON element.
-
A list of IP ranges and actions depending on the visitor IP - how?
DavidAM replied to ionicle's topic in PHP Coding Help
If you are using a database at all, I would definitely put this in a table in the DB. If you are not using a database, I would consider using one for this. In any case I would recommend that all "single" IP addresses be stored as a range -- the start and end of the range is the same value. This way you have one "table" (or file) with a consistent record structure. With a database you could then do: SELECT COUNT(*) FROM IPTable WHERE 'visitorsIP' BETWEEN startIP AND endIPYou should get a result of zero or one -- one meaning they are in the list. You can use ip2long() if you want, but unless there are going to be a large number of them, I'm not sure I would. If you use a file, follow a few simple "rules": 1. Always store all of the addresses as 15 characters -- use leading zeros: 010.002.123.003. 2. Use a standard delimiter between the two elements of the range: space or tab 3. Always sort the file after making changes. You are going to have to process each line in the file one at a time. If you have it sorted, then once you reach a range that is greater than the visitor's address, you can stop checking because all of the remaining entries are also greater. -
Brackets are not required for simple variable names in a string. But the closing parenthesis for the VALUES clause is missing. When mysql_query fails, it will return false. You can use mysql_error to find out why. Also, it is recommended that you build the query in a variable instead of executing directly. Then you can print the query along with the error, this will help you see what is wrong. $sql = "INSERT INTO myDataBase (stringData) VALUES ('$myString'"; $res = mysql_query($sql); if ($res === false) echo 'Query Failed: ' . $sql . ' Error: ' . mysql_error();
-
Each of the methods you describe have their uses. The first method applies the settings AS THEY ARE NOW when the record is created. So the parent levels may be changed without affecting existing children. If you WANT to affect children, you have the added complication of: how can you tell if the value of a setting is, say, "4" because it was inherited or because that is what someone wants it to be? The second method keeps the settings inherited, regardless of changes to the parent levels. As to the complitcated query, I would consider creating a VIEW called "IndividualSettings" with the joins and COALESCE(). Then select from the View or JOIN to it if other data is needed from the Individual table.
-
mod_rewrite is an internal opteration (mostly). Its purpose is to let you provide people with easy to remember and SEO friendly URLs and then direct that url to your script. For instance: I can advertise a url as SeeMyStuff.com/blue/knit/sweaters then have mod_rewrite change that to www.SeeMyStuff.com/products.php?product=sweaters&type=knit&color=blue then my script (called products.php) can retrieve the parameters ($_GET['product'], etc) to build the page. The fact that you activate mod_rewrite, does NOT automatically change the URLs displayed on your pages from the second format to the first. Whatever you put in the <A href="here"> tag will be the value sent to the browser and sent to the server when the user clicks it. So you have to change all the Anchor tags to the new format (#1 above) before you send it to the user (i.e. in your code) if you want them shown in the new format. Of course, the second format will still work from links or the address bar as well. When used in this way, the URL in the address bar DOES NOT CHANGE. If the user clicks a link containing either of the urls shown above, that is what they will see in the address bar, regardless of how you rewrite it.
-
adding to an array with each recursive function
DavidAM replied to 1internet's topic in PHP Coding Help
I'm not sure what line 23 is, since you did not post your new code. But that error comes from a change in PHP. To pass a variable by reference, you used to use: $result = myFunction(&$varByReference); # See the "&" in the call to the function The "&" in the call is no longer needed. If the function is defined to accept a parameter by reference, any call to it will be by reference. So: function myFunction(&$paramByReference) { // Do something } $varByReference = null; # Or whatever value you want to start with $result = myFunction($varByReference); # Note NO "&" in the call $result = myFunction(&$varByReference); # This line produces that error -
On an UPDATE statement, you really can't leave it to the database. There may already be a value there that needs to be cleared out. You will have to build your query string dynamically. One way to do it is: $columns = array(); $columns[] = "Opposition = " . ($opposition === null ? "NULL" : "'$opposition'"); $columns[] = "match_date = " . ($fixture_date === null ? "NULL" : "'$fixture_date'"); # ... and so on $columns[] = "bonus_posting = " . ($bonus === null ? "NULL" : $bonus); $sql = "UPDATE tbl_fixtures SET " . implode(", ", $columns) . "WHERE match_id = '$match_id'"; Notes:- I don't use back-ticks. They are not needed unless the column or table name is a mySql reserved word - We can't really use empty for the tests since empty() will be true for a zero value or an empty string; so we have to use the exact comparison (three equal-signs). - You should not put quotes around numeric values in the query - There are NO quotes around the term NULL, if you put quotes around it (in the query) it will be a string of four characters.
-
adding to an array with each recursive function
DavidAM replied to 1internet's topic in PHP Coding Help
Since you are modifying the array inside the function and expecting that change to be available to the code that calls the function (recursively), you must pass the array by reference: function structure($x, &$structure = array()) { However, I think that would mean you have to pass an empty array in your initial call (I'm not sure, maybe not - I'm not real clear on omitting a reference parameter): $result = array(); structure(22, $result); var_dump($result);You are returning the array correctly from the function, but you are not capturing the returned value in the initial call. Also, you cannot echo an array. $structure = structure(22); var_dump($structure); Note: Avoid using the same name for a function as you use for a variable. It makes the code confusing: echo structure(22); Is that supposed to be echo $structure[22];? I generally try to use a verb phrase for functions (they perform actions) and a noun for variables (they name a thing). So $structure and buildStructure(). -
And always exit after a header redirect. if(!isset($_SESSION['myusername'])){ header("location:main_login.php"); exit(); }
-
You would just write another UPDATE statement with an additional JOIN to the table containing the data you need. But Do NOT do that and undo the location fix You should not de-normalize your data for your output routines. You should use a SELECT in the feed generator with the JOINs you need to get your data. If you can not modify the generator and it will only accept a table name, then create a VIEW in the database and use the view name as the table name for the generator. The generator should not be able to tell the difference. When you de-normalize your data like this, you run the risk of data getting out of sync. What if a user changes the location of a job -- maybe they fill the job, and then have a new one with the same requirements but a different location; or they spelled it wrong and call you and ask you to fix it. Now your data is out of sync. Maybe your front-end does not allow the user to change it, but what if you change the front-end later or someone changes it in the database without knowing that they have to change it in two places? Unless you have triggers on the base table to update the de-normalized tables when the data changes, your data will get out of sync and you will be searching for a bug in the application when the problem is with the data. Use a VIEW and save yourself trouble down the road. So, instead of the UPDATE statement you have, create a view: CREATE VIEW Jobs_Location_View AS SELECT *, jobs_locations.display_name as job_location FROM jobs JOIN jobs_locations ON (jobs.id = jobs_locations.job_id);
-
When you put a slash at the beginning of a filepath, it points to the root of the SYSTEM. You need to specify where that file is: So the include should be something like this: include('/home/myusername/public_html/sys/important/HTML.class.php if it is in the public path.
-
As the error says: The execute method of whatever object you are using is building an INSERT statement. But the number of columns specified in the INSERT statement; or if none are specified; the number of columns in the table; does not match the number of values provided.
-
Issues trying: Multiple LIKES && ORDER BY multiple values
DavidAM replied to justin7410's topic in MySQL Help
First: You don't need to use back-ticks for column names and table names unless the name is a mySql reserved word. I never use them as they clutter up the code and make it difficult to read (IMO). This leads to one problem in your query: `votes'. In the ORDER BY clause you started with a back-tick to surround "votes" but closed it with a single-quote. Second: When mixing AND and OR in a WHERE clause, It is best to use parenthesis to group the conditions. Your statement is actually evaluated as: WHERE `release_date` LIKE '%2013-06%' OR `release_date` LIKE '%2013-05%' OR (`release_date` LIKE '%2013-04%' AND `release_country` = 'USA' AND `is_active_member` = 1 ) Which I doubt is your intention. Try: WHERE (`release_date` LIKE '%2013-06%' OR `release_date` LIKE '%2013-05%' OR `release_date` LIKE '%2013-04%') AND `release_country` = 'USA' AND `is_active_member` = 1 Finally: If release_date is a DATE or DATETIME (or even TIMESTAMP) column, you do not need the percent symbol at the beginning of those LIKE expressions. The format of a DATE column in mySQL is: YYYY-MM-DD.- 3 replies
-
- mysql
- phpmyadmin
-
(and 3 more)
Tagged with:
-
Need help wont display database first result
DavidAM replied to davidolson's topic in PHP Coding Help
Well, then, delete that code. It's wrong anyway. ->fetch retrieves a row from the database. It does NOT retrieve the record count. So, with that code you are retrieving the first row. Then you start your loop by retrieving the NEXT row, but you have never processed the first one. Note: The reason prepared statements were invented (many years ago) was to speed up processing when running queries in a loop. But to benefit from that, you would PREPARE the statement OUTSIDE of the loop. HOWEVER, in this case (as in most cases) you can and should get all of the data in your first query using a JOIN. -
Best way to approach missing image in database
DavidAM replied to justin7410's topic in PHP Coding Help
You have already modified $id with md5() so this comparison is invalid. However, hard-coding a test like this ($id > 230467) is not generally a good idea. You will soon forget this check is buried deep in the code, and some change to the data will make it obsolete or not 100% accurate. If the image_key test is correct, use it. Better yet, add a column to the database either indicating there is or is NOT an image, or store the complete URL of the image (and NULL for none).- 11 replies
-
In general, you want to avoid running queries in a loop. It would be better to issue a single select for all of the selected IDs and then loop over the results: if(isset($_POST['submit'])) { // Since they are checkboxes, it is possible that the store_id element does not exist if (isset($_POST['store_id'])) { $store = array_map('intval', $_POST['store_id']); // Protect against invalid input $sql = 'SELECT * FROM stores WHERE id IN (' . implode(',', $store) . ')'; $res = mysql_query($sql); if ($res) { while ($row = mysql_fetch_assoc($res)){ // Process the data } } } }Assuming, of course that the store id is an integer.