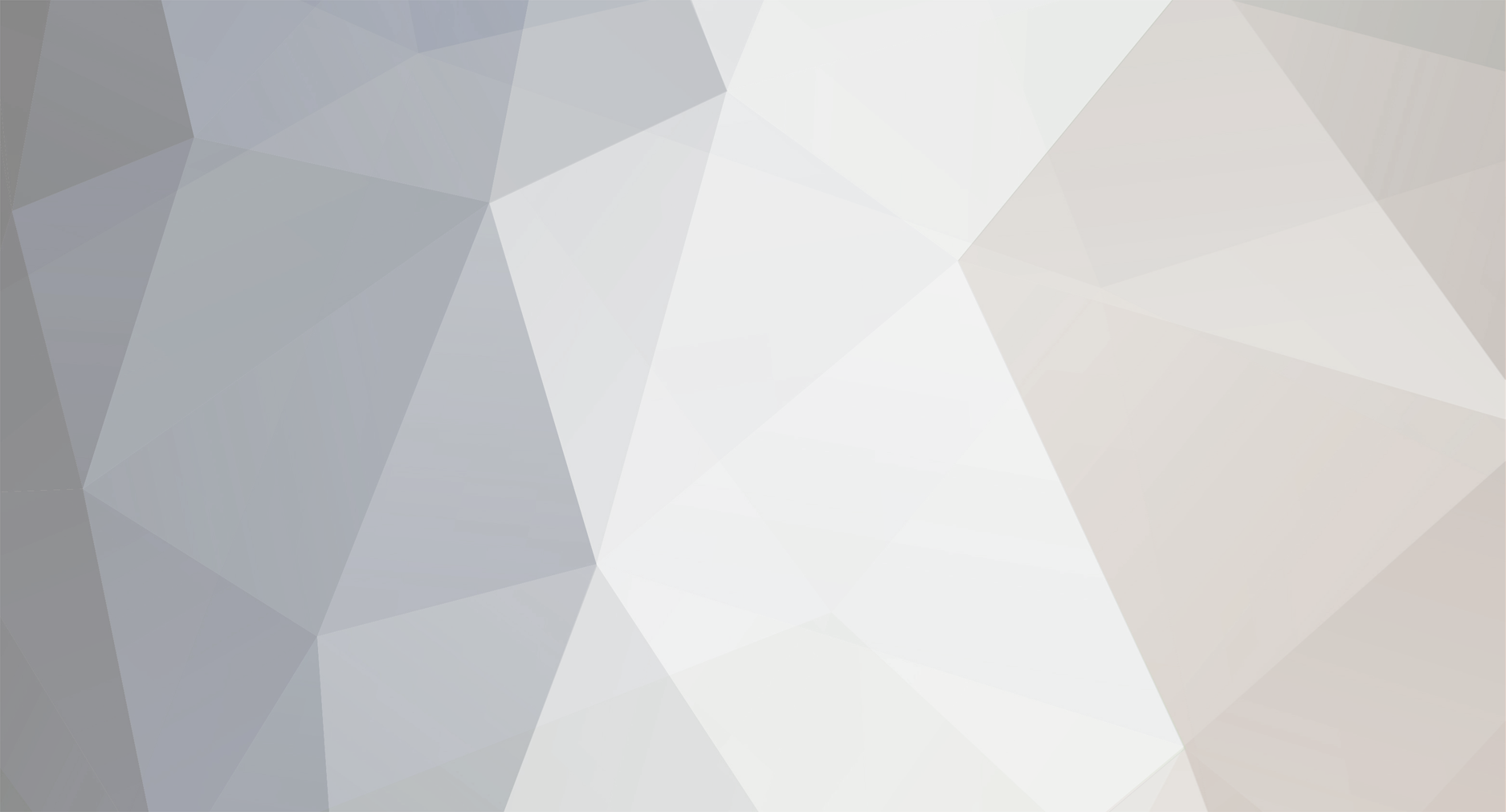
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
The Content-Length would have to be the length of the data being sent. Since he is decoding the base64 data from the database, the Content-Length, is not the length of the encoded data. So taking the length of the data in the database will be too high. You would have to do something like: $out = base64_decode($a['data']); header('Content-Length: ' . str_len($out)); echo $out; Note: I personally do not recommend storing files in a database. I would put the file in the filesystem (with a unique name), and store a path to the file in the database. Databases are designed for storing, manipulating and retrieving (structured) data.
-
You won't get a PHP message if the query is bad. But you are not testing to see if the query succeeded: if (! mysqli_query($this->Connection, $SQL)) { printf("Error: %s<BR>%s<BR>", $SQL, mysqli_error($this->Connection)); }
-
At the risk of repeating myself: I still do not see the Update() method. Forgot about the "@" operator. It hides errors it does not fix them. Use proper error reporting. Also, when a mysqli* method fails, you need to display the mysqli_error so you can see what the problem is. You did not answer my question: The second code snippet DOES HAVE single quotes in it - your post said it did not - so what are you talking about?
-
Without seeing the Update() method, we can't really tell what is happening. However, you are using single-quotes (') in the second code snippet. Were you referring to the back-ticks (`)? They are completely different puncuation and have completely different purposes. Also, what exactly does "it does not work" mean? You pobably need to output the error from the database client library. What database library are you using (mySql, mySqli, pdo, odbc)?
-
How long do you think it will take him to notice? Welcome aboard, jcbones.
-
I was born in The U.S.A., fifty-some years ago; and I'm still learning it. -- Actually, these young whipper-snappers keep changing it on us. Anyway, welcome to the club. Isn't there a rule that the new guy buys the first round? Can we call jazzman1 and jcbones appointments a tie and get two first rounds out of it?
-
Apparently the "email" column exists in both tables. You will need to qualify it in the WHERE clause with the name of the table you want to compare it to: ... WHERE users.email = '$email' -- OR ... WHERE confirm.email = '$eamil' $users_info is the reqult of a mysql_query call. It is a resource for accessing the data. You need to use mysql_fetch_assoc or one of its siblings to actually get the data.
-
Sending emails in a foreach loop. Best way to do this?
DavidAM replied to eMonk's topic in PHP Coding Help
Keep in mind, the mail specification does NOT require that the BCC list NOT be distributed. That is, the people in the TO and CC (which would be empty in your example) will NOT see the BCC list. However, the full list of BCC recipients might be shown to all BCC recipients in the list. That would then be effectively the same as putting everyone in the TO list (in this case). The "correct" way to do this is to send a separate message to each recipient, or use a true list service. -
That is an indication that there is no "item_id" parameter provided in the URL (for $_GET). That indicates (based on your query) that there is no column named id in the store_categories table Check the spelling and cAsE in both cases. If the page might be requested without an item_id, then check for it before doing the query and take some appropriate action when it is not present. Hint: Any publicly available page might be requested without the expected url parameters.
-
Not really a "quirk". When you SELECT an aggregate, you will always get a row. If the table is empty, or no rows match the WHERE clause, the "MIN" value is NULL. You need to check the value that is returned: if (empty($oldestYear)) ... Or you can add COUNT(*) to the query and see how many rows matched the WHERE clause (zero if the table is empty). Even in that case, you will get 1 row returned.
-
Building a string using double-quotes causes variables to be interpreted. So the code is trying interpret $text[] = $row[0] However, to my knowledge, you can not do an assignment inside a double-quoted string. So, the PHP engine expectes $text[] to have an array index inside the square-brackets so it can interpret the variable (and insert the value). Try using single-quotes: $fcontent = '.... Of course then, the new-lines are not going to be interpreted as newlines, they will instead be literal \n (backslash "n"). Since you don't close the quotes between lines, you don't really need them anyway (unless you want the code double-spaced). Same goes for the single-quotes inside the string: they need to be escaped or changed to double-quotes: $fcontent = '$query = \'SELECT title FROM home\'; if($result = mysqli_query($link,$query)) { $pages = mysqli_num_rows($result); while($row=mysqli_fetch_row($result)) { $text[] = $row[0]; } mysqli_free_result($result); }'; If you need (or want) the double-quotes around the string, then escape all of the dollar-signs with a backslash so PHP will not interpret it as a variable name:$fcontent = "\n\$query = 'SELECT title FROM home'; ...
-
When using PHP as the SRC attribute of an IMG tag, it can be difficult to debug. But, you can request the "image" directly in the address bar of your browser. If you have error reporting on (as you should in development), you should be able to see any error messages from PHP. Be sure you don't output the "Content-type" header until you are ready to send content. The error messages need to come out before you tell the browser it is an image or the browser will think it is a broken image. Take out that GIF header (at the beginning), you don't need it. Turn on error reporting at the beginning of your script, if it is not already on error_reporting(E_ALL); ini_set('display_errors', 1);I suspect the problem is it cannot find the font file.
-
When query() or multi_query() fails (returns false), you should have a look at the error message from the database server: echo $mysqli->error. This will explain the problem. In the code you posted, you need a semi-colon between the two queries: $res = array(); //output array $query = "SELECT COUNT(*) AS count FROM tbl_1;"; // Need a semicolon between statements in a multi-query $query .= "SELECT COUNT(*) AS count FROM tbl_2"; if($mysqli->multi_query($query)){ do { if ($result = $mysqli->store_result()) { $row = $result->fetch(); //tried this with fetch_row() and while loop $res[] = $row['count']; } else { echo "store result returned false."; } $result->free(); } while ($mysqli->more_results() && $mysqli->next_result()); } else { // Why did it fail? echo "Query Failed: " . $mysqli->error . ' -- ' . $query; } $mysqli->close(); print_r($res);
-
I am not an expert at CSS, but I have had problems with styles when there is a mixture of classes and ids involved in an HTML heirarchy. Is the DIV contained inside another HTML element? How are the containing elements styled? PHP simply writes HTML for you, it has no control on how the HTML is interpreted. If there is a problem, it is with the generated HTML, not with the fact that PHP wrote it. Use the View Source feature of the browser to check your HTML. Is the DIV shown there with the ID as it should be? Check the validity of the HTML and the CSS (at W3C QA Toolbox). Invalid markup can cause problems. Also, make sure the link for the style sheet is correct; and that the urls in the stylesheet are correct. Using relative paths, can lead to problems if the HTML is in a different directory than the style sheet. Since you are using a relative path for the HREF, it is relative to the path of the HTML url. The image paths are also relative, but they will be relative to the CSS url. I highly recommend using absolute (url) paths for all of these. If the problem persists, you might post the HTML (from View Source) showing the DIV and its containing elements, along with your CSS.
-
There is no CSS style for #my_div. And there is no HTML element with id="send_img". So the posted CSS will have no impact on the posted HTML. Note: please use tags when posting code.
-
No, you are not. Firefox is a browser for viewing websites. It does not build websites. Since it is just a viewer, it has nothing to do with the PHP code since PHP runs on the server and is done and gone before the browser ever sees it. Every server has its own set of configuration files. There is obviously something different between the two servers. You need to have error_reporting set to E_ALL and display_errors set to on in development. You also need to be sure that output_buffering is OFF. These are some of the settings that may be different between the two servers that would allow the code to "work" on one and not the other. As Psycho said, we have suggested "something" already to address the specific problems you have posted. "rectify this"? Not going to happen. Well, unless (possibly) if you pony up some money and pay someone to fix it. We are here to help you help yourself, not to do it for you. No, we can't, there are too many things we don't know about your application and database. I can tell you (some of) what is wrong with it: 1. ob_start(): Output buffering is almost never needed. It is usually (and in this case, apparently) used as a band-aid for a "headers already sent" error. You need to fix the root cause of the problem. 2. global: It is almost never a good idea or even necessary to use globals. Besides, you are using it incorrectly, and it is completely NOT needed in the code in this particular post (#17). 3. You are SELECTing everything from every row in the visitors table. Then walking through every row, and setting a scalar variable. At the end of the loop, the value ($total) will be the value from the last row. Which may or may not be the row you are trying to pull from. This is a terrible waste of resources. Select ONLY the column(s) and ONLY the row(s) that you actually need. 4. You are UPDATEing every row in the visitors table with the new total value. Is there only one row in this table, or do you need a WHERE condition on the UPDATE statement (as well as the SELECT statement)? 5. There is no session_start() at the beginning of this script. Unless this script is included from another script that has session_start(), -- which means this is not the final code (at least it is not the complete final code) -- there will be no $_SESSION available so the IF test will always be true, but the assignment will not affect any session. 6. You are using the mysql extension functions in new development. This extension has been deprecated. You should use the mysqli extension for all new development. Since this script does not output anything, I don't see how 1) it can be of any use at all; 2) it could be producing any headers errors. If this is an entire file that is included in another script, then there may need to be changes in the other file AS WELL. If this is the entire code it could be reduced to: session_start(); -- database connection code here if (!isset($_SESSION['__name__'])) { $sql = 'UPDATE visitors SET TOTAL = TOTAL + 1'; mysql_query($sql) or die('failed to update: ' . mysql_error()); $_SESSION['__name__'] = 'Guest'; }
-
True, but it is not on line 143. @Pawan_Agarwal Read the error message it tells you exactly where the error occurred and exactly where the output started (the reason for the error). That is the same "output started at" as your original post. Figure out why you have output on line 17 of index.php and fix it (or post it -in code tags- so we can help.
-
I think the problem is the way you are specifying the separator between the two variables: http://www.domain.com?Variable=value http://www.domain.com?Variable=value&Another=somethingElseTo introduce the first parameter, you use the question-mark ("?") To introduce the second (and subsequent) parameter, you use an ampersand ("&")
-
You are also going to have to escape the data. See mysql_real_escape_string. You need to escape the filename as well. Other things to consider: 1) The mysql_ extension has been depricated. New development should use the improved version: mysqli. 2) Storing the actual image in a database is generally not very beneficial.
- 4 replies
-
- mysql database
- uploading image
-
(and 2 more)
Tagged with:
-
That is not a valid Header redirect. Perhaps you need to tell us what "mystring1" actually is, and why you are urlencode'ing it. It is true that $mylink (probably) needs to be urlencode'd, but the url you are redirecting to needs to be clean. $url = 'http://mydomain.com/redirect.php?url=' . urlencode($mylink);
-
urlencode will encode the directory separators (slashes) in MM_UPLOADPATH (and in $row['picture'] if it has any subdirectories). You have to encode each component of the path individually and then piece it back together. Or use the shortcut from AbraCadaver if you are sure you will never have any other special characters. If you know MM_UPLOADPATH is clean, and $row['picture'] will not have subdirectories, you can just encode $row['picture'] before concatenation.
- 8 replies
-
- php5
- white space
-
(and 1 more)
Tagged with:
-
switch case returns partial results - logic issue?
DavidAM replied to learningcurve's topic in PHP Coding Help
Have a look at the "View Source" feature of your browser. You are missing double-quotes on several of the "value=" attributes, and you have "closing" double-quotes on some that you never put "openning" double-quotes on. Clean up your HTML and see if that solves your problems. -
substr() returns a portion of a string. If you post some code and the (entire) error message, we may be able to help. Also, you might post the value being passed IN to the function and the value it RETURNS along with telling us what you EXPECT it to return.
-
What error message? We can't really help if we don't know what the problem is. Post the error message, and the code around the line number specified in the message.