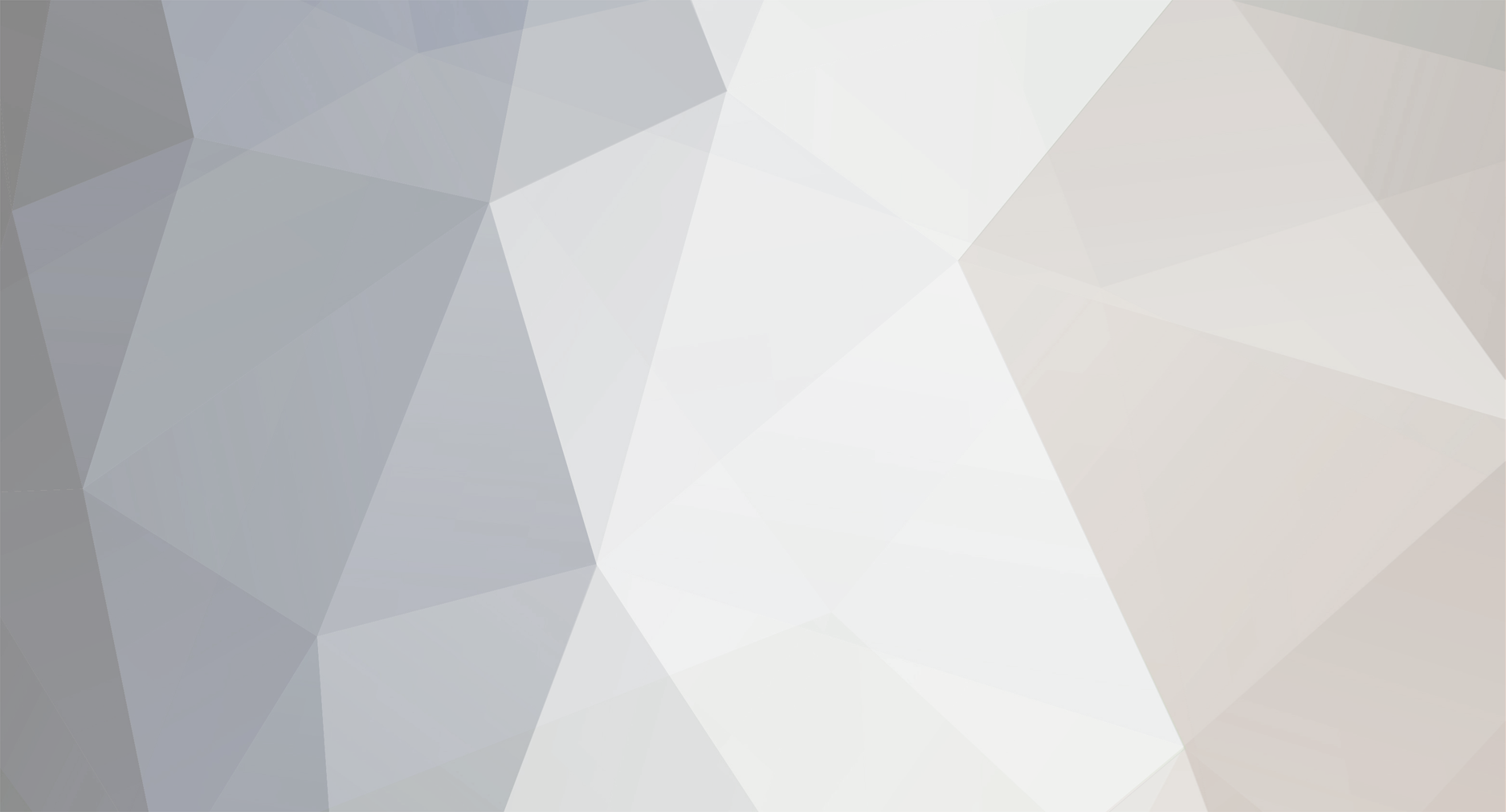
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
That error message is coming from the database server. You only sent one line of code to the server, so the error is on line 1 (of the query). The database knows nothing of your PHP script. INSERT INTO references VALUES ('','$name','$value') This should report the error on line 2
-
I think this is saying: "I currently (already) have 542,888 bytes allocated, and I tried to allocate 804,524,032 bytes. But there's not that much memory available." -- or something to that affect. As PFMaBiSmAd said, there is a limit on the size of the packets when sending data to the database. If you insist on storing very large files in the database, you are going to have to look into reading chunks from the filesystem and writing chunks to the database. Reading is straightforward: $fp = fopen("filename"); while ( !feof( $fp ) ) { $buffer = fread( $fp, 1024 ); // 1024 bytes or whatever size you want/can // send chunk to database } fclose($fp); I'm not sure how to send chunks to a BLOB in the database, but I am pretty sure there is a way to do it. However, I too, would stop and ask myself if it is necessary to save the file in the database. There may be a reason to do so, but if not, it would likely be best to store the file in the filesystem and store the path and filename in the database.
-
Glad to hear it works. Your GetSQLValueString() function may be returning the string with the quotes already around it. I use one like that as well.
-
mysql_query("DELETE FROM events WHERE 'date' < CURDATE()"); If "date" were a reserved word (as I had thought) you need back-ticks, NOT quotes mysql_query("DELETE FROM events WHERE `date` < CURDATE()"); Since "date" is NOT a reserved word, you can do it without mysql_query("DELETE FROM events WHERE date < CURDATE()");
-
sprintf("SELECT * FROM hotels WHERE est_town LIKE %s AND featured = 'N' AND premier = 'N'", GetSQLValueString($searchterm_standard_listing, "text")) "%s" is a placeholder that is replaced by the value (in this case returned by "GetSQLValueStrin()" Your proposed SQL code is not valid WHERE est_town OR est_county OR est_postcode LIKE ........ You would have to use WHERE est_town LIKE ... OR est_county LIKE ... OR est_postcode LIKE ... To do that with sprintf() you can use numbered placeholders: sprintf("SELECT * FROM hotels WHERE (est_town LIKE '%1\$s' OR est_county LIKE '%1\$s' OR est_postcode LIKE '%1\$s') AND featured = 'N' AND premier = 'N'", GetSQLValueString($searchterm_standard_listing, "text")) which will put the first value in each "%1$s" placeholder. Take note of the parenthesis to separate the OR's from the AND's; and the backslashes to keep PHP from trying to interpret the "$s" as a variable. Also, note that you have to use wildcards to make the LIKE effective. The "%" symbol will match any number of characters. So you would use "%me%' to match the term "me" with anything before it and/or anything after it. Also, the term needs to be in quotes. Without seeing the GetSQLValueString() definition, we can't tell if it is returning the "%" and/or the quotes. The final SQL would look something like: SELECT * FROM hotels WHERE (est_town LIKE '%here%' OR est_county LIKE '%here%' OR est_postcode LIKE '%here%') AND featured = 'N' AND premier = 'N' Using LIKE with a "%" at the beginning of the term will NOT allow the query to use an index. So it will have to scan every row in the table. Using LIKE without any "%" is useless and would be better written as "=" (instead of LIKE).
-
That indicates you have a column defined with a unique index. You are providing the value "127" for that column, but there is already a row with that value in that column. It looks like your "id" column is defined as AUTO INCREMENT. Is that column also defined as TINYINT? A tinyint is one byte which can range from -128 to +127. If you make it UNSIGNED you could go from 0 to 255. You may need to make that column a larger integer type and, for AUTO INCREMENT, you may as well use UNSIGNED since the system will never assign a negative number you may as well use the extra values allowed.
-
I figured the userid would be unique. It would not be much use otherwise. The COUNT(userid) provides a count of the rows that satisfy the WHERE clause. You can use COUNT(*) if it makes you more comfortable. I prefer to count the primary key column because I believe it is more efficient. I have no proof that it is, so don't ask me to provide my sources. I just figure it is -- or can be. If that is not what you were referring to, please elaborate on why you think my solution is not correct. Or how it failed when you tried it.
-
Wow, you learn something new every day! I'd go check, but I always have trouble finding the "reserved words" list in the mySql documentation. How can it not be reserved when it is the name of a datatype? Makes no sense to me. In any case, I would avoid using it as a column name (or table name or database name).
-
Count rows where one column or the other (or both) is NULL SELECT COUNT(userid) FROM fishing WHERE fav_fish IS NULL OR fav_lake IS NULL Count rows where BOTH columns are NULL SELECT COUNT(userid) FROM fishing WHERE fav_fish IS NULL AND fav_lake IS NULL Of course, I don't think I would write the row if neither value was provided, so the query to count users who do not have a fishing record could be (depending on the name of your users table): SELECT COUNT(users.userid) FROM users LEFT JOIN fishing ON users.userid = fishing.userid WHERE fishing.userid IS NULL
-
When comparing the STRING representation of dates, you have to build the string starting with the largest component: i.e. Year, Month, Day, Hour, Minute, Second. $today = date("dmY"); $vLongDate = date("dmY", strtotime($thisDate)); if ($today > $vLongDate) { Using Day, Month, Year; you end up with: 02092011 (Sep 2, 2011) which (as a string) is LESS than 03011900 (Jan 3, 1900). Using Year, Month, Day; it would be 20110902 and 19000103, which would compare safely as a string. Also, your delete logic is deleting all records with an ID less than the current ID (AND NOT EQUAL TO IT), when you have confirmed the date is LESS. You really should ONLY be deleting the ONE ROW that you have tested. However, don't waste resources and time doing this in PHP. Make sure your "date" column is a DATE or DATETIME datatype, then remove the loop and just use a SINGLE query: DELETE FROM events WHERE `date` < CURDATE() Note: Date is a reserved word in SQL so we have to include it in backticks. I highly recommend you rename that column to avoid confusion and problems.
-
1) There is a "SOLVED" button at the bottom of the page. Use it to mark the topic as solved. 2) You should start a new topic for a new question - especially since fwrite() has nothing to do with "String between characters" How are you looking at the results of your fwrite(). If you are viewing it in the browser, the browser changes a newline into a space. Use the View Source feature of the browser to see the newlines or look at the original file. If this is not the problem, we will probably have to see some code.
-
1) Don't use short tags "<?", always use the full open tag "<?php". 2) It looks like the code depends on registered_globals being on. This has been deprecated and off by default for a long time. It is a security problem. $REMOTE_ADDR should be $_SERVER['REMOTE_ADDR'] -- same with HTTP_USER_AGENT $action is probably a form field, so I guess it should be $_POST['action'] -- same with $fname, $vemail, $comments 3) Turn on error reporting, and fix the errors/warnings and notices. error_reporting(E_ALL); ini_set("display_errors", 1);
-
Rather than use a couple of IF statements listing out 16 or's, you can use the modulus operator with a little math. date_default_timezone_set('Etc/GMT-1'); $hour = date("H"); // Current Hour // If the hour is NOT a "3" hour, increase it to the next "3" hour if ($hour % 3) $hour += 3 - ($hour % 3); // The math turns it into an integer, so make sure it is 2-digits if ($hour < 10) $hour = '0' . $hour; // Get the date and tack the hour on the end $today = date("Ymd") . $hour; $pattern = '/WaveHeight_'.$today.'_mic.png'; The % is the modulus operator. It returns the remainder when (in this case) $hour is divided by 3. The if condition will always be zero -- false -- at 0, 3, 6, 9, 12, 15, 18, 21. At the other hours, we add 3 to move forward then subtract the modulus value, which leaves us with a "3" hour. Since we have done integer math on the $hour we have to turn it back into a 2-digit string if the value is less than ten. Originally, I thought you were looking for the most recently PAST "3" hour, but then I checked the site and they do have FUTURE maps. If you wanted the most recently PAST "3" hour we change one line of code (well, two if you count the comment) #// If the hour is NOT a "3" hour, increase it to the next "3" hour #if ($hour % 3) $hour += 3 - ($hour % 3); // If the hour is NOT a "3" hour, subtract the modulus to get the last "3" hour $hour -= ($hour % 3); // Use to get the hour in the PAST we don't need an IF here, since any 3 hour modulus 3 returns zero and we are just subtracting zero from the hour.
-
No, you just use the get_instance static method, it returns an object instance: $config_root = 'config' . DS . 'config.ini'; $document = new Document; $config = $document->read($config_root); $registry = Registry::get_instance(); $registry->config = $config;
-
In your Index you are using new to instantiate a Registry object. Then you assign the $config to a property of this new object. Later (in Model) you are calling the get_instance static method. This method is checking the static property $_instance. THIS PROPERTY IS NULL, so it creates another instance of the object which DOES NOT CONTAIN a value for the config property that you assigned TO THE OTHER INSTANCE. I suspect you should be using get_instance in the Index file instead of new. When I create a class that is intended to be a singleton (which is what it looks like you are trying to do), I usually add private function __construct() { } to the class. Using private should prevent the use of the new operator outside of the class itself. In other words, your get_instance will still be able to instantiate the object, but other code will NOT.
-
Using a separate table is the proper way to do it. It does not make the database un-tidy; it makes it normalized. You are not going to find an efficient or accurate way to query the tags if they are stored in a delimited field that way. Once they are in a separate table, it is very simple to determine the number of posts associated with each tag. As it is, you will have to read every post in the database to get a tag count, and you will have to read every post in the database to find all posts associated with a specific tag. This will put an unnecessary load on the database. Having a normalized database will actually result in less of a load when looking for tag counts, or posts associated with a tag, or any other query against the tags.
-
Can you add a query string in the .htaccess file? I don't know if it is supported or not and I don't have access to an environment here to try it. ErrorDocument 404 /errorDoc.php?status=404 ErrorDocument 401 /errorDoc.php?status=401 Also, when using mod_rewrite, I notice that there are additional keys in the $_SERVER array indicating the original request and the redirected request. You might try looking at what is in $_SERVER when you reach the error page.
-
Is it possible to hold MySQL commit over dialogs?
DavidAM replied to jayteepics's topic in PHP Coding Help
When a script finishes, all database connections are closed. When a database connection is closed, any un-committed transactions are rolled-back. So you cannot carry a transaction from one page to the next. Even if you could, with persistent connections (which I doubt), you do NOT want to do it. Consider this: A guy gets to the payment page and his wife screams, he runs to the kitchen to put out a grease fire, calms his wife down, cleans up the kitchen, calls the alarm company to tell them the house is NOT on fire, opens the windows, drags in the fans, airs out the room, calms his wife down, calls domino's (cause dinner is ruined), ... All this time your script is holding a transaction open. During a transaction, the user has locks on the table. These locks can prevent other users from updating the database. So this one guy has effectively shutdown your store. I would suggest completing the order in the database with a flag that indicates NOT PAID. Then have the payment process update this flag as part of its processing. You can setup a cron job to delete unpaid-old orders every night. Of course, your other processes may have to take this flag into account when considering inventory available, etc. -
mysql_query returns a resource, you have to fetch the data using the resource: mysql_select_db($database_uploader, $uploader); $totalHits = mysql_query("SELECT SUM(hits) AS totalHits FROM userDataTracking") or die(mysql_error()); // Add this line to fetch the data $row = mysql_fetch_row($totalHits); mysql_close($con); // Access the data from the array echo $row[0];
-
Explode accepts an optional third parameter to specify the maximum number of elements to return: $str = 'John Smith Wilson'; if( ($pos = strpos($str,' ')) !== FALSE ) { list( $first, $last ) = explode( ' ', $str , 2 ); // WE ONLY WANT TWO NAME PARTS echo "'$first' '$last'"; } else echo $str;
-
Appears to be a hash string. It appears that each pair of characters in that string represent a single byte (in hexadecimal). For instance: "36" is the hexadecimal representation for the character "6"; and 0x72 represents a lower-case "R". These are the first two values in your second example. The parts of the (second) string that start with "%" are, apparently, urlencoded representations of the rest of the string. I'm not explaining this very well ... but, if you pack the first string back into a raw-digest, and then urlencode that value, you get your second value: $hash = '3672E428DFE497D9DC10B054D2A49EF9F4CE010B'; $res = '6r%E4%28%DF%E4%97%D9%DC%10%B0T%D2%A4%9E%F9%F4%CE%01%0B'; $p = pack('H*', $hash); print(urlencode($p) . PHP_EOL); print($res . PHP_EOL); // prints the exact value in your second string: 6r%E4%28%DF%E4%97%D9%DC%10%B0T%D2%A4%9E%F9%F4%CE%01%0B // OR GO THE OTHER WAY $u = unpack('H*', urldecode($res)); print($u[1] . PHP_EOL); print($hash . PHP_EOL); // prints the exact value in your first string (well, in lowercase): 3672e428dfe497d9dc10b054d2a49ef9f4ce010b
-
You need to define $i outside of the loop. (show more code if you need help figuring out where) Consider this: $i++; is the same as $i = $i + 1; In this case, the "$i =" is not evaluated until after the "$i + 1" is evaluated, and at that point, $i has not been defined If you wrote: $a = $b + 1; Your error message is $b is undefined. Yes, "$a = ..." will define $a, but $b is not defined.
-
Contact Form From Hell – Help Needed
DavidAM replied to ceilingwalker's topic in Third Party Scripts
I really don't know how to answer that question. It would depend on what you are comfortable with and what your level of expertise is. In your original post, you stated: But there is no <FORM> tag in the code. I do not see any way that the form is submitting to your PHP code without a FORM tag. So, I don't know if that is all of the code, or most of the code, or what. And, I really cannot say whether the code is salvageable or not. If your code is executing and saying it sent the email, and you do not receive the email, there are a few things to look at there: 1) the mail function simply submits the mail to the (local) mail server. If it hands it off OK the function returns true. So, you need to make sure you have a working mail system installed. 2) The email address supplied in the FROM header really needs to be an email address that belongs to the server sending the mail. Otherwise, the server sending the mail may think you are trying to send a forged email and refuse to SEND it. Also, the server receiving the mail may believe it is forged and refuse to deliver it. (or it might be in your junk mail folder). -
If you have control of the database and this is an important piece of the application, I would consider the following: Add a column to the table called partnumber_search, use the same datatype as partnumber, consider indexing this column (NOT UNIQUE) Decide on a strategy: numbers to letters or letters to numbers (I would probably use numbers to letters) Write an SQL function to translate a partnumber to the value it would have if all numbers were converted to their potential mistaken letters (or vice-versa if you choose the letters to numbers option) Update the table setting the new column from the partnumber using the function When searching for a partnumber, search the new field using this function against the user's input Possibly add to the start of the ORDER clause IF(partnumber = userinput,0,1) - so exact matches appear first in the list Revise your INSERTS/UPDATES (or use a trigger) to set the new column's value You have to consider if the additional disk space required for this "solution" offsets the additional processing of trying to calculate and query EVERY conceivable permutation of the user's input or missing some potential rows In building the function for translating the input, I would ignore the case of the entered letters. In fact, I would convert the input value to uppercase and then translate (so "1" would become "L" not "l"). This will account for the situation where the user thinks it is an "l" (lowercase "L") but enters "L" (uppercase "L") because that's the way they think.
-
Dynamic Form with Database Values FRIEND SYSTEM
DavidAM replied to JohnnyKennedy's topic in PHP Coding Help
First, your WHERE clause is not quite right. Although, it may work (especially with limited data). Your query essentially says: WHERE `usera`='$me' AND (`confirmed`='$one' OR `userb`='$me') AND `confirmed`='$one' You need to wrap the two separate conditions in parenthesis WHERE (`usera`='$me' AND `confirmed`='$one') OR (`userb`='$me' AND `confirmed`='$one') Second, you are not looping through the results, so you are only getting one result. Something like this would give you all results: $buddy = mysql_query($query_buddy, $NewConnection) or die(mysql_error()); $totalRows_buddy = mysql_num_rows($buddy); while ($row_buddy = mysql_fetch_assoc($buddy)) { // Do something with the results echo $row_buddy['usera'], $row_buddy['userb']; } To echo only the friend that is NOT the user, you can use a conditional in PHP: echo ($row_boddy['usera'] == $me ? $row_buddy['userb'] : $row_buddy['usera']); or you can have the query return the "other" person: SELECT *, IF (usera = '$me', userb, usera) AS Other FROM buddies WHERE '$me' IN (usera, userb) AND confirmed='$one'