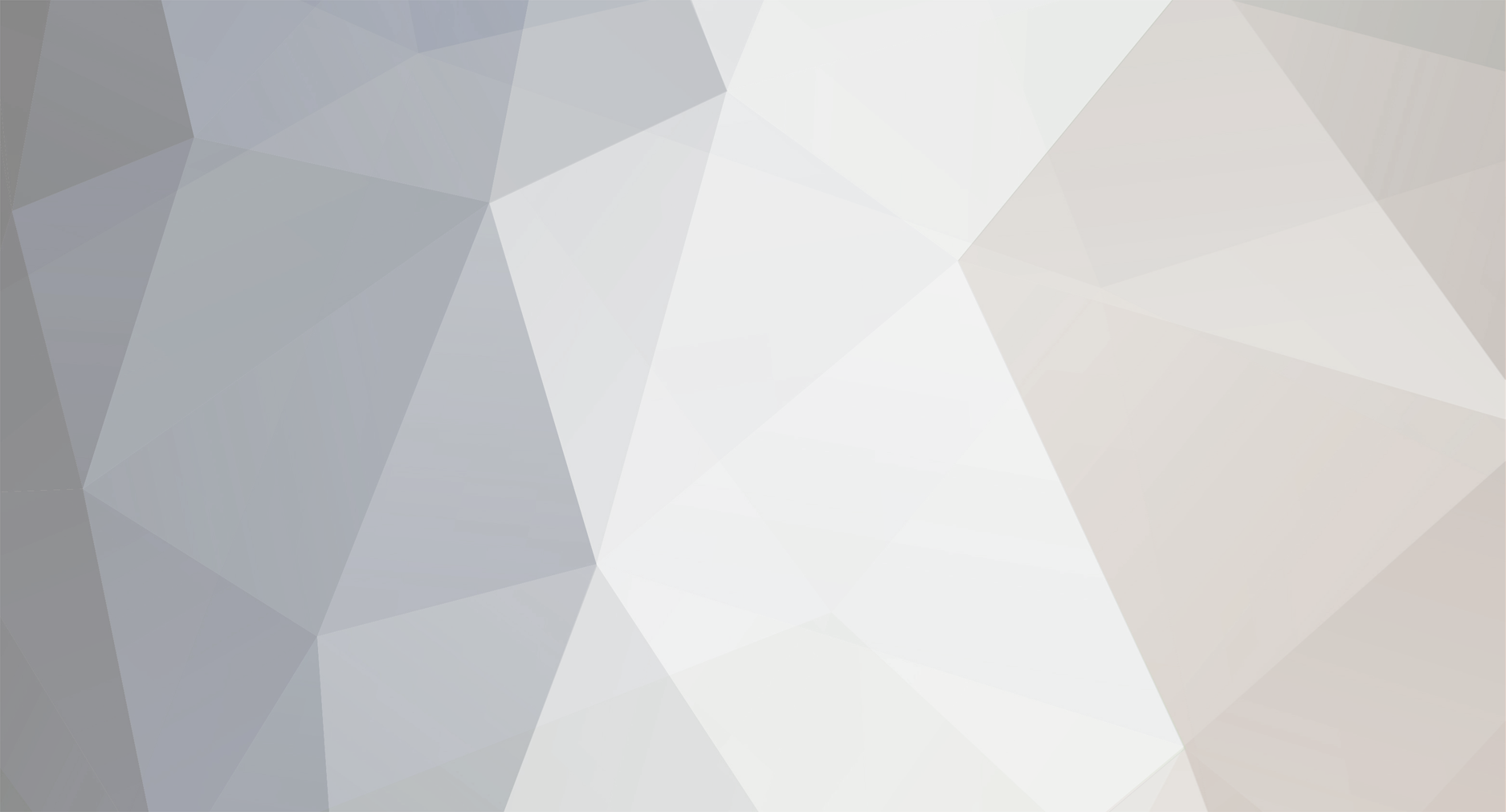
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
Pass the array as a reference so that any changes you make are made to the original copy. function readImportFiles($taxonomyFile, $target_path, $fileName='', &$ArrayOfTaxonomies = array()) // << Pass array as reference { while(!feof($taxonomyFile)) { $line = fgets($taxonomyFile); //code for getting the $schemaLocation if ($schemaLocation && !in_array($schemaLocation, $ArrayOfTaxonomies)) { $ArrayOfTaxonomies[]= $schemaLocation; $importSchema = fopen($schemaLocation, "r"); $target_path = substr($schemaLocation, 0, (strlen($schemaLocation) - strlen(strrchr($schemaLocation, "/")))); readImportFiles($importSchema, $target_path, $schemaLocation, $ArrayOfTaxonomies); } } }
-
Add the hyphen to the list of allowed characters (between the brackets). Since the hyphen has special meaning inside the square brackets, it will have to be escaped or added at the beginning or end: $new_string = preg_replace('/ [a-zA-Z-]+\.\.\./', '', $string); Now, to answer your next question (before you ask it): what about "words" with numbers in them? Add the numbers, or we can change the original suggestion to just catch everything except a space ... $new_string = preg_replace('/ [a-zA-Z0-9-]+\.\.\./', '', $string); // A-Z (upper or lowercase), numbers and hyphen $new_string = preg_replace('/ [^ ]+\.\.\./', '', $string); // Anything that is not a space. In every case, since we are using the "+" qualifier, you will not be getting rid of ellipses that immediately follow a space. If you change the "+" to an "*", you should cover that case as well. Note: This is NOT anchored at the end of the string, if there is something... in the middle of the string, it will go away, too. To anchor it at the end of the string add a "$" to the end of the regexp: $new_string = preg_replace('/ [^ ]+\.\.\.$/', '', $string);
-
Using the MYSQLI Object fetch_assoc() problem...
DavidAM replied to Eggzorcist's topic in PHP Coding Help
Your problem stems from the fact that the query is failing because you do not have quotes around the username. The DB Server is trying to compare the value in the column named "username" with the value in the column named "jaipai", which is (probably) NOT a column. You should always check to see if the query succeeded. In this case, $results will be false. Also, I don't think that fetch_assoc() is a method of the database object, I think it is a method of the result-set object. Of course, I have not used mysqli much, so I could be wrong. I think the correct code for that method would be more like this: public function pageInfo() { $query = "SELECT * FROM users WHERE username = 'jaipai'"; $results = $this->db->query($query); if (! $results) return false; // OR return something else, like: "*UNKNOWN USER*" $result = $results->fetch_assoc(); return $result['username']; } -
You have run htmlentities() on the value from the database and stored it in the array. Then later on: You are using the array value in the query. Since there are Swedish characters in the strname, they have been converted to htmlentities and the value no longer matches the value in the database. Only use htmlentities() when you are send the string to the page. In the script you should always work with the raw value.
-
You need to understand what that statement is saying. "OR DIE" is NOT a construct of PHP. It is TWO operations: OR -- is a logical operator DIE() -- is a language construct. The "OR DIE" sequence of constructs works, because PHP "short-circuits" conditionals. What that statement says is to evaluate mysql_num_rows($qCheckUser), if it is TRUE (i.e. NOT zero or anything else that PHP considers to be FALSE), then assign the value to $total2 -- OR if it is NOT TRUE (anything that PHP considers to be FALSE) evaluate the die() call. Since an OR will be TRUE if either expression is TRUE, the DIE() is not executed when the first expression is NOT FALSE. However, in this case, when mysql_num_rows() returns zero because there are zero rows, PHP considers it false and executes the die() function. Instead of using "OR DIE" you should just do the assignment and follow-up with an IF test $total2 = mysql_num_rows($qCheckUser); if ($total2 < 1) { // WE DID NOT FIND ANY ROWS // DO WHATEVER YOU NEED TO DO WHEN THERE ARE NO ROWS } else { // DO WHATEVER YOU DO WHEN YOU DO FIND ROWS }
-
Drongo_III - At some point someone suggested changing your method to this: function check_input($name) { $data = trim($name); $data = stripslashes($name); $data = htmlspecialchars($name); return $name; } If you take a close look at it, that method DOES NOTHING. It returns EXACTLY WHAT WAS PASSED IN. All of the operations in it are performed against $name and assigned to $data, but $name is returned UNMODIFIED. Make sure you fix this method. Your original method was fine - I don't know why it was suggested to change the parameter name from $data to $name, but you either need to change all of the $name variables to $data or change all of the $data variables to $name.
-
You will have to use a couple of LEFT JOINS. SELECT whatever FROM Videos LEFT JOIN Themes ON Videos.ThemeID = Themes.ThemID LEFT JOIN Categories ON Videos.CategoryID = Categories.CategoryID By the way, in the database world, you should assign NULL to a column that is not being used (CategoryID or ThemeID) instead of zero. That would mean that you need to allow NULLS in the table definition.
-
If you store it in the database that way, you can not let the user edit their post. The BBcode will be gone. If you want to allow the user to edit the post, you should store it in the database (using mysql_real_escape_string()). Then use your BBcode Formatter and htmlentities() when you want to display it.
-
"Call to a member function ... on a non-object" means the object you used to call the method is NOT actually an object. In your case, $test_stmt is NOT an object. This means the call to $db_connection2->prepare() failed to return an object, which implies that there is something wrong with the query string. As far as I know, the parameters you want to bind to are indicated by a "?" -- of course, I don't use prepared statements much, so I'm not 100% sure, but I don't think ":tst: is a valid parameter for binding. Also, you are binding a variable that you do not define until AFTER the bind. I don't know if this works or not, but I would define the variable BEFORE binding it. By the way, I don't know any reason that you need two database connections, unless they are separate servers. I would think you could do all of this on a single connection.
-
When I started using PHP, I used echo. But lately, I've been using print() for most output. Mostly because I now lean heavily on printf and sprintf, which makes it easier to see what data is being injected into the print string.
-
Use strpos $id = 1234-54678; if (strpos($id, '-') !== false) { list($id1, $id2) = explode('-', $id); } else { $id1 = $id; } Note the two equal-signs in the IF statement. strpos will return FALSE if the delimiter is not found; but it may also return zero (which would evaluate to false using !=) if the delimiter is at the beginning of the string.
-
You have an extra set of single-quotes at the start of the second row. $outputtable = '<tr>' . '<td>ID#: </td>' . '<td><input type="text" name="IDa" value="' . $idas . '" readonly="readonly"/></td>' . '</tr>' . '''<tr>' . // <<RIGHT HERE But must likely the problem is that you don't have a table tag. You need to start a table with <TABLE> and end it with </TABLE>.
-
You are defining the field as an array, but then referencing it as if it were a string. Without seeing the rest of your code, I suspect you need to reference the first element of the array: $IDa = $_POST['checkbox'][0]; or change the checkbox so it does not create an array field: <input type="checkbox" name="checkbox" value="' . $row['IDa'] . '"/>
-
The same logic applies to your UPDATE statement. The WHERE clause of the UPDATE should basically be the same as the SELECT.
-
You need to determine something that makes a row unique. How do you know if a row is changed or if it was added? Your select statement is looking for a row that matches ALL of the CURRENT values (which might have changed). So, if someone changes the description for item number 4, your select will NOT find the row and will insert it. If you choose something that is unique -- I would guess the itemNumber -- then select JUST on the item number, you can do the INSERT or UPDATE based on whether you find it or not.
-
I'm guessing that is not the full HTML, but 1) You are starting a new TABLE for each row, but you never close out any of them. I count 4 tables being started and never ended. 2) You have a break <BR /> outside of a row </TR>, so it is NOT in a cell, it is not valid markup, and is probably screwing up the layout. 3) This code snippet is also closing 2 DIVs that were never openned. Clean up the process that generates the main table. If you are still having problems. Request the page in your browser, use the View Source command, copy and paste the HTML here, along with a description of the problem.
-
You moved the building of the SQL inside the loop, but you left the execution of the query (mysql_query()) outside the loop. So it is only executing the last query. Or you can use Pikachu's or Paul's suggestion and build a single query to execute after the loop is done. (I don't belong to the "NEVER run a query in a loop" club - I believe there are times when it is appropriate. Since I don't know your application or the importance of this data, or what else your application is doing; I can't really advise other than to say, if you run the separate queries in the loop, you need to capture the errors and let the user know which one(s) (if any) failed.) By the way, your echo statement says "$row record added", but I don't see $row defined anywhere. So, unless it is defined elsewhere, it will be blank.
-
1) Is $_SESSION['id'] REALLY an ARRAY? You assign it to $id, and insert it as the user_id, so I'm suspecting it is NOT an array, but a scalar value containing the current user's ID. But you are using count($id) to determine how many entries are in the POSTed array. I suspect you should be counting one of the actual arrays. 2) Your INSERT statement is OUTSIDE of your FOR loop, so it is only being executed AFTER the last pass of the loop, which means you are only INSERTing the last element of the array.
-
Or, if you really want the zeros: SELECT source, destination, SUM(IF(g1.group_id = g2.group_id,0,CallDuration)) as total_duration FROM calls_table JOIN group_table as g1 ON calls_table.Source = g1.number JOIN group_table as g2 ON calls_table.Destination = g2.number GROUP BY source, duration
-
Do you have the Groups defined in a database table? And if so, can you post the table structure?
-
Try your var_dump tests inside the functions again but DO NOT put the var_dump BEFORE the global command. I suspect that calling var_dump($db) BEFORE global, defines a new variable called $db because you have referenced it. Since you are "defining" it without assigning a value it is NULL. Leave the var_dump AFTER the global, and see what it tells us.
-
Trouble getting checkbox on form uploaded to SQL
DavidAM replied to Dave2136's topic in PHP Coding Help
That code uses a variable called $choice, but ONLY inside the loop. It is used to hold the value from each checkbox, in turn, for only a moment. We use that value to update the array -- $whatDidTheUserCheck -- with TRUE for those boxes that the user checked. Since you have never provided any comments or code indicating how you want to use these values, we have not provided any suggestions on how to do it. There are many, many, many, different ways to use the value(s). Another way to do it, and I'm sure this is not the best way: $userCheckedA = false; $userCheckedB = false; $userCheckedC = false; if (isset($_POST["formcheck"])) { foreach($_POST["formcheck"] as $choice) { if ($choice == 'A') $userCheckedA = true; if ($choice == 'B') $userCheckedB = true; if ($choice == 'C') $userCheckedC = true; } } else { // None of the boxes was checked } -
Save the extra steps: $query = $DB->Execute("SELECT * FROM `config`"); $result = $query->getArray(); $config = array(); foreach($result as $row) { $config[$row['name']] = $row['value']; }
-
Trouble getting checkbox on form uploaded to SQL
DavidAM replied to Dave2136's topic in PHP Coding Help
Your (original) input fields: <input type="checkbox" name="formcheck[]" value="A" /> <input type="checkbox" name="formcheck[]" value="B" /> <input type="checkbox" name="formcheck[]" value="C" /> Will allow zero, one or more boxes to be checked. If one or more are checked, an array is posted to your script. If the user checked "A" and "B", the array would be: $_POST['formcheck'][0] = 'A'; $_POST['formcheck'][1] = 'B' If "B" and "C" were checked it would be: $_POST['formcheck'][0] = 'B'; $_POST['formcheck'][1] = 'C' So, the code I posted, walks through this array looking at the values checked. To make is clearer: $whatDidTheUserCheck = array('A' => false, 'B' => false, 'C' => false); if (isset($_POST["formcheck"])) { foreach($_POST["formcheck"] as $choice) { // At this point, $choice will be A or B or C // So do what you want with it $whatDidTheUserCheck[$choice] = true; } } else { // None of the boxes was checked } // This will give you a DEBUG dump of what the user checked var_dump($whatDidTheUserCheck); Just to be clear, Browsers do NOT post checkboxes that are NOT checked. So, the number of elements in the $_POST['formcheck'] array will be the number of boxes checked. As in my examples above, if the user did NOT check box "C", you will NOT get a field POSTed for "C". That is the reason I have the first IF in there -- if the user does NOT check ANY boxes, the 'formcheck' element of the POST array will NOT even exist. -
The errors you are getting have nothing to do with the database. That is PHP telling you that you have not defined the variables: $first_name, $last_name, and $log_number. They are not defined because your IF tests are based on ALL THREE having been passed from the form. But there is no INPUT field on the form called log_number, so your if (isset($_POST['log_number']) && isset($_POST['first_name']) && isset($_POST['last_name']) ) { is never true, so NONE of the fields are defined. Then you test for the SUBMIT button to generate the UPDATE query. Well, the submit button exists, so you try to create the query and you can't because those fields are not defined. Since your UPDATE depends on the value of log_number, you need to get that value from somewhere. It looks like you expected it to be on the form, so maybe you need to look at the code that generates the form and fix it to add the log_number.